
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
host_test_registry.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 class HostTestRegistry : 00019 """ Simple class used to register and store 00020 host test plugins for further usage 00021 """ 00022 # Here we actually store all the plugins 00023 PLUGINS = {} # 'Plugin Name' : Plugin Object 00024 00025 def print_error(self, text): 00026 print "Plugin load failed. Reason: %s"% text 00027 00028 def register_plugin (self, plugin): 00029 """ Registers and stores plugin inside registry for further use. 00030 Method also calls plugin's setup() function to configure plugin if needed. 00031 00032 Note: Different groups of plugins may demand different extra parameter. Plugins 00033 should be at least for one type of plugin configured with the same parameters 00034 because we do not know which of them will actually use particular parameter. 00035 """ 00036 # TODO: 00037 # - check for unique caps for specified type 00038 if plugin.name not in self.PLUGINS : 00039 if plugin.setup(): # Setup plugin can be completed without errors 00040 self.PLUGINS [plugin.name] = plugin 00041 return True 00042 else: 00043 self.print_error ("%s setup failed"% plugin.name) 00044 else: 00045 self.print_error ("%s already loaded"% plugin.name) 00046 return False 00047 00048 def call_plugin (self, type, capability, *args, **kwargs): 00049 """ Execute plugin functionality respectively to its purpose 00050 """ 00051 for plugin_name in self.PLUGINS : 00052 plugin = self.PLUGINS [plugin_name] 00053 if plugin.type == type and capability in plugin.capabilities: 00054 return plugin.execute(capability, *args, **kwargs) 00055 return False 00056 00057 def get_plugin_caps (self, type): 00058 """ Returns list of all capabilities for plugin family with the same type. 00059 If there are no capabilities empty list is returned 00060 """ 00061 result = [] 00062 for plugin_name in self.PLUGINS : 00063 plugin = self.PLUGINS [plugin_name] 00064 if plugin.type == type: 00065 result.extend(plugin.capabilities) 00066 return sorted(result) 00067 00068 def load_plugin (self, name): 00069 """ Used to load module from 00070 """ 00071 mod = __import__("module_%s"% name) 00072 return mod 00073 00074 def __str__ (self): 00075 """ User friendly printing method to show hooked plugins 00076 """ 00077 from prettytable import PrettyTable 00078 column_names = ['name', 'type', 'capabilities', 'stable'] 00079 pt = PrettyTable(column_names) 00080 for column in column_names: 00081 pt.align[column] = 'l' 00082 for plugin_name in sorted(self.PLUGINS .keys()): 00083 name = self.PLUGINS [plugin_name].name 00084 type = self.PLUGINS [plugin_name].type 00085 stable = self.PLUGINS [plugin_name].stable 00086 capabilities = ', '.join(self.PLUGINS [plugin_name].capabilities) 00087 row = [name, type, capabilities, stable] 00088 pt.add_row(row) 00089 return pt.get_string()
Generated on Sun Jul 17 2022 08:25:23 by
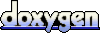