
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
eui64.h
00001 /* 00002 * eui64.h - EUI64 routines for IPv6CP. 00003 * 00004 * Copyright (c) 1999 Tommi Komulainen. All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * 2. Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in 00015 * the documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * 3. The name(s) of the authors of this software must not be used to 00019 * endorse or promote products derived from this software without 00020 * prior written permission. 00021 * 00022 * 4. Redistributions of any form whatsoever must retain the following 00023 * acknowledgment: 00024 * "This product includes software developed by Tommi Komulainen 00025 * <Tommi.Komulainen@iki.fi>". 00026 * 00027 * THE AUTHORS OF THIS SOFTWARE DISCLAIM ALL WARRANTIES WITH REGARD TO 00028 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00029 * AND FITNESS, IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY 00030 * SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00031 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00032 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00033 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00034 * 00035 * $Id: eui64.h,v 1.6 2002/12/04 23:03:32 paulus Exp $ 00036 */ 00037 00038 #include "netif/ppp/ppp_opts.h" 00039 #if PPP_SUPPORT && PPP_IPV6_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00040 00041 #ifndef EUI64_H 00042 #define EUI64_H 00043 00044 /* 00045 * @todo: 00046 * 00047 * Maybe this should be done by processing struct in6_addr directly... 00048 */ 00049 typedef union 00050 { 00051 u8_t e8[8]; 00052 u16_t e16[4]; 00053 u32_t e32[2]; 00054 } eui64_t; 00055 00056 #define eui64_iszero(e) (((e).e32[0] | (e).e32[1]) == 0) 00057 #define eui64_equals(e, o) (((e).e32[0] == (o).e32[0]) && \ 00058 ((e).e32[1] == (o).e32[1])) 00059 #define eui64_zero(e) (e).e32[0] = (e).e32[1] = 0; 00060 00061 #define eui64_copy(s, d) memcpy(&(d), &(s), sizeof(eui64_t)) 00062 00063 #define eui64_magic(e) do { \ 00064 (e).e32[0] = magic(); \ 00065 (e).e32[1] = magic(); \ 00066 (e).e8[0] &= ~2; \ 00067 } while (0) 00068 #define eui64_magic_nz(x) do { \ 00069 eui64_magic(x); \ 00070 } while (eui64_iszero(x)) 00071 #define eui64_magic_ne(x, y) do { \ 00072 eui64_magic(x); \ 00073 } while (eui64_equals(x, y)) 00074 00075 #define eui64_get(ll, cp) do { \ 00076 eui64_copy((*cp), (ll)); \ 00077 (cp) += sizeof(eui64_t); \ 00078 } while (0) 00079 00080 #define eui64_put(ll, cp) do { \ 00081 eui64_copy((ll), (*cp)); \ 00082 (cp) += sizeof(eui64_t); \ 00083 } while (0) 00084 00085 #define eui64_set32(e, l) do { \ 00086 (e).e32[0] = 0; \ 00087 (e).e32[1] = lwip_htonl(l); \ 00088 } while (0) 00089 #define eui64_setlo32(e, l) eui64_set32(e, l) 00090 00091 char *eui64_ntoa(eui64_t); /* Returns ascii representation of id */ 00092 00093 #endif /* EUI64_H */ 00094 #endif /* PPP_SUPPORT && PPP_IPV6_SUPPORT */
Generated on Sun Jul 17 2022 08:25:22 by
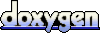