
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ethernet_tasklet.c
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <string.h> //memset 00018 #include "eventOS_event_timer.h" 00019 #include "common_functions.h" 00020 #include "net_interface.h" 00021 #include "ip6string.h" //ip6tos 00022 #include "nsdynmemLIB.h" 00023 #include "include/mesh_system.h" 00024 #include "ns_event_loop.h" 00025 #include "mesh_interface_types.h" 00026 #include "eventOS_event.h" 00027 00028 // For tracing we need to define flag, have include and define group 00029 #include "ns_trace.h" 00030 #define TRACE_GROUP "IPV6" 00031 00032 #include "ethernet_mac_api.h" 00033 00034 #define INTERFACE_NAME "eth0" 00035 00036 // Tasklet timer events 00037 #define TIMER_EVENT_START_BOOTSTRAP 1 00038 00039 #define INVALID_INTERFACE_ID (-1) 00040 00041 #define STR_HELPER(x) #x 00042 #define STR(x) STR_HELPER(x) 00043 00044 /* 00045 * Mesh tasklet states. 00046 */ 00047 typedef enum { 00048 TASKLET_STATE_CREATED = 0, 00049 TASKLET_STATE_INITIALIZED, 00050 TASKLET_STATE_BOOTSTRAP_STARTED, 00051 TASKLET_STATE_BOOTSTRAP_FAILED, 00052 TASKLET_STATE_BOOTSTRAP_READY 00053 } tasklet_state_t; 00054 00055 /* 00056 * Mesh tasklet data structure. 00057 */ 00058 typedef struct { 00059 void (*mesh_api_cb)(mesh_connection_status_t nwk_status); 00060 tasklet_state_t tasklet_state; 00061 int8_t node_main_tasklet_id; 00062 int8_t network_interface_id; 00063 int8_t tasklet; 00064 } tasklet_data_str_t; 00065 00066 /* Tasklet data */ 00067 static tasklet_data_str_t *tasklet_data_ptr = NULL; 00068 static eth_mac_api_t *eth_mac_api = NULL; 00069 typedef void (*mesh_interface_cb)(mesh_connection_status_t mesh_status); 00070 00071 00072 /* private function prototypes */ 00073 static void enet_tasklet_main(arm_event_s *event); 00074 static void enet_tasklet_network_state_changed(mesh_connection_status_t status); 00075 static void enet_tasklet_parse_network_event(arm_event_s *event); 00076 static void enet_tasklet_configure_and_connect_to_network(void); 00077 00078 /* 00079 * \brief A function which will be eventually called by NanoStack OS when ever the OS has an event to deliver. 00080 * @param event, describes the sender, receiver and event type. 00081 * 00082 * NOTE: Interrupts requested by HW are possible during this function! 00083 */ 00084 void enet_tasklet_main(arm_event_s *event) 00085 { 00086 arm_library_event_type_e event_type; 00087 event_type = (arm_library_event_type_e) event->event_type; 00088 00089 switch (event_type) { 00090 case ARM_LIB_NWK_INTERFACE_EVENT: 00091 /* This event is delivered every and each time when there is new 00092 * information of network connectivity. 00093 */ 00094 enet_tasklet_parse_network_event(event); 00095 break; 00096 00097 case ARM_LIB_TASKLET_INIT_EVENT: 00098 /* Event with type EV_INIT is an initializer event of NanoStack OS. 00099 * The event is delivered when the NanoStack OS is running fine. 00100 * This event should be delivered ONLY ONCE. 00101 */ 00102 tasklet_data_ptr->node_main_tasklet_id = event->receiver; 00103 mesh_system_send_connect_event(tasklet_data_ptr->tasklet); 00104 break; 00105 00106 case ARM_LIB_SYSTEM_TIMER_EVENT: 00107 eventOS_event_timer_cancel(event->event_id, 00108 tasklet_data_ptr->node_main_tasklet_id); 00109 00110 if (event->event_id == TIMER_EVENT_START_BOOTSTRAP) { 00111 tr_debug("Restart bootstrap"); 00112 enet_tasklet_configure_and_connect_to_network(); 00113 } 00114 break; 00115 00116 case APPLICATION_EVENT: 00117 if (event->event_id == APPL_EVENT_CONNECT) { 00118 enet_tasklet_configure_and_connect_to_network(); 00119 } 00120 break; 00121 00122 default: 00123 break; 00124 } // switch(event_type) 00125 } 00126 00127 /** 00128 * \brief Network state event handler. 00129 * \param event show network start response or current network state. 00130 * 00131 * - ARM_NWK_BOOTSTRAP_READY: Save NVK persistent data to NVM and Net role 00132 * - ARM_NWK_NWK_SCAN_FAIL: Link Layer Active Scan Fail, Stack is Already at Idle state 00133 * - ARM_NWK_IP_ADDRESS_ALLOCATION_FAIL: No ND Router at current Channel Stack is Already at Idle state 00134 * - ARM_NWK_NWK_CONNECTION_DOWN: Connection to Access point is lost wait for Scan Result 00135 * - ARM_NWK_NWK_PARENT_POLL_FAIL: Host should run net start without any PAN-id filter and all channels 00136 * - ARM_NWK_AUHTENTICATION_FAIL: Pana Authentication fail, Stack is Already at Idle state 00137 */ 00138 void enet_tasklet_parse_network_event(arm_event_s *event) 00139 { 00140 arm_nwk_interface_status_type_e status = (arm_nwk_interface_status_type_e) event->event_data; 00141 tr_debug("app_parse_network_event() %d", status); 00142 switch (status) { 00143 case ARM_NWK_BOOTSTRAP_READY: 00144 /* Network is ready and node is connected to Access Point */ 00145 if (tasklet_data_ptr->tasklet_state != TASKLET_STATE_BOOTSTRAP_READY) { 00146 tr_info("IPv6 bootstrap ready"); 00147 tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_READY; 00148 enet_tasklet_network_state_changed(MESH_CONNECTED); 00149 } 00150 break; 00151 case ARM_NWK_IP_ADDRESS_ALLOCATION_FAIL: 00152 /* No ND Router at current Channel Stack is Already at Idle state */ 00153 tr_info("Bootstrap fail"); 00154 tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00155 break; 00156 case ARM_NWK_NWK_CONNECTION_DOWN: 00157 /* Connection to Access point is lost wait for Scan Result */ 00158 tr_info("Connection lost"); 00159 tasklet_data_ptr->tasklet_state = TASKLET_STATE_BOOTSTRAP_FAILED; 00160 break; 00161 default: 00162 tr_warn("Unknown event %d", status); 00163 break; 00164 } 00165 00166 if (tasklet_data_ptr->tasklet_state != TASKLET_STATE_BOOTSTRAP_READY) { 00167 // Set 5s timer for new network scan 00168 eventOS_event_timer_request(TIMER_EVENT_START_BOOTSTRAP, 00169 ARM_LIB_SYSTEM_TIMER_EVENT, 00170 tasklet_data_ptr->node_main_tasklet_id, 00171 5000); 00172 } 00173 } 00174 00175 /* 00176 * \brief Configure and establish network connection 00177 * 00178 */ 00179 void enet_tasklet_configure_and_connect_to_network(void) 00180 { 00181 arm_nwk_interface_up(tasklet_data_ptr->network_interface_id); 00182 } 00183 00184 /* 00185 * Inform application about network state change 00186 */ 00187 void enet_tasklet_network_state_changed(mesh_connection_status_t status) 00188 { 00189 if (tasklet_data_ptr->mesh_api_cb) { 00190 (tasklet_data_ptr->mesh_api_cb)(status); 00191 } 00192 } 00193 00194 /* Public functions */ 00195 int8_t enet_tasklet_get_ip_address(char *address, int8_t len) 00196 { 00197 uint8_t binary_ipv6[16]; 00198 00199 if ((len >= 40) && (0 == arm_net_address_get( 00200 tasklet_data_ptr->network_interface_id, ADDR_IPV6_GP, binary_ipv6))) { 00201 ip6tos(binary_ipv6, address); 00202 //tr_debug("IP address: %s", address); 00203 return 0; 00204 } else { 00205 return -1; 00206 } 00207 } 00208 00209 int8_t enet_tasklet_connect(mesh_interface_cb callback, int8_t nwk_interface_id) 00210 { 00211 int8_t re_connecting = true; 00212 int8_t tasklet_id = tasklet_data_ptr->tasklet; 00213 00214 if (tasklet_data_ptr->tasklet_state == TASKLET_STATE_CREATED) { 00215 re_connecting = false; 00216 } 00217 00218 memset(tasklet_data_ptr, 0, sizeof(tasklet_data_str_t)); 00219 tasklet_data_ptr->mesh_api_cb = callback; 00220 tasklet_data_ptr->network_interface_id = nwk_interface_id; 00221 tasklet_data_ptr->tasklet_state = TASKLET_STATE_INITIALIZED; 00222 00223 if (re_connecting == false) { 00224 tasklet_data_ptr->tasklet = eventOS_event_handler_create(&enet_tasklet_main, 00225 ARM_LIB_TASKLET_INIT_EVENT); 00226 if (tasklet_data_ptr->tasklet < 0) { 00227 // -1 handler already used by other tasklet 00228 // -2 memory allocation failure 00229 return tasklet_data_ptr->tasklet; 00230 } 00231 } else { 00232 tasklet_data_ptr->tasklet = tasklet_id; 00233 mesh_system_send_connect_event(tasklet_data_ptr->tasklet); 00234 } 00235 00236 return 0; 00237 } 00238 00239 int8_t enet_tasklet_disconnect(bool send_cb) 00240 { 00241 int8_t status = -1; 00242 if (tasklet_data_ptr != NULL) { 00243 if (tasklet_data_ptr->network_interface_id != INVALID_INTERFACE_ID) { 00244 status = arm_nwk_interface_down(tasklet_data_ptr->network_interface_id); 00245 tasklet_data_ptr->network_interface_id = INVALID_INTERFACE_ID; 00246 if (send_cb == true) { 00247 enet_tasklet_network_state_changed(MESH_DISCONNECTED); 00248 } 00249 } 00250 tasklet_data_ptr->mesh_api_cb = NULL; 00251 } 00252 return status; 00253 } 00254 00255 void enet_tasklet_init(void) 00256 { 00257 if (tasklet_data_ptr == NULL) { 00258 tasklet_data_ptr = ns_dyn_mem_alloc(sizeof(tasklet_data_str_t)); 00259 tasklet_data_ptr->tasklet_state = TASKLET_STATE_CREATED; 00260 tasklet_data_ptr->network_interface_id = INVALID_INTERFACE_ID; 00261 } 00262 } 00263 00264 int8_t enet_tasklet_network_init(int8_t device_id) 00265 { 00266 if (tasklet_data_ptr->network_interface_id != -1) { 00267 tr_debug("Interface already at active state\n"); 00268 return tasklet_data_ptr->network_interface_id; 00269 } 00270 if (!eth_mac_api) { 00271 eth_mac_api = ethernet_mac_create(device_id); 00272 } 00273 00274 tasklet_data_ptr->network_interface_id = arm_nwk_interface_ethernet_init(eth_mac_api, "eth0"); 00275 00276 tr_debug("interface ID: %d", tasklet_data_ptr->network_interface_id); 00277 arm_nwk_interface_configure_ipv6_bootstrap_set( 00278 tasklet_data_ptr->network_interface_id, NET_IPV6_BOOTSTRAP_AUTONOMOUS, NULL); 00279 return tasklet_data_ptr->network_interface_id; 00280 }
Generated on Sun Jul 17 2022 08:25:22 by
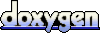