
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
des.c
00001 /* 00002 * FIPS-46-3 compliant Triple-DES implementation 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * DES, on which TDES is based, was originally designed by Horst Feistel 00023 * at IBM in 1974, and was adopted as a standard by NIST (formerly NBS). 00024 * 00025 * http://csrc.nist.gov/publications/fips/fips46-3/fips46-3.pdf 00026 */ 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "mbedtls/config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #if defined(MBEDTLS_DES_C) 00035 00036 #include "mbedtls/des.h" 00037 00038 #include <string.h> 00039 00040 #if defined(MBEDTLS_SELF_TEST) 00041 #if defined(MBEDTLS_PLATFORM_C) 00042 #include "mbedtls/platform.h" 00043 #else 00044 #include <stdio.h> 00045 #define mbedtls_printf printf 00046 #endif /* MBEDTLS_PLATFORM_C */ 00047 #endif /* MBEDTLS_SELF_TEST */ 00048 00049 #if !defined(MBEDTLS_DES_ALT) 00050 00051 /* Implementation that should never be optimized out by the compiler */ 00052 static void mbedtls_zeroize( void *v, size_t n ) { 00053 volatile unsigned char *p = (unsigned char*)v; while( n-- ) *p++ = 0; 00054 } 00055 00056 /* 00057 * 32-bit integer manipulation macros (big endian) 00058 */ 00059 #ifndef GET_UINT32_BE 00060 #define GET_UINT32_BE(n,b,i) \ 00061 { \ 00062 (n) = ( (uint32_t) (b)[(i) ] << 24 ) \ 00063 | ( (uint32_t) (b)[(i) + 1] << 16 ) \ 00064 | ( (uint32_t) (b)[(i) + 2] << 8 ) \ 00065 | ( (uint32_t) (b)[(i) + 3] ); \ 00066 } 00067 #endif 00068 00069 #ifndef PUT_UINT32_BE 00070 #define PUT_UINT32_BE(n,b,i) \ 00071 { \ 00072 (b)[(i) ] = (unsigned char) ( (n) >> 24 ); \ 00073 (b)[(i) + 1] = (unsigned char) ( (n) >> 16 ); \ 00074 (b)[(i) + 2] = (unsigned char) ( (n) >> 8 ); \ 00075 (b)[(i) + 3] = (unsigned char) ( (n) ); \ 00076 } 00077 #endif 00078 00079 /* 00080 * Expanded DES S-boxes 00081 */ 00082 static const uint32_t SB1[64] = 00083 { 00084 0x01010400, 0x00000000, 0x00010000, 0x01010404, 00085 0x01010004, 0x00010404, 0x00000004, 0x00010000, 00086 0x00000400, 0x01010400, 0x01010404, 0x00000400, 00087 0x01000404, 0x01010004, 0x01000000, 0x00000004, 00088 0x00000404, 0x01000400, 0x01000400, 0x00010400, 00089 0x00010400, 0x01010000, 0x01010000, 0x01000404, 00090 0x00010004, 0x01000004, 0x01000004, 0x00010004, 00091 0x00000000, 0x00000404, 0x00010404, 0x01000000, 00092 0x00010000, 0x01010404, 0x00000004, 0x01010000, 00093 0x01010400, 0x01000000, 0x01000000, 0x00000400, 00094 0x01010004, 0x00010000, 0x00010400, 0x01000004, 00095 0x00000400, 0x00000004, 0x01000404, 0x00010404, 00096 0x01010404, 0x00010004, 0x01010000, 0x01000404, 00097 0x01000004, 0x00000404, 0x00010404, 0x01010400, 00098 0x00000404, 0x01000400, 0x01000400, 0x00000000, 00099 0x00010004, 0x00010400, 0x00000000, 0x01010004 00100 }; 00101 00102 static const uint32_t SB2[64] = 00103 { 00104 0x80108020, 0x80008000, 0x00008000, 0x00108020, 00105 0x00100000, 0x00000020, 0x80100020, 0x80008020, 00106 0x80000020, 0x80108020, 0x80108000, 0x80000000, 00107 0x80008000, 0x00100000, 0x00000020, 0x80100020, 00108 0x00108000, 0x00100020, 0x80008020, 0x00000000, 00109 0x80000000, 0x00008000, 0x00108020, 0x80100000, 00110 0x00100020, 0x80000020, 0x00000000, 0x00108000, 00111 0x00008020, 0x80108000, 0x80100000, 0x00008020, 00112 0x00000000, 0x00108020, 0x80100020, 0x00100000, 00113 0x80008020, 0x80100000, 0x80108000, 0x00008000, 00114 0x80100000, 0x80008000, 0x00000020, 0x80108020, 00115 0x00108020, 0x00000020, 0x00008000, 0x80000000, 00116 0x00008020, 0x80108000, 0x00100000, 0x80000020, 00117 0x00100020, 0x80008020, 0x80000020, 0x00100020, 00118 0x00108000, 0x00000000, 0x80008000, 0x00008020, 00119 0x80000000, 0x80100020, 0x80108020, 0x00108000 00120 }; 00121 00122 static const uint32_t SB3[64] = 00123 { 00124 0x00000208, 0x08020200, 0x00000000, 0x08020008, 00125 0x08000200, 0x00000000, 0x00020208, 0x08000200, 00126 0x00020008, 0x08000008, 0x08000008, 0x00020000, 00127 0x08020208, 0x00020008, 0x08020000, 0x00000208, 00128 0x08000000, 0x00000008, 0x08020200, 0x00000200, 00129 0x00020200, 0x08020000, 0x08020008, 0x00020208, 00130 0x08000208, 0x00020200, 0x00020000, 0x08000208, 00131 0x00000008, 0x08020208, 0x00000200, 0x08000000, 00132 0x08020200, 0x08000000, 0x00020008, 0x00000208, 00133 0x00020000, 0x08020200, 0x08000200, 0x00000000, 00134 0x00000200, 0x00020008, 0x08020208, 0x08000200, 00135 0x08000008, 0x00000200, 0x00000000, 0x08020008, 00136 0x08000208, 0x00020000, 0x08000000, 0x08020208, 00137 0x00000008, 0x00020208, 0x00020200, 0x08000008, 00138 0x08020000, 0x08000208, 0x00000208, 0x08020000, 00139 0x00020208, 0x00000008, 0x08020008, 0x00020200 00140 }; 00141 00142 static const uint32_t SB4[64] = 00143 { 00144 0x00802001, 0x00002081, 0x00002081, 0x00000080, 00145 0x00802080, 0x00800081, 0x00800001, 0x00002001, 00146 0x00000000, 0x00802000, 0x00802000, 0x00802081, 00147 0x00000081, 0x00000000, 0x00800080, 0x00800001, 00148 0x00000001, 0x00002000, 0x00800000, 0x00802001, 00149 0x00000080, 0x00800000, 0x00002001, 0x00002080, 00150 0x00800081, 0x00000001, 0x00002080, 0x00800080, 00151 0x00002000, 0x00802080, 0x00802081, 0x00000081, 00152 0x00800080, 0x00800001, 0x00802000, 0x00802081, 00153 0x00000081, 0x00000000, 0x00000000, 0x00802000, 00154 0x00002080, 0x00800080, 0x00800081, 0x00000001, 00155 0x00802001, 0x00002081, 0x00002081, 0x00000080, 00156 0x00802081, 0x00000081, 0x00000001, 0x00002000, 00157 0x00800001, 0x00002001, 0x00802080, 0x00800081, 00158 0x00002001, 0x00002080, 0x00800000, 0x00802001, 00159 0x00000080, 0x00800000, 0x00002000, 0x00802080 00160 }; 00161 00162 static const uint32_t SB5[64] = 00163 { 00164 0x00000100, 0x02080100, 0x02080000, 0x42000100, 00165 0x00080000, 0x00000100, 0x40000000, 0x02080000, 00166 0x40080100, 0x00080000, 0x02000100, 0x40080100, 00167 0x42000100, 0x42080000, 0x00080100, 0x40000000, 00168 0x02000000, 0x40080000, 0x40080000, 0x00000000, 00169 0x40000100, 0x42080100, 0x42080100, 0x02000100, 00170 0x42080000, 0x40000100, 0x00000000, 0x42000000, 00171 0x02080100, 0x02000000, 0x42000000, 0x00080100, 00172 0x00080000, 0x42000100, 0x00000100, 0x02000000, 00173 0x40000000, 0x02080000, 0x42000100, 0x40080100, 00174 0x02000100, 0x40000000, 0x42080000, 0x02080100, 00175 0x40080100, 0x00000100, 0x02000000, 0x42080000, 00176 0x42080100, 0x00080100, 0x42000000, 0x42080100, 00177 0x02080000, 0x00000000, 0x40080000, 0x42000000, 00178 0x00080100, 0x02000100, 0x40000100, 0x00080000, 00179 0x00000000, 0x40080000, 0x02080100, 0x40000100 00180 }; 00181 00182 static const uint32_t SB6[64] = 00183 { 00184 0x20000010, 0x20400000, 0x00004000, 0x20404010, 00185 0x20400000, 0x00000010, 0x20404010, 0x00400000, 00186 0x20004000, 0x00404010, 0x00400000, 0x20000010, 00187 0x00400010, 0x20004000, 0x20000000, 0x00004010, 00188 0x00000000, 0x00400010, 0x20004010, 0x00004000, 00189 0x00404000, 0x20004010, 0x00000010, 0x20400010, 00190 0x20400010, 0x00000000, 0x00404010, 0x20404000, 00191 0x00004010, 0x00404000, 0x20404000, 0x20000000, 00192 0x20004000, 0x00000010, 0x20400010, 0x00404000, 00193 0x20404010, 0x00400000, 0x00004010, 0x20000010, 00194 0x00400000, 0x20004000, 0x20000000, 0x00004010, 00195 0x20000010, 0x20404010, 0x00404000, 0x20400000, 00196 0x00404010, 0x20404000, 0x00000000, 0x20400010, 00197 0x00000010, 0x00004000, 0x20400000, 0x00404010, 00198 0x00004000, 0x00400010, 0x20004010, 0x00000000, 00199 0x20404000, 0x20000000, 0x00400010, 0x20004010 00200 }; 00201 00202 static const uint32_t SB7[64] = 00203 { 00204 0x00200000, 0x04200002, 0x04000802, 0x00000000, 00205 0x00000800, 0x04000802, 0x00200802, 0x04200800, 00206 0x04200802, 0x00200000, 0x00000000, 0x04000002, 00207 0x00000002, 0x04000000, 0x04200002, 0x00000802, 00208 0x04000800, 0x00200802, 0x00200002, 0x04000800, 00209 0x04000002, 0x04200000, 0x04200800, 0x00200002, 00210 0x04200000, 0x00000800, 0x00000802, 0x04200802, 00211 0x00200800, 0x00000002, 0x04000000, 0x00200800, 00212 0x04000000, 0x00200800, 0x00200000, 0x04000802, 00213 0x04000802, 0x04200002, 0x04200002, 0x00000002, 00214 0x00200002, 0x04000000, 0x04000800, 0x00200000, 00215 0x04200800, 0x00000802, 0x00200802, 0x04200800, 00216 0x00000802, 0x04000002, 0x04200802, 0x04200000, 00217 0x00200800, 0x00000000, 0x00000002, 0x04200802, 00218 0x00000000, 0x00200802, 0x04200000, 0x00000800, 00219 0x04000002, 0x04000800, 0x00000800, 0x00200002 00220 }; 00221 00222 static const uint32_t SB8[64] = 00223 { 00224 0x10001040, 0x00001000, 0x00040000, 0x10041040, 00225 0x10000000, 0x10001040, 0x00000040, 0x10000000, 00226 0x00040040, 0x10040000, 0x10041040, 0x00041000, 00227 0x10041000, 0x00041040, 0x00001000, 0x00000040, 00228 0x10040000, 0x10000040, 0x10001000, 0x00001040, 00229 0x00041000, 0x00040040, 0x10040040, 0x10041000, 00230 0x00001040, 0x00000000, 0x00000000, 0x10040040, 00231 0x10000040, 0x10001000, 0x00041040, 0x00040000, 00232 0x00041040, 0x00040000, 0x10041000, 0x00001000, 00233 0x00000040, 0x10040040, 0x00001000, 0x00041040, 00234 0x10001000, 0x00000040, 0x10000040, 0x10040000, 00235 0x10040040, 0x10000000, 0x00040000, 0x10001040, 00236 0x00000000, 0x10041040, 0x00040040, 0x10000040, 00237 0x10040000, 0x10001000, 0x10001040, 0x00000000, 00238 0x10041040, 0x00041000, 0x00041000, 0x00001040, 00239 0x00001040, 0x00040040, 0x10000000, 0x10041000 00240 }; 00241 00242 /* 00243 * PC1: left and right halves bit-swap 00244 */ 00245 static const uint32_t LHs[16] = 00246 { 00247 0x00000000, 0x00000001, 0x00000100, 0x00000101, 00248 0x00010000, 0x00010001, 0x00010100, 0x00010101, 00249 0x01000000, 0x01000001, 0x01000100, 0x01000101, 00250 0x01010000, 0x01010001, 0x01010100, 0x01010101 00251 }; 00252 00253 static const uint32_t RHs[16] = 00254 { 00255 0x00000000, 0x01000000, 0x00010000, 0x01010000, 00256 0x00000100, 0x01000100, 0x00010100, 0x01010100, 00257 0x00000001, 0x01000001, 0x00010001, 0x01010001, 00258 0x00000101, 0x01000101, 0x00010101, 0x01010101, 00259 }; 00260 00261 /* 00262 * Initial Permutation macro 00263 */ 00264 #define DES_IP(X,Y) \ 00265 { \ 00266 T = ((X >> 4) ^ Y) & 0x0F0F0F0F; Y ^= T; X ^= (T << 4); \ 00267 T = ((X >> 16) ^ Y) & 0x0000FFFF; Y ^= T; X ^= (T << 16); \ 00268 T = ((Y >> 2) ^ X) & 0x33333333; X ^= T; Y ^= (T << 2); \ 00269 T = ((Y >> 8) ^ X) & 0x00FF00FF; X ^= T; Y ^= (T << 8); \ 00270 Y = ((Y << 1) | (Y >> 31)) & 0xFFFFFFFF; \ 00271 T = (X ^ Y) & 0xAAAAAAAA; Y ^= T; X ^= T; \ 00272 X = ((X << 1) | (X >> 31)) & 0xFFFFFFFF; \ 00273 } 00274 00275 /* 00276 * Final Permutation macro 00277 */ 00278 #define DES_FP(X,Y) \ 00279 { \ 00280 X = ((X << 31) | (X >> 1)) & 0xFFFFFFFF; \ 00281 T = (X ^ Y) & 0xAAAAAAAA; X ^= T; Y ^= T; \ 00282 Y = ((Y << 31) | (Y >> 1)) & 0xFFFFFFFF; \ 00283 T = ((Y >> 8) ^ X) & 0x00FF00FF; X ^= T; Y ^= (T << 8); \ 00284 T = ((Y >> 2) ^ X) & 0x33333333; X ^= T; Y ^= (T << 2); \ 00285 T = ((X >> 16) ^ Y) & 0x0000FFFF; Y ^= T; X ^= (T << 16); \ 00286 T = ((X >> 4) ^ Y) & 0x0F0F0F0F; Y ^= T; X ^= (T << 4); \ 00287 } 00288 00289 /* 00290 * DES round macro 00291 */ 00292 #define DES_ROUND(X,Y) \ 00293 { \ 00294 T = *SK++ ^ X; \ 00295 Y ^= SB8[ (T ) & 0x3F ] ^ \ 00296 SB6[ (T >> 8) & 0x3F ] ^ \ 00297 SB4[ (T >> 16) & 0x3F ] ^ \ 00298 SB2[ (T >> 24) & 0x3F ]; \ 00299 \ 00300 T = *SK++ ^ ((X << 28) | (X >> 4)); \ 00301 Y ^= SB7[ (T ) & 0x3F ] ^ \ 00302 SB5[ (T >> 8) & 0x3F ] ^ \ 00303 SB3[ (T >> 16) & 0x3F ] ^ \ 00304 SB1[ (T >> 24) & 0x3F ]; \ 00305 } 00306 00307 #define SWAP(a,b) { uint32_t t = a; a = b; b = t; t = 0; } 00308 00309 void mbedtls_des_init( mbedtls_des_context *ctx ) 00310 { 00311 memset( ctx, 0, sizeof( mbedtls_des_context ) ); 00312 } 00313 00314 void mbedtls_des_free( mbedtls_des_context *ctx ) 00315 { 00316 if( ctx == NULL ) 00317 return; 00318 00319 mbedtls_zeroize( ctx, sizeof( mbedtls_des_context ) ); 00320 } 00321 00322 void mbedtls_des3_init( mbedtls_des3_context *ctx ) 00323 { 00324 memset( ctx, 0, sizeof( mbedtls_des3_context ) ); 00325 } 00326 00327 void mbedtls_des3_free( mbedtls_des3_context *ctx ) 00328 { 00329 if( ctx == NULL ) 00330 return; 00331 00332 mbedtls_zeroize( ctx, sizeof( mbedtls_des3_context ) ); 00333 } 00334 00335 static const unsigned char odd_parity_table[128] = { 1, 2, 4, 7, 8, 00336 11, 13, 14, 16, 19, 21, 22, 25, 26, 28, 31, 32, 35, 37, 38, 41, 42, 44, 00337 47, 49, 50, 52, 55, 56, 59, 61, 62, 64, 67, 69, 70, 73, 74, 76, 79, 81, 00338 82, 84, 87, 88, 91, 93, 94, 97, 98, 100, 103, 104, 107, 109, 110, 112, 00339 115, 117, 118, 121, 122, 124, 127, 128, 131, 133, 134, 137, 138, 140, 00340 143, 145, 146, 148, 151, 152, 155, 157, 158, 161, 162, 164, 167, 168, 00341 171, 173, 174, 176, 179, 181, 182, 185, 186, 188, 191, 193, 194, 196, 00342 199, 200, 203, 205, 206, 208, 211, 213, 214, 217, 218, 220, 223, 224, 00343 227, 229, 230, 233, 234, 236, 239, 241, 242, 244, 247, 248, 251, 253, 00344 254 }; 00345 00346 void mbedtls_des_key_set_parity( unsigned char key[MBEDTLS_DES_KEY_SIZE] ) 00347 { 00348 int i; 00349 00350 for( i = 0; i < MBEDTLS_DES_KEY_SIZE; i++ ) 00351 key[i] = odd_parity_table[key[i] / 2]; 00352 } 00353 00354 /* 00355 * Check the given key's parity, returns 1 on failure, 0 on SUCCESS 00356 */ 00357 int mbedtls_des_key_check_key_parity( const unsigned char key[MBEDTLS_DES_KEY_SIZE] ) 00358 { 00359 int i; 00360 00361 for( i = 0; i < MBEDTLS_DES_KEY_SIZE; i++ ) 00362 if( key[i] != odd_parity_table[key[i] / 2] ) 00363 return( 1 ); 00364 00365 return( 0 ); 00366 } 00367 00368 /* 00369 * Table of weak and semi-weak keys 00370 * 00371 * Source: http://en.wikipedia.org/wiki/Weak_key 00372 * 00373 * Weak: 00374 * Alternating ones + zeros (0x0101010101010101) 00375 * Alternating 'F' + 'E' (0xFEFEFEFEFEFEFEFE) 00376 * '0xE0E0E0E0F1F1F1F1' 00377 * '0x1F1F1F1F0E0E0E0E' 00378 * 00379 * Semi-weak: 00380 * 0x011F011F010E010E and 0x1F011F010E010E01 00381 * 0x01E001E001F101F1 and 0xE001E001F101F101 00382 * 0x01FE01FE01FE01FE and 0xFE01FE01FE01FE01 00383 * 0x1FE01FE00EF10EF1 and 0xE01FE01FF10EF10E 00384 * 0x1FFE1FFE0EFE0EFE and 0xFE1FFE1FFE0EFE0E 00385 * 0xE0FEE0FEF1FEF1FE and 0xFEE0FEE0FEF1FEF1 00386 * 00387 */ 00388 00389 #define WEAK_KEY_COUNT 16 00390 00391 static const unsigned char weak_key_table[WEAK_KEY_COUNT][MBEDTLS_DES_KEY_SIZE] = 00392 { 00393 { 0x01, 0x01, 0x01, 0x01, 0x01, 0x01, 0x01, 0x01 }, 00394 { 0xFE, 0xFE, 0xFE, 0xFE, 0xFE, 0xFE, 0xFE, 0xFE }, 00395 { 0x1F, 0x1F, 0x1F, 0x1F, 0x0E, 0x0E, 0x0E, 0x0E }, 00396 { 0xE0, 0xE0, 0xE0, 0xE0, 0xF1, 0xF1, 0xF1, 0xF1 }, 00397 00398 { 0x01, 0x1F, 0x01, 0x1F, 0x01, 0x0E, 0x01, 0x0E }, 00399 { 0x1F, 0x01, 0x1F, 0x01, 0x0E, 0x01, 0x0E, 0x01 }, 00400 { 0x01, 0xE0, 0x01, 0xE0, 0x01, 0xF1, 0x01, 0xF1 }, 00401 { 0xE0, 0x01, 0xE0, 0x01, 0xF1, 0x01, 0xF1, 0x01 }, 00402 { 0x01, 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x01, 0xFE }, 00403 { 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x01, 0xFE, 0x01 }, 00404 { 0x1F, 0xE0, 0x1F, 0xE0, 0x0E, 0xF1, 0x0E, 0xF1 }, 00405 { 0xE0, 0x1F, 0xE0, 0x1F, 0xF1, 0x0E, 0xF1, 0x0E }, 00406 { 0x1F, 0xFE, 0x1F, 0xFE, 0x0E, 0xFE, 0x0E, 0xFE }, 00407 { 0xFE, 0x1F, 0xFE, 0x1F, 0xFE, 0x0E, 0xFE, 0x0E }, 00408 { 0xE0, 0xFE, 0xE0, 0xFE, 0xF1, 0xFE, 0xF1, 0xFE }, 00409 { 0xFE, 0xE0, 0xFE, 0xE0, 0xFE, 0xF1, 0xFE, 0xF1 } 00410 }; 00411 00412 int mbedtls_des_key_check_weak( const unsigned char key[MBEDTLS_DES_KEY_SIZE] ) 00413 { 00414 int i; 00415 00416 for( i = 0; i < WEAK_KEY_COUNT; i++ ) 00417 if( memcmp( weak_key_table[i], key, MBEDTLS_DES_KEY_SIZE) == 0 ) 00418 return( 1 ); 00419 00420 return( 0 ); 00421 } 00422 00423 #if !defined(MBEDTLS_DES_SETKEY_ALT) 00424 void mbedtls_des_setkey( uint32_t SK[32], const unsigned char key[MBEDTLS_DES_KEY_SIZE] ) 00425 { 00426 int i; 00427 uint32_t X, Y, T; 00428 00429 GET_UINT32_BE( X, key, 0 ); 00430 GET_UINT32_BE( Y, key, 4 ); 00431 00432 /* 00433 * Permuted Choice 1 00434 */ 00435 T = ((Y >> 4) ^ X) & 0x0F0F0F0F; X ^= T; Y ^= (T << 4); 00436 T = ((Y ) ^ X) & 0x10101010; X ^= T; Y ^= (T ); 00437 00438 X = (LHs[ (X ) & 0xF] << 3) | (LHs[ (X >> 8) & 0xF ] << 2) 00439 | (LHs[ (X >> 16) & 0xF] << 1) | (LHs[ (X >> 24) & 0xF ] ) 00440 | (LHs[ (X >> 5) & 0xF] << 7) | (LHs[ (X >> 13) & 0xF ] << 6) 00441 | (LHs[ (X >> 21) & 0xF] << 5) | (LHs[ (X >> 29) & 0xF ] << 4); 00442 00443 Y = (RHs[ (Y >> 1) & 0xF] << 3) | (RHs[ (Y >> 9) & 0xF ] << 2) 00444 | (RHs[ (Y >> 17) & 0xF] << 1) | (RHs[ (Y >> 25) & 0xF ] ) 00445 | (RHs[ (Y >> 4) & 0xF] << 7) | (RHs[ (Y >> 12) & 0xF ] << 6) 00446 | (RHs[ (Y >> 20) & 0xF] << 5) | (RHs[ (Y >> 28) & 0xF ] << 4); 00447 00448 X &= 0x0FFFFFFF; 00449 Y &= 0x0FFFFFFF; 00450 00451 /* 00452 * calculate subkeys 00453 */ 00454 for( i = 0; i < 16; i++ ) 00455 { 00456 if( i < 2 || i == 8 || i == 15 ) 00457 { 00458 X = ((X << 1) | (X >> 27)) & 0x0FFFFFFF; 00459 Y = ((Y << 1) | (Y >> 27)) & 0x0FFFFFFF; 00460 } 00461 else 00462 { 00463 X = ((X << 2) | (X >> 26)) & 0x0FFFFFFF; 00464 Y = ((Y << 2) | (Y >> 26)) & 0x0FFFFFFF; 00465 } 00466 00467 *SK++ = ((X << 4) & 0x24000000) | ((X << 28) & 0x10000000) 00468 | ((X << 14) & 0x08000000) | ((X << 18) & 0x02080000) 00469 | ((X << 6) & 0x01000000) | ((X << 9) & 0x00200000) 00470 | ((X >> 1) & 0x00100000) | ((X << 10) & 0x00040000) 00471 | ((X << 2) & 0x00020000) | ((X >> 10) & 0x00010000) 00472 | ((Y >> 13) & 0x00002000) | ((Y >> 4) & 0x00001000) 00473 | ((Y << 6) & 0x00000800) | ((Y >> 1) & 0x00000400) 00474 | ((Y >> 14) & 0x00000200) | ((Y ) & 0x00000100) 00475 | ((Y >> 5) & 0x00000020) | ((Y >> 10) & 0x00000010) 00476 | ((Y >> 3) & 0x00000008) | ((Y >> 18) & 0x00000004) 00477 | ((Y >> 26) & 0x00000002) | ((Y >> 24) & 0x00000001); 00478 00479 *SK++ = ((X << 15) & 0x20000000) | ((X << 17) & 0x10000000) 00480 | ((X << 10) & 0x08000000) | ((X << 22) & 0x04000000) 00481 | ((X >> 2) & 0x02000000) | ((X << 1) & 0x01000000) 00482 | ((X << 16) & 0x00200000) | ((X << 11) & 0x00100000) 00483 | ((X << 3) & 0x00080000) | ((X >> 6) & 0x00040000) 00484 | ((X << 15) & 0x00020000) | ((X >> 4) & 0x00010000) 00485 | ((Y >> 2) & 0x00002000) | ((Y << 8) & 0x00001000) 00486 | ((Y >> 14) & 0x00000808) | ((Y >> 9) & 0x00000400) 00487 | ((Y ) & 0x00000200) | ((Y << 7) & 0x00000100) 00488 | ((Y >> 7) & 0x00000020) | ((Y >> 3) & 0x00000011) 00489 | ((Y << 2) & 0x00000004) | ((Y >> 21) & 0x00000002); 00490 } 00491 } 00492 #endif /* !MBEDTLS_DES_SETKEY_ALT */ 00493 00494 /* 00495 * DES key schedule (56-bit, encryption) 00496 */ 00497 int mbedtls_des_setkey_enc( mbedtls_des_context *ctx, const unsigned char key[MBEDTLS_DES_KEY_SIZE] ) 00498 { 00499 mbedtls_des_setkey( ctx->sk , key ); 00500 00501 return( 0 ); 00502 } 00503 00504 /* 00505 * DES key schedule (56-bit, decryption) 00506 */ 00507 int mbedtls_des_setkey_dec( mbedtls_des_context *ctx, const unsigned char key[MBEDTLS_DES_KEY_SIZE] ) 00508 { 00509 int i; 00510 00511 mbedtls_des_setkey( ctx->sk , key ); 00512 00513 for( i = 0; i < 16; i += 2 ) 00514 { 00515 SWAP( ctx->sk [i ], ctx->sk [30 - i] ); 00516 SWAP( ctx->sk [i + 1], ctx->sk [31 - i] ); 00517 } 00518 00519 return( 0 ); 00520 } 00521 00522 static void des3_set2key( uint32_t esk[96], 00523 uint32_t dsk[96], 00524 const unsigned char key[MBEDTLS_DES_KEY_SIZE*2] ) 00525 { 00526 int i; 00527 00528 mbedtls_des_setkey( esk, key ); 00529 mbedtls_des_setkey( dsk + 32, key + 8 ); 00530 00531 for( i = 0; i < 32; i += 2 ) 00532 { 00533 dsk[i ] = esk[30 - i]; 00534 dsk[i + 1] = esk[31 - i]; 00535 00536 esk[i + 32] = dsk[62 - i]; 00537 esk[i + 33] = dsk[63 - i]; 00538 00539 esk[i + 64] = esk[i ]; 00540 esk[i + 65] = esk[i + 1]; 00541 00542 dsk[i + 64] = dsk[i ]; 00543 dsk[i + 65] = dsk[i + 1]; 00544 } 00545 } 00546 00547 /* 00548 * Triple-DES key schedule (112-bit, encryption) 00549 */ 00550 int mbedtls_des3_set2key_enc( mbedtls_des3_context *ctx, 00551 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 2] ) 00552 { 00553 uint32_t sk[96]; 00554 00555 des3_set2key( ctx->sk , sk, key ); 00556 mbedtls_zeroize( sk, sizeof( sk ) ); 00557 00558 return( 0 ); 00559 } 00560 00561 /* 00562 * Triple-DES key schedule (112-bit, decryption) 00563 */ 00564 int mbedtls_des3_set2key_dec( mbedtls_des3_context *ctx, 00565 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 2] ) 00566 { 00567 uint32_t sk[96]; 00568 00569 des3_set2key( sk, ctx->sk , key ); 00570 mbedtls_zeroize( sk, sizeof( sk ) ); 00571 00572 return( 0 ); 00573 } 00574 00575 static void des3_set3key( uint32_t esk[96], 00576 uint32_t dsk[96], 00577 const unsigned char key[24] ) 00578 { 00579 int i; 00580 00581 mbedtls_des_setkey( esk, key ); 00582 mbedtls_des_setkey( dsk + 32, key + 8 ); 00583 mbedtls_des_setkey( esk + 64, key + 16 ); 00584 00585 for( i = 0; i < 32; i += 2 ) 00586 { 00587 dsk[i ] = esk[94 - i]; 00588 dsk[i + 1] = esk[95 - i]; 00589 00590 esk[i + 32] = dsk[62 - i]; 00591 esk[i + 33] = dsk[63 - i]; 00592 00593 dsk[i + 64] = esk[30 - i]; 00594 dsk[i + 65] = esk[31 - i]; 00595 } 00596 } 00597 00598 /* 00599 * Triple-DES key schedule (168-bit, encryption) 00600 */ 00601 int mbedtls_des3_set3key_enc( mbedtls_des3_context *ctx, 00602 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 3] ) 00603 { 00604 uint32_t sk[96]; 00605 00606 des3_set3key( ctx->sk , sk, key ); 00607 mbedtls_zeroize( sk, sizeof( sk ) ); 00608 00609 return( 0 ); 00610 } 00611 00612 /* 00613 * Triple-DES key schedule (168-bit, decryption) 00614 */ 00615 int mbedtls_des3_set3key_dec( mbedtls_des3_context *ctx, 00616 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 3] ) 00617 { 00618 uint32_t sk[96]; 00619 00620 des3_set3key( sk, ctx->sk , key ); 00621 mbedtls_zeroize( sk, sizeof( sk ) ); 00622 00623 return( 0 ); 00624 } 00625 00626 /* 00627 * DES-ECB block encryption/decryption 00628 */ 00629 #if !defined(MBEDTLS_DES_CRYPT_ECB_ALT) 00630 int mbedtls_des_crypt_ecb( mbedtls_des_context *ctx, 00631 const unsigned char input[8], 00632 unsigned char output[8] ) 00633 { 00634 int i; 00635 uint32_t X, Y, T, *SK; 00636 00637 SK = ctx->sk ; 00638 00639 GET_UINT32_BE( X, input, 0 ); 00640 GET_UINT32_BE( Y, input, 4 ); 00641 00642 DES_IP( X, Y ); 00643 00644 for( i = 0; i < 8; i++ ) 00645 { 00646 DES_ROUND( Y, X ); 00647 DES_ROUND( X, Y ); 00648 } 00649 00650 DES_FP( Y, X ); 00651 00652 PUT_UINT32_BE( Y, output, 0 ); 00653 PUT_UINT32_BE( X, output, 4 ); 00654 00655 return( 0 ); 00656 } 00657 #endif /* !MBEDTLS_DES_CRYPT_ECB_ALT */ 00658 00659 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00660 /* 00661 * DES-CBC buffer encryption/decryption 00662 */ 00663 int mbedtls_des_crypt_cbc( mbedtls_des_context *ctx, 00664 int mode, 00665 size_t length, 00666 unsigned char iv[8], 00667 const unsigned char *input, 00668 unsigned char *output ) 00669 { 00670 int i; 00671 unsigned char temp[8]; 00672 00673 if( length % 8 ) 00674 return( MBEDTLS_ERR_DES_INVALID_INPUT_LENGTH ); 00675 00676 if( mode == MBEDTLS_DES_ENCRYPT ) 00677 { 00678 while( length > 0 ) 00679 { 00680 for( i = 0; i < 8; i++ ) 00681 output[i] = (unsigned char)( input[i] ^ iv[i] ); 00682 00683 mbedtls_des_crypt_ecb( ctx, output, output ); 00684 memcpy( iv, output, 8 ); 00685 00686 input += 8; 00687 output += 8; 00688 length -= 8; 00689 } 00690 } 00691 else /* MBEDTLS_DES_DECRYPT */ 00692 { 00693 while( length > 0 ) 00694 { 00695 memcpy( temp, input, 8 ); 00696 mbedtls_des_crypt_ecb( ctx, input, output ); 00697 00698 for( i = 0; i < 8; i++ ) 00699 output[i] = (unsigned char)( output[i] ^ iv[i] ); 00700 00701 memcpy( iv, temp, 8 ); 00702 00703 input += 8; 00704 output += 8; 00705 length -= 8; 00706 } 00707 } 00708 00709 return( 0 ); 00710 } 00711 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00712 00713 /* 00714 * 3DES-ECB block encryption/decryption 00715 */ 00716 #if !defined(MBEDTLS_DES3_CRYPT_ECB_ALT) 00717 int mbedtls_des3_crypt_ecb( mbedtls_des3_context *ctx, 00718 const unsigned char input[8], 00719 unsigned char output[8] ) 00720 { 00721 int i; 00722 uint32_t X, Y, T, *SK; 00723 00724 SK = ctx->sk ; 00725 00726 GET_UINT32_BE( X, input, 0 ); 00727 GET_UINT32_BE( Y, input, 4 ); 00728 00729 DES_IP( X, Y ); 00730 00731 for( i = 0; i < 8; i++ ) 00732 { 00733 DES_ROUND( Y, X ); 00734 DES_ROUND( X, Y ); 00735 } 00736 00737 for( i = 0; i < 8; i++ ) 00738 { 00739 DES_ROUND( X, Y ); 00740 DES_ROUND( Y, X ); 00741 } 00742 00743 for( i = 0; i < 8; i++ ) 00744 { 00745 DES_ROUND( Y, X ); 00746 DES_ROUND( X, Y ); 00747 } 00748 00749 DES_FP( Y, X ); 00750 00751 PUT_UINT32_BE( Y, output, 0 ); 00752 PUT_UINT32_BE( X, output, 4 ); 00753 00754 return( 0 ); 00755 } 00756 #endif /* !MBEDTLS_DES3_CRYPT_ECB_ALT */ 00757 00758 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00759 /* 00760 * 3DES-CBC buffer encryption/decryption 00761 */ 00762 int mbedtls_des3_crypt_cbc( mbedtls_des3_context *ctx, 00763 int mode, 00764 size_t length, 00765 unsigned char iv[8], 00766 const unsigned char *input, 00767 unsigned char *output ) 00768 { 00769 int i; 00770 unsigned char temp[8]; 00771 00772 if( length % 8 ) 00773 return( MBEDTLS_ERR_DES_INVALID_INPUT_LENGTH ); 00774 00775 if( mode == MBEDTLS_DES_ENCRYPT ) 00776 { 00777 while( length > 0 ) 00778 { 00779 for( i = 0; i < 8; i++ ) 00780 output[i] = (unsigned char)( input[i] ^ iv[i] ); 00781 00782 mbedtls_des3_crypt_ecb( ctx, output, output ); 00783 memcpy( iv, output, 8 ); 00784 00785 input += 8; 00786 output += 8; 00787 length -= 8; 00788 } 00789 } 00790 else /* MBEDTLS_DES_DECRYPT */ 00791 { 00792 while( length > 0 ) 00793 { 00794 memcpy( temp, input, 8 ); 00795 mbedtls_des3_crypt_ecb( ctx, input, output ); 00796 00797 for( i = 0; i < 8; i++ ) 00798 output[i] = (unsigned char)( output[i] ^ iv[i] ); 00799 00800 memcpy( iv, temp, 8 ); 00801 00802 input += 8; 00803 output += 8; 00804 length -= 8; 00805 } 00806 } 00807 00808 return( 0 ); 00809 } 00810 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00811 00812 #endif /* !MBEDTLS_DES_ALT */ 00813 00814 #if defined(MBEDTLS_SELF_TEST) 00815 /* 00816 * DES and 3DES test vectors from: 00817 * 00818 * http://csrc.nist.gov/groups/STM/cavp/documents/des/tripledes-vectors.zip 00819 */ 00820 static const unsigned char des3_test_keys[24] = 00821 { 00822 0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF, 00823 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF, 0x01, 00824 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF, 0x01, 0x23 00825 }; 00826 00827 static const unsigned char des3_test_buf[8] = 00828 { 00829 0x4E, 0x6F, 0x77, 0x20, 0x69, 0x73, 0x20, 0x74 00830 }; 00831 00832 static const unsigned char des3_test_ecb_dec[3][8] = 00833 { 00834 { 0xCD, 0xD6, 0x4F, 0x2F, 0x94, 0x27, 0xC1, 0x5D }, 00835 { 0x69, 0x96, 0xC8, 0xFA, 0x47, 0xA2, 0xAB, 0xEB }, 00836 { 0x83, 0x25, 0x39, 0x76, 0x44, 0x09, 0x1A, 0x0A } 00837 }; 00838 00839 static const unsigned char des3_test_ecb_enc[3][8] = 00840 { 00841 { 0x6A, 0x2A, 0x19, 0xF4, 0x1E, 0xCA, 0x85, 0x4B }, 00842 { 0x03, 0xE6, 0x9F, 0x5B, 0xFA, 0x58, 0xEB, 0x42 }, 00843 { 0xDD, 0x17, 0xE8, 0xB8, 0xB4, 0x37, 0xD2, 0x32 } 00844 }; 00845 00846 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00847 static const unsigned char des3_test_iv[8] = 00848 { 00849 0x12, 0x34, 0x56, 0x78, 0x90, 0xAB, 0xCD, 0xEF, 00850 }; 00851 00852 static const unsigned char des3_test_cbc_dec[3][8] = 00853 { 00854 { 0x12, 0x9F, 0x40, 0xB9, 0xD2, 0x00, 0x56, 0xB3 }, 00855 { 0x47, 0x0E, 0xFC, 0x9A, 0x6B, 0x8E, 0xE3, 0x93 }, 00856 { 0xC5, 0xCE, 0xCF, 0x63, 0xEC, 0xEC, 0x51, 0x4C } 00857 }; 00858 00859 static const unsigned char des3_test_cbc_enc[3][8] = 00860 { 00861 { 0x54, 0xF1, 0x5A, 0xF6, 0xEB, 0xE3, 0xA4, 0xB4 }, 00862 { 0x35, 0x76, 0x11, 0x56, 0x5F, 0xA1, 0x8E, 0x4D }, 00863 { 0xCB, 0x19, 0x1F, 0x85, 0xD1, 0xED, 0x84, 0x39 } 00864 }; 00865 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00866 00867 /* 00868 * Checkup routine 00869 */ 00870 int mbedtls_des_self_test( int verbose ) 00871 { 00872 int i, j, u, v, ret = 0; 00873 mbedtls_des_context ctx; 00874 mbedtls_des3_context ctx3; 00875 unsigned char buf[8]; 00876 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00877 unsigned char prv[8]; 00878 unsigned char iv[8]; 00879 #endif 00880 00881 mbedtls_des_init( &ctx ); 00882 mbedtls_des3_init( &ctx3 ); 00883 /* 00884 * ECB mode 00885 */ 00886 for( i = 0; i < 6; i++ ) 00887 { 00888 u = i >> 1; 00889 v = i & 1; 00890 00891 if( verbose != 0 ) 00892 mbedtls_printf( " DES%c-ECB-%3d (%s): ", 00893 ( u == 0 ) ? ' ' : '3', 56 + u * 56, 00894 ( v == MBEDTLS_DES_DECRYPT ) ? "dec" : "enc" ); 00895 00896 memcpy( buf, des3_test_buf, 8 ); 00897 00898 switch( i ) 00899 { 00900 case 0: 00901 mbedtls_des_setkey_dec( &ctx, des3_test_keys ); 00902 break; 00903 00904 case 1: 00905 mbedtls_des_setkey_enc( &ctx, des3_test_keys ); 00906 break; 00907 00908 case 2: 00909 mbedtls_des3_set2key_dec( &ctx3, des3_test_keys ); 00910 break; 00911 00912 case 3: 00913 mbedtls_des3_set2key_enc( &ctx3, des3_test_keys ); 00914 break; 00915 00916 case 4: 00917 mbedtls_des3_set3key_dec( &ctx3, des3_test_keys ); 00918 break; 00919 00920 case 5: 00921 mbedtls_des3_set3key_enc( &ctx3, des3_test_keys ); 00922 break; 00923 00924 default: 00925 return( 1 ); 00926 } 00927 00928 for( j = 0; j < 10000; j++ ) 00929 { 00930 if( u == 0 ) 00931 mbedtls_des_crypt_ecb( &ctx, buf, buf ); 00932 else 00933 mbedtls_des3_crypt_ecb( &ctx3, buf, buf ); 00934 } 00935 00936 if( ( v == MBEDTLS_DES_DECRYPT && 00937 memcmp( buf, des3_test_ecb_dec[u], 8 ) != 0 ) || 00938 ( v != MBEDTLS_DES_DECRYPT && 00939 memcmp( buf, des3_test_ecb_enc[u], 8 ) != 0 ) ) 00940 { 00941 if( verbose != 0 ) 00942 mbedtls_printf( "failed\n" ); 00943 00944 ret = 1; 00945 goto exit; 00946 } 00947 00948 if( verbose != 0 ) 00949 mbedtls_printf( "passed\n" ); 00950 } 00951 00952 if( verbose != 0 ) 00953 mbedtls_printf( "\n" ); 00954 00955 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00956 /* 00957 * CBC mode 00958 */ 00959 for( i = 0; i < 6; i++ ) 00960 { 00961 u = i >> 1; 00962 v = i & 1; 00963 00964 if( verbose != 0 ) 00965 mbedtls_printf( " DES%c-CBC-%3d (%s): ", 00966 ( u == 0 ) ? ' ' : '3', 56 + u * 56, 00967 ( v == MBEDTLS_DES_DECRYPT ) ? "dec" : "enc" ); 00968 00969 memcpy( iv, des3_test_iv, 8 ); 00970 memcpy( prv, des3_test_iv, 8 ); 00971 memcpy( buf, des3_test_buf, 8 ); 00972 00973 switch( i ) 00974 { 00975 case 0: 00976 mbedtls_des_setkey_dec( &ctx, des3_test_keys ); 00977 break; 00978 00979 case 1: 00980 mbedtls_des_setkey_enc( &ctx, des3_test_keys ); 00981 break; 00982 00983 case 2: 00984 mbedtls_des3_set2key_dec( &ctx3, des3_test_keys ); 00985 break; 00986 00987 case 3: 00988 mbedtls_des3_set2key_enc( &ctx3, des3_test_keys ); 00989 break; 00990 00991 case 4: 00992 mbedtls_des3_set3key_dec( &ctx3, des3_test_keys ); 00993 break; 00994 00995 case 5: 00996 mbedtls_des3_set3key_enc( &ctx3, des3_test_keys ); 00997 break; 00998 00999 default: 01000 return( 1 ); 01001 } 01002 01003 if( v == MBEDTLS_DES_DECRYPT ) 01004 { 01005 for( j = 0; j < 10000; j++ ) 01006 { 01007 if( u == 0 ) 01008 mbedtls_des_crypt_cbc( &ctx, v, 8, iv, buf, buf ); 01009 else 01010 mbedtls_des3_crypt_cbc( &ctx3, v, 8, iv, buf, buf ); 01011 } 01012 } 01013 else 01014 { 01015 for( j = 0; j < 10000; j++ ) 01016 { 01017 unsigned char tmp[8]; 01018 01019 if( u == 0 ) 01020 mbedtls_des_crypt_cbc( &ctx, v, 8, iv, buf, buf ); 01021 else 01022 mbedtls_des3_crypt_cbc( &ctx3, v, 8, iv, buf, buf ); 01023 01024 memcpy( tmp, prv, 8 ); 01025 memcpy( prv, buf, 8 ); 01026 memcpy( buf, tmp, 8 ); 01027 } 01028 01029 memcpy( buf, prv, 8 ); 01030 } 01031 01032 if( ( v == MBEDTLS_DES_DECRYPT && 01033 memcmp( buf, des3_test_cbc_dec[u], 8 ) != 0 ) || 01034 ( v != MBEDTLS_DES_DECRYPT && 01035 memcmp( buf, des3_test_cbc_enc[u], 8 ) != 0 ) ) 01036 { 01037 if( verbose != 0 ) 01038 mbedtls_printf( "failed\n" ); 01039 01040 ret = 1; 01041 goto exit; 01042 } 01043 01044 if( verbose != 0 ) 01045 mbedtls_printf( "passed\n" ); 01046 } 01047 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01048 01049 if( verbose != 0 ) 01050 mbedtls_printf( "\n" ); 01051 01052 exit: 01053 mbedtls_des_free( &ctx ); 01054 mbedtls_des3_free( &ctx3 ); 01055 01056 return( ret ); 01057 } 01058 01059 #endif /* MBEDTLS_SELF_TEST */ 01060 01061 #endif /* MBEDTLS_DES_C */
Generated on Sun Jul 17 2022 08:25:21 by
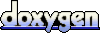