
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
example1.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 */ 00018 00019 /** @file example1.cpp Test case to demonstrates each API function works correctly. 00020 * 00021 * \par Example 1 Notes 00022 * 00023 * The example test does the following CFSTORE operations: 00024 * - initialises 00025 * - creates a key-value pair (KV). 00026 * - writes the data for the KV 00027 * - closes KV. 00028 * - flushes the KV to flash 00029 * - opens KV for reading. 00030 * - reads KV and checks the value blob was the same as previously written. 00031 * - closes KV. 00032 * - finds a KV (there is only 1 to find). 00033 * - for the KV returned, get the key name. 00034 * - for the KV returned, get the value length. 00035 * - for the KV returned, delete the KV. 00036 * - find another KV (which fails as there are no more keys to find). 00037 * - flushes the updated state to flash to store the removal of the deleted KV. 00038 * - uninitialises 00039 * - stops 00040 * 00041 * This test is coded so as to work in the following modes: 00042 * - flash sync mode i.e. with caps.asynchronous_ops == false 00043 * - flash async mode i.e. with caps.asynchronous_ops == true 00044 * 00045 * The dual async/sync mode support with the same code is more complicated 00046 * than if the implementation just supported sync mode for example. However, 00047 * it has the benefit of being more versatile. 00048 * 00049 * The test leaves the flash in the same state as at the beginning of the test so 00050 * it can be run a second time on the device without flashing, and the test should 00051 * still work. 00052 * 00053 * \par How to Build Example1 as a Stand-alone Application 00054 * 00055 * This example can be build as a stand-alone application as follows: 00056 * - Create a new mbed application using the `mbed new .` command. 00057 * - Copy this file example1.cpp from the to the top level application directory and rename the file to main.cpp. 00058 * - Build the application with `mbed compile -v -m <target> -t <toolchain> -DCFSTORE_EXAMPLE1_APP` e.g. `mbed compile -v -m K64F -t GCC_ARM -DCFSTORE_EXAMPLE1_APP`. 00059 */ 00060 #if defined __MBED__ && ! defined TOOLCHAIN_GCC_ARM 00061 00062 #include "mbed.h" 00063 #include "cfstore_config.h" 00064 #include "Driver_Common.h" 00065 #include "cfstore_debug.h" 00066 #include "cfstore_test.h" 00067 #include "configuration_store.h" 00068 #include "utest/utest.h" 00069 #include "unity/unity.h" 00070 #include "greentea-client/test_env.h" 00071 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00072 #include "uvisor-lib/uvisor-lib.h" 00073 #include "cfstore_uvisor.h" 00074 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00075 00076 #include <stdio.h> 00077 #include <stdlib.h> 00078 #include <string.h> 00079 #include <inttypes.h> 00080 00081 using namespace utest::v1; 00082 00083 static control_t cfstore_example1_test_00(const size_t call_count) 00084 { 00085 (void) call_count; 00086 printf("Not implemented for ARM toolchain\n"); 00087 return CaseNext; 00088 } 00089 00090 00091 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00092 { 00093 GREENTEA_SETUP(100, "default_auto"); 00094 return greentea_test_setup_handler(number_of_cases); 00095 } 00096 00097 Case cases[] = { 00098 /* 1 2 3 4 5 6 7 */ 00099 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00100 Case("EXAMPLE1_test_00", cfstore_example1_test_00), 00101 }; 00102 00103 00104 /* Declare your test specification with a custom setup handler */ 00105 Specification specification(greentea_setup, cases); 00106 00107 int main() 00108 { 00109 return !Harness::run(specification); 00110 } 00111 00112 00113 #else 00114 00115 00116 #include "mbed.h" 00117 00118 #ifndef CFSTORE_EXAMPLE1_APP 00119 /* when built as Configuration-Store example, include greentea support otherwise omit */ 00120 #include "utest/utest.h" 00121 #include "unity/unity.h" 00122 #include "greentea-client/test_env.h" 00123 00124 #else // CFSTORE_EXAMPLE1_APP 00125 // map utest types for building as stand alone example 00126 #define control_t void 00127 #define CaseNext 00128 #endif // CFSTORE_EXAMPLE1_APP 00129 00130 #include "cfstore_config.h" 00131 #include "configuration_store.h" 00132 00133 #ifdef CFSTORE_CONFIG_BACKEND_FLASH_ENABLED 00134 #include "flash_journal_strategy_sequential.h" 00135 #include "flash_journal.h" 00136 #include "Driver_Common.h" 00137 #endif /* CFSTORE_CONFIG_BACKEND_FLASH_ENABLED */ 00138 00139 #ifdef YOTTA_CFG_CONFIG_UVISOR 00140 #include "uvisor-lib/uvisor-lib.h" 00141 #endif /* YOTTA_CFG_CONFIG_UVISOR */ 00142 00143 #include <stdio.h> 00144 #include <stdlib.h> 00145 #include <string.h> 00146 00147 /// @cond CFSTORE_DOXYGEN_DISABLE 00148 #ifndef CFSTORE_EXAMPLE1_APP 00149 using namespace utest::v1; 00150 #endif 00151 00152 00153 #define CFSTORE_EX1_TEST_ASSERT(Expr) if (!(Expr)) { printf("%s:%u: assertion failure\r\n", __FUNCTION__, __LINE__); while (1) ;} 00154 #define CFSTORE_EX1_TEST_ASSERT_EQUAL(expected, actual) if ((expected) != (actual)) {printf("%s:%u: assertion failure\r\n", __FUNCTION__, __LINE__); while (1) ;} 00155 #define CFSTORE_EX1_TEST_ASSERT_NOT_EQUAL(expected, actual) if ((expected) == (actual)) {printf("%s:%u: assertion failure\r\n", __FUNCTION__, __LINE__); while (1) ;} 00156 00157 #define CFSTORE_EX1_TEST_ASSERT_MSG(Expr, _fmt, ...) \ 00158 do \ 00159 { \ 00160 if (!(Expr)) \ 00161 { \ 00162 printf(_fmt, __VA_ARGS__); \ 00163 while (1) ; \ 00164 } \ 00165 }while(0); 00166 00167 #define CFSTORE_EX1_LOG(_fmt, ...) \ 00168 do \ 00169 { \ 00170 printf(_fmt, __VA_ARGS__); \ 00171 }while(0); 00172 00173 00174 const char* cfstore_ex_opcode_str[] = 00175 { 00176 "UNDEFINED", 00177 "CFSTORE_OPCODE_CLOSE", 00178 "CFSTORE_OPCODE_CREATE", 00179 "CFSTORE_OPCODE_DELETE", 00180 "CFSTORE_OPCODE_FIND", 00181 "CFSTORE_OPCODE_FLUSH", 00182 "CFSTORE_OPCODE_GET_KEY_NAME", 00183 "CFSTORE_OPCODE_GET_STATUS", 00184 "CFSTORE_OPCODE_GET_VALUE_LEN", 00185 "CFSTORE_OPCODE_INITIALIZE", 00186 "CFSTORE_OPCODE_OPEN", 00187 "CFSTORE_OPCODE_POWER_CONTROL", 00188 "CFSTORE_OPCODE_READ", 00189 "CFSTORE_OPCODE_RSEEK", 00190 "CFSTORE_OPCODE_UNINITIALIZE", 00191 "CFSTORE_OPCODE_WRITE", 00192 "CFSTORE_OPCODE_MAX" 00193 }; 00194 00195 bool cfstore_example1_done = false; 00196 const char* cfstore_ex_kv_name = "basement.medicine.pavement.government.trenchcoat.off.cough.off.kid.did.when.again.alleyway.friend.cap.pen.dollarbills.ten.foot.soot.put.but.anyway.say.May.DA.kid.did.toes.bows.those.hose.nose.clothes.man.blows.well.well"; 00197 const char* cfstore_ex_kv_value = "TheRollingStone"; 00198 #define CFSTORE_EX1_RSEEK_OFFSET 10 /* offset to S of Stone */ 00199 00200 typedef enum cfstore_ex_state_t { 00201 CFSTORE_EX_STATE_INITIALIZING = 1, 00202 CFSTORE_EX_STATE_INIT_DONE, 00203 CFSTORE_EX_STATE_CREATING, 00204 CFSTORE_EX_STATE_CREATE_DONE, 00205 CFSTORE_EX_STATE_WRITING, 00206 CFSTORE_EX_STATE_WRITE_DONE, 00207 CFSTORE_EX_STATE_CLOSING1, 00208 CFSTORE_EX_STATE_CLOSE_DONE1, 00209 CFSTORE_EX_STATE_FLUSHING1, 00210 CFSTORE_EX_STATE_FLUSH_DONE1, 00211 CFSTORE_EX_STATE_OPENING, 00212 CFSTORE_EX_STATE_OPEN_DONE, 00213 CFSTORE_EX_STATE_READING1, 00214 CFSTORE_EX_STATE_READ_DONE1, 00215 CFSTORE_EX_STATE_RSEEKING, 00216 CFSTORE_EX_STATE_RSEEK_DONE, 00217 CFSTORE_EX_STATE_READING2, 00218 CFSTORE_EX_STATE_READ_DONE2, 00219 CFSTORE_EX_STATE_CLOSING2, 00220 CFSTORE_EX_STATE_CLOSE_DONE2, 00221 CFSTORE_EX_STATE_FINDING1, 00222 CFSTORE_EX_STATE_FIND_DONE1, 00223 CFSTORE_EX_STATE_GETTING_KEY_NAME, 00224 CFSTORE_EX_STATE_GET_KEY_NAME_DONE, 00225 CFSTORE_EX_STATE_GETTING_VALUE_LEN, 00226 CFSTORE_EX_STATE_GET_VALUE_LEN_DONE, 00227 CFSTORE_EX_STATE_DELETING, 00228 CFSTORE_EX_STATE_DELETE_DONE, 00229 CFSTORE_EX_STATE_FINDING2, 00230 CFSTORE_EX_STATE_FIND_DONE2, 00231 CFSTORE_EX_STATE_FLUSHING2, 00232 CFSTORE_EX_STATE_FLUSH_DONE2, 00233 CFSTORE_EX_STATE_UNINITIALIZING, 00234 CFSTORE_EX_STATE_UNINIT_DONE 00235 } cfstore_ex_state_t; 00236 00237 typedef struct cfstore_example1_ctx_t 00238 { 00239 ARM_CFSTORE_CAPABILITIES caps; 00240 uint8_t hkey[CFSTORE_HANDLE_BUFSIZE]; 00241 uint8_t hkey_next_buf[CFSTORE_HANDLE_BUFSIZE]; 00242 uint8_t hkey_prev_buf[CFSTORE_HANDLE_BUFSIZE]; 00243 ARM_CFSTORE_HANDLE hkey_next; 00244 ARM_CFSTORE_HANDLE hkey_prev; 00245 cfstore_ex_state_t state; 00246 ARM_CFSTORE_SIZE len; 00247 ARM_CFSTORE_KEYDESC kdesc; 00248 ARM_CFSTORE_FMODE flags; 00249 char value[CFSTORE_KEY_NAME_MAX_LENGTH+1]; 00250 /* callback attributes*/ 00251 int32_t callback_status; 00252 ARM_CFSTORE_HANDLE callback_handle; 00253 } cfstore_example1_ctx_t; 00254 00255 static cfstore_example1_ctx_t cfstore_example1_ctx_g; 00256 00257 extern ARM_CFSTORE_DRIVER cfstore_driver; 00258 ARM_CFSTORE_DRIVER *cfstore_drv = &cfstore_driver; 00259 00260 /* forward declarations */ 00261 static void cfstore_ex_fms_update(cfstore_example1_ctx_t* ctx); 00262 /// @endcond 00263 00264 00265 #ifdef CFSTORE_CONFIG_BACKEND_FLASH_ENABLED 00266 00267 #define CFSTORE_FLASH_START_FORMAT (FLASH_JOURNAL_OPCODE_RESET + 0x00010000) 00268 00269 typedef enum cfstore_ex_flash_state_t { 00270 CFSTORE_EX_FLASH_STATE_STARTING = 1, 00271 CFSTORE_EX_FLASH_STATE_FORMATTING, 00272 CFSTORE_EX_FLASH_STATE_INITIALIZING, 00273 CFSTORE_EX_FLASH_STATE_RESETTING, 00274 CFSTORE_EX_FLASH_STATE_READY, 00275 } cfstore_ex_flash_state_t; 00276 00277 typedef struct cfstore_example1_flash_ctx_t 00278 { 00279 volatile cfstore_ex_flash_state_t state; 00280 } cfstore_example1_flash_ctx_t; 00281 00282 static cfstore_example1_flash_ctx_t cfstore_example1_flash_ctx_g; 00283 00284 00285 static void cfstore_ex_flash_journal_callback(int32_t status, FlashJournal_OpCode_t cmd_code) 00286 { 00287 static FlashJournal_t jrnl; 00288 extern ARM_DRIVER_STORAGE ARM_Driver_Storage_MTD_K64F; 00289 const ARM_DRIVER_STORAGE *drv = &ARM_Driver_Storage_MTD_K64F; 00290 00291 00292 if(cmd_code == (FlashJournal_OpCode_t) CFSTORE_FLASH_START_FORMAT) { 00293 CFSTORE_EX1_LOG("FORMATTING%s", "\n"); 00294 status = flashJournalStrategySequential_format(drv, 4, cfstore_ex_flash_journal_callback); 00295 CFSTORE_EX1_TEST_ASSERT_MSG(status >= JOURNAL_STATUS_OK, "%s:Error: FlashJournal_format() failed (status=%d)\r\n", __func__, (int) status); 00296 if(status == 0) { 00297 /* async completion pending */ 00298 return; 00299 } 00300 /* status > 0 implies operation completed synchronously 00301 * intentional fall through */ 00302 } 00303 00304 switch(cmd_code) 00305 { 00306 case FLASH_JOURNAL_OPCODE_FORMAT: 00307 /* format done */ 00308 CFSTORE_EX1_TEST_ASSERT_MSG(status > JOURNAL_STATUS_OK, "%s:Error: FlashJournal_format() failed (status=%d)\r\n", __func__, (int) status); 00309 cfstore_example1_flash_ctx_g.state = CFSTORE_EX_FLASH_STATE_INITIALIZING; 00310 00311 CFSTORE_EX1_LOG("FLASH INITIALIZING%s", "\n"); 00312 status = FlashJournal_initialize(&jrnl, drv, &FLASH_JOURNAL_STRATEGY_SEQUENTIAL, NULL); 00313 CFSTORE_EX1_TEST_ASSERT_MSG(status >= JOURNAL_STATUS_OK, "%s:Error: FlashJournal_initialize() failed (status=%d)\r\n", __func__, (int) status); 00314 if(status == 0) { 00315 /* async completion pending */ 00316 break; 00317 } 00318 /* status > 0 implies operation completed synchronously 00319 * intentional fall through */ 00320 case FLASH_JOURNAL_OPCODE_INITIALIZE: 00321 /* initialize done */ 00322 CFSTORE_EX1_TEST_ASSERT_MSG(status > JOURNAL_STATUS_OK, "%s:Error: FlashJournal_initialize() failed (status=%d)\r\n", __func__, (int) status); 00323 cfstore_example1_flash_ctx_g.state = CFSTORE_EX_FLASH_STATE_RESETTING; 00324 00325 CFSTORE_EX1_LOG("FLASH RESETTING%s", "\n"); 00326 status = FlashJournal_reset(&jrnl); 00327 CFSTORE_EX1_TEST_ASSERT_MSG(status >= JOURNAL_STATUS_OK, "%s:Error: FlashJournal_reset() failed (status=%d)\r\n", __func__, (int) status); 00328 /* intentional fall through */ 00329 case FLASH_JOURNAL_OPCODE_RESET: 00330 /* reset done */ 00331 CFSTORE_EX1_LOG("FLASH RESET DONE%s", "\n"); 00332 cfstore_example1_flash_ctx_g.state = CFSTORE_EX_FLASH_STATE_READY; 00333 break; 00334 00335 default: 00336 CFSTORE_EX1_LOG("%s:Error: notification of unsupported cmd_code event (status=%d, cmd_code=%d)\n", __func__, (int) status, (int) cmd_code); 00337 return; 00338 } 00339 return; 00340 } 00341 #endif /* CFSTORE_CONFIG_BACKEND_FLASH_ENABLED */ 00342 00343 00344 00345 /* @brief test startup code to reset flash for testing purposes. 00346 */ 00347 static int32_t cfstore_test_startup(void) 00348 { 00349 ARM_CFSTORE_CAPABILITIES caps = cfstore_driver.GetCapabilities(); 00350 CFSTORE_EX1_LOG("INITIALIZING: caps.asynchronous_ops=%d\n", (int) caps.asynchronous_ops); 00351 00352 #ifdef CFSTORE_CONFIG_BACKEND_FLASH_ENABLED 00353 00354 memset(&cfstore_example1_flash_ctx_g, 0, sizeof(cfstore_example1_flash_ctx_g)); 00355 cfstore_example1_flash_ctx_g.state = CFSTORE_EX_FLASH_STATE_STARTING; 00356 cfstore_ex_flash_journal_callback(JOURNAL_STATUS_OK, (FlashJournal_OpCode_t) CFSTORE_FLASH_START_FORMAT); 00357 while(cfstore_example1_flash_ctx_g.state != CFSTORE_EX_FLASH_STATE_READY) { 00358 /* spin */ 00359 } 00360 00361 #endif /* CFSTORE_CONFIG_BACKEND_FLASH_ENABLED */ 00362 00363 return ARM_DRIVER_OK; 00364 } 00365 00366 00367 static void cfstore_ex_callback(int32_t status, ARM_CFSTORE_OPCODE cmd_code, void *client_context, ARM_CFSTORE_HANDLE handle) 00368 { 00369 (void) handle; 00370 00371 cfstore_example1_ctx_t* ctx = (cfstore_example1_ctx_t*) client_context; 00372 00373 /* CFSTORE_EX1_LOG("%s:entered: status=%d, cmd_code=%d (%s) handle=%p\r\n", __func__, (int) status, (int) cmd_code, cfstore_ex_opcode_str[cmd_code], handle); */ 00374 switch(cmd_code) 00375 { 00376 case CFSTORE_OPCODE_INITIALIZE: 00377 ctx->state = CFSTORE_EX_STATE_INIT_DONE; 00378 break; 00379 case CFSTORE_OPCODE_CREATE: 00380 ctx->state = CFSTORE_EX_STATE_CREATE_DONE; 00381 break; 00382 case CFSTORE_OPCODE_WRITE: 00383 ctx->state = CFSTORE_EX_STATE_WRITE_DONE; 00384 break; 00385 case CFSTORE_OPCODE_CLOSE: 00386 switch(ctx->state) 00387 { 00388 case(CFSTORE_EX_STATE_CLOSING1): 00389 ctx->state = CFSTORE_EX_STATE_CLOSE_DONE1; 00390 break; 00391 case(CFSTORE_EX_STATE_CLOSING2): 00392 ctx->state = CFSTORE_EX_STATE_CLOSE_DONE2; 00393 break; 00394 default: 00395 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown Close() error (line=%u)\r\n", __func__, __LINE__); 00396 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00397 * and hence is commented out. Re-instate if previous assert is removed. 00398 * break; */ 00399 } 00400 break; 00401 case CFSTORE_OPCODE_FLUSH: 00402 switch(ctx->state) 00403 { 00404 case(CFSTORE_EX_STATE_FLUSHING1): 00405 ctx->state = CFSTORE_EX_STATE_FLUSH_DONE1; 00406 break; 00407 case(CFSTORE_EX_STATE_FLUSHING2): 00408 ctx->state = CFSTORE_EX_STATE_FLUSH_DONE2; 00409 break; 00410 default: 00411 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown Find() error (line=%u)\r\n", __func__, __LINE__); 00412 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00413 * and hence is commented out. Re-instate if previous assert is removed. 00414 * break; */ 00415 } 00416 break; 00417 case CFSTORE_OPCODE_OPEN: 00418 ctx->state = CFSTORE_EX_STATE_OPEN_DONE; 00419 break; 00420 case CFSTORE_OPCODE_FIND: 00421 switch(ctx->state) 00422 { 00423 case(CFSTORE_EX_STATE_FINDING1): 00424 ctx->state = CFSTORE_EX_STATE_FIND_DONE1; 00425 break; 00426 case(CFSTORE_EX_STATE_FINDING2): 00427 ctx->state = CFSTORE_EX_STATE_FIND_DONE2; 00428 break; 00429 default: 00430 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown Find() error (line=%u)\r\n", __func__, __LINE__); 00431 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00432 * and hence is commented out. Re-instate if previous assert is removed. 00433 * break; */ 00434 } 00435 break; 00436 case CFSTORE_OPCODE_GET_KEY_NAME: 00437 ctx->state = CFSTORE_EX_STATE_GET_KEY_NAME_DONE; 00438 break; 00439 case CFSTORE_OPCODE_GET_VALUE_LEN: 00440 ctx->state = CFSTORE_EX_STATE_GET_VALUE_LEN_DONE; 00441 break; 00442 case CFSTORE_OPCODE_READ: 00443 switch(ctx->state) 00444 { 00445 case(CFSTORE_EX_STATE_READING1): 00446 ctx->state = CFSTORE_EX_STATE_READ_DONE1; 00447 break; 00448 case(CFSTORE_EX_STATE_READING2): 00449 ctx->state = CFSTORE_EX_STATE_READ_DONE2; 00450 break; 00451 default: 00452 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown Read() error (line=%u)\r\n", __func__, __LINE__); 00453 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00454 * and hence is commented out. Re-instate if previous assert is removed. 00455 * break; */ 00456 } 00457 break; 00458 case CFSTORE_OPCODE_RSEEK: 00459 ctx->state = CFSTORE_EX_STATE_RSEEK_DONE; 00460 break; 00461 case CFSTORE_OPCODE_DELETE: 00462 ctx->state = CFSTORE_EX_STATE_DELETE_DONE; 00463 break; 00464 case CFSTORE_OPCODE_UNINITIALIZE: 00465 ctx->state = CFSTORE_EX_STATE_UNINIT_DONE; 00466 break; 00467 case CFSTORE_OPCODE_GET_STATUS: 00468 case CFSTORE_OPCODE_POWER_CONTROL: 00469 default: 00470 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: received asynchronous notification for opcode=%d (%s)\r\b", __func__, cmd_code, cmd_code < CFSTORE_OPCODE_MAX ? cfstore_ex_opcode_str[cmd_code] : "unknown"); 00471 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00472 * and hence is commented out. Re-instate if previous assert is removed. 00473 * break; */ 00474 } 00475 ctx->callback_status = status; 00476 ctx->callback_handle = handle; 00477 cfstore_ex_fms_update(ctx); 00478 return; 00479 } 00480 00481 static void cfstore_ex_fms_update(cfstore_example1_ctx_t* ctx) 00482 { 00483 int32_t ret; 00484 00485 switch (ctx->state) 00486 { 00487 case CFSTORE_EX_STATE_INITIALIZING: 00488 CFSTORE_EX1_LOG("INITIALIZING%s", "\r\n"); 00489 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_INITIALIZE can be invoked before Initialize() has returned 00490 ret = cfstore_drv->Initialize(cfstore_ex_callback, ctx); 00491 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Initialize() should return ret >= 0 for async/synch modes(ret=%ld)\r\n", __func__, ret); 00492 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00493 ctx->state = CFSTORE_EX_STATE_INIT_DONE; 00494 break; 00495 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00496 // await pending notification of completion. 00497 break; 00498 } 00499 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00500 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00501 * and hence is commented out. Re-instate if previous assert is removed. 00502 * break; */ 00503 00504 case CFSTORE_EX_STATE_INIT_DONE: 00505 CFSTORE_EX1_LOG("INIT_DONE%s", "\r\n"); 00506 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Initialize() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00507 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_INITIALIZE) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00508 ctx->state = CFSTORE_EX_STATE_CREATING; 00509 // intentional fall-through 00510 00511 case CFSTORE_EX_STATE_CREATING: 00512 CFSTORE_EX1_LOG("CREATING%s", "\r\n"); 00513 memset(&ctx->kdesc, 0, sizeof(ARM_CFSTORE_KEYDESC)); 00514 ctx->kdesc.drl = ARM_RETENTION_NVM; 00515 ctx->len = strlen(cfstore_ex_kv_value); 00516 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_CREATE can be invoked before Create() has returned 00517 ret = cfstore_drv->Create(cfstore_ex_kv_name, ctx->len, &ctx->kdesc, ctx->hkey); 00518 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Create() failed (ret=%ld)\r\n", __func__, ret); 00519 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00520 ctx->state = CFSTORE_EX_STATE_CREATE_DONE; 00521 break; 00522 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00523 // await pending notification of completion. 00524 break; 00525 } 00526 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00527 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00528 * and hence is commented out. Re-instate if previous assert is removed. 00529 * break; */ 00530 00531 case CFSTORE_EX_STATE_CREATE_DONE: 00532 CFSTORE_EX1_LOG("CREATE_DONE%s", "\r\n"); 00533 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Create() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00534 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_CREATE) received handle (%p) is not the hkey supplied to Create()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey); 00535 ctx->state = CFSTORE_EX_STATE_WRITING; 00536 // intentional fall-through 00537 00538 case CFSTORE_EX_STATE_WRITING: 00539 CFSTORE_EX1_LOG("WRITING%s", "\r\n"); 00540 ctx->len = strlen(cfstore_ex_kv_value); 00541 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_WRITE can be invoked before Write() has returned 00542 ret = cfstore_drv->Write(ctx->hkey, cfstore_ex_kv_value, &ctx->len); 00543 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Write() failed (ret=%ld)\r\n", __func__, ret); 00544 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00545 ctx->state = CFSTORE_EX_STATE_WRITE_DONE; 00546 break; 00547 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00548 // await pending notification of completion. 00549 break; 00550 } 00551 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00552 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00553 * and hence is commented out. Re-instate if previous assert is removed. 00554 * break; */ 00555 00556 case CFSTORE_EX_STATE_WRITE_DONE: 00557 CFSTORE_EX1_LOG("WRITE_DONE%s", "\r\n"); 00558 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Write() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00559 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == (int32_t) strlen(cfstore_ex_kv_value), "%s:Error: Write() number of octets written (i.e. completion status (%ld)) != strlen(ctx->value)(%ld)\r\n", __func__, ctx->callback_status, (int32_t) strlen(cfstore_ex_kv_value)); 00560 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == (int32_t) ctx->len, "%s:Error: Write() number of octets written (i.e. completion status (%ld)) != updated value of len parameter (%ld)\r\n", __func__, ctx->callback_status, (int32_t) ctx->len); 00561 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_WRITE) received handle (%p) is not the hkey supplied to Write()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey); 00562 ctx->state = CFSTORE_EX_STATE_CLOSING1; 00563 // intentional fall-through 00564 00565 case CFSTORE_EX_STATE_CLOSING1: 00566 CFSTORE_EX1_LOG("CLOSING1%s", "\r\n"); 00567 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_CLOSE can be invoked before Close() has returned 00568 ret = cfstore_drv->Close(ctx->hkey); 00569 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Close() failed (ret=%ld)\r\n", __func__, ret); 00570 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00571 ctx->state = CFSTORE_EX_STATE_CLOSE_DONE1; 00572 break; 00573 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00574 // await pending notification of completion. 00575 break; 00576 } 00577 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00578 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00579 * and hence is commented out. Re-instate if previous assert is removed. 00580 * break; */ 00581 00582 case CFSTORE_EX_STATE_CLOSE_DONE1: 00583 CFSTORE_EX1_LOG("CLOSE_DONE1%s", "\r\n"); 00584 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Close() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00585 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_CLOSE) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00586 ctx->state = CFSTORE_EX_STATE_FLUSHING1; 00587 // intentional fall-through 00588 00589 case CFSTORE_EX_STATE_FLUSHING1: 00590 CFSTORE_EX1_LOG("FLUSHING1%s", "\r\n"); 00591 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_FLUSH can be invoked before Flush() has returned 00592 ret = cfstore_drv->Flush(); 00593 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Flush() failed (ret=%ld)\r\n", __func__, ret); 00594 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00595 ctx->state = CFSTORE_EX_STATE_FLUSH_DONE1; 00596 break; 00597 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00598 // await pending notification of completion. 00599 break; 00600 } 00601 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00602 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00603 * and hence is commented out. Re-instate if previous assert is removed. 00604 * break; */ 00605 00606 case CFSTORE_EX_STATE_FLUSH_DONE1: 00607 CFSTORE_EX1_LOG("FLUSH_DONE1%s", "\r\n"); 00608 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Flush() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00609 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_FLUSH) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00610 ctx->state = CFSTORE_EX_STATE_OPENING; 00611 // intentional fall-through 00612 00613 case CFSTORE_EX_STATE_OPENING: 00614 CFSTORE_EX1_LOG("OPENING%s", "\r\n"); 00615 memset(&ctx->flags, 0, sizeof(ctx->flags)); 00616 memset(&ctx->hkey, 0, CFSTORE_HANDLE_BUFSIZE); 00617 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_OPEN can be invoked before Open() has returned 00618 ret = cfstore_drv->Open(cfstore_ex_kv_name, ctx->flags, ctx->hkey); 00619 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Open() failed (ret=%ld)\r\n", __func__, ret); 00620 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00621 ctx->state = CFSTORE_EX_STATE_OPEN_DONE; 00622 break; 00623 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00624 // await pending notification of completion. 00625 break; 00626 } 00627 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00628 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00629 * and hence is commented out. Re-instate if previous assert is removed. 00630 * break; */ 00631 00632 case CFSTORE_EX_STATE_OPEN_DONE: 00633 CFSTORE_EX1_LOG("OPEN_DONE%s", "\r\n"); 00634 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Open() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00635 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_OPEN) received handle (%p) is not the hkey supplied to Open()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey); 00636 ctx->state = CFSTORE_EX_STATE_READING1; 00637 // intentional fall-through 00638 00639 case CFSTORE_EX_STATE_READING1: 00640 CFSTORE_EX1_LOG("READING1%s", "\r\n"); 00641 ctx->len = CFSTORE_KEY_NAME_MAX_LENGTH; 00642 memset(ctx->value, 0, CFSTORE_KEY_NAME_MAX_LENGTH+1); 00643 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_READ can be invoked before Read() has returned 00644 ret = cfstore_drv->Read(ctx->hkey, ctx->value, &ctx->len); 00645 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Read() failed (ret=%ld)\r\n", __func__, ret); 00646 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00647 ctx->state = CFSTORE_EX_STATE_READ_DONE1; 00648 break; 00649 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00650 // await pending notification of completion. 00651 break; 00652 } 00653 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00654 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00655 * and hence is commented out. Re-instate if previous assert is removed. 00656 * break; */ 00657 00658 case CFSTORE_EX_STATE_READ_DONE1: 00659 CFSTORE_EX1_LOG("READ_DONE1%s", "\r\n"); 00660 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Read() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00661 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == (int32_t) strlen(cfstore_ex_kv_value), "%s:Error: Read() number of octets read (i.e. completion status (%ld)) != strlen(ctx->value)(%ld)\r\n", __func__, ctx->callback_status, (int32_t) strlen(cfstore_ex_kv_value)); 00662 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == (int32_t) ctx->len, "%s:Error: Read() number of octets read (i.e. completion status (%ld)) != updated value of len parameter (%ld)\r\n", __func__, ctx->callback_status, (int32_t) ctx->len); 00663 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_READ) received handle (%p) is not the hkey supplied to Read()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey); 00664 CFSTORE_EX1_TEST_ASSERT_MSG(strncmp(ctx->value, cfstore_ex_kv_value, strlen(cfstore_ex_kv_value)) == 0, "%s:Error: the read value (%s) is not as expected (%s)\r\n", __func__, ctx->value, cfstore_ex_kv_value); 00665 ctx->state = CFSTORE_EX_STATE_RSEEKING; 00666 // intentional fall-through 00667 00668 case CFSTORE_EX_STATE_RSEEKING: 00669 CFSTORE_EX1_LOG("RSEEKING%s", "\r\n"); 00670 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_READ can be invoked before Read() has returned 00671 ret = cfstore_drv->Rseek(ctx->hkey, CFSTORE_EX1_RSEEK_OFFSET); 00672 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Rseek() failed (ret=%ld)\r\n", __func__, ret); 00673 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00674 ctx->state = CFSTORE_EX_STATE_RSEEK_DONE; 00675 break; 00676 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00677 // await pending notification of completion. 00678 break; 00679 } 00680 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00681 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00682 * and hence is commented out. Re-instate if previous assert is removed. 00683 * break; */ 00684 00685 case CFSTORE_EX_STATE_RSEEK_DONE: 00686 CFSTORE_EX1_LOG("RSEEK_DONE%s", "\r\n"); 00687 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Read() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00688 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_RSEEK) received handle (%p) is not the hkey supplied to Read()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey); 00689 ctx->state = CFSTORE_EX_STATE_READING2; 00690 // intentional fall-through 00691 00692 case CFSTORE_EX_STATE_READING2: 00693 CFSTORE_EX1_LOG("READING2%s", "\r\n"); 00694 ctx->len = CFSTORE_KEY_NAME_MAX_LENGTH; 00695 memset(ctx->value, 0, CFSTORE_KEY_NAME_MAX_LENGTH+1); 00696 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_READ can be invoked before Read() has returned 00697 ret = cfstore_drv->Read(ctx->hkey, ctx->value, &ctx->len); 00698 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Read() failed (ret=%ld)\r\n", __func__, ret); 00699 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00700 ctx->state = CFSTORE_EX_STATE_READ_DONE2; 00701 break; 00702 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00703 // await pending notification of completion. 00704 break; 00705 } 00706 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00707 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00708 * and hence is commented out. Re-instate if previous assert is removed. 00709 * break; */ 00710 00711 case CFSTORE_EX_STATE_READ_DONE2: 00712 CFSTORE_EX1_LOG("READ_DONE2%s", "\r\n"); 00713 CFSTORE_EX1_LOG("%s: value=%s\r\n", __func__, ctx->value); 00714 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Read() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00715 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == (int32_t) strlen(&cfstore_ex_kv_value[CFSTORE_EX1_RSEEK_OFFSET]), "%s:Error: Read() number of octets read (i.e. completion status (%ld)) != strlen(ctx->value)(%ld)\r\n", __func__, ctx->callback_status, (int32_t) strlen(&cfstore_ex_kv_value[CFSTORE_EX1_RSEEK_OFFSET])); 00716 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == (int32_t) ctx->len, "%s:Error: Read() number of octets read (i.e. completion status (%ld)) != updated value of len parameter (%ld)\r\n", __func__, ctx->callback_status, (int32_t) ctx->len); 00717 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_READ) received handle (%p) is not the hkey supplied to Read()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey); 00718 CFSTORE_EX1_TEST_ASSERT_MSG(strncmp(ctx->value, &cfstore_ex_kv_value[CFSTORE_EX1_RSEEK_OFFSET], strlen(&cfstore_ex_kv_value[CFSTORE_EX1_RSEEK_OFFSET])) == 0, "%s:Error: the read value (%s) is not as expected (%s)\r\n", __func__, ctx->value, &cfstore_ex_kv_value[CFSTORE_EX1_RSEEK_OFFSET]); 00719 ctx->state = CFSTORE_EX_STATE_CLOSING2; 00720 // intentional fall-through 00721 00722 case CFSTORE_EX_STATE_CLOSING2: 00723 CFSTORE_EX1_LOG("CLOSING2%s", "\r\n"); 00724 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_CLOSE can be invoked before Close() has returned 00725 ret = cfstore_drv->Close(ctx->hkey); 00726 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Close() failed (ret=%ld)\r\n", __func__, ret); 00727 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00728 ctx->state = CFSTORE_EX_STATE_CLOSE_DONE2; 00729 break; 00730 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00731 // await pending notification of completion. 00732 break; 00733 } 00734 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00735 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00736 * and hence is commented out. Re-instate if previous assert is removed. 00737 * break; */ 00738 00739 case CFSTORE_EX_STATE_CLOSE_DONE2: 00740 CFSTORE_EX1_LOG("CLOSE_DONE2%s", "\r\n"); 00741 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Close() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00742 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_CLOSE) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00743 ctx->state = CFSTORE_EX_STATE_FINDING1; 00744 // intentional fall-through 00745 00746 case CFSTORE_EX_STATE_FINDING1: 00747 CFSTORE_EX1_LOG("FINDING1%s", "\r\n"); 00748 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_FIND can be invoked before Find() has returned 00749 ret = cfstore_drv->Find("*", ctx->hkey_next, ctx->hkey_prev); 00750 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Find() failed (ret=%ld)\r\n", __func__, ret); 00751 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00752 ctx->state = CFSTORE_EX_STATE_FIND_DONE1; 00753 break; 00754 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00755 // await pending notification of completion. 00756 break; 00757 } 00758 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00759 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00760 * and hence is commented out. Re-instate if previous assert is removed. 00761 * break; */ 00762 00763 case CFSTORE_EX_STATE_FIND_DONE1: 00764 CFSTORE_EX1_LOG("FIND_DONE1%s", "\r\n"); 00765 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == ARM_DRIVER_OK, "%s:Error: Find() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00766 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey_prev, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_FIND) received handle (%p) is not the hkey supplied to Find()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey_prev); 00767 ctx->state = CFSTORE_EX_STATE_GETTING_KEY_NAME; 00768 // intentional fall-through 00769 00770 case CFSTORE_EX_STATE_GETTING_KEY_NAME: 00771 CFSTORE_EX1_LOG("GETTING_KEY_NAME%s", "\r\n"); 00772 ctx->len = CFSTORE_KEY_NAME_MAX_LENGTH; 00773 memset(ctx->value, 0, CFSTORE_KEY_NAME_MAX_LENGTH+1); 00774 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_GET_KEY_NAME can be invoked before GetKeyName() has returned 00775 ret = cfstore_drv->GetKeyName(ctx->hkey_prev, ctx->value, (uint8_t*) &ctx->len); 00776 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: GetKeyName() failed (ret=%ld)\r\n", __func__, ret); 00777 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00778 ctx->state = CFSTORE_EX_STATE_GET_KEY_NAME_DONE; 00779 break; 00780 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00781 // await pending notification of completion. 00782 break; 00783 } 00784 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00785 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00786 * and hence is commented out. Re-instate if previous assert is removed. 00787 * break; */ 00788 00789 case CFSTORE_EX_STATE_GET_KEY_NAME_DONE: 00790 CFSTORE_EX1_LOG("GET_KEY_NAME_DONE%s", "\r\n"); 00791 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: GetKeyName() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00792 CFSTORE_EX1_TEST_ASSERT_MSG( ((int32_t) ctx->len == ((int32_t) strlen(cfstore_ex_kv_name)+1)), "%s:Error: GetKeyName() updated value of len parameter (%ld) != strlen(cfstore_ex_kv_name) (%ld) (\r\n", __func__, (int32_t) ctx->len, (int32_t) strlen(cfstore_ex_kv_name)); 00793 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey_prev, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_GET_KEY_NAME) received handle (%p) is not the hkey supplied to GetKeyName()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey_prev); 00794 CFSTORE_EX1_TEST_ASSERT_MSG(strncmp(ctx->value, cfstore_ex_kv_name, strlen(cfstore_ex_kv_name)) == 0, "%s:Error: the key name (%s) is not as expected (%s)\r\n", __func__, ctx->value, cfstore_ex_kv_name); 00795 ctx->state = CFSTORE_EX_STATE_GETTING_VALUE_LEN; 00796 // intentional fall-through 00797 00798 case CFSTORE_EX_STATE_GETTING_VALUE_LEN: 00799 CFSTORE_EX1_LOG("GETTING_VALUE_LEN%s", "\r\n"); 00800 ctx->len = CFSTORE_KEY_NAME_MAX_LENGTH; 00801 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_GET_VALUE_LEN can be invoked before GetValueLen() has returned 00802 ret = cfstore_drv->GetValueLen(ctx->hkey_prev, &ctx->len); 00803 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: GetValueLen() failed (ret=%ld)\r\n", __func__, ret); 00804 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00805 ctx->state = CFSTORE_EX_STATE_GET_VALUE_LEN_DONE; 00806 break; 00807 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00808 // await pending notification of completion. 00809 break; 00810 } 00811 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00812 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00813 * and hence is commented out. Re-instate if previous assert is removed. 00814 * break; */ 00815 00816 case CFSTORE_EX_STATE_GET_VALUE_LEN_DONE: 00817 CFSTORE_EX1_LOG("GET_VALUE_LEN_DONE%s", "\r\n"); 00818 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: GetValueLen() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00819 CFSTORE_EX1_TEST_ASSERT_MSG((int32_t) ctx->len == (int32_t) strlen(cfstore_ex_kv_value), "%s:Error: GetValueLen() updated value of len parameter (%ld) != strlen(cfstore_ex_kv_value)(%ld) \r\n", __func__, (int32_t) ctx->len, (int32_t) strlen(cfstore_ex_kv_value)); 00820 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == ctx->hkey_prev, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_GET_VALUE_LEN) received handle (%p) is not the hkey supplied to GetValueLen()(%p)\r\n", __func__, ctx->callback_handle, ctx->hkey_prev); 00821 ctx->state = CFSTORE_EX_STATE_DELETING; 00822 // intentional fall-through 00823 00824 case CFSTORE_EX_STATE_DELETING: 00825 CFSTORE_EX1_LOG("DELETING%s", "\r\n"); 00826 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_DELETE can be invoked before Delete() has returned 00827 ret = cfstore_drv->Delete(ctx->callback_handle); 00828 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Close() failed (ret=%ld)\r\n", __func__, ret); 00829 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00830 ctx->state = CFSTORE_EX_STATE_DELETE_DONE; 00831 break; 00832 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00833 // await pending notification of completion. 00834 break; 00835 } 00836 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00837 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00838 * and hence is commented out. Re-instate if previous assert is removed. 00839 * break; */ 00840 00841 case CFSTORE_EX_STATE_DELETE_DONE: 00842 CFSTORE_EX1_LOG("DELETE_DONE%s", "\r\n"); 00843 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Delete() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00844 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_DELETE) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00845 CFSTORE_HANDLE_SWAP(ctx->hkey_prev, ctx->hkey_next); 00846 ctx->state = CFSTORE_EX_STATE_FINDING2; 00847 // intentional fall-through 00848 00849 case CFSTORE_EX_STATE_FINDING2: 00850 CFSTORE_EX1_LOG("FINDING2%s", "\r\n"); 00851 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_FIND can be invoked before Find() has returned 00852 ret = cfstore_drv->Find("*", ctx->hkey_next, ctx->hkey_prev); 00853 CFSTORE_EX1_TEST_ASSERT_MSG(ret == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND, "%s:Error: Find() failed to return expected value of ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND (ret=%ld)\r\n", __func__, ret); 00854 if(ret == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND && ctx->caps.asynchronous_ops == false) { 00855 ctx->state = CFSTORE_EX_STATE_FIND_DONE2; 00856 break; 00857 } else if(ret == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND && ctx->caps.asynchronous_ops == true) { 00858 // await pending notification of completion. 00859 break; 00860 } 00861 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00862 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00863 * and hence is commented out. Re-instate if previous assert is removed. 00864 * break; */ 00865 00866 case CFSTORE_EX_STATE_FIND_DONE2: 00867 CFSTORE_EX1_LOG("FIND_DONE2%s", "\r\n"); 00868 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status == ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND, "%s:Error: Find() completion should have been ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND (status=%ld)\r\n", __func__, ctx->callback_status); 00869 ctx->state = CFSTORE_EX_STATE_FLUSHING2; 00870 // intentional fall-through 00871 00872 case CFSTORE_EX_STATE_FLUSHING2: 00873 CFSTORE_EX1_LOG("FLUSHING2%s", "\r\n"); 00874 // note that cfstore_ex_callback() for cmd_code==CFSTORE_OPCODE_FLUSH can be invoked before Flush() has returned 00875 ret = cfstore_drv->Flush(); 00876 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error:2: Flush() failed (ret=%ld)\r\n", __func__, ret); 00877 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00878 ctx->state = CFSTORE_EX_STATE_FLUSH_DONE2; 00879 break; 00880 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00881 // await pending notification of completion. 00882 break; 00883 } 00884 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00885 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00886 * and hence is commented out. Re-instate if previous assert is removed. 00887 * break; */ 00888 00889 case CFSTORE_EX_STATE_FLUSH_DONE2: 00890 CFSTORE_EX1_LOG("FLUSH_DONE2%s", "\r\n"); 00891 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_status >= ARM_DRIVER_OK, "%s:Error: Flush() completion failed (status=%ld)\r\n", __func__, ctx->callback_status); 00892 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_FLUSH) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00893 ctx->state = CFSTORE_EX_STATE_UNINITIALIZING; 00894 // intentional fall-through 00895 00896 case CFSTORE_EX_STATE_UNINITIALIZING: 00897 CFSTORE_EX1_LOG("UNINITIALIZING%s", "\r\n"); 00898 ret = cfstore_drv->Uninitialize(); 00899 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: Uninitialize() should return ret >= 0 for async/synch modes(ret=%ld)\r\n", __func__, ret); 00900 if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == false) { 00901 ctx->state = CFSTORE_EX_STATE_UNINIT_DONE; 00902 break; 00903 } else if(ret >= ARM_DRIVER_OK && ctx->caps.asynchronous_ops == true) { 00904 // await pending notification of completion. 00905 break; 00906 } 00907 CFSTORE_EX1_TEST_ASSERT_MSG(false, "%s:Error: unknown error (line=%u)\r\n", __func__, __LINE__); 00908 /* The following 'break' statement generates ARMCC #111-D: statement is unreachable warning 00909 * and hence is commented out. Re-instate if previous assert is removed. 00910 * break; */ 00911 00912 case CFSTORE_EX_STATE_UNINIT_DONE: 00913 CFSTORE_EX1_LOG("UNINIT_DONE%s", "\r\n"); 00914 CFSTORE_EX1_TEST_ASSERT_MSG(ctx->callback_handle == NULL, "%s:Error: the cfstore_ex_callback(cmd_code==CFSTORE_OPCODE_UNINITIALIZE) received non-NULL handle (%p)\r\n", __func__, ctx->callback_handle); 00915 cfstore_example1_done = true; 00916 CFSTORE_EX1_LOG("***************%s", "\r\n"); 00917 CFSTORE_EX1_LOG("*** SUCCESS ***%s", "\r\n"); 00918 CFSTORE_EX1_LOG("***************%s", "\r\n"); 00919 break; 00920 } 00921 } 00922 00923 static control_t cfstore_example1_app_start(const size_t call_count) 00924 { 00925 cfstore_example1_ctx_t* ctx = &cfstore_example1_ctx_g; 00926 00927 (void) call_count; 00928 00929 /* initialise the context */ 00930 memset(ctx, 0, sizeof(cfstore_example1_ctx_t)); 00931 cfstore_example1_done = false; 00932 ctx->hkey_next = ctx->hkey_next_buf; 00933 ctx->hkey_prev = ctx->hkey_prev_buf; 00934 ctx->callback_status = ARM_DRIVER_ERROR; 00935 ctx->state = CFSTORE_EX_STATE_INITIALIZING; 00936 ctx->caps = cfstore_drv->GetCapabilities(); 00937 cfstore_ex_fms_update(ctx); 00938 00939 /* main application worker loop */ 00940 while (!cfstore_example1_done) 00941 { 00942 // do some work 00943 CFSTORE_EX1_LOG("%s: going to sleep!\r\n", __func__); 00944 00945 #if defined CFSTORE_CONFIG_MBED_OS_VERSION && CFSTORE_CONFIG_MBED_OS_VERSION == 3 00946 __WFE(); 00947 #endif /* CFSTORE_CONFIG_MBED_OS_VERSION == 3 */ 00948 00949 #if defined CFSTORE_CONFIG_MBED_OS_VERSION && CFSTORE_CONFIG_MBED_OS_VERSION == 4 00950 /* mbedosV3++ 00951 * todo: port __WFE() 00952 */ 00953 #endif /* CFSTORE_CONFIG_MBED_OS_VERSION == 4 */ 00954 CFSTORE_EX1_LOG("%s: woke up!\r\n", __func__); 00955 } 00956 return CaseNext; 00957 } 00958 00959 #ifndef CFSTORE_EXAMPLE1_APP 00960 /* when built as Configuration-Store example, include greentea support otherwise omit */ 00961 00962 /* report whether built/configured for flash sync or async mode */ 00963 static control_t cfstore_example1_test_00(const size_t call_count) 00964 { 00965 int32_t ret = ARM_DRIVER_ERROR; 00966 (void) call_count; 00967 00968 ret = cfstore_test_startup(); 00969 CFSTORE_EX1_TEST_ASSERT_MSG(ret >= ARM_DRIVER_OK, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00970 return CaseNext; 00971 } 00972 00973 /// @cond CFSTORE_DOXYGEN_DISABLE 00974 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00975 { 00976 GREENTEA_SETUP(25, "default_auto"); 00977 return greentea_test_setup_handler(number_of_cases); 00978 } 00979 00980 Case cases[] = { 00981 /* 1 2 3 4 5 6 7 */ 00982 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00983 Case("EXAMPLE1_test_00", cfstore_example1_test_00), 00984 Case("EXAMPLE1_test_01_start", cfstore_example1_app_start), 00985 }; 00986 00987 00988 /* Declare your test specification with a custom setup handler */ 00989 Specification specification(greentea_setup, cases); 00990 00991 int main() 00992 { 00993 return !Harness::run(specification); 00994 } 00995 /// @endcond 00996 00997 00998 #else // CFSTORE_EXAMPLE1_APP 00999 01000 // stand alone Configuration-Store-Example 01001 void app_start(int argc __unused, char** argv __unused) 01002 { 01003 cfstore_example1_app_start(0); 01004 } 01005 01006 01007 01008 #endif // CFSTORE_EXAMPLE1_APP 01009 01010 01011 #endif // __MBED__ && ! defined TOOLCHAIN_GCC_ARM
Generated on Sun Jul 17 2022 08:25:22 by
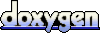