
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
__init__.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2014-2016 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from os.path import splitext, basename 00018 from tools.targets import TARGET_MAP 00019 from tools.export.exporters import Exporter, apply_supported_whitelist 00020 00021 00022 POST_BINARY_WHITELIST = set([ 00023 "TEENSY3_1Code.binary_hook", 00024 "LPCTargetCode.lpc_patch", 00025 "LPC4088Code.binary_hook" 00026 ]) 00027 00028 00029 class EmBitz(Exporter ): 00030 NAME = 'EmBitz' 00031 TOOLCHAIN = 'GCC_ARM' 00032 00033 MBED_CONFIG_HEADER_SUPPORTED = True 00034 00035 FILE_TYPES = { 00036 'headers': 'h', 00037 'c_sources': 'c', 00038 's_sources': 'a', 00039 'cpp_sources': 'cpp' 00040 } 00041 00042 @classmethod 00043 def is_target_supported(cls, target_name): 00044 target = TARGET_MAP[target_name] 00045 return apply_supported_whitelist( 00046 cls.TOOLCHAIN, POST_BINARY_WHITELIST, target) 00047 00048 @staticmethod 00049 def _remove_symbols(sym_list): 00050 return [s for s in sym_list if not s.startswith("-D")] 00051 00052 def generate(self): 00053 self.resources.win_to_unix() 00054 source_files = [] 00055 for r_type, n in self.FILE_TYPES.iteritems(): 00056 for file in getattr(self.resources, r_type): 00057 source_files.append({ 00058 'name': file, 'type': n 00059 }) 00060 00061 libraries = [] 00062 for lib in self.resources.libraries: 00063 l, _ = splitext(basename(lib)) 00064 libraries.append(l[3:]) 00065 00066 00067 if self.resources.linker_script is None: 00068 self.resources.linker_script = '' 00069 00070 ctx = { 00071 'name': self.project_name, 00072 'target': self.target, 00073 'toolchain': self.toolchain.name, 00074 'source_files': source_files, 00075 'include_paths': self.resources.inc_dirs, 00076 'script_file': self.resources.linker_script, 00077 'library_paths': self.resources.lib_dirs, 00078 'libraries': libraries, 00079 'symbols': self.toolchain.get_symbols(), 00080 'object_files': self.resources.objects, 00081 'sys_libs': self.toolchain.sys_libs, 00082 'cc_org': (self.flags['common_flags'] + 00083 self._remove_symbols(self.flags['c_flags'])), 00084 'ld_org': self.flags['ld_flags'], 00085 'cppc_org': (self.flags['common_flags'] + 00086 self._remove_symbols(self.flags['cxx_flags'])) 00087 } 00088 00089 self.gen_file('embitz/eix.tmpl', ctx, '%s.eix' % self.project_name)
Generated on Sun Jul 17 2022 08:25:18 by
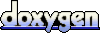