
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ioper_test_fs.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2015 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 00017 Author: Przemyslaw Wirkus <Przemyslaw.Wirkus@arm.com> 00018 00019 """ 00020 00021 import os.path 00022 from ioper_base import IOperTestCaseBase 00023 00024 00025 class IOperTest_FileStructure(IOperTestCaseBase): 00026 00027 def __init__(self, scope=None): 00028 IOperTestCaseBase.__init__(self, scope) 00029 00030 def if_file_exist(self, fname, fail_severity=None): 00031 file_path = os.path.join(self.param['mount_point'], fname) 00032 exist = os.path.isfile(file_path) 00033 tr_name = "FILE_EXIST(%s)" % fname.upper() 00034 if exist: 00035 self.result.append((self.PASS, tr_name, self.scope, "File '%s' exists" % file_path)) 00036 else: 00037 self.result.append((fail_severity if fail_severity else self.ERROR, tr_name, self.scope, "File '%s' not found" % file_path)) 00038 00039 def test(self, param=None): 00040 self.result = [] 00041 if param: 00042 pass 00043 return self.result 00044 00045 00046 class IOperTest_FileStructure_Basic(IOperTest_FileStructure): 00047 def __init__(self, scope=None): 00048 IOperTest_FileStructure.__init__(self, scope) 00049 00050 def test(self, param=None): 00051 self.param = param 00052 self.result = [] 00053 if param: 00054 self.if_file_exist('mbed.htm', self.ERROR) 00055 return self.result 00056 00057 00058 class IOperTest_FileStructure_MbedEnabled(IOperTest_FileStructure): 00059 def __init__(self, scope=None): 00060 IOperTest_FileStructure.__init__(self, scope) 00061 00062 def test(self, param=None): 00063 self.param = param 00064 self.result = [] 00065 if param: 00066 self.if_file_exist('mbed.htm', self.ERROR) 00067 self.if_file_exist('DETAILS.TXT', self.ERROR) 00068 self.if_file_exist('FAIL.TXT', self.INFO) 00069 return self.result
Generated on Sun Jul 17 2022 08:25:23 by
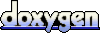