
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_env.h
00001 00002 /** \addtogroup frameworks */ 00003 /** @{*/ 00004 /* 00005 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00006 * SPDX-License-Identifier: Apache-2.0 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00009 * not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00016 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 */ 00020 00021 #ifndef GREENTEA_CLIENT_TEST_ENV_H_ 00022 #define GREENTEA_CLIENT_TEST_ENV_H_ 00023 00024 #ifdef __cplusplus 00025 #ifdef YOTTA_GREENTEA_CLIENT_VERSION_STRING 00026 #define MBED_GREENTEA_CLIENT_VERSION_STRING YOTTA_GREENTEA_CLIENT_VERSION_STRING 00027 #else 00028 #define MBED_GREENTEA_CLIENT_VERSION_STRING "1.3.0" 00029 #endif 00030 00031 #include <stdio.h> 00032 00033 /** 00034 * Auxilary macros 00035 */ 00036 #define NL "\n" 00037 #define RCNL "\r\n" 00038 00039 /** 00040 * Auxilary macros to keep mbed-drivers compatibility with utest before greentea-client 00041 */ 00042 #define TEST_ENV_TESTCASE_COUNT GREENTEA_TEST_ENV_TESTCASE_COUNT 00043 #define TEST_ENV_TESTCASE_START GREENTEA_TEST_ENV_TESTCASE_START 00044 #define TEST_ENV_TESTCASE_FINISH GREENTEA_TEST_ENV_TESTCASE_FINISH 00045 #define TEST_ENV_TESTCASE_SUMMARY GREENTEA_TEST_ENV_TESTCASE_SUMMARY 00046 00047 /** 00048 * Default length for UUID buffers (used during the sync process) 00049 */ 00050 #define GREENTEA_UUID_LENGTH 48 00051 00052 /** 00053 * Generic test suite transport protocol keys 00054 */ 00055 extern const char* GREENTEA_TEST_ENV_END; 00056 extern const char* GREENTEA_TEST_ENV_EXIT; 00057 extern const char* GREENTEA_TEST_ENV_SYNC; 00058 extern const char* GREENTEA_TEST_ENV_TIMEOUT; 00059 extern const char* GREENTEA_TEST_ENV_HOST_TEST_NAME; 00060 extern const char* GREENTEA_TEST_ENV_HOST_TEST_VERSION; 00061 00062 /** 00063 * Test suite success code strings 00064 */ 00065 extern const char* GREENTEA_TEST_ENV_SUCCESS; 00066 extern const char* GREENTEA_TEST_ENV_FAILURE; 00067 00068 /** 00069 * Test case transport protocol start/finish keys 00070 */ 00071 extern const char* GREENTEA_TEST_ENV_TESTCASE_NAME; 00072 extern const char* GREENTEA_TEST_ENV_TESTCASE_COUNT; 00073 extern const char* GREENTEA_TEST_ENV_TESTCASE_START; 00074 extern const char* GREENTEA_TEST_ENV_TESTCASE_FINISH; 00075 extern const char* GREENTEA_TEST_ENV_TESTCASE_SUMMARY; 00076 00077 /** 00078 * Code Coverage (LCOV) transport protocol keys 00079 */ 00080 extern const char* GREENTEA_TEST_ENV_LCOV_START; 00081 00082 /** 00083 * Greentea-client related API for communication with host side 00084 */ 00085 void GREENTEA_SETUP_UUID(const int timeout, const char *host_test_name, char *buffer, size_t size); 00086 void GREENTEA_TESTSUITE_RESULT(const int); 00087 void GREENTEA_TESTCASE_START(const char *test_case_name); 00088 void GREENTEA_TESTCASE_FINISH(const char *test_case_name, const size_t passes, const size_t failed); 00089 00090 /** 00091 * Test suite result related notification API 00092 */ 00093 void greentea_send_kv(const char *, const int); 00094 void greentea_send_kv(const char *, const int, const int); 00095 void greentea_send_kv(const char *, const char *, const int); 00096 void greentea_send_kv(const char *, const char *, const int, const int); 00097 00098 #ifdef MBED_CFG_DEBUG_OPTIONS_COVERAGE 00099 /** 00100 * Code Coverage API 00101 */ 00102 void greentea_notify_coverage_start(const char *path); 00103 void greentea_notify_coverage_end(); 00104 #endif // MBED_CFG_DEBUG_OPTIONS_COVERAGE 00105 00106 #endif // __cplusplus 00107 00108 #ifdef __cplusplus 00109 extern "C" { 00110 #endif 00111 00112 /** 00113 * Greentea-client C API 00114 */ 00115 void GREENTEA_SETUP(const int timeout, const char * host_test); 00116 void greentea_send_kv(const char * key, const char * val); 00117 int greentea_parse_kv(char * key, char * val, 00118 const int key_len, const int val_len); 00119 int greentea_getc(); 00120 00121 #ifdef __cplusplus 00122 } 00123 #endif 00124 00125 #endif // GREENTEA_CLIENT_TEST_ENV_H_ 00126 00127 /** @}*/
Generated on Sun Jul 17 2022 08:25:32 by
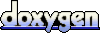