
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ipc.h
00001 /* 00002 * Copyright (c) 2017, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_IPC_H__ 00018 #define __UVISOR_API_IPC_H__ 00019 00020 #include "api/inc/ipc_exports.h" 00021 #include "api/inc/uvisor_exports.h" 00022 #include <stdint.h> 00023 #include <stddef.h> 00024 00025 00026 /** Wait for any of the specified IPC operations to complete. 00027 * 00028 * @note This function currently spins, burning through power. 00029 * 00030 * @param[in] wait_tokens a bitfield of tokens to wait on 00031 * @param[out] done_tokens a bitfield which tokens completed 00032 * @param[in] timeout_ms how long to wait (in ms) for an IPC operation 00033 * before returning. 0 means don't wait at all. Any 00034 * other value means wait forever. 00035 * @return 0 on success, non-zero error code otherwise 00036 */ 00037 UVISOR_EXTERN int ipc_waitforany(uint32_t wait_tokens, uint32_t * done_tokens, uint32_t timeout_ms); 00038 00039 /** Wait for all of the specified IPC operations to complete. 00040 * 00041 * @note This function currently spins, burning through power. 00042 * 00043 * @param[in] wait_tokens a bitfield of tokens to wait on 00044 * @param[out] done_tokens a bitfield which tokens completed 00045 * @param[in] timeout_ms how long to wait (in ms) for an IPC operation 00046 * before returning. 0 means don't wait at all. 00047 * Any other value means wait forever. 00048 * @return 0 on success, non-zero error code otherwise 00049 */ 00050 UVISOR_EXTERN int ipc_waitforall(uint32_t wait_tokens, uint32_t * done_tokens, uint32_t timeout_ms); 00051 00052 /** Asynchronously send an IPC message 00053 * 00054 * @note The memory used for receiving the message (pointed to by msg) and the 00055 * IPC descriptor (pointed to by desc) must be valid until after the send is 00056 * complete. In addition, each IPC message should use its own IPC descriptor. 00057 * Reusing an IPC descriptor will lead to unpredictable behaviours. 00058 * 00059 * @param[inout] desc an IPC descriptor for the message 00060 * @param[in] msg the message to send 00061 * 00062 * @return 0 on success, non-zero error code otherwise 00063 * */ 00064 UVISOR_EXTERN int ipc_send(uvisor_ipc_desc_t * desc, const void * msg); 00065 00066 /** Asynchronously receive an IPC message 00067 * 00068 * @note The memory used for receiving the message (pointed to by msg) and the 00069 * IPC descriptor (pointed to by desc) must be valid until after the receive is 00070 * complete. In addition, each IPC message should use its own IPC descriptor. 00071 * Reusing an IPC descriptor will lead to unpredictable behaviours. 00072 * 00073 * @param[inout] desc an IPC descriptor for the message 00074 * @param[out] msg the memory to copy the message to 00075 * 00076 * @return 0 on success, non-zero error code otherwise 00077 */ 00078 UVISOR_EXTERN int ipc_recv(uvisor_ipc_desc_t * desc, void * msg); 00079 00080 #endif /* __UVISOR_API_IPC_H__ */
Generated on Sun Jul 17 2022 08:25:24 by
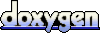