
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
libraries.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from tools.paths import MBED_LIBRARIES,\ 00018 MBED_RPC, RPC_LIBRARY, USB, USB_LIBRARIES, \ 00019 DSP_ABSTRACTION, DSP_CMSIS, DSP_LIBRARIES,\ 00020 CPPUTEST_SRC,\ 00021 CPPUTEST_PLATFORM_SRC, CPPUTEST_TESTRUNNER_SCR, CPPUTEST_LIBRARY,\ 00022 CPPUTEST_INC, CPPUTEST_PLATFORM_INC, CPPUTEST_TESTRUNNER_INC,\ 00023 CPPUTEST_INC_EXT 00024 from tools.data.support import DEFAULT_SUPPORT 00025 from tools.tests import TEST_MBED_LIB 00026 00027 00028 LIBRARIES = [ 00029 # RPC 00030 { 00031 "id": "rpc", 00032 "source_dir": MBED_RPC, 00033 "build_dir": RPC_LIBRARY, 00034 "dependencies": [MBED_LIBRARIES], 00035 }, 00036 00037 # USB Device libraries 00038 { 00039 "id": "usb", 00040 "source_dir": USB, 00041 "build_dir": USB_LIBRARIES, 00042 "dependencies": [MBED_LIBRARIES], 00043 }, 00044 00045 # DSP libraries 00046 { 00047 "id": "dsp", 00048 "source_dir": [DSP_ABSTRACTION, DSP_CMSIS], 00049 "build_dir": DSP_LIBRARIES, 00050 "dependencies": [MBED_LIBRARIES] 00051 }, 00052 00053 # Unit Testing library 00054 { 00055 "id": "cpputest", 00056 "source_dir": [CPPUTEST_SRC, CPPUTEST_PLATFORM_SRC, 00057 CPPUTEST_TESTRUNNER_SCR], 00058 "build_dir": CPPUTEST_LIBRARY, 00059 "dependencies": [MBED_LIBRARIES], 00060 'inc_dirs': [CPPUTEST_INC, CPPUTEST_PLATFORM_INC, 00061 CPPUTEST_TESTRUNNER_INC, TEST_MBED_LIB], 00062 'inc_dirs_ext': [CPPUTEST_INC_EXT], 00063 'macros': ["CPPUTEST_USE_MEM_LEAK_DETECTION=0", 00064 "CPPUTEST_USE_STD_CPP_LIB=0", "CPPUTEST=1"], 00065 }, 00066 ] 00067 00068 00069 LIBRARY_MAP = dict([(library['id'], library) for library in LIBRARIES]) 00070 00071 00072 class Library (object): 00073 """A library representation that allows for querying of support""" 00074 def __init__(self, lib_id): 00075 lib = LIBRARY_MAP[lib_id] 00076 self.supported = lib.get("supported", DEFAULT_SUPPORT) 00077 self.dependencies = lib.get("dependencies", None) 00078 # Include dirs required by library build 00079 self.inc_dirs = lib.get("inc_dirs", None) 00080 # Include dirs required by others to use with this library 00081 self.inc_dirs_ext = lib.get("inc_dirs_ext", None) 00082 # Additional macros you want to define when building library 00083 self.macros = lib.get("macros", None) 00084 00085 self.source_dir = lib["source_dir"] 00086 self.build_dir = lib["build_dir"] 00087 00088 def is_supported (self, target, toolchain): 00089 """Check if a target toolchain combination is supported 00090 00091 Positional arguments: 00092 target - the MCU or board 00093 toolchain - the compiler 00094 """ 00095 if not hasattr(self, 'supported'): 00096 return True 00097 return (target.name in self.supported ) and \ 00098 (toolchain in self.supported [target.name])
Generated on Sun Jul 17 2022 08:25:24 by
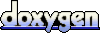