
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
__init__.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2014-2017 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from os.path import splitext, basename 00018 from tools.targets import TARGET_MAP 00019 from tools.export.exporters import Exporter 00020 from tools.export.makefile import GccArm 00021 00022 class QtCreator(GccArm ): 00023 NAME = 'QtCreator' 00024 00025 MBED_CONFIG_HEADER_SUPPORTED = True 00026 00027 def generate(self): 00028 self.resources.win_to_unix() 00029 00030 defines = [] # list of tuples ('D'/'U', [key, value]) (value is optional) 00031 forced_includes = [] # list of strings 00032 sources = [] # list of strings 00033 include_paths = [] # list of strings 00034 00035 next_is_include = False 00036 for f in self.flags['c_flags'] + self.flags['cxx_flags']: 00037 f=f.strip() 00038 if next_is_include: 00039 forced_includes.append(f) 00040 next_is_include = False 00041 continue 00042 if f.startswith('-D'): 00043 defines.append(('D', f[2:].split('=', 1))) 00044 elif f.startswith('-U'): 00045 defines.append(('U', [f[2:]])) 00046 elif f == "-include": 00047 next_is_include = True 00048 00049 for r_type in ['headers', 'c_sources', 's_sources', 'cpp_sources']: 00050 sources.extend(getattr(self.resources, r_type)) 00051 00052 include_paths = self.resources.inc_dirs 00053 00054 ctx = { 00055 'defines': defines, 00056 'forced_includes': forced_includes, 00057 'sources': sources, 00058 'include_paths': self.resources.inc_dirs 00059 } 00060 00061 for ext in ['creator', 'files', 'includes', 'config']: 00062 self.gen_file('qtcreator/%s.tmpl' % ext, ctx, "%s.%s" % (self.project_name, ext)) 00063 00064 # finally, generate the Makefile 00065 super(QtCreator, self).generate()
Generated on Sun Jul 17 2022 08:25:18 by
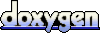