
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ioper_base.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2015 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 00017 Author: Przemyslaw Wirkus <Przemyslaw.Wirkus@arm.com> 00018 00019 """ 00020 00021 import sys 00022 00023 try: 00024 from colorama import Fore 00025 except: 00026 pass 00027 00028 COLORAMA = 'colorama' in sys.modules 00029 00030 00031 class IOperTestCaseBase (): 00032 """ Interoperability test case base class 00033 @return list of tuple (severity, Description) 00034 Example: (result.append((IOperTestSeverity.INFO, "")) 00035 """ 00036 00037 def __init__(self, scope=None): 00038 self.PASS = 'PASS' 00039 self.INFO = 'INFO' 00040 self.ERROR = 'ERROR' 00041 self.WARN = 'WARN' 00042 00043 self.scope = scope # Default test scope (basic, pedantic, mbed-enabled etc...) 00044 00045 def test(self, param=None): 00046 result = [] 00047 return result 00048 00049 def RED(self, text): 00050 return self.color_text (text, color=Fore.RED, delim=Fore.RESET) if COLORAMA else text 00051 00052 def GREEN(self, text): 00053 return self.color_text (text, color=Fore.GREEN, delim=Fore.RESET) if COLORAMA else text 00054 00055 def YELLOW(self, text): 00056 return self.color_text (text, color=Fore.YELLOW, delim=Fore.RESET) if COLORAMA else text 00057 00058 def color_text(self, text, color='', delim=''): 00059 return color + text + color + delim 00060 00061 def COLOR(self, severity, text): 00062 colors = { 00063 self.PASS : self.GREEN , 00064 self.ERROR : self.RED , 00065 self.WARN : self.YELLOW 00066 } 00067 if severity in colors: 00068 return colors[severity](text) 00069 return text
Generated on Sun Jul 17 2022 08:25:23 by
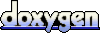