
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
find2.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 */ 00018 00019 /** @file find2.cpp Test cases to find KVs in the CFSTORE using the drv->Find() interface. 00020 * 00021 * Please consult the documentation under the test-case functions for 00022 * a description of the individual test case. 00023 */ 00024 00025 #include "mbed.h" 00026 #include "cfstore_config.h" 00027 #include "cfstore_test.h" 00028 #include "cfstore_debug.h" 00029 #include "Driver_Common.h" 00030 #include "configuration_store.h" 00031 #include "utest/utest.h" 00032 #include "unity/unity.h" 00033 #include "greentea-client/test_env.h" 00034 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00035 #include "uvisor-lib/uvisor-lib.h" 00036 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00037 00038 #include <stdio.h> 00039 #include <stdlib.h> 00040 #include <string.h> 00041 #include <assert.h> 00042 #include <inttypes.h> 00043 00044 using namespace utest::v1; 00045 00046 static char cfstore_find2_utest_msg_g[CFSTORE_UTEST_MSG_BUF_SIZE]; 00047 00048 /* Configure secure box. */ 00049 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00050 UVISOR_BOX_NAMESPACE("com.arm.mbed.cfstore.test.find2.box1"); 00051 UVISOR_BOX_CONFIG(cfstore_find2_box1, UVISOR_BOX_STACK_SIZE); 00052 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00053 00054 /// @cond CFSTORE_DOXYGEN_DISABLE 00055 #ifdef CFSTORE_DEBUG 00056 #define CFSTORE_FIND2_GREENTEA_TIMEOUT_S 360 00057 #else 00058 #define CFSTORE_FIND2_GREENTEA_TIMEOUT_S 60 00059 #endif 00060 #define CFSTORE_FIND2_TEST_02_VALUE_SIZE 191 00061 00062 extern ARM_CFSTORE_DRIVER cfstore_driver; 00063 00064 void cfstore_find2_callback(int32_t status, ARM_CFSTORE_OPCODE cmd_code, void *client_context, ARM_CFSTORE_HANDLE handle) 00065 { 00066 (void) status; 00067 (void) cmd_code; 00068 (void) client_context; 00069 (void) handle; 00070 return; 00071 } 00072 /// @endcond 00073 00074 /* report whether built/configured for flash sync or async mode */ 00075 static control_t cfstore_find2_test_00(const size_t call_count) 00076 { 00077 int32_t ret = ARM_DRIVER_ERROR; 00078 00079 (void) call_count; 00080 ret = cfstore_test_startup(); 00081 CFSTORE_TEST_UTEST_MESSAGE(cfstore_find2_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00082 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_find2_utest_msg_g); 00083 return CaseNext; 00084 } 00085 00086 00087 static control_t cfstore_find2_test_01(const size_t call_count) 00088 { 00089 char keyBuffer[128] = "com.arm.mbed.manifest-manager.root.AQAAAAAAAAA-.manifest"; 00090 int32_t rc; 00091 ARM_CFSTORE_HANDLE_INIT(hkey); 00092 ARM_CFSTORE_HANDLE_INIT(prev); 00093 00094 // Initialize the config store 00095 (void) call_count; 00096 cfstore_driver.Initialize(cfstore_find2_callback, NULL); 00097 cfstore_driver.PowerControl(ARM_POWER_FULL); 00098 00099 // Find the target key 00100 rc = cfstore_driver.Find(keyBuffer, prev, hkey); 00101 00102 // If the target key was not found 00103 if (rc == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND) { 00104 ARM_CFSTORE_KEYDESC kdesc = { 00105 .acl = { 00106 .perm_owner_read = 1, 00107 .perm_owner_write = 1, 00108 .perm_owner_execute = 0, 00109 .perm_other_read = 1, 00110 .perm_other_write = 0, 00111 .perm_other_execute = 0, 00112 /* added initialisers */ 00113 .reserved = 0 00114 }, .drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE, 00115 .security = { 00116 .acls = 1, 00117 .rollback_protection = 0, 00118 .tamper_proof = 0, 00119 .internal_flash = 0, 00120 /* added initialisers */ 00121 .reserved1 = 0, 00122 .software_attacks = 0, 00123 .board_level_attacks = 0, 00124 .chip_level_attacks = 0, 00125 .side_channel_attacks = 0, 00126 .reserved2 = 0 00127 }, 00128 .flags = { 00129 .continuous = 0, 00130 .lazy_flush = 1, 00131 .flush_on_close = 1, 00132 .read = 0, 00133 .write = 1, 00134 .execute = 0, 00135 .storage_detect = 1, 00136 /* added initialisers */ 00137 .reserved = 0 00138 } 00139 }; 00140 00141 // Create the target key 00142 rc = cfstore_driver.Create(keyBuffer, 191, &kdesc, hkey); 00143 } 00144 return CaseNext; 00145 } 00146 00147 00148 /* fixed version of brendans code */ 00149 static control_t cfstore_find2_test_02(const size_t call_count) 00150 { 00151 char keyBuffer[128] = "com.arm.mbed.manifest-manager.root.AQAAAAAAAAA-.manifest"; 00152 00153 int32_t rc; 00154 ARM_CFSTORE_HANDLE_INIT(hkey); 00155 ARM_CFSTORE_HANDLE_INIT(prev); 00156 ARM_CFSTORE_SIZE length; 00157 char value[CFSTORE_FIND2_TEST_02_VALUE_SIZE]; 00158 00159 // Initialize the config store 00160 (void) call_count; 00161 cfstore_driver.Initialize(NULL, NULL); /* non-null client_callback not supported for MBED_V_0_1_x */ 00162 cfstore_driver.PowerControl(ARM_POWER_FULL); 00163 memset(value, 0, 191); 00164 00165 // Find the target key 00166 rc = cfstore_driver.Find(keyBuffer, prev, hkey); 00167 00168 // If the target key was not found 00169 if (rc == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND) { 00170 ARM_CFSTORE_KEYDESC kdesc = { 00171 .acl = { 00172 .perm_owner_read = 1, 00173 .perm_owner_write = 1, 00174 .perm_owner_execute = 0, 00175 .perm_other_read = 1, 00176 .perm_other_write = 0, 00177 .perm_other_execute = 0, 00178 .reserved = 0 00179 }, .drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE, /* DATA_RETENTION_NVM not supported for MBED_V_0_1_x */ 00180 .security = { 00181 .acls = 0, /* protection against internal software attacks using ACLs not supported for MBED_V_0_1_x */ 00182 .rollback_protection = 0, 00183 .tamper_proof = 0, 00184 .internal_flash = 0, 00185 .reserved1 = 0, 00186 .software_attacks = 0, 00187 .board_level_attacks = 0, 00188 .chip_level_attacks = 0, 00189 .side_channel_attacks = 0, 00190 .reserved2 = 0 00191 }, 00192 .flags = { 00193 .continuous = 0, 00194 .lazy_flush = 0, /* lazy flush not supported for MBED_V_0_1_x */ 00195 .flush_on_close = 0, /* flush on close not supported for MBED_V_0_1_x */ 00196 .read = 0, 00197 .write = 1, 00198 .execute = 0, 00199 .storage_detect = 0, /* storage detect supported for MBED_V_0_1_x */ 00200 /* added initialisers */ 00201 .reserved = 0 00202 00203 } 00204 }; 00205 00206 // Create the target key 00207 rc = cfstore_driver.Create(keyBuffer, 191, &kdesc, hkey); 00208 CFSTORE_TEST_UTEST_MESSAGE(cfstore_find2_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%sError: failed to create key\n", __func__); 00209 TEST_ASSERT_MESSAGE(rc >= ARM_DRIVER_OK, cfstore_find2_utest_msg_g); 00210 00211 strncpy(value, "MyValueData", CFSTORE_FIND2_TEST_02_VALUE_SIZE); 00212 length = strlen(value); 00213 rc = cfstore_driver.Write(hkey, value, &length); 00214 CFSTORE_TEST_UTEST_MESSAGE(cfstore_find2_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%sError: failed to write key\n", __func__); 00215 TEST_ASSERT_MESSAGE(rc >= ARM_DRIVER_OK, cfstore_find2_utest_msg_g); 00216 /* revert to CFSTORE_LOG if more trace required */ 00217 CFSTORE_DBGLOG("Success!%s", "\n"); 00218 } 00219 return CaseNext; 00220 } 00221 00222 /// @cond CFSTORE_DOXYGEN_DISABLE 00223 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00224 { 00225 GREENTEA_SETUP(CFSTORE_FIND2_GREENTEA_TIMEOUT_S, "default_auto"); 00226 return greentea_test_setup_handler(number_of_cases); 00227 } 00228 00229 Case cases[] = { 00230 /* 1 2 3 4 5 6 7 */ 00231 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00232 Case("FIND2_test_00", cfstore_find2_test_00), 00233 Case("FIND2_test_01", cfstore_find2_test_01), 00234 Case("FIND2_test_02", cfstore_find2_test_02), 00235 }; 00236 00237 00238 /* Declare your test specification with a custom setup handler */ 00239 Specification specification(greentea_setup, cases); 00240 00241 int main() 00242 { 00243 return !Harness::run(specification); 00244 } 00245 /// @endcond
Generated on Sun Jul 17 2022 08:25:23 by
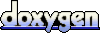