
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
dhm.c
00001 /* 00002 * Diffie-Hellman-Merkle key exchange 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * The following sources were referenced in the design of this implementation 00023 * of the Diffie-Hellman-Merkle algorithm: 00024 * 00025 * [1] Handbook of Applied Cryptography - 1997, Chapter 12 00026 * Menezes, van Oorschot and Vanstone 00027 * 00028 */ 00029 00030 #if !defined(MBEDTLS_CONFIG_FILE) 00031 #include "mbedtls/config.h" 00032 #else 00033 #include MBEDTLS_CONFIG_FILE 00034 #endif 00035 00036 #if defined(MBEDTLS_DHM_C) 00037 00038 #include "mbedtls/dhm.h" 00039 00040 #include <string.h> 00041 00042 #if defined(MBEDTLS_PEM_PARSE_C) 00043 #include "mbedtls/pem.h" 00044 #endif 00045 00046 #if defined(MBEDTLS_ASN1_PARSE_C) 00047 #include "mbedtls/asn1.h" 00048 #endif 00049 00050 #if defined(MBEDTLS_PLATFORM_C) 00051 #include "mbedtls/platform.h" 00052 #else 00053 #include <stdlib.h> 00054 #include <stdio.h> 00055 #define mbedtls_printf printf 00056 #define mbedtls_calloc calloc 00057 #define mbedtls_free free 00058 #endif 00059 00060 /* Implementation that should never be optimized out by the compiler */ 00061 static void mbedtls_zeroize( void *v, size_t n ) { 00062 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00063 } 00064 00065 /* 00066 * helper to validate the mbedtls_mpi size and import it 00067 */ 00068 static int dhm_read_bignum( mbedtls_mpi *X, 00069 unsigned char **p, 00070 const unsigned char *end ) 00071 { 00072 int ret, n; 00073 00074 if( end - *p < 2 ) 00075 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00076 00077 n = ( (*p)[0] << 8 ) | (*p)[1]; 00078 (*p) += 2; 00079 00080 if( (int)( end - *p ) < n ) 00081 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00082 00083 if( ( ret = mbedtls_mpi_read_binary( X, *p, n ) ) != 0 ) 00084 return( MBEDTLS_ERR_DHM_READ_PARAMS_FAILED + ret ); 00085 00086 (*p) += n; 00087 00088 return( 0 ); 00089 } 00090 00091 /* 00092 * Verify sanity of parameter with regards to P 00093 * 00094 * Parameter should be: 2 <= public_param <= P - 2 00095 * 00096 * For more information on the attack, see: 00097 * http://www.cl.cam.ac.uk/~rja14/Papers/psandqs.pdf 00098 * http://web.nvd.nist.gov/view/vuln/detail?vulnId=CVE-2005-2643 00099 */ 00100 static int dhm_check_range( const mbedtls_mpi *param, const mbedtls_mpi *P ) 00101 { 00102 mbedtls_mpi L, U; 00103 int ret = MBEDTLS_ERR_DHM_BAD_INPUT_DATA; 00104 00105 mbedtls_mpi_init( &L ); mbedtls_mpi_init( &U ); 00106 00107 MBEDTLS_MPI_CHK( mbedtls_mpi_lset( &L, 2 ) ); 00108 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &U, P, 2 ) ); 00109 00110 if( mbedtls_mpi_cmp_mpi( param, &L ) >= 0 && 00111 mbedtls_mpi_cmp_mpi( param, &U ) <= 0 ) 00112 { 00113 ret = 0; 00114 } 00115 00116 cleanup: 00117 mbedtls_mpi_free( &L ); mbedtls_mpi_free( &U ); 00118 return( ret ); 00119 } 00120 00121 void mbedtls_dhm_init( mbedtls_dhm_context *ctx ) 00122 { 00123 memset( ctx, 0, sizeof( mbedtls_dhm_context ) ); 00124 } 00125 00126 /* 00127 * Parse the ServerKeyExchange parameters 00128 */ 00129 int mbedtls_dhm_read_params( mbedtls_dhm_context *ctx, 00130 unsigned char **p, 00131 const unsigned char *end ) 00132 { 00133 int ret; 00134 00135 if( ( ret = dhm_read_bignum( &ctx->P , p, end ) ) != 0 || 00136 ( ret = dhm_read_bignum( &ctx->G , p, end ) ) != 0 || 00137 ( ret = dhm_read_bignum( &ctx->GY , p, end ) ) != 0 ) 00138 return( ret ); 00139 00140 if( ( ret = dhm_check_range( &ctx->GY , &ctx->P ) ) != 0 ) 00141 return( ret ); 00142 00143 ctx->len = mbedtls_mpi_size( &ctx->P ); 00144 00145 return( 0 ); 00146 } 00147 00148 /* 00149 * Setup and write the ServerKeyExchange parameters 00150 */ 00151 int mbedtls_dhm_make_params( mbedtls_dhm_context *ctx, int x_size, 00152 unsigned char *output, size_t *olen, 00153 int (*f_rng)(void *, unsigned char *, size_t), 00154 void *p_rng ) 00155 { 00156 int ret, count = 0; 00157 size_t n1, n2, n3; 00158 unsigned char *p; 00159 00160 if( mbedtls_mpi_cmp_int( &ctx->P , 0 ) == 0 ) 00161 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00162 00163 /* 00164 * Generate X as large as possible ( < P ) 00165 */ 00166 do 00167 { 00168 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &ctx->X , x_size, f_rng, p_rng ) ); 00169 00170 while( mbedtls_mpi_cmp_mpi( &ctx->X , &ctx->P ) >= 0 ) 00171 MBEDTLS_MPI_CHK( mbedtls_mpi_shift_r( &ctx->X , 1 ) ); 00172 00173 if( count++ > 10 ) 00174 return( MBEDTLS_ERR_DHM_MAKE_PARAMS_FAILED ); 00175 } 00176 while( dhm_check_range( &ctx->X , &ctx->P ) != 0 ); 00177 00178 /* 00179 * Calculate GX = G^X mod P 00180 */ 00181 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &ctx->GX , &ctx->G , &ctx->X , 00182 &ctx->P , &ctx->RP ) ); 00183 00184 if( ( ret = dhm_check_range( &ctx->GX , &ctx->P ) ) != 0 ) 00185 return( ret ); 00186 00187 /* 00188 * export P, G, GX 00189 */ 00190 #define DHM_MPI_EXPORT(X,n) \ 00191 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( X, p + 2, n ) ); \ 00192 *p++ = (unsigned char)( n >> 8 ); \ 00193 *p++ = (unsigned char)( n ); p += n; 00194 00195 n1 = mbedtls_mpi_size( &ctx->P ); 00196 n2 = mbedtls_mpi_size( &ctx->G ); 00197 n3 = mbedtls_mpi_size( &ctx->GX ); 00198 00199 p = output; 00200 DHM_MPI_EXPORT( &ctx->P , n1 ); 00201 DHM_MPI_EXPORT( &ctx->G , n2 ); 00202 DHM_MPI_EXPORT( &ctx->GX , n3 ); 00203 00204 *olen = p - output; 00205 00206 ctx->len = n1; 00207 00208 cleanup: 00209 00210 if( ret != 0 ) 00211 return( MBEDTLS_ERR_DHM_MAKE_PARAMS_FAILED + ret ); 00212 00213 return( 0 ); 00214 } 00215 00216 /* 00217 * Import the peer's public value G^Y 00218 */ 00219 int mbedtls_dhm_read_public( mbedtls_dhm_context *ctx, 00220 const unsigned char *input, size_t ilen ) 00221 { 00222 int ret; 00223 00224 if( ctx == NULL || ilen < 1 || ilen > ctx->len ) 00225 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00226 00227 if( ( ret = mbedtls_mpi_read_binary( &ctx->GY , input, ilen ) ) != 0 ) 00228 return( MBEDTLS_ERR_DHM_READ_PUBLIC_FAILED + ret ); 00229 00230 return( 0 ); 00231 } 00232 00233 /* 00234 * Create own private value X and export G^X 00235 */ 00236 int mbedtls_dhm_make_public( mbedtls_dhm_context *ctx, int x_size, 00237 unsigned char *output, size_t olen, 00238 int (*f_rng)(void *, unsigned char *, size_t), 00239 void *p_rng ) 00240 { 00241 int ret, count = 0; 00242 00243 if( ctx == NULL || olen < 1 || olen > ctx->len ) 00244 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00245 00246 if( mbedtls_mpi_cmp_int( &ctx->P , 0 ) == 0 ) 00247 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00248 00249 /* 00250 * generate X and calculate GX = G^X mod P 00251 */ 00252 do 00253 { 00254 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &ctx->X , x_size, f_rng, p_rng ) ); 00255 00256 while( mbedtls_mpi_cmp_mpi( &ctx->X , &ctx->P ) >= 0 ) 00257 MBEDTLS_MPI_CHK( mbedtls_mpi_shift_r( &ctx->X , 1 ) ); 00258 00259 if( count++ > 10 ) 00260 return( MBEDTLS_ERR_DHM_MAKE_PUBLIC_FAILED ); 00261 } 00262 while( dhm_check_range( &ctx->X , &ctx->P ) != 0 ); 00263 00264 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &ctx->GX , &ctx->G , &ctx->X , 00265 &ctx->P , &ctx->RP ) ); 00266 00267 if( ( ret = dhm_check_range( &ctx->GX , &ctx->P ) ) != 0 ) 00268 return( ret ); 00269 00270 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( &ctx->GX , output, olen ) ); 00271 00272 cleanup: 00273 00274 if( ret != 0 ) 00275 return( MBEDTLS_ERR_DHM_MAKE_PUBLIC_FAILED + ret ); 00276 00277 return( 0 ); 00278 } 00279 00280 /* 00281 * Use the blinding method and optimisation suggested in section 10 of: 00282 * KOCHER, Paul C. Timing attacks on implementations of Diffie-Hellman, RSA, 00283 * DSS, and other systems. In : Advances in Cryptology-CRYPTO'96. Springer 00284 * Berlin Heidelberg, 1996. p. 104-113. 00285 */ 00286 static int dhm_update_blinding( mbedtls_dhm_context *ctx, 00287 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00288 { 00289 int ret, count; 00290 00291 /* 00292 * Don't use any blinding the first time a particular X is used, 00293 * but remember it to use blinding next time. 00294 */ 00295 if( mbedtls_mpi_cmp_mpi( &ctx->X , &ctx->pX ) != 0 ) 00296 { 00297 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &ctx->pX , &ctx->X ) ); 00298 MBEDTLS_MPI_CHK( mbedtls_mpi_lset( &ctx->Vi , 1 ) ); 00299 MBEDTLS_MPI_CHK( mbedtls_mpi_lset( &ctx->Vf , 1 ) ); 00300 00301 return( 0 ); 00302 } 00303 00304 /* 00305 * Ok, we need blinding. Can we re-use existing values? 00306 * If yes, just update them by squaring them. 00307 */ 00308 if( mbedtls_mpi_cmp_int( &ctx->Vi , 1 ) != 0 ) 00309 { 00310 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->Vi , &ctx->Vi , &ctx->Vi ) ); 00311 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->Vi , &ctx->Vi , &ctx->P ) ); 00312 00313 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->Vf , &ctx->Vf , &ctx->Vf ) ); 00314 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->Vf , &ctx->Vf , &ctx->P ) ); 00315 00316 return( 0 ); 00317 } 00318 00319 /* 00320 * We need to generate blinding values from scratch 00321 */ 00322 00323 /* Vi = random( 2, P-1 ) */ 00324 count = 0; 00325 do 00326 { 00327 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &ctx->Vi , mbedtls_mpi_size( &ctx->P ), f_rng, p_rng ) ); 00328 00329 while( mbedtls_mpi_cmp_mpi( &ctx->Vi , &ctx->P ) >= 0 ) 00330 MBEDTLS_MPI_CHK( mbedtls_mpi_shift_r( &ctx->Vi , 1 ) ); 00331 00332 if( count++ > 10 ) 00333 return( MBEDTLS_ERR_MPI_NOT_ACCEPTABLE ); 00334 } 00335 while( mbedtls_mpi_cmp_int( &ctx->Vi , 1 ) <= 0 ); 00336 00337 /* Vf = Vi^-X mod P */ 00338 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->Vf , &ctx->Vi , &ctx->P ) ); 00339 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &ctx->Vf , &ctx->Vf , &ctx->X , &ctx->P , &ctx->RP ) ); 00340 00341 cleanup: 00342 return( ret ); 00343 } 00344 00345 /* 00346 * Derive and export the shared secret (G^Y)^X mod P 00347 */ 00348 int mbedtls_dhm_calc_secret( mbedtls_dhm_context *ctx, 00349 unsigned char *output, size_t output_size, size_t *olen, 00350 int (*f_rng)(void *, unsigned char *, size_t), 00351 void *p_rng ) 00352 { 00353 int ret; 00354 mbedtls_mpi GYb; 00355 00356 if( ctx == NULL || output_size < ctx->len ) 00357 return( MBEDTLS_ERR_DHM_BAD_INPUT_DATA ); 00358 00359 if( ( ret = dhm_check_range( &ctx->GY , &ctx->P ) ) != 0 ) 00360 return( ret ); 00361 00362 mbedtls_mpi_init( &GYb ); 00363 00364 /* Blind peer's value */ 00365 if( f_rng != NULL ) 00366 { 00367 MBEDTLS_MPI_CHK( dhm_update_blinding( ctx, f_rng, p_rng ) ); 00368 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &GYb, &ctx->GY , &ctx->Vi ) ); 00369 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &GYb, &GYb, &ctx->P ) ); 00370 } 00371 else 00372 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &GYb, &ctx->GY ) ); 00373 00374 /* Do modular exponentiation */ 00375 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &ctx->K , &GYb, &ctx->X , 00376 &ctx->P , &ctx->RP ) ); 00377 00378 /* Unblind secret value */ 00379 if( f_rng != NULL ) 00380 { 00381 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->K , &ctx->K , &ctx->Vf ) ); 00382 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->K , &ctx->K , &ctx->P ) ); 00383 } 00384 00385 *olen = mbedtls_mpi_size( &ctx->K ); 00386 00387 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( &ctx->K , output, *olen ) ); 00388 00389 cleanup: 00390 mbedtls_mpi_free( &GYb ); 00391 00392 if( ret != 0 ) 00393 return( MBEDTLS_ERR_DHM_CALC_SECRET_FAILED + ret ); 00394 00395 return( 0 ); 00396 } 00397 00398 /* 00399 * Free the components of a DHM key 00400 */ 00401 void mbedtls_dhm_free( mbedtls_dhm_context *ctx ) 00402 { 00403 mbedtls_mpi_free( &ctx->pX ); mbedtls_mpi_free( &ctx->Vf ); mbedtls_mpi_free( &ctx->Vi ); 00404 mbedtls_mpi_free( &ctx->RP ); mbedtls_mpi_free( &ctx->K ); mbedtls_mpi_free( &ctx->GY ); 00405 mbedtls_mpi_free( &ctx->GX ); mbedtls_mpi_free( &ctx->X ); mbedtls_mpi_free( &ctx->G ); 00406 mbedtls_mpi_free( &ctx->P ); 00407 00408 mbedtls_zeroize( ctx, sizeof( mbedtls_dhm_context ) ); 00409 } 00410 00411 #if defined(MBEDTLS_ASN1_PARSE_C) 00412 /* 00413 * Parse DHM parameters 00414 */ 00415 int mbedtls_dhm_parse_dhm( mbedtls_dhm_context *dhm, const unsigned char *dhmin, 00416 size_t dhminlen ) 00417 { 00418 int ret; 00419 size_t len; 00420 unsigned char *p, *end; 00421 #if defined(MBEDTLS_PEM_PARSE_C) 00422 mbedtls_pem_context pem; 00423 00424 mbedtls_pem_init( &pem ); 00425 00426 /* Avoid calling mbedtls_pem_read_buffer() on non-null-terminated string */ 00427 if( dhminlen == 0 || dhmin[dhminlen - 1] != '\0' ) 00428 ret = MBEDTLS_ERR_PEM_NO_HEADER_FOOTER_PRESENT; 00429 else 00430 ret = mbedtls_pem_read_buffer( &pem, 00431 "-----BEGIN DH PARAMETERS-----", 00432 "-----END DH PARAMETERS-----", 00433 dhmin, NULL, 0, &dhminlen ); 00434 00435 if( ret == 0 ) 00436 { 00437 /* 00438 * Was PEM encoded 00439 */ 00440 dhminlen = pem.buflen ; 00441 } 00442 else if( ret != MBEDTLS_ERR_PEM_NO_HEADER_FOOTER_PRESENT ) 00443 goto exit; 00444 00445 p = ( ret == 0 ) ? pem.buf : (unsigned char *) dhmin; 00446 #else 00447 p = (unsigned char *) dhmin; 00448 #endif /* MBEDTLS_PEM_PARSE_C */ 00449 end = p + dhminlen; 00450 00451 /* 00452 * DHParams ::= SEQUENCE { 00453 * prime INTEGER, -- P 00454 * generator INTEGER, -- g 00455 * privateValueLength INTEGER OPTIONAL 00456 * } 00457 */ 00458 if( ( ret = mbedtls_asn1_get_tag( &p, end, &len, 00459 MBEDTLS_ASN1_CONSTRUCTED | MBEDTLS_ASN1_SEQUENCE ) ) != 0 ) 00460 { 00461 ret = MBEDTLS_ERR_DHM_INVALID_FORMAT + ret; 00462 goto exit; 00463 } 00464 00465 end = p + len; 00466 00467 if( ( ret = mbedtls_asn1_get_mpi( &p, end, &dhm->P ) ) != 0 || 00468 ( ret = mbedtls_asn1_get_mpi( &p, end, &dhm->G ) ) != 0 ) 00469 { 00470 ret = MBEDTLS_ERR_DHM_INVALID_FORMAT + ret; 00471 goto exit; 00472 } 00473 00474 if( p != end ) 00475 { 00476 /* This might be the optional privateValueLength. 00477 * If so, we can cleanly discard it */ 00478 mbedtls_mpi rec; 00479 mbedtls_mpi_init( &rec ); 00480 ret = mbedtls_asn1_get_mpi( &p, end, &rec ); 00481 mbedtls_mpi_free( &rec ); 00482 if ( ret != 0 ) 00483 { 00484 ret = MBEDTLS_ERR_DHM_INVALID_FORMAT + ret; 00485 goto exit; 00486 } 00487 if ( p != end ) 00488 { 00489 ret = MBEDTLS_ERR_DHM_INVALID_FORMAT + 00490 MBEDTLS_ERR_ASN1_LENGTH_MISMATCH; 00491 goto exit; 00492 } 00493 } 00494 00495 ret = 0; 00496 00497 dhm->len = mbedtls_mpi_size( &dhm->P ); 00498 00499 exit: 00500 #if defined(MBEDTLS_PEM_PARSE_C) 00501 mbedtls_pem_free( &pem ); 00502 #endif 00503 if( ret != 0 ) 00504 mbedtls_dhm_free( dhm ); 00505 00506 return( ret ); 00507 } 00508 00509 #if defined(MBEDTLS_FS_IO) 00510 /* 00511 * Load all data from a file into a given buffer. 00512 * 00513 * The file is expected to contain either PEM or DER encoded data. 00514 * A terminating null byte is always appended. It is included in the announced 00515 * length only if the data looks like it is PEM encoded. 00516 */ 00517 static int load_file( const char *path, unsigned char **buf, size_t *n ) 00518 { 00519 FILE *f; 00520 long size; 00521 00522 if( ( f = fopen( path, "rb" ) ) == NULL ) 00523 return( MBEDTLS_ERR_DHM_FILE_IO_ERROR ); 00524 00525 fseek( f, 0, SEEK_END ); 00526 if( ( size = ftell( f ) ) == -1 ) 00527 { 00528 fclose( f ); 00529 return( MBEDTLS_ERR_DHM_FILE_IO_ERROR ); 00530 } 00531 fseek( f, 0, SEEK_SET ); 00532 00533 *n = (size_t) size; 00534 00535 if( *n + 1 == 0 || 00536 ( *buf = mbedtls_calloc( 1, *n + 1 ) ) == NULL ) 00537 { 00538 fclose( f ); 00539 return( MBEDTLS_ERR_DHM_ALLOC_FAILED ); 00540 } 00541 00542 if( fread( *buf, 1, *n, f ) != *n ) 00543 { 00544 fclose( f ); 00545 mbedtls_free( *buf ); 00546 return( MBEDTLS_ERR_DHM_FILE_IO_ERROR ); 00547 } 00548 00549 fclose( f ); 00550 00551 (*buf)[*n] = '\0'; 00552 00553 if( strstr( (const char *) *buf, "-----BEGIN " ) != NULL ) 00554 ++*n; 00555 00556 return( 0 ); 00557 } 00558 00559 /* 00560 * Load and parse DHM parameters 00561 */ 00562 int mbedtls_dhm_parse_dhmfile( mbedtls_dhm_context *dhm, const char *path ) 00563 { 00564 int ret; 00565 size_t n; 00566 unsigned char *buf; 00567 00568 if( ( ret = load_file( path, &buf, &n ) ) != 0 ) 00569 return( ret ); 00570 00571 ret = mbedtls_dhm_parse_dhm( dhm, buf, n ); 00572 00573 mbedtls_zeroize( buf, n ); 00574 mbedtls_free( buf ); 00575 00576 return( ret ); 00577 } 00578 #endif /* MBEDTLS_FS_IO */ 00579 #endif /* MBEDTLS_ASN1_PARSE_C */ 00580 00581 #if defined(MBEDTLS_SELF_TEST) 00582 00583 static const char mbedtls_test_dhm_params[] = 00584 "-----BEGIN DH PARAMETERS-----\r\n" 00585 "MIGHAoGBAJ419DBEOgmQTzo5qXl5fQcN9TN455wkOL7052HzxxRVMyhYmwQcgJvh\r\n" 00586 "1sa18fyfR9OiVEMYglOpkqVoGLN7qd5aQNNi5W7/C+VBdHTBJcGZJyyP5B3qcz32\r\n" 00587 "9mLJKudlVudV0Qxk5qUJaPZ/xupz0NyoVpviuiBOI1gNi8ovSXWzAgEC\r\n" 00588 "-----END DH PARAMETERS-----\r\n"; 00589 00590 static const size_t mbedtls_test_dhm_params_len = sizeof( mbedtls_test_dhm_params ); 00591 00592 /* 00593 * Checkup routine 00594 */ 00595 int mbedtls_dhm_self_test( int verbose ) 00596 { 00597 int ret; 00598 mbedtls_dhm_context dhm; 00599 00600 mbedtls_dhm_init( &dhm ); 00601 00602 if( verbose != 0 ) 00603 mbedtls_printf( " DHM parameter load: " ); 00604 00605 if( ( ret = mbedtls_dhm_parse_dhm( &dhm, 00606 (const unsigned char *) mbedtls_test_dhm_params, 00607 mbedtls_test_dhm_params_len ) ) != 0 ) 00608 { 00609 if( verbose != 0 ) 00610 mbedtls_printf( "failed\n" ); 00611 00612 ret = 1; 00613 goto exit; 00614 } 00615 00616 if( verbose != 0 ) 00617 mbedtls_printf( "passed\n\n" ); 00618 00619 exit: 00620 mbedtls_dhm_free( &dhm ); 00621 00622 return( ret ); 00623 } 00624 00625 #endif /* MBEDTLS_SELF_TEST */ 00626 00627 #endif /* MBEDTLS_DHM_C */
Generated on Sun Jul 17 2022 08:25:21 by
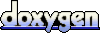