
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
__init__.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2017 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 from os.path import splitext, basename, join 00019 from tools.utils import mkdir 00020 from tools.export.gnuarmeclipse import GNUARMEclipse 00021 from tools.export.gnuarmeclipse import UID 00022 from tools.build_api import prepare_toolchain 00023 from sys import flags, platform 00024 00025 # Global random number generator instance. 00026 u = UID() 00027 00028 00029 class Sw4STM32 (GNUARMEclipse): 00030 """ 00031 Sw4STM32 class 00032 """ 00033 NAME = 'Sw4STM32' 00034 TOOLCHAIN = 'GCC_ARM' 00035 00036 BOARDS = { 00037 'B96B_F446VE': 00038 { 00039 'name': 'B96B-F446VE', 00040 'mcuId': 'STM32F446VETx' 00041 }, 00042 'DISCO_F051R8': 00043 { 00044 'name': 'STM32F0DISCOVERY', 00045 'mcuId': 'STM32F051R8Tx' 00046 }, 00047 'DISCO_F303VC': 00048 { 00049 'name': 'STM32F3DISCOVERY', 00050 'mcuId': 'STM32F303VCTx' 00051 }, 00052 'DISCO_F334C8': 00053 { 00054 'name': 'STM32F3348DISCOVERY', 00055 'mcuId': 'STM32F334C8Tx' 00056 }, 00057 'DISCO_F401VC': 00058 { 00059 'name': 'STM32F401C-DISCO', 00060 'mcuId': 'STM32F401VCTx' 00061 }, 00062 'DISCO_F407VG': 00063 { 00064 'name': 'STM32F4DISCOVERY', 00065 'mcuId': 'STM32F407VGTx' 00066 }, 00067 'DISCO_F413ZH': 00068 { 00069 'name': 'DISCO_F413', 00070 'mcuId': 'STM32F413ZHTx' 00071 }, 00072 'DISCO_F429ZI': 00073 { 00074 'name': 'STM32F429I-DISCO', 00075 'mcuId': 'STM32F429ZITx' 00076 }, 00077 'DISCO_F469NI': 00078 { 00079 'name': 'DISCO-F469NI', 00080 'mcuId': 'STM32F469NIHx' 00081 }, 00082 'DISCO_F746NG': 00083 { 00084 'name': 'STM32F746G-DISCO', 00085 'mcuId': 'STM32F746NGHx' 00086 }, 00087 'DISCO_F769NI': 00088 { 00089 'name': 'DISCO-F769NI', 00090 'mcuId': 'STM32F769NIHx' 00091 }, 00092 'DISCO_L053C8': 00093 { 00094 'name': 'STM32L0538DISCOVERY', 00095 'mcuId': 'STM32L053C8Tx' 00096 }, 00097 'DISCO_L072CZ_LRWAN1': 00098 { 00099 'name': 'DISCO-L072CZ-LRWAN1', 00100 'mcuId': 'STM32L072CZTx' 00101 }, 00102 'DISCO_L475VG_IOT01A': 00103 { 00104 'name': 'STM32L475G-DISCO', 00105 'mcuId': 'STM32L475VGTx' 00106 }, 00107 'DISCO_L476VG': 00108 { 00109 'name': 'STM32L476G-DISCO', 00110 'mcuId': 'STM32L476VGTx' 00111 }, 00112 'NUCLEO_F030R8': 00113 { 00114 'name': 'NUCLEO-F030R8', 00115 'mcuId': 'STM32F030R8Tx' 00116 }, 00117 'NUCLEO_F031K6': 00118 { 00119 'name': 'NUCLEO-F031K6', 00120 'mcuId': 'STM32F031K6Tx' 00121 }, 00122 'NUCLEO_F042K6': 00123 { 00124 'name': 'NUCLEO-F042K6', 00125 'mcuId': 'STM32F042K6Tx' 00126 }, 00127 'NUCLEO_F070RB': 00128 { 00129 'name': 'NUCLEO-F070RB', 00130 'mcuId': 'STM32F070RBTx' 00131 }, 00132 'NUCLEO_F072RB': 00133 { 00134 'name': 'NUCLEO-F072RB', 00135 'mcuId': 'STM32F072RBTx' 00136 }, 00137 'NUCLEO_F091RC': 00138 { 00139 'name': 'NUCLEO-F091RC', 00140 'mcuId': 'STM32F091RCTx' 00141 }, 00142 'NUCLEO_F103RB': 00143 { 00144 'name': 'NUCLEO-F103RB', 00145 'mcuId': 'STM32F103RBTx' 00146 }, 00147 'NUCLEO_F207ZG': 00148 { 00149 'name': 'NUCLEO-F207ZG', 00150 'mcuId': 'STM32F207ZGTx' 00151 }, 00152 'NUCLEO_F302R8': 00153 { 00154 'name': 'NUCLEO-F302R8', 00155 'mcuId': 'STM32F302R8Tx' 00156 }, 00157 'NUCLEO_F303K8': 00158 { 00159 'name': 'NUCLEO-F303K8', 00160 'mcuId': 'STM32F303K8Tx' 00161 }, 00162 'NUCLEO_F303RE': 00163 { 00164 'name': 'NUCLEO-F303RE', 00165 'mcuId': 'STM32F303RETx' 00166 }, 00167 'NUCLEO_F303ZE': 00168 { 00169 'name': 'NUCLEO-F303ZE', 00170 'mcuId': 'STM32F303ZETx' 00171 }, 00172 'NUCLEO_F334R8': 00173 { 00174 'name': 'NUCLEO-F334R8', 00175 'mcuId': 'STM32F334R8Tx' 00176 }, 00177 'NUCLEO_F401RE': 00178 { 00179 'name': 'NUCLEO-F401RE', 00180 'mcuId': 'STM32F401RETx' 00181 }, 00182 'NUCLEO_F410RB': 00183 { 00184 'name': 'NUCLEO-F410RB', 00185 'mcuId': 'STM32F410RBTx' 00186 }, 00187 'NUCLEO_F411RE': 00188 { 00189 'name': 'NUCLEO-F411RE', 00190 'mcuId': 'STM32F411RETx' 00191 }, 00192 'NUCLEO_F429ZI': 00193 { 00194 'name': 'NUCLEO-F429ZI', 00195 'mcuId': 'STM32F429ZITx' 00196 }, 00197 'NUCLEO_F446RE': 00198 { 00199 'name': 'NUCLEO-F446RE', 00200 'mcuId': 'STM32F446RETx' 00201 }, 00202 'NUCLEO_F446ZE': 00203 { 00204 'name': 'NUCLEO-F446ZE', 00205 'mcuId': 'STM32F446ZETx' 00206 }, 00207 'NUCLEO_F746ZG': 00208 { 00209 'name': 'NUCLEO-F746ZG', 00210 'mcuId': 'STM32F746ZGTx' 00211 }, 00212 'NUCLEO_F767ZI': 00213 { 00214 'name': 'NUCLEO-F767ZI', 00215 'mcuId': 'STM32F767ZITx' 00216 }, 00217 'NUCLEO_L011K4': 00218 { 00219 'name': 'NUCLEO-L011K4', 00220 'mcuId': 'STM32L011K4Tx' 00221 }, 00222 'NUCLEO_L031K6': 00223 { 00224 'name': 'NUCLEO-L031K6', 00225 'mcuId': 'STM32L031K6Tx' 00226 }, 00227 'NUCLEO_L053R8': 00228 { 00229 'name': 'NUCLEO-L053R8', 00230 'mcuId': 'STM32L053R8Tx' 00231 }, 00232 'NUCLEO_L073RZ': 00233 { 00234 'name': 'NUCLEO-L073RZ', 00235 'mcuId': 'STM32L073RZTx' 00236 }, 00237 'NUCLEO_L152RE': 00238 { 00239 'name': 'NUCLEO-L152RE', 00240 'mcuId': 'STM32L152RETx' 00241 }, 00242 'NUCLEO_L432KC': 00243 { 00244 'name': 'NUCLEO-L432KC', 00245 'mcuId': 'STM32L432KCUx' 00246 }, 00247 'NUCLEO_L476RG': 00248 { 00249 'name': 'NUCLEO-L476RG', 00250 'mcuId': 'STM32L476RGTx' 00251 }, 00252 'NUCLEO_L486RG': 00253 { 00254 'name': 'NUCLEO-L486RG', 00255 'mcuId': 'STM32L486RGTx' 00256 }, 00257 'NUCLEO_L496ZG': 00258 { 00259 'name': 'NUCLEO-L496ZG', 00260 'mcuId': 'STM32L496ZGTx' 00261 }, 00262 'NUCLEO_L496ZG_P': 00263 { 00264 'name': 'NUCLEO-L496ZG', 00265 'mcuId': 'STM32L496ZGTx' 00266 }, 00267 } 00268 00269 TARGETS = BOARDS.keys() 00270 00271 def __gen_dir(self, dir_name): 00272 """ 00273 Method that creates directory 00274 """ 00275 settings = join(self.export_dir, dir_name) 00276 mkdir(settings) 00277 00278 def get_fpu_hardware (self, fpu_unit): 00279 """ 00280 Convert fpu unit name into hardware name. 00281 """ 00282 hw = '' 00283 fpus = { 00284 'fpv4spd16': 'fpv4-sp-d16', 00285 'fpv5d16': 'fpv5-d16', 00286 'fpv5spd16': 'fpv5-sp-d16' 00287 } 00288 if fpu_unit in fpus: 00289 hw = fpus[fpu_unit] 00290 return hw 00291 00292 def process_sw_options (self, opts, flags_in): 00293 """ 00294 Process System Workbench specific options. 00295 00296 System Workbench for STM32 has some compile options, which are not recognized by the GNUARMEclipse exporter. 00297 Those are handled in this method. 00298 """ 00299 opts['c']['preprocess'] = False 00300 if '-E' in flags_in['c_flags']: 00301 opts['c']['preprocess'] = True 00302 opts['cpp']['preprocess'] = False 00303 if '-E' in flags_in['cxx_flags']: 00304 opts['cpp']['preprocess'] = True 00305 opts['c']['slowflashdata'] = False 00306 if '-mslow-flash-data' in flags_in['c_flags']: 00307 opts['c']['slowflashdata'] = True 00308 opts['cpp']['slowflashdata'] = False 00309 if '-mslow-flash-data' in flags_in['cxx_flags']: 00310 opts['cpp']['slowflashdata'] = True 00311 if opts['common']['optimization.messagelength']: 00312 opts['common']['optimization.other'] += ' -fmessage-length=0' 00313 if opts['common']['optimization.signedchar']: 00314 opts['common']['optimization.other'] += ' -fsigned-char' 00315 if opts['common']['optimization.nocommon']: 00316 opts['common']['optimization.other'] += ' -fno-common' 00317 if opts['common']['optimization.noinlinefunctions']: 00318 opts['common']['optimization.other'] += ' -fno-inline-functions' 00319 if opts['common']['optimization.freestanding']: 00320 opts['common']['optimization.other'] += ' -ffreestanding' 00321 if opts['common']['optimization.nobuiltin']: 00322 opts['common']['optimization.other'] += ' -fno-builtin' 00323 if opts['common']['optimization.spconstant']: 00324 opts['common']['optimization.other'] += ' -fsingle-precision-constant' 00325 if opts['common']['optimization.nomoveloopinvariants']: 00326 opts['common']['optimization.other'] += ' -fno-move-loop-invariants' 00327 if opts['common']['warnings.unused']: 00328 opts['common']['warnings.other'] += ' -Wunused' 00329 if opts['common']['warnings.uninitialized']: 00330 opts['common']['warnings.other'] += ' -Wuninitialized' 00331 if opts['common']['warnings.missingdeclaration']: 00332 opts['common']['warnings.other'] += ' -Wmissing-declarations' 00333 if opts['common']['warnings.pointerarith']: 00334 opts['common']['warnings.other'] += ' -Wpointer-arith' 00335 if opts['common']['warnings.padded']: 00336 opts['common']['warnings.other'] += ' -Wpadded' 00337 if opts['common']['warnings.shadow']: 00338 opts['common']['warnings.other'] += ' -Wshadow' 00339 if opts['common']['warnings.logicalop']: 00340 opts['common']['warnings.other'] += ' -Wlogical-op' 00341 if opts['common']['warnings.agreggatereturn']: 00342 opts['common']['warnings.other'] += ' -Waggregate-return' 00343 if opts['common']['warnings.floatequal']: 00344 opts['common']['warnings.other'] += ' -Wfloat-equal' 00345 opts['ld']['strip'] = False 00346 if '-s' in flags_in['ld_flags']: 00347 opts['ld']['strip'] = True 00348 opts['ld']['shared'] = False 00349 if '-shared' in flags_in['ld_flags']: 00350 opts['ld']['shared'] = True 00351 opts['ld']['soname'] = '' 00352 opts['ld']['implname'] = '' 00353 opts['ld']['defname'] = '' 00354 for item in flags_in['ld_flags']: 00355 if item.startswith('-Wl,-soname='): 00356 opts['ld']['soname'] = item[len('-Wl,-soname='):] 00357 if item.startswith('-Wl,--out-implib='): 00358 opts['ld']['implname'] = item[len('-Wl,--out-implib='):] 00359 if item.startswith('-Wl,--output-def='): 00360 opts['ld']['defname'] = item[len('-Wl,--output-def='):] 00361 opts['common']['arm.target.fpu.hardware'] = self.get_fpu_hardware ( 00362 opts['common']['arm.target.fpu.unit']) 00363 opts['common']['debugging.codecov'] = False 00364 if '-fprofile-arcs' in flags_in['common_flags'] and '-ftest-coverage' in flags_in['common_flags']: 00365 opts['common']['debugging.codecov'] = True 00366 # Passing linker options to linker with '-Wl,'-prefix. 00367 for index in range(len(opts['ld']['flags'])): 00368 item = opts['ld']['flags'][index] 00369 if not item.startswith('-Wl,'): 00370 opts['ld']['flags'][index] = '-Wl,' + item 00371 # Strange System Workbench feature: If first parameter in Other flags is a 00372 # define (-D...), Other flags will be replaced by defines and other flags 00373 # are completely ignored. Moving -D parameters to defines. 00374 for compiler in ['c', 'cpp', 'as']: 00375 tmpList = opts[compiler]['other'].split(' ') 00376 otherList = [] 00377 for item in tmpList: 00378 if item.startswith('-D'): 00379 opts[compiler]['defines'].append(str(item[2:])) 00380 else: 00381 otherList.append(item) 00382 opts[compiler]['other'] = ' '.join(otherList) 00383 # Assembler options 00384 for as_def in opts['as']['defines']: 00385 if '=' in as_def: 00386 opts['as']['other'] += ' --defsym ' + as_def 00387 else: 00388 opts['as']['other'] += ' --defsym ' + as_def + '=1' 00389 00390 def generate (self): 00391 """ 00392 Generate the .project and .cproject files. 00393 """ 00394 options = {} 00395 00396 if not self.resources.linker_script: 00397 raise NotSupportedException("No linker script found.") 00398 00399 print ('\nCreate a System Workbench for STM32 managed project') 00400 print ('Project name: {0}'.format(self.project_name)) 00401 print ('Target: {0}'.format(self.toolchain.target.name)) 00402 print ('Toolchain: {0}'.format(self.TOOLCHAIN ) + '\n') 00403 00404 self.resources.win_to_unix() 00405 00406 config_header = self.filter_dot(self.toolchain.get_config_header()) 00407 00408 libraries = [] 00409 for lib in self.resources.libraries: 00410 library, _ = splitext(basename(lib)) 00411 libraries.append(library[3:]) 00412 00413 self.system_libraries = [ 00414 'stdc++', 'supc++', 'm', 'c', 'gcc', 'nosys' 00415 ] 00416 00417 profiles = self.get_all_profiles() 00418 self.as_defines = [s.replace('"', '"') 00419 for s in self.toolchain.get_symbols(True)] 00420 self.c_defines = [s.replace('"', '"') 00421 for s in self.toolchain.get_symbols()] 00422 self.cpp_defines = self.c_defines 00423 print 'Symbols: {0}'.format(len(self.c_defines )) 00424 00425 self.include_path = [] 00426 for s in self.resources.inc_dirs: 00427 self.include_path .append("../" + self.filter_dot(s)) 00428 print ('Include folders: {0}'.format(len(self.include_path ))) 00429 00430 self.compute_exclusions() 00431 00432 print ('Exclude folders: {0}'.format(len(self.excluded_folders))) 00433 00434 ld_script = self.filter_dot(self.resources.linker_script) 00435 print ('Linker script: {0}'.format(ld_script)) 00436 00437 lib_dirs = [self.filter_dot(s) for s in self.resources.lib_dirs] 00438 00439 preproc_cmd = basename(self.toolchain.preproc[0]) + " " + " ".join(self.toolchain.preproc[1:]) 00440 00441 for id in ['debug', 'release']: 00442 opts = {} 00443 opts['common'] = {} 00444 opts['as'] = {} 00445 opts['c'] = {} 00446 opts['cpp'] = {} 00447 opts['ld'] = {} 00448 00449 opts['id'] = id 00450 opts['name'] = opts['id'].capitalize() 00451 00452 # TODO: Add prints to log or console in verbose mode. 00453 #print ('\nBuild configuration: {0}'.format(opts['name'])) 00454 00455 profile = profiles[id] 00456 00457 # A small hack, do not bother with src_path again, 00458 # pass an empty string to avoid crashing. 00459 src_paths = [''] 00460 toolchain = prepare_toolchain( 00461 src_paths, "", self.toolchain.target.name, self.TOOLCHAIN , build_profile=[profile]) 00462 00463 # Hack to fill in build_dir 00464 toolchain.build_dir = self.toolchain.build_dir 00465 00466 flags = self.toolchain_flags(toolchain) 00467 00468 # TODO: Add prints to log or console in verbose mode. 00469 # print 'Common flags:', ' '.join(flags['common_flags']) 00470 # print 'C++ flags:', ' '.join(flags['cxx_flags']) 00471 # print 'C flags:', ' '.join(flags['c_flags']) 00472 # print 'ASM flags:', ' '.join(flags['asm_flags']) 00473 # print 'Linker flags:', ' '.join(flags['ld_flags']) 00474 00475 # Most GNU ARM Eclipse options have a parent, 00476 # either debug or release. 00477 if '-O0' in flags['common_flags'] or '-Og' in flags['common_flags']: 00478 opts['parent_id'] = 'debug' 00479 else: 00480 opts['parent_id'] = 'release' 00481 00482 self.process_options(opts, flags) 00483 00484 opts['c']['defines'] = self.c_defines 00485 opts['cpp']['defines'] = self.cpp_defines 00486 opts['as']['defines'] = self.as_defines 00487 00488 self.process_sw_options (opts, flags) 00489 00490 opts['ld']['library_paths'] = [ 00491 self.filter_dot(s) for s in self.resources.lib_dirs] 00492 00493 opts['ld']['user_libraries'] = libraries 00494 opts['ld']['system_libraries'] = self.system_libraries 00495 opts['ld']['script'] = "linker-script-" + id + ".ld" 00496 00497 # Unique IDs used in multiple places. 00498 uid = {} 00499 uid['config'] = u.id 00500 uid['tool_c_compiler'] = u.id 00501 uid['tool_c_compiler_input'] = u.id 00502 uid['tool_cpp_compiler'] = u.id 00503 uid['tool_cpp_compiler_input'] = u.id 00504 00505 opts['uid'] = uid 00506 00507 options[id] = opts 00508 00509 ctx = { 00510 'name': self.project_name, 00511 'platform': platform, 00512 'include_paths': self.include_path , 00513 'config_header': config_header, 00514 'exclude_paths': '|'.join(self.excluded_folders), 00515 'ld_script': ld_script, 00516 'library_paths': lib_dirs, 00517 'object_files': self.resources.objects, 00518 'libraries': libraries, 00519 'board_name': self.BOARDS [self.target.upper()]['name'], 00520 'mcu_name': self.BOARDS [self.target.upper()]['mcuId'], 00521 'cpp_cmd': preproc_cmd, 00522 'options': options, 00523 # id property of 'u' will generate new random identifier every time 00524 # when called. 00525 'u': u 00526 } 00527 00528 self.__gen_dir ('.settings') 00529 self.gen_file('sw4stm32/language_settings_commom.tmpl', 00530 ctx, '.settings/language.settings.xml') 00531 self.gen_file('sw4stm32/project_common.tmpl', ctx, '.project') 00532 self.gen_file('sw4stm32/cproject_common.tmpl', ctx, '.cproject') 00533 self.gen_file('sw4stm32/makefile.targets.tmpl', ctx, 00534 'makefile.targets', trim_blocks=True, lstrip_blocks=True) 00535 self.gen_file('sw4stm32/launch.tmpl', ctx, self.project_name + 00536 ' ' + options['debug']['name'] + '.launch')
Generated on Sun Jul 17 2022 08:25:18 by
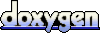