
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
equeue_platform.h
00001 00002 /** \addtogroup events */ 00003 /** @{*/ 00004 /* 00005 * System specific implementation 00006 * 00007 * Copyright (c) 2016 Christopher Haster 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 #ifndef EQUEUE_PLATFORM_H 00022 #define EQUEUE_PLATFORM_H 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 #include <stdbool.h> 00029 00030 // Currently supported platforms 00031 // 00032 // Uncomment to select a supported platform or reimplement this file 00033 // for a specific target. 00034 //#define EQUEUE_PLATFORM_POSIX 00035 //#define EQUEUE_PLATFORM_MBED 00036 00037 // Try to infer a platform if none was manually selected 00038 #if !defined(EQUEUE_PLATFORM_POSIX) \ 00039 && !defined(EQUEUE_PLATFORM_MBED) 00040 #if defined(__unix__) 00041 #define EQUEUE_PLATFORM_POSIX 00042 #elif defined(__MBED__) 00043 #define EQUEUE_PLATFORM_MBED 00044 #else 00045 #warning "Unknown platform! Please update equeue_platform.h" 00046 #endif 00047 #endif 00048 00049 // Platform includes 00050 #if defined(EQUEUE_PLATFORM_POSIX) 00051 #include <pthread.h> 00052 #elif defined(EQUEUE_PLATFORM_MBED) 00053 #include "cmsis_os2.h" 00054 #include "mbed_rtos_storage.h" 00055 #endif 00056 00057 00058 // Platform millisecond counter 00059 // 00060 // Return a tick that represents the number of milliseconds that have passed 00061 // since an arbitrary point in time. The granularity does not need to be at 00062 // the millisecond level, however the accuracy of the equeue library is 00063 // limited by the accuracy of this tick. 00064 // 00065 // Must intentionally overflow to 0 after 2^32-1 00066 unsigned equeue_tick(void); 00067 00068 00069 // Platform mutex type 00070 // 00071 // The equeue library requires at minimum a non-recursive mutex that is 00072 // safe in interrupt contexts. The mutex section is help for a bounded 00073 // amount of time, so simply disabling interrupts is acceptable 00074 // 00075 // If irq safety is not required, a regular blocking mutex can be used. 00076 #if defined(EQUEUE_PLATFORM_POSIX) 00077 typedef pthread_mutex_t equeue_mutex_t; 00078 #elif defined(EQUEUE_PLATFORM_WINDOWS) 00079 typedef CRITICAL_SECTION equeue_mutex_t; 00080 #elif defined(EQUEUE_PLATFORM_MBED) 00081 typedef unsigned equeue_mutex_t; 00082 #elif defined(EQUEUE_PLATFORM_FREERTOS) 00083 typedef UBaseType_t equeue_mutex_t; 00084 #endif 00085 00086 // Platform mutex operations 00087 // 00088 // The equeue_mutex_create and equeue_mutex_destroy manage the lifetime 00089 // of the mutex. On error, equeue_mutex_create should return a negative 00090 // error code. 00091 // 00092 // The equeue_mutex_lock and equeue_mutex_unlock lock and unlock the 00093 // underlying mutex. 00094 int equeue_mutex_create(equeue_mutex_t *mutex); 00095 void equeue_mutex_destroy(equeue_mutex_t *mutex); 00096 void equeue_mutex_lock(equeue_mutex_t *mutex); 00097 void equeue_mutex_unlock(equeue_mutex_t *mutex); 00098 00099 00100 // Platform semaphore type 00101 // 00102 // The equeue library requires a binary semaphore type that can be safely 00103 // signaled from interrupt contexts and from inside a equeue_mutex section. 00104 // 00105 // The equeue_signal_wait is relied upon by the equeue library to sleep the 00106 // processor between events. Spurious wakeups have no negative-effects. 00107 // 00108 // A counting semaphore will also work, however may cause the event queue 00109 // dispatch loop to run unnecessarily. For that matter, equeue_signal_wait 00110 // may even be implemented as a single return statement. 00111 #if defined(EQUEUE_PLATFORM_POSIX) 00112 typedef struct equeue_sema { 00113 pthread_mutex_t mutex; 00114 pthread_cond_t cond; 00115 bool signal; 00116 } equeue_sema_t; 00117 #elif defined(EQUEUE_PLATFORM_MBED) && defined(MBED_CONF_RTOS_PRESENT) 00118 typedef struct equeue_sema { 00119 osEventFlagsId_t id; 00120 mbed_rtos_storage_event_flags_t mem; 00121 } equeue_sema_t; 00122 #elif defined(EQUEUE_PLATFORM_MBED) 00123 typedef volatile int equeue_sema_t; 00124 #endif 00125 00126 // Platform semaphore operations 00127 // 00128 // The equeue_sema_create and equeue_sema_destroy manage the lifetime 00129 // of the semaphore. On error, equeue_sema_create should return a negative 00130 // error code. 00131 // 00132 // The equeue_sema_signal marks a semaphore as signalled such that the next 00133 // equeue_sema_wait will return true. 00134 // 00135 // The equeue_sema_wait waits for a semaphore to be signalled or returns 00136 // immediately if equeue_sema_signal had been called since the last 00137 // equeue_sema_wait. The equeue_sema_wait returns true if it detected that 00138 // equeue_sema_signal had been called. If ms is negative, equeue_sema_wait 00139 // will wait for a signal indefinitely. 00140 int equeue_sema_create(equeue_sema_t *sema); 00141 void equeue_sema_destroy(equeue_sema_t *sema); 00142 void equeue_sema_signal(equeue_sema_t *sema); 00143 bool equeue_sema_wait(equeue_sema_t *sema, int ms); 00144 00145 00146 #ifdef __cplusplus 00147 } 00148 #endif 00149 00150 #endif 00151 00152 /** @}*/
Generated on Sun Jul 17 2022 08:25:22 by
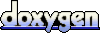