
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
eap.h
00001 /* 00002 * eap.h - Extensible Authentication Protocol for PPP (RFC 2284) 00003 * 00004 * Copyright (c) 2001 by Sun Microsystems, Inc. 00005 * All rights reserved. 00006 * 00007 * Non-exclusive rights to redistribute, modify, translate, and use 00008 * this software in source and binary forms, in whole or in part, is 00009 * hereby granted, provided that the above copyright notice is 00010 * duplicated in any source form, and that neither the name of the 00011 * copyright holder nor the author is used to endorse or promote 00012 * products derived from this software. 00013 * 00014 * THIS SOFTWARE IS PROVIDED ``AS IS'' AND WITHOUT ANY EXPRESS OR 00015 * IMPLIED WARRANTIES, INCLUDING, WITHOUT LIMITATION, THE IMPLIED 00016 * WARRANTIES OF MERCHANTIBILITY AND FITNESS FOR A PARTICULAR PURPOSE. 00017 * 00018 * Original version by James Carlson 00019 * 00020 * $Id: eap.h,v 1.2 2003/06/11 23:56:26 paulus Exp $ 00021 */ 00022 00023 #include "netif/ppp/ppp_opts.h" 00024 #if PPP_SUPPORT && EAP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00025 00026 #ifndef PPP_EAP_H 00027 #define PPP_EAP_H 00028 00029 #include "ppp.h" 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 /* 00036 * Packet header = Code, id, length. 00037 */ 00038 #define EAP_HEADERLEN 4 00039 00040 00041 /* EAP message codes. */ 00042 #define EAP_REQUEST 1 00043 #define EAP_RESPONSE 2 00044 #define EAP_SUCCESS 3 00045 #define EAP_FAILURE 4 00046 00047 /* EAP types */ 00048 #define EAPT_IDENTITY 1 00049 #define EAPT_NOTIFICATION 2 00050 #define EAPT_NAK 3 /* (response only) */ 00051 #define EAPT_MD5CHAP 4 00052 #define EAPT_OTP 5 /* One-Time Password; RFC 1938 */ 00053 #define EAPT_TOKEN 6 /* Generic Token Card */ 00054 /* 7 and 8 are unassigned. */ 00055 #define EAPT_RSA 9 /* RSA Public Key Authentication */ 00056 #define EAPT_DSS 10 /* DSS Unilateral */ 00057 #define EAPT_KEA 11 /* KEA */ 00058 #define EAPT_KEA_VALIDATE 12 /* KEA-VALIDATE */ 00059 #define EAPT_TLS 13 /* EAP-TLS */ 00060 #define EAPT_DEFENDER 14 /* Defender Token (AXENT) */ 00061 #define EAPT_W2K 15 /* Windows 2000 EAP */ 00062 #define EAPT_ARCOT 16 /* Arcot Systems */ 00063 #define EAPT_CISCOWIRELESS 17 /* Cisco Wireless */ 00064 #define EAPT_NOKIACARD 18 /* Nokia IP smart card */ 00065 #define EAPT_SRP 19 /* Secure Remote Password */ 00066 /* 20 is deprecated */ 00067 00068 /* EAP SRP-SHA1 Subtypes */ 00069 #define EAPSRP_CHALLENGE 1 /* Request 1 - Challenge */ 00070 #define EAPSRP_CKEY 1 /* Response 1 - Client Key */ 00071 #define EAPSRP_SKEY 2 /* Request 2 - Server Key */ 00072 #define EAPSRP_CVALIDATOR 2 /* Response 2 - Client Validator */ 00073 #define EAPSRP_SVALIDATOR 3 /* Request 3 - Server Validator */ 00074 #define EAPSRP_ACK 3 /* Response 3 - final ack */ 00075 #define EAPSRP_LWRECHALLENGE 4 /* Req/resp 4 - Lightweight rechal */ 00076 00077 #define SRPVAL_EBIT 0x00000001 /* Use shared key for ECP */ 00078 00079 #define SRP_PSEUDO_ID "pseudo_" 00080 #define SRP_PSEUDO_LEN 7 00081 00082 #define MD5_SIGNATURE_SIZE 16 00083 #define EAP_MIN_CHALLENGE_LENGTH 17 00084 #define EAP_MAX_CHALLENGE_LENGTH 24 00085 #define EAP_MIN_MAX_POWER_OF_TWO_CHALLENGE_LENGTH 3 /* 2^3-1 = 7, 17+7 = 24 */ 00086 00087 #define EAP_STATES \ 00088 "Initial", "Pending", "Closed", "Listen", "Identify", \ 00089 "SRP1", "SRP2", "SRP3", "MD5Chall", "Open", "SRP4", "BadAuth" 00090 00091 #define eap_client_active(pcb) ((pcb)->eap.es_client.ea_state == eapListen) 00092 #if PPP_SERVER 00093 #define eap_server_active(pcb) \ 00094 ((pcb)->eap.es_server.ea_state >= eapIdentify && \ 00095 (pcb)->eap.es_server.ea_state <= eapMD5Chall) 00096 #endif /* PPP_SERVER */ 00097 00098 /* 00099 * Complete EAP state for one PPP session. 00100 */ 00101 enum eap_state_code { 00102 eapInitial = 0, /* No EAP authentication yet requested */ 00103 eapPending, /* Waiting for LCP (no timer) */ 00104 eapClosed, /* Authentication not in use */ 00105 eapListen, /* Client ready (and timer running) */ 00106 eapIdentify, /* EAP Identify sent */ 00107 eapSRP1, /* Sent EAP SRP-SHA1 Subtype 1 */ 00108 eapSRP2, /* Sent EAP SRP-SHA1 Subtype 2 */ 00109 eapSRP3, /* Sent EAP SRP-SHA1 Subtype 3 */ 00110 eapMD5Chall, /* Sent MD5-Challenge */ 00111 eapOpen, /* Completed authentication */ 00112 eapSRP4, /* Sent EAP SRP-SHA1 Subtype 4 */ 00113 eapBadAuth /* Failed authentication */ 00114 }; 00115 00116 struct eap_auth { 00117 const char *ea_name; /* Our name */ 00118 char ea_peer[MAXNAMELEN +1]; /* Peer's name */ 00119 void *ea_session; /* Authentication library linkage */ 00120 u_char *ea_skey; /* Shared encryption key */ 00121 u_short ea_namelen; /* Length of our name */ 00122 u_short ea_peerlen; /* Length of peer's name */ 00123 enum eap_state_code ea_state; 00124 u_char ea_id; /* Current id */ 00125 u_char ea_requests; /* Number of Requests sent/received */ 00126 u_char ea_responses; /* Number of Responses */ 00127 u_char ea_type; /* One of EAPT_* */ 00128 u32_t ea_keyflags; /* SRP shared key usage flags */ 00129 }; 00130 00131 #ifndef EAP_MAX_CHALLENGE_LENGTH 00132 #define EAP_MAX_CHALLENGE_LENGTH 24 00133 #endif 00134 typedef struct eap_state { 00135 struct eap_auth es_client; /* Client (authenticatee) data */ 00136 #if PPP_SERVER 00137 struct eap_auth es_server; /* Server (authenticator) data */ 00138 #endif /* PPP_SERVER */ 00139 int es_savedtime; /* Saved timeout */ 00140 int es_rechallenge; /* EAP rechallenge interval */ 00141 int es_lwrechallenge; /* SRP lightweight rechallenge inter */ 00142 u8_t es_usepseudo; /* Use SRP Pseudonym if offered one */ 00143 int es_usedpseudo; /* Set if we already sent PN */ 00144 int es_challen; /* Length of challenge string */ 00145 u_char es_challenge[EAP_MAX_CHALLENGE_LENGTH]; 00146 } eap_state; 00147 00148 /* 00149 * Timeouts. 00150 */ 00151 #if 0 /* moved to ppp_opts.h */ 00152 #define EAP_DEFTIMEOUT 3 /* Timeout (seconds) for rexmit */ 00153 #define EAP_DEFTRANSMITS 10 /* max # times to transmit */ 00154 #define EAP_DEFREQTIME 20 /* Time to wait for peer request */ 00155 #define EAP_DEFALLOWREQ 20 /* max # times to accept requests */ 00156 #endif /* moved to ppp_opts.h */ 00157 00158 void eap_authwithpeer(ppp_pcb *pcb, const char *localname); 00159 void eap_authpeer(ppp_pcb *pcb, const char *localname); 00160 00161 extern const struct protent eap_protent; 00162 00163 #ifdef __cplusplus 00164 } 00165 #endif 00166 00167 #endif /* PPP_EAP_H */ 00168 00169 #endif /* PPP_SUPPORT && EAP_SUPPORT */
Generated on Sun Jul 17 2022 08:25:22 by
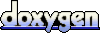