
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
emac_stack_lwip.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #if DEVICE_EMAC 00018 00019 #include "emac_stack_mem.h" 00020 #include "pbuf.h" 00021 00022 emac_stack_mem_t *emac_stack_mem_alloc(emac_stack_t* stack, uint32_t size, uint32_t align) 00023 { 00024 00025 struct pbuf *pbuf = pbuf_alloc(PBUF_RAW, size + align, PBUF_RAM); 00026 if (pbuf == NULL) { 00027 return NULL; 00028 } 00029 00030 if (align) { 00031 uint32_t remainder = (uint32_t)pbuf->payload % align; 00032 uint32_t offset = align - remainder; 00033 if (offset >= align) { 00034 offset = align; 00035 } 00036 00037 pbuf->payload = (void*)((char*)pbuf->payload + offset); 00038 pbuf->tot_len -= offset; 00039 pbuf->len -= offset; 00040 } 00041 00042 return (emac_stack_mem_t*)pbuf; 00043 } 00044 00045 void emac_stack_mem_free(emac_stack_t* stack, emac_stack_mem_t *mem) 00046 { 00047 pbuf_free((struct pbuf*)mem); 00048 } 00049 00050 void emac_stack_mem_copy(emac_stack_t* stack, emac_stack_mem_t *to, emac_stack_mem_t *from) 00051 { 00052 pbuf_copy((struct pbuf*)to, (struct pbuf*)from); 00053 } 00054 00055 void *emac_stack_mem_ptr(emac_stack_t* stack, emac_stack_mem_t *mem) 00056 { 00057 return ((struct pbuf*)mem)->payload; 00058 } 00059 00060 uint32_t emac_stack_mem_len(emac_stack_t* stack, emac_stack_mem_t *mem) 00061 { 00062 return ((struct pbuf*)mem)->len; 00063 } 00064 00065 void emac_stack_mem_set_len(emac_stack_t* stack, emac_stack_mem_t *mem, uint32_t len) 00066 { 00067 struct pbuf *pbuf = (struct pbuf*)mem; 00068 00069 pbuf->len = len; 00070 } 00071 00072 emac_stack_mem_t *emac_stack_mem_chain_dequeue(emac_stack_t* stack, emac_stack_mem_chain_t **chain) 00073 { 00074 struct pbuf **list = (struct pbuf**)chain; 00075 struct pbuf *head = *list; 00076 *list = (*list)->next; 00077 00078 return (emac_stack_mem_t *)head; 00079 } 00080 00081 uint32_t emac_stack_mem_chain_len(emac_stack_t* stack, emac_stack_mem_chain_t *chain) 00082 { 00083 return ((struct pbuf*)chain)->tot_len; 00084 } 00085 00086 void emac_stack_mem_ref(emac_stack_t* stack, emac_stack_mem_t *mem) 00087 { 00088 pbuf_ref((struct pbuf*)mem); 00089 } 00090 00091 #endif /* DEVICE_EMAC */
Generated on Sun Jul 17 2022 08:25:22 by
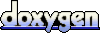