
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
flush2.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 /** @file flush2.cpp Test cases to flush KVs in the CFSTORE using the drv->Flush() interface. 00019 * 00020 * Please consult the documentation under the test-case functions for 00021 * a description of the individual test case. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "utest/utest.h" 00026 #include "unity/unity.h" 00027 #include "cfstore_config.h" 00028 #include "configuration_store.h" 00029 #include "greentea-client/test_env.h" 00030 #include "cfstore_test.h" 00031 #include "cfstore_debug.h" 00032 #include "cfstore_utest.h" 00033 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00034 #include "uvisor-lib/uvisor-lib.h" 00035 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00036 00037 #include <stdio.h> 00038 #include <stdlib.h> 00039 #include <string.h> 00040 #include <inttypes.h> 00041 00042 using namespace utest::v1; 00043 00044 /// @cond CFSTORE_DOXYGEN_DISABLE 00045 /* 00046 * Defines 00047 */ 00048 #define CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE 256 00049 #define cfstore_flush_fsm_null NULL 00050 #define CFSTORE_FLUSH_CASE_TIMEOUT_MS 10000 00051 00052 /* Configure secure box. */ 00053 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00054 UVISOR_BOX_NAMESPACE("com.arm.mbed.cfstore.test.flush2.box1"); 00055 UVISOR_BOX_CONFIG(cfstore_flush2_box1, UVISOR_BOX_STACK_SIZE); 00056 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00057 00058 00059 /* 00060 * Globals 00061 * 00062 * cfstore_flush_utest_msg_g 00063 * buffer for storing TEST_ASSERT_xxx_MESSAGE messages 00064 */ 00065 static char cfstore_flush_utest_msg_g[CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE]; 00066 00067 /* KV data for test_03 */ 00068 static cfstore_kv_data_t cfstore_flush_test_02_kv_data[] = { 00069 { "com.arm.mbed.configurationstore.test.flush.cfstoreflushtest02", "1"}, 00070 /* 1 2 3 4 5 6 7 */ 00071 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00072 { NULL, NULL}, 00073 }; 00074 00075 /* async test version */ 00076 00077 typedef struct cfstore_flush_ctx_t 00078 { 00079 int32_t status; 00080 ARM_CFSTORE_OPCODE cmd_code; 00081 } cfstore_flush_ctx_t; 00082 /// @endcond 00083 00084 /* 00085 * Globals 00086 */ 00087 static cfstore_flush_ctx_t cfstore_flush_ctx_g; 00088 00089 /* 00090 * context related methods 00091 */ 00092 00093 /* @brief get a pointer to the global context data structure */ 00094 static cfstore_flush_ctx_t* cfstore_flush_ctx_get(void) 00095 { 00096 return &cfstore_flush_ctx_g; 00097 } 00098 00099 /* @brief initialize global context data structure */ 00100 static void cfstore_flush_ctx_init(cfstore_flush_ctx_t* ctx) 00101 { 00102 TEST_ASSERT_MESSAGE(ctx != NULL, "ctx is NULL"); 00103 00104 CFSTORE_FENTRYLOG("%s:entered\r\n", __func__); 00105 memset(&cfstore_flush_ctx_g, 0, sizeof(cfstore_flush_ctx_g)); 00106 } 00107 00108 00109 /* report whether built/configured for flash sync or async mode */ 00110 static control_t cfstore_flush2_test_00(const size_t call_count) 00111 { 00112 int32_t ret = ARM_DRIVER_ERROR; 00113 00114 (void) call_count; 00115 ret = cfstore_test_startup(); 00116 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00117 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00118 return CaseNext; 00119 } 00120 00121 /** 00122 * @brief 00123 * 00124 * 00125 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00126 */ 00127 control_t cfstore_flush2_test_01_start (const size_t call_count) 00128 { 00129 int32_t ret = ARM_DRIVER_ERROR; 00130 cfstore_flush_ctx_t* ctx = cfstore_flush_ctx_get(); 00131 const ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00132 00133 /* check that the mtd is in synchronous mode */ 00134 CFSTORE_FENTRYLOG("%s:entered:\r\n", __func__); 00135 (void) call_count; 00136 cfstore_flush_ctx_init(ctx); 00137 ret = drv->Initialize(cfstore_utest_default_callback, ctx); 00138 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: failed to initialize CFSTORE (ret=%d)\r\n", __func__, (int) ret); 00139 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00140 return CaseTimeout(100000); 00141 } 00142 00143 00144 /** 00145 * @brief 00146 * 00147 * 00148 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00149 */ 00150 control_t cfstore_flush2_test_01_mid (const size_t call_count) 00151 { 00152 00153 bool bfound = false; 00154 int32_t ivalue = 0; 00155 int32_t ret = ARM_DRIVER_ERROR; 00156 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00157 const char* key_name_query = "*"; 00158 char value[CFSTORE_KEY_NAME_MAX_LENGTH+1]; 00159 ARM_CFSTORE_SIZE len = CFSTORE_KEY_NAME_MAX_LENGTH+1; 00160 ARM_CFSTORE_HANDLE_INIT(next); 00161 ARM_CFSTORE_HANDLE_INIT(prev); 00162 ARM_CFSTORE_KEYDESC kdesc; 00163 00164 /* check that the mtd is in synchronous mode */ 00165 CFSTORE_FENTRYLOG("%s:entered: \r\n", __func__); 00166 (void) call_count; 00167 memset(&kdesc, 0, sizeof(kdesc)); 00168 00169 /* try to read key; should not be found */ 00170 ret = cfstore_test_kv_is_found(cfstore_flush_test_02_kv_data->key_name, &bfound); 00171 if(ret != ARM_DRIVER_OK && ret != ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND){ 00172 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: cfstore_test_kv_is_found() call failed (ret=%d).\r\n", __func__, (int) ret); 00173 TEST_ASSERT_MESSAGE(false, cfstore_flush_utest_msg_g); 00174 } 00175 00176 if(!bfound) 00177 { 00178 /* first time start up. nothing is stored in cfstore flash. check this is the case */ 00179 while(drv->Find(key_name_query, prev, next) == ARM_DRIVER_OK) 00180 { 00181 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: have found an entry in cfstore when none should be present", __func__); 00182 TEST_ASSERT_MESSAGE(false, cfstore_flush_utest_msg_g); 00183 } 00184 /* no entries found, which is correct. 00185 * store a value */ 00186 len = strlen(cfstore_flush_test_02_kv_data->value); 00187 ret = cfstore_test_create(cfstore_flush_test_02_kv_data->key_name, cfstore_flush_test_02_kv_data->value, &len, &kdesc); 00188 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error:1: failed to write kv data (ret=%d).\r\n", __func__, (int) ret); 00189 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00190 /* flush to flash */ 00191 ret = drv->Flush(); 00192 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: failed to flush data to cfstore flash (ret=%d).\r\n", __func__, (int) ret); 00193 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00194 00195 } else { 00196 /*read the value, increment by 1 and write value back */ 00197 len = CFSTORE_KEY_NAME_MAX_LENGTH+1; 00198 ret = cfstore_test_read(cfstore_flush_test_02_kv_data->key_name, value, &len); 00199 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: failed to read kv data (ret=%d).\r\n", __func__, (int) ret); 00200 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00201 00202 /* increment value */ 00203 ivalue = atoi(value); 00204 ++ivalue; 00205 snprintf(value, CFSTORE_KEY_NAME_MAX_LENGTH+1, "%d", (int) ivalue); 00206 len = strlen(value); 00207 ret = cfstore_test_write(cfstore_flush_test_02_kv_data->key_name, value, &len); 00208 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: failed to write kv data (ret=%d).\r\n", __func__, (int) ret); 00209 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00210 00211 /* flush to flash */ 00212 ret = drv->Flush(); 00213 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_FLUSH_UTEST_MSG_BUF_SIZE, "%s:Error: failed to flush data to cfstore flash (ret=%d).\r\n", __func__, (int) ret); 00214 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00215 } 00216 return CaseTimeout(100000); 00217 } 00218 00219 /** 00220 * @brief 00221 * 00222 * 00223 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00224 */ 00225 control_t cfstore_flush2_test_01_end (const size_t call_count) 00226 { 00227 const ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00228 00229 CFSTORE_FENTRYLOG("%s:entered:\r\n", __func__); 00230 (void) call_count; 00231 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00232 TEST_ASSERT_MESSAGE(drv->Uninitialize() >= ARM_DRIVER_OK, cfstore_flush_utest_msg_g); 00233 return CaseNext; 00234 } 00235 00236 00237 /** 00238 * @brief test to open(create) a very large value, write the data, close, then flush. 00239 * for a N keys simultaneously. 00240 * 00241 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00242 */ 00243 control_t cfstore_flush2_test_02(const size_t call_count) 00244 { 00245 (void) call_count; 00246 //todo: implement test 00247 CFSTORE_TEST_UTEST_MESSAGE(cfstore_flush_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Warn: Not implemented\n", __func__); 00248 CFSTORE_DBGLOG("%s: WARN: requires implementation\n", __func__); 00249 TEST_ASSERT_MESSAGE(true, cfstore_flush_utest_msg_g); 00250 return CaseNext; 00251 } 00252 00253 00254 /// @cond CFSTORE_DOXYGEN_DISABLE 00255 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00256 { 00257 // Call the default reporting function- 00258 GREENTEA_SETUP(100, "default_auto"); 00259 return greentea_test_setup_handler(number_of_cases); 00260 } 00261 00262 Case cases[] = { 00263 Case("CFSTORE_FLUSH2_test_00", cfstore_flush2_test_00), 00264 Case("CFSTORE_FLUSH2_test_01_start", cfstore_flush2_test_01_start ), 00265 Case("CFSTORE_FLUSH2_test_01_mid", cfstore_flush2_test_01_mid ), 00266 Case("CFSTORE_FLUSH2_test_01_end", cfstore_flush2_test_01_end ), 00267 Case("CFSTORE_FLUSH2_test_02_start", cfstore_utest_default_start), 00268 Case("CFSTORE_FLUSH2_test_02_end", cfstore_flush2_test_02), 00269 }; 00270 00271 00272 // Declare your test specification with a custom setup handler 00273 Specification specification(greentea_setup, cases); 00274 00275 int main() 00276 { 00277 return !Harness::run(specification); 00278 } 00279 /// @endcond
Generated on Sun Jul 17 2022 08:25:23 by
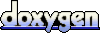