
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
host_test_plugins.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 from os import access, F_OK 00019 from sys import stdout 00020 from time import sleep 00021 from subprocess import call 00022 00023 00024 class HostTestPluginBase : 00025 """ Base class for all plug-ins used with host tests. 00026 """ 00027 ########################################################################### 00028 # Interface: 00029 ########################################################################### 00030 00031 ########################################################################### 00032 # Interface attributes defining plugin name, type etc. 00033 ########################################################################### 00034 name = "HostTestPluginBase" # Plugin name, can be plugin class name 00035 type = "BasePlugin" # Plugin type: ResetMethod, Copymethod etc. 00036 capabilities = [] # Capabilities names: what plugin can achieve 00037 # (e.g. reset using some external command line tool) 00038 stable = False # Determine if plugin is stable and can be used 00039 00040 ########################################################################### 00041 # Interface methods 00042 ########################################################################### 00043 def setup(self, *args, **kwargs): 00044 """ Configure plugin, this function should be called before plugin execute() method is used. 00045 """ 00046 return False 00047 00048 def execute (self, capabilitity, *args, **kwargs): 00049 """ Executes capability by name. 00050 Each capability e.g. may directly just call some command line 00051 program or execute building pythonic function 00052 """ 00053 return False 00054 00055 ########################################################################### 00056 # Interface helper methods - overload only if you need to have custom behaviour 00057 ########################################################################### 00058 def print_plugin_error(self, text): 00059 """ Function prints error in console and exits always with False 00060 """ 00061 print "Plugin error: %s::%s: %s"% (self.name, self.type , text) 00062 return False 00063 00064 def print_plugin_info (self, text, NL=True): 00065 """ Function prints notification in console and exits always with True 00066 """ 00067 if NL: 00068 print "Plugin info: %s::%s: %s"% (self.name, self.type , text) 00069 else: 00070 print "Plugin info: %s::%s: %s"% (self.name, self.type , text), 00071 return True 00072 00073 def print_plugin_char (self, char): 00074 """ Function prints char on stdout 00075 """ 00076 stdout.write(char) 00077 stdout.flush() 00078 return True 00079 00080 def check_mount_point_ready (self, destination_disk, init_delay=0.2, loop_delay=0.25): 00081 """ Checks if destination_disk is ready and can be accessed by e.g. copy commands 00082 @init_delay - Initial delay time before first access check 00083 @loop_delay - pooling delay for access check 00084 """ 00085 if not access(destination_disk, F_OK): 00086 self.print_plugin_info ("Waiting for mount point '%s' to be ready..."% destination_disk, NL=False) 00087 sleep(init_delay) 00088 while not access(destination_disk, F_OK): 00089 sleep(loop_delay) 00090 self.print_plugin_char ('.') 00091 00092 def check_parameters (self, capabilitity, *args, **kwargs): 00093 """ This function should be ran each time we call execute() 00094 to check if none of the required parameters is missing. 00095 """ 00096 missing_parameters = [] 00097 for parameter in self.required_parameters: 00098 if parameter not in kwargs: 00099 missing_parameters.append(parameter) 00100 if len(missing_parameters) > 0: 00101 self.print_plugin_error("execute parameter(s) '%s' missing!"% (', '.join(parameter))) 00102 return False 00103 return True 00104 00105 def run_command (self, cmd, shell=True): 00106 """ Runs command from command line. 00107 """ 00108 result = True 00109 ret = 0 00110 try: 00111 ret = call(cmd, shell=shell) 00112 if ret: 00113 self.print_plugin_error("[ret=%d] Command: %s"% (int(ret), cmd)) 00114 return False 00115 except Exception as e: 00116 result = False 00117 self.print_plugin_error("[ret=%d] Command: %s"% (int(ret), cmd)) 00118 self.print_plugin_error(str(e)) 00119 return result
Generated on Sun Jul 17 2022 08:25:23 by
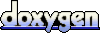