
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
intel_hex_utils.py
00001 from intelhex import IntelHex 00002 from cStringIO import StringIO 00003 00004 00005 def sections(h): 00006 start, last_address = None, None 00007 for a in h.addresses(): 00008 if last_address is None: 00009 start, last_address = a, a 00010 continue 00011 00012 if a > last_address + 1: 00013 yield (start, last_address) 00014 start = a 00015 00016 last_address = a 00017 00018 if start: 00019 yield (start, last_address) 00020 00021 00022 def print_sections(h): 00023 for s in sections(h): 00024 print "[0x%08X - 0x%08X]" % s 00025 00026 00027 def decode(record): 00028 h = IntelHex() 00029 f = StringIO(record) 00030 h.loadhex(f) 00031 h.dump()
Generated on Sun Jul 17 2022 08:25:23 by
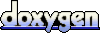