
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
fhss_config.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file fhss_config.h 00017 * \brief 00018 */ 00019 00020 #ifndef FHSS_CONFIG_H 00021 #define FHSS_CONFIG_H 00022 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /** 00029 * \brief Struct fhss_tuning_parameter defines FHSS tuning parameters. 00030 * All delays are given in microseconds. 00031 */ 00032 typedef struct fhss_tuning_parameter 00033 { 00034 /** Delay between data pushed to PHY TX function and TX started (Contains CSMA-CA maximum random period). */ 00035 uint32_t tx_processing_delay; 00036 00037 /** Delay between TX done (by transmitter) and received data pushed to MAC (by receiver). */ 00038 uint32_t rx_processing_delay; 00039 00040 /** Delay between TX done (by transmitter) and Ack transmission started (by receiver) */ 00041 uint32_t ack_processing_delay; 00042 } fhss_tuning_parameter_t; 00043 00044 /** 00045 * \brief Struct fhss_configuration defines basic configuration of FHSS. 00046 */ 00047 typedef struct fhss_configuration 00048 { 00049 /** Tuning parameters can be used to enhance synchronization accuracy*/ 00050 fhss_tuning_parameter_t fhss_tuning_parameters; 00051 00052 /** Maximum used interval for requesting synchronization info from FHSS parent device (seconds). */ 00053 uint16_t fhss_max_synch_interval; 00054 00055 /** Number of channel retries. */ 00056 uint8_t fhss_number_of_channel_retries; 00057 00058 /** Channel mask */ 00059 uint32_t channel_mask[8]; 00060 00061 } fhss_configuration_t; 00062 00063 /** 00064 * \brief Struct fhss_timer defines interface between FHSS and FHSS platform timer. 00065 * Application must implement FHSS timer driver which is then used by FHSS with this interface. 00066 */ 00067 typedef struct fhss_timer 00068 { 00069 /** Start timeout (1us) */ 00070 int (*fhss_timer_start)(uint32_t, void (*fhss_timer_callback)(const fhss_api_t *fhss_api, uint16_t), const fhss_api_t *fhss_api); 00071 00072 /** Stop timeout */ 00073 int (*fhss_timer_stop)(const fhss_api_t *fhss_api); 00074 00075 /** Get remaining time of started timeout*/ 00076 uint32_t (*fhss_get_remaining_slots)(const fhss_api_t *fhss_api); 00077 00078 /** Get timestamp since initialization of driver. Overflow of 32-bit counter is allowed (1us) */ 00079 uint32_t (*fhss_get_timestamp)(const fhss_api_t *fhss_api); 00080 00081 /** Divide 1 microsecond resolution. E.g. to use 64us resolution, use fhss_resolution_divider = 64*/ 00082 uint8_t fhss_resolution_divider; 00083 } fhss_timer_t; 00084 00085 /** 00086 * \brief Struct fhss_synch_configuration defines the synchronization time configurations. 00087 * Border router application must define and set these configuration for FHSS network. 00088 */ 00089 typedef struct fhss_synch_configuration 00090 { 00091 /** Number of broadcast channels. */ 00092 uint8_t fhss_number_of_bc_channels; 00093 00094 /** TX slots per channel. */ 00095 uint8_t fhss_number_of_tx_slots; 00096 00097 /** Length of superframe(microseconds) * Number of superframes defines the 00098 channel dwell time. E.g. 50000us * 8 -> Channel dwell time 400ms */ 00099 uint16_t fhss_superframe_length; 00100 00101 /** Number of superframes. */ 00102 uint8_t fhss_number_of_superframes; 00103 } fhss_synch_configuration_t; 00104 00105 00106 /** 00107 * \brief Struct fhss_statistics defines the available FHSS statistics. 00108 */ 00109 typedef struct fhss_statistics 00110 { 00111 /** FHSS synchronization drift compensation (us/channel). */ 00112 int16_t fhss_drift_compensation; 00113 00114 /** FHSS hop count. */ 00115 uint8_t fhss_hop_count; 00116 00117 /** FHSS synchronization interval (s). */ 00118 uint16_t fhss_synch_interval; 00119 00120 /** Average of 5 preceding synchronization fixes (us). Updated after every fifth synch fix. */ 00121 int16_t fhss_prev_avg_synch_fix; 00122 00123 /** FHSS synchronization lost counter. */ 00124 uint32_t fhss_synch_lost; 00125 } fhss_statistics_t; 00126 00127 /** 00128 * \brief Enumeration fhss_channel_mode_e defines the channel modes. 00129 */ 00130 typedef enum fhss_channel_mode_e { 00131 SINGLE_CHANNEL, //< Single channel 00132 FHSS, //< Frequency hopping mode 00133 } fhss_channel_mode_e; 00134 00135 #ifdef __cplusplus 00136 } 00137 #endif 00138 00139 #endif // FHSS_CONFIG_H
Generated on Sun Jul 17 2022 08:25:23 by
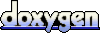