
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
detect_targets_test.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2017 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import unittest 00019 from mock import patch 00020 from tools.detect_targets import get_interface_version 00021 00022 00023 class MbedLsToolsMock (): 00024 """ 00025 Mock of mbedls tools 00026 """ 00027 00028 def __init__(self, test_type): 00029 self.interface_test_type = test_type 00030 00031 def get_details_txt(self, mount_point): 00032 return self.details_txt_types [self.interface_test_type ]; 00033 00034 # Static details.txt types. 00035 details_txt_types = { 00036 'details_valid_interface_version' : { 00037 'Unique ID': '0226000029164e45002f0012706e0006f301000097969900', 00038 'HIF ID': '97969900', 00039 'Auto Reset': '0', 00040 'Automation allowed': '0', 00041 'Daplink Mode': 'Interface', 00042 'Interface Version': '0240', 00043 'Git SHA': 'c765cbb590f57598756683254ca38b211693ae5e', 00044 'Local Mods': '0', 00045 'USB Interfaces': 'MSD, CDC, HID', 00046 'Interface CRC': '0x26764ebf' 00047 }, 00048 'details_valid_version' : { 00049 'Version': '0226', 00050 'Build': 'Aug 24 2015 17:06:30', 00051 'Git Commit SHA': '27a236b9fe39c674a703c5c89655fbd26b8e27e1', 00052 'Git Local mods': 'Yes' 00053 }, 00054 'details_missing_interface_version' : { 00055 'Unique ID': '0226000033514e450044500585d4001de981000097969900', 00056 'HIC ID': '97969900', 00057 'Auto Reset': '0', 00058 'Automation allowed': '0', 00059 'Overflow detection': '0', 00060 'Daplink Mode': 'Interface', 00061 'Git SHA': 'b403a07e3696cee1e116d44cbdd64446e056ce38', 00062 'Local Mods': '0', 00063 'USB Interfaces': 'MSD, CDC, HID', 00064 'Interface CRC': '0x4d98bf7e', 00065 'Remount count': '0' 00066 }, 00067 'details_invalid_none' : None 00068 } 00069 00070 """ 00071 Tests for detect_targets.py 00072 """ 00073 00074 class DetectTargetsTest (unittest.TestCase): 00075 """ 00076 Test cases for Detect Target functionality 00077 """ 00078 00079 def setUp (self): 00080 """ 00081 Called before each test case 00082 00083 :return: 00084 """ 00085 self.missing_mount_point = None 00086 self.mount_point = "D:" 00087 00088 def tearDown (self): 00089 """ 00090 Nothing to tear down. 00091 Called after each test case 00092 00093 :return: 00094 """ 00095 pass 00096 00097 @patch("mbed_lstools.create", return_value=MbedLsToolsMock('details_valid_interface_version')) 00098 def test_interface_version_valid (self, mbed_lstools_mock): 00099 """ 00100 Test that checks function returns correctly when given a valid Interface Version 00101 00102 :param mbed_lstools_mock: Mocks Mbed LS tools with MbedLsToolsMock 00103 :return 00104 """ 00105 00106 interface_version = get_interface_version(self.mount_point ) 00107 assert interface_version == '0240' 00108 00109 @patch("mbed_lstools.create", return_value=MbedLsToolsMock('details_valid_version')) 00110 def test_version_valid (self, mbed_lstools_mock): 00111 """ 00112 Test that checks function returns correctly when given a valid Version 00113 00114 :param mbed_lstools_mock: Mocks Mbed LS tools with MbedLsToolsMock 00115 :return 00116 """ 00117 00118 interface_version = get_interface_version(self.mount_point ) 00119 assert interface_version == '0226' 00120 00121 @patch("mbed_lstools.create", return_value=MbedLsToolsMock('details_missing_interface_version')) 00122 def test_interface_version_missing_interface_version (self, mbed_lstools_mock): 00123 """ 00124 Test that checks function returns correctly when DETAILS.txt is present 00125 but an interface version is not listed. 00126 00127 :param mbed_lstools_mock: Mocks Mbed LS tools with MbedLsToolsMock 00128 :return 00129 """ 00130 00131 interface_version = get_interface_version(self.mount_point ) 00132 assert interface_version == 'unknown' 00133 00134 @patch("mbed_lstools.create", return_value=MbedLsToolsMock('details_invalid_none')) 00135 def test_version_none (self, mbed_lstools_mock): 00136 """ 00137 Test that checks function returns correctly when a valid mount point is supplied 00138 but DETAILS.txt is not present. 00139 00140 :param mbed_lstools_mock: Mocks Mbed LS tools with MbedLsToolsMock 00141 :return 00142 """ 00143 00144 interface_version = get_interface_version(self.mount_point ) 00145 assert interface_version == 'unknown' 00146 00147 @patch("mbed_lstools.create", return_value=MbedLsToolsMock('details_invalid_none')) 00148 def test_interface_version_missing_mount_point (self, mbed_lstools_mock): 00149 """ 00150 Test that checks function returns correctly when no mount point is supplied. 00151 00152 :param mbed_lstools_mock: Mocks Mbed LS tools with MbedLsToolsMock 00153 :return 00154 """ 00155 00156 interface_version = get_interface_version(self.missing_mount_point ) 00157 assert interface_version == 'unknown' 00158 00159 if __name__ == '__main__': 00160 unittest.main()
Generated on Sun Jul 17 2022 08:25:21 by
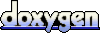