
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
fsm.h
00001 /* 00002 * fsm.h - {Link, IP} Control Protocol Finite State Machine definitions. 00003 * 00004 * Copyright (c) 1984-2000 Carnegie Mellon University. All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * 2. Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in 00015 * the documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * 3. The name "Carnegie Mellon University" must not be used to 00019 * endorse or promote products derived from this software without 00020 * prior written permission. For permission or any legal 00021 * details, please contact 00022 * Office of Technology Transfer 00023 * Carnegie Mellon University 00024 * 5000 Forbes Avenue 00025 * Pittsburgh, PA 15213-3890 00026 * (412) 268-4387, fax: (412) 268-7395 00027 * tech-transfer@andrew.cmu.edu 00028 * 00029 * 4. Redistributions of any form whatsoever must retain the following 00030 * acknowledgment: 00031 * "This product includes software developed by Computing Services 00032 * at Carnegie Mellon University (http://www.cmu.edu/computing/)." 00033 * 00034 * CARNEGIE MELLON UNIVERSITY DISCLAIMS ALL WARRANTIES WITH REGARD TO 00035 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00036 * AND FITNESS, IN NO EVENT SHALL CARNEGIE MELLON UNIVERSITY BE LIABLE 00037 * FOR ANY SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00038 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00039 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00040 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00041 * 00042 * $Id: fsm.h,v 1.10 2004/11/13 02:28:15 paulus Exp $ 00043 */ 00044 00045 #include "netif/ppp/ppp_opts.h" 00046 #if PPP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00047 00048 #ifndef FSM_H 00049 #define FSM_H 00050 00051 #include "ppp.h" 00052 00053 /* 00054 * Packet header = Code, id, length. 00055 */ 00056 #define HEADERLEN 4 00057 00058 00059 /* 00060 * CP (LCP, IPCP, etc.) codes. 00061 */ 00062 #define CONFREQ 1 /* Configuration Request */ 00063 #define CONFACK 2 /* Configuration Ack */ 00064 #define CONFNAK 3 /* Configuration Nak */ 00065 #define CONFREJ 4 /* Configuration Reject */ 00066 #define TERMREQ 5 /* Termination Request */ 00067 #define TERMACK 6 /* Termination Ack */ 00068 #define CODEREJ 7 /* Code Reject */ 00069 00070 00071 /* 00072 * Each FSM is described by an fsm structure and fsm callbacks. 00073 */ 00074 typedef struct fsm { 00075 ppp_pcb *pcb; /* PPP Interface */ 00076 const struct fsm_callbacks *callbacks; /* Callback routines */ 00077 const char *term_reason; /* Reason for closing protocol */ 00078 u8_t seen_ack; /* Have received valid Ack/Nak/Rej to Req */ 00079 /* -- This is our only flag, we might use u_int :1 if we have more flags */ 00080 u16_t protocol; /* Data Link Layer Protocol field value */ 00081 u8_t state; /* State */ 00082 u8_t flags; /* Contains option bits */ 00083 u8_t id; /* Current id */ 00084 u8_t reqid; /* Current request id */ 00085 u8_t retransmits; /* Number of retransmissions left */ 00086 u8_t nakloops; /* Number of nak loops since last ack */ 00087 u8_t rnakloops; /* Number of naks received */ 00088 u8_t maxnakloops; /* Maximum number of nak loops tolerated 00089 (necessary because IPCP require a custom large max nak loops value) */ 00090 u8_t term_reason_len; /* Length of term_reason */ 00091 } fsm; 00092 00093 00094 typedef struct fsm_callbacks { 00095 void (*resetci) /* Reset our Configuration Information */ 00096 (fsm *); 00097 int (*cilen) /* Length of our Configuration Information */ 00098 (fsm *); 00099 void (*addci) /* Add our Configuration Information */ 00100 (fsm *, u_char *, int *); 00101 int (*ackci) /* ACK our Configuration Information */ 00102 (fsm *, u_char *, int); 00103 int (*nakci) /* NAK our Configuration Information */ 00104 (fsm *, u_char *, int, int); 00105 int (*rejci) /* Reject our Configuration Information */ 00106 (fsm *, u_char *, int); 00107 int (*reqci) /* Request peer's Configuration Information */ 00108 (fsm *, u_char *, int *, int); 00109 void (*up) /* Called when fsm reaches PPP_FSM_OPENED state */ 00110 (fsm *); 00111 void (*down) /* Called when fsm leaves PPP_FSM_OPENED state */ 00112 (fsm *); 00113 void (*starting) /* Called when we want the lower layer */ 00114 (fsm *); 00115 void (*finished) /* Called when we don't want the lower layer */ 00116 (fsm *); 00117 void (*protreject) /* Called when Protocol-Reject received */ 00118 (int); 00119 void (*retransmit) /* Retransmission is necessary */ 00120 (fsm *); 00121 int (*extcode) /* Called when unknown code received */ 00122 (fsm *, int, int, u_char *, int); 00123 const char *proto_name; /* String name for protocol (for messages) */ 00124 } fsm_callbacks; 00125 00126 00127 /* 00128 * Link states. 00129 */ 00130 #define PPP_FSM_INITIAL 0 /* Down, hasn't been opened */ 00131 #define PPP_FSM_STARTING 1 /* Down, been opened */ 00132 #define PPP_FSM_CLOSED 2 /* Up, hasn't been opened */ 00133 #define PPP_FSM_STOPPED 3 /* Open, waiting for down event */ 00134 #define PPP_FSM_CLOSING 4 /* Terminating the connection, not open */ 00135 #define PPP_FSM_STOPPING 5 /* Terminating, but open */ 00136 #define PPP_FSM_REQSENT 6 /* We've sent a Config Request */ 00137 #define PPP_FSM_ACKRCVD 7 /* We've received a Config Ack */ 00138 #define PPP_FSM_ACKSENT 8 /* We've sent a Config Ack */ 00139 #define PPP_FSM_OPENED 9 /* Connection available */ 00140 00141 00142 /* 00143 * Flags - indicate options controlling FSM operation 00144 */ 00145 #define OPT_PASSIVE 1 /* Don't die if we don't get a response */ 00146 #define OPT_RESTART 2 /* Treat 2nd OPEN as DOWN, UP */ 00147 #define OPT_SILENT 4 /* Wait for peer to speak first */ 00148 00149 00150 /* 00151 * Timeouts. 00152 */ 00153 #if 0 /* moved to ppp_opts.h */ 00154 #define DEFTIMEOUT 3 /* Timeout time in seconds */ 00155 #define DEFMAXTERMREQS 2 /* Maximum Terminate-Request transmissions */ 00156 #define DEFMAXCONFREQS 10 /* Maximum Configure-Request transmissions */ 00157 #define DEFMAXNAKLOOPS 5 /* Maximum number of nak loops */ 00158 #endif /* moved to ppp_opts.h */ 00159 00160 00161 /* 00162 * Prototypes 00163 */ 00164 void fsm_init(fsm *f); 00165 void fsm_lowerup(fsm *f); 00166 void fsm_lowerdown(fsm *f); 00167 void fsm_open(fsm *f); 00168 void fsm_close(fsm *f, const char *reason); 00169 void fsm_input(fsm *f, u_char *inpacket, int l); 00170 void fsm_protreject(fsm *f); 00171 void fsm_sdata(fsm *f, u_char code, u_char id, const u_char *data, int datalen); 00172 00173 00174 #endif /* FSM_H */ 00175 #endif /* PPP_SUPPORT */
Generated on Sun Jul 17 2022 08:25:23 by
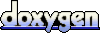