
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
configuration_store.h
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 */ 00018 00019 /** @file configuration_store.h 00020 * 00021 * This is the interface file to configuration store. 00022 * 00023 * The following (referred to as [REFERENCE_1] in other parts of the documentation) 00024 * discusses synchronous/asynchronous completion of \ref ARM_CFSTORE_DRIVER methods 00025 * and the inter-relationship of 00026 * \ref ARM_CFSTORE_OPCODE and \ref ARM_CFSTORE_CALLBACK. 00027 * 00028 * Configuration Store driver dispatch methods can operate in 2 modes: 00029 00030 * - Synchronous (i.e. when 00031 * \ref ARM_CFSTORE_CAPABILITIES ::asynchronous_ops == 0) 00032 * then the \ref ARM_CFSTORE_DRIVER ::Dispatch_Method_Xxx() will return: 00033 * - >= 0 => CFSTORE Dispatch_Method_Xxx() completed successfully 00034 * - otherwise CFSTORE Dispatch_Method_Xxx() did not complete successfully 00035 * (return code supplies further details). 00036 * 00037 * In synchronous mode it is optional as to whether the client registers 00038 * a client callback with the Initialize() method. If one is registered, it 00039 * will be called to indicate the synchronous completion status. 00040 * 00041 * - Asynchronous (i.e. when 00042 * \ref ARM_CFSTORE_CAPABILITIES ::asynchronous_ops == 1) 00043 * then the \ref ARM_CFSTORE_DRIVER ::Dispatch_Method_Xxx() will return: 00044 * - return_value = ARM_DRIVER_OK (i.e. ==0) implies CFSTORE 00045 * Dispatch_Method_Xxx() transaction pending. Dispatch_Method_Xxx() 00046 * completion status will be indicated via 00047 * an asynchronous call to \ref ARM_CFSTORE_CALLBACK registered with the 00048 * \ref ARM_CFSTORE_DRIVER ::(*Initialize)(), where the status supplied to 00049 * the callback will be the final status of the call. 00050 * - return_value > ARM_DRIVER_OK (i.e. > 0) implies CFSTORE Dispatch_Method_Xxx() completely 00051 * synchronously and successfully. The return_value has specific 00052 * meaning for the Dispatch_Method_Xxx() e.g. for the Read() method 00053 * return_value is the number of bytes read. 00054 * - otherwise return_value < 0 => CFSTORE Dispatch_Method_Xxx() 00055 * completed unsuccessfully (return code supplies further details). 00056 * 00057 * The client registered asynchronous callback method \ref ARM_CFSTORE_CALLBACK is 00058 * registered by the client using: 00059 * \ref ARM_CFSTORE_DRIVER ::(*Initialize)(\ref ARM_CFSTORE_CALLBACK callback, void* client_context) 00060 * See the (*Initialize) documentation for more details. 00061 * 00062 * The registered callback has the following prototype: 00063 * 00064 * typedef void (* \refARM_CFSTORE_CALLBACK)( 00065 * int32_t status, 00066 * \ref ARM_CFSTORE_OPCODE cmd_code, 00067 * void *client_context, 00068 * \ref ARM_CFSTORE_HANDLE handle); 00069 * 00070 * Before an asynchronous notification is received, a client can check on the 00071 * status of the call by calling \ref ARM_CFSTORE_DRIVER ::(*GetStatus)(). 00072 * 00073 */ 00074 00075 #ifndef __CONFIGURATION_STORE_H 00076 #define __CONFIGURATION_STORE_H 00077 00078 #ifdef __cplusplus 00079 extern "C" { 00080 #endif 00081 00082 #include <stdint.h> 00083 #include <string.h> /* requierd for memset() in ARM_CFSTORE_HANDLE_INIT() */ 00084 00085 #include "mbed_toolchain.h" /* required for MBED_DEPRECATED_SINCE */ 00086 00087 #define DEVICE_STORAGE 1 /* enable storage */ 00088 /// @cond CFSTORE_DOXYGEN_DISABLE 00089 #include <Driver_Storage.h> 00090 #include <Driver_Common.h> 00091 00092 #define ARM_CFSTORE_API_VERSION ARM_DRIVER_VERSION_MAJOR_MINOR(1,0) /* API version */ 00093 #define ARM_CFSTORE_DRV_VERSION ARM_DRIVER_VERSION_MAJOR_MINOR(0,1) /* DRV version */ 00094 00095 /* CFSTORE Specific error codes*/ 00096 #define ARM_CFSTORE_DRIVER_ERROR_UNINITIALISED -1000 /* Start of driver specific errors */ 00097 #define ARM_CFSTORE_DRIVER_ERROR_PREEXISTING_KEY -1001 00098 #define ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND -1002 00099 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_HANDLE -1003 00100 #define ARM_CFSTORE_DRIVER_ERROR_OUT_OF_MEMORY -1004 00101 #define ARM_CFSTORE_DRIVER_ERROR_PREEXISTING_KEY_DELETING -1005 00102 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_HANDLE_BUF -1006 00103 #define ARM_CFSTORE_DRIVER_ERROR_INTERNAL -1007 00104 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_KEY_NAME -1008 00105 #define ARM_CFSTORE_DRIVER_ERROR_VALUE_SIZE_TOO_LARGE -1009 00106 #define ARM_CFSTORE_DRIVER_ERROR_KEY_READ_ONLY -1010 00107 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_SEEK -1011 00108 #define ARM_CFSTORE_DRIVER_ERROR_KEY_UNREADABLE -1012 00109 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_WRITE_BUFFER -1013 00110 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_KEY_LEN -1014 00111 #define ARM_CFSTORE_DRIVER_ERROR_NOT_SUPPORTED -1015 00112 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_READ_BUFFER -1016 00113 #define ARM_CFSTORE_DRIVER_ERROR_INVALID_KEY_DESCRIPTOR -1017 00114 #define ARM_CFSTORE_DRIVER_ERROR_PERM_NO_READ_ACCESS -1018 00115 #define ARM_CFSTORE_DRIVER_ERROR_PERM_NO_WRITE_ACCESS -1019 00116 #define ARM_CFSTORE_DRIVER_ERROR_PERM_NO_EXECUTE_ACCESS -1020 00117 #define ARM_CFSTORE_DRIVER_ERROR_NO_PERMISSIONS -1021 00118 #define ARM_CFSTORE_DRIVER_ERROR_HANDLE_COUNT_MAX -1022 00119 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_ERROR -1023 00120 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_BUSY -1024 00121 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_TIMEOUT -1025 00122 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_UNSUPPORTED -1026 00123 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_PARAMETER -1027 00124 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_BOUNDED_CAPACITY -1028 00125 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_STORAGE_API_ERROR -1029 00126 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_STORAGE_IO_ERROR -1030 00127 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_NOT_INITIALIZED -1031 00128 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_ATTEMPTING_COMMIT_WITHOUT_WRITE -1032 00129 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_EMPTY -1033 00130 #define ARM_CFSTORE_DRIVER_ERROR_JOURNAL_STATUS_SMALL_LOG_REQUEST -1034 00131 #define ARM_CFSTORE_DRIVER_ERROR_OPERATION_PENDING -1035 00132 #define ARM_CFSTORE_DRIVER_ERROR_UVISOR_BOX_ID -1036 00133 #define ARM_CFSTORE_DRIVER_ERROR_UVISOR_NAMESPACE -1037 00134 /// @endcond 00135 00136 00137 /* Type Definitions */ 00138 typedef void *ARM_CFSTORE_HANDLE ; //!< opaque cfstore handle for manipulating cfstore data objects e.g. KV pairs. 00139 typedef size_t ARM_CFSTORE_SIZE; //!< CFSTORE type for size parameters. 00140 typedef size_t ARM_CFSTORE_OFFSET; //!< CFSTORE type for offset parameters. 00141 00142 /** @brief 00143 * 00144 * Status structure returned from the \ref ARM_CFSTORE_DRIVER (*GetStatus)() function. 00145 */ 00146 typedef struct _ARM_CFSTORE_STATUS { 00147 uint32_t in_progress : 1; //!< indicates a previous \ref ARM_CFSTORE_DRIVER ::Dispatch_Method_Xxx() 00148 //!< function is still in progress and will complete sometime in the future. 00149 uint32_t error : 1; //!< indicates a previous \ref ARM_CFSTORE_DRIVER ::Dispatch_Method_Xxx() 00150 //!< function is errored and will complete sometime in the future. 00151 } ARM_CFSTORE_STATUS; 00152 00153 /* Defines */ 00154 #define CFSTORE_KEY_NAME_MAX_LENGTH 220 //!< The maximum length of the null terminated character 00155 //!< string used as a key name string. 00156 #define CFSTORE_VALUE_SIZE_MAX (1<<26) //!< Max size of the KV value blob (currently 64MB) 00157 #define CFSTORE_HANDLE_BUFSIZE 24 //!< size of the buffer owned and supplied by client 00158 //!< to CFSTORE to hold internal data structures, referenced by the key handle. 00159 00160 /** @brief Helper macro to declare handle and client owned buffer supplied 00161 * to CFSTORE for storing opaque handle state 00162 */ 00163 #define ARM_CFSTORE_HANDLE_INIT(__name) \ 00164 uint8_t (__name##_buf_cFsToRe)[CFSTORE_HANDLE_BUFSIZE]; \ 00165 ARM_CFSTORE_HANDLE (__name) = (ARM_CFSTORE_HANDLE) (__name##_buf_cFsToRe); \ 00166 memset((__name##_buf_cFsToRe), 0, CFSTORE_HANDLE_BUFSIZE) 00167 00168 #if defined __MBED__ && (defined TOOLCHAIN_GCC_ARM || defined TOOLCHAIN_ARMC6) 00169 /** @brief Helper macro to swap 2 handles, which is useful for the Find() idiom. */ 00170 #define CFSTORE_HANDLE_SWAP(__a_HaNdLe, __b_HaNdLe) \ 00171 do{ ARM_CFSTORE_HANDLE __temp_HaNdLe = (__a_HaNdLe); \ 00172 __asm volatile("" ::: "memory"); \ 00173 (__a_HaNdLe) = (__b_HaNdLe); \ 00174 __asm volatile("" ::: "memory"); \ 00175 (__b_HaNdLe) = (__temp_HaNdLe); \ 00176 __asm volatile("" ::: "memory"); \ 00177 }while(0) 00178 00179 #elif defined __MBED__ && defined TOOLCHAIN_ARM 00180 /** @brief Helper macro to swap 2 handles, which is useful for the Find() idiom. */ 00181 #define CFSTORE_HANDLE_SWAP(__a_HaNdLe, __b_HaNdLe) \ 00182 do{ ARM_CFSTORE_HANDLE __temp_HaNdLe = (__a_HaNdLe); \ 00183 __dmb(0xf); \ 00184 (__a_HaNdLe) = (__b_HaNdLe); \ 00185 __dmb(0xf); \ 00186 (__b_HaNdLe) = (__temp_HaNdLe); \ 00187 __dmb(0xf); \ 00188 }while(0) 00189 00190 #elif defined __MBED__ && defined TOOLCHAIN_IAR 00191 /** @brief Helper macro to swap 2 handles, which is useful for the Find() idiom. */ 00192 /* note, memory barriers may be required in the following implementation */ 00193 #define CFSTORE_HANDLE_SWAP(__a_HaNdLe, __b_HaNdLe) \ 00194 do{ ARM_CFSTORE_HANDLE __temp_HaNdLe = (__a_HaNdLe); \ 00195 (__a_HaNdLe) = (__b_HaNdLe); \ 00196 (__b_HaNdLe) = (__temp_HaNdLe); \ 00197 }while(0) 00198 #endif 00199 00200 /** @brief The access control permissions for the key-value. */ 00201 typedef struct ARM_CFSTORE_ACCESS_CONTROL_LIST 00202 { 00203 uint32_t perm_owner_read : 1; //!< When set this KV is owner readable 00204 uint32_t perm_owner_write : 1; //!< When set this KV is owner writable. The owner should set this bit to be able delete the KV. 00205 uint32_t perm_owner_execute : 1; //!< When set this KV is owner executable 00206 uint32_t perm_other_read : 1; //!< When set this KV is world readable 00207 uint32_t perm_other_write : 1; //!< When set this KV is world writable. If set then a world client can delete this KV. 00208 uint32_t perm_other_execute : 1; //!< When set this KV is world executable (currently not supported) 00209 uint32_t reserved : 26; //!< reserved for future use. 00210 } ARM_CFSTORE_ACCESS_CONTROL_LIST; 00211 00212 00213 /** @brief 00214 * 00215 * File mode bit-field structure for specifying flags for the 00216 * following operations: 00217 * - ARM_CFSTORE_DRIVER::(*Create)(), when creating a KV. 00218 * - ARM_CFSTORE_DRIVER::(*Open)(), when opening a pre-existing KV. 00219 */ 00220 typedef struct ARM_CFSTORE_FMODE 00221 { 00222 uint32_t continuous : 1; //!< If set, the key value should be stored in a continuous sequence 00223 //!< of hardware addresses (not implemented). 00224 uint32_t lazy_flush : 1; //!< If set then configuration store will defer flushing the KV 00225 //!< changes until an optimal time. e.g. to save energy rather than 00226 //!< performing the operation immediately (not implemented). 00227 uint32_t flush_on_close : 1; //!< If set then the key-value should be flushed to the backing store 00228 //!< when the key is closed (not implemented). 00229 uint32_t read : 1; //!< If set then the KV can be read. 00230 uint32_t write : 1; //!< If set then the KV can be written. 00231 uint32_t execute : 1; //!< If set then the KV can be executed (not implemented). 00232 uint32_t storage_detect : 1; //!< If set then the call to ARM_CFSTORE_DRIVER::(*Create)() returns 00233 //!< whether a key could be created with the required storage 00234 //!< characteristics. The key is not created. 00235 uint32_t reserved : 25; //!< Reserved. 00236 } ARM_CFSTORE_FMODE; 00237 00238 00239 /** @brief Descriptor used to create keys */ 00240 typedef struct ARM_CFSTORE_KEYDESC 00241 { 00242 /*key descriptor attributes */ 00243 ARM_CFSTORE_ACCESS_CONTROL_LIST acl; //!< Access Control List specifying the access permissions of the KV. 00244 uint8_t drl; //!< Data retention level for the KV required by the client. 00245 //!< CFSTORE will store the KV in the specified type store/media. 00246 ARM_STORAGE_SECURITY_FEATURES security; //!< flash security properties for the KV required by the client. 00247 //!< CFSTORE will store the KV in a storage media supporting 00248 //!< the specified security attributes. 00249 ARM_CFSTORE_FMODE flags; //!< A bitfield containing the access mode setting for the key. 00250 } ARM_CFSTORE_KEYDESC; 00251 00252 00253 /** @brief 00254 * 00255 * These are the command code values used for the cmd_code argument to the 00256 * \ref ARM_CFSTORE_CALLBACK callback handler. The command code indicates the 00257 * \ref ARM_CFSTORE_DRIVER dispatch method previously invocated. 00258 */ 00259 typedef enum ARM_CFSTORE_OPCODE { 00260 CFSTORE_OPCODE_CLOSE = 1, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Close)() call. 00261 CFSTORE_OPCODE_CREATE, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Create)() call. 00262 CFSTORE_OPCODE_DELETE, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Delete)() call. 00263 CFSTORE_OPCODE_FIND, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Find)() call. 00264 CFSTORE_OPCODE_FLUSH, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Flush)() call. 00265 CFSTORE_OPCODE_GET_KEY_NAME, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*GetKeyName)() call. 00266 CFSTORE_OPCODE_GET_STATUS, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*GetStatus)() call. 00267 CFSTORE_OPCODE_GET_VALUE_LEN, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*GetValueLen)() call. 00268 CFSTORE_OPCODE_INITIALIZE, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Initialize)() call. 00269 CFSTORE_OPCODE_OPEN, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Open)() call. 00270 CFSTORE_OPCODE_POWER_CONTROL, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*PowerControl)() call. 00271 CFSTORE_OPCODE_READ, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Read)() call. 00272 CFSTORE_OPCODE_RSEEK, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Rseek)() call. 00273 CFSTORE_OPCODE_UNINITIALIZE, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Uninitialize)() call. 00274 CFSTORE_OPCODE_WRITE, //!< used for \ref ARM_CFSTORE_CALLBACK ::cmd_code argument when indicating status for a previous \ref ARM_CFSTORE_DRIVER ::(*Write)() call. 00275 CFSTORE_OPCODE_MAX //!< Sentinel 00276 } ARM_CFSTORE_OPCODE; 00277 00278 00279 /** @brief client registered callback type for receiving asynchronous event 00280 * notifications. 00281 * 00282 * @param status 00283 * In the case that cmd_code is one of ARM_CFSTORE_OPCODE then this 00284 * is the asynchronous completion status of the associated 00285 * ARM_CFSTORE_DRIVER::Dispatch_Method_Xxx() 00286 * @param cmd_code 00287 * ARM_CFSTORE_OPCODE for the 00288 * ARM_CFSTORE_DRIVER::Dispatch_Method_Xxx() for which this 00289 * invocation of the callback is an asynchronous completion event. 00290 * @param client_context 00291 * The client ARM_CFSTORE_CALLBACK context registered with the 00292 * ARM_CFSTORE_DRIVER::(*Initialize)() method. 00293 * @param handle 00294 * For certain ARM_CFSTORE_DRIVER::Dispatch_Method_Xxx() e.g. 00295 * ARM_CFSTORE_DRIVER::(*Create)() 00296 * ARM_CFSTORE_DRIVER::(*Open)() 00297 * ARM_CFSTORE_DRIVER::(*Find)() 00298 * then an open key handle is supplied upon asynchronous completion. 00299 * See the documentation of the ARM_CFSTORE_OpCode_e and the 00300 * ARM_CFSTORE_DRIVER::Dispatch_Method_Xxx() for further details. 00301 * 00302 */ 00303 typedef void (*ARM_CFSTORE_CALLBACK)(int32_t status, ARM_CFSTORE_OPCODE cmd_code, void *client_context, ARM_CFSTORE_HANDLE handle); 00304 00305 00306 /** @brief Find the capabilities of the configuration store. */ 00307 typedef struct ARM_CFSTORE_CAPABILITIES 00308 { 00309 uint32_t asynchronous_ops : 1; //!< When set then the configuration store dispatch interface is operating in non-blocking (asynchronous) mode. 00310 //!< When unset then the configuration store dispatch interface is operating in blocking (synchronous) mode. 00311 uint32_t uvisor_support_enabled : 1; //!< The configuration store is using uvisor security contexts. 00312 } ARM_CFSTORE_CAPABILITIES; 00313 00314 00315 /** 00316 * This is the set of operations constituting the Configuration Store driver. 00317 * 00318 * The functions dispatch methods can operate synchronously or asynchronously 00319 * depending on the underlying implementation. The client of this interface 00320 * should not assume one or other mode of operation but use the 00321 * (*GetCapabilities) method to determine the operational mode and then 00322 * behave accordingly. 00323 */ 00324 typedef struct _ARM_DRIVER_CFSTORE 00325 { 00326 /** @brief Get driver version. 00327 * 00328 * The synchronous function GetVersion() returns version information of the 00329 * driver implementation in ARM_DRIVER_VERSION. 00330 * - ARM_DRIVER_VERSION::api 00331 * the version of the CMSIS-Driver specification used to implement 00332 * this driver. 00333 * - ARM_DRIVER_VERSION::drv 00334 * the source code version of the actual configuration store driver 00335 * implementation. 00336 * 00337 * @return \ref ARM_DRIVER_VERSION, the configuration store driver 00338 * version information 00339 */ 00340 ARM_DRIVER_VERSION (*GetVersion)(void); 00341 00342 00343 /** @brief Close the hkey context previously recovered from CFSTORE. 00344 * 00345 * @param hkey 00346 * IN: a previously returned handle from (*Create((), (*Open)() 00347 * or (*Find)() to close. 00348 * 00349 * @return 00350 * 00351 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00352 * ARM_CFSTORE_DRIVER::(*Close)() asynchronous completion command code 00353 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00354 * @param status 00355 * ARM_DRIVER_OK => success, KV deleted, else failure. 00356 * @param cmd_code == CFSTORE_OPCODE_CLOSE 00357 * @param client context 00358 * as registered with ARM_CFSTORE_DRIVER::(*Initialize)() 00359 * @param hkey == NULL 00360 */ 00361 int32_t (*Close)(ARM_CFSTORE_HANDLE hkey); 00362 00363 00364 /** @brief Create a key-value int he configuration strore. 00365 * 00366 * Once created, the following attributes of a KV cannot be changed: 00367 * - the key_name 00368 * - the permissions or attributes specifies by the key descriptor kdesc. 00369 * To change these properties, the key must be deleted and then created 00370 * again with the new properties. 00371 * 00372 * @param key_name 00373 * IN: zero terminated string specifying the key name. 00374 * @param value_len 00375 * the client specifies the length of the value data item in the 00376 * KV being created. 00377 * @param kdesc 00378 * 00379 * IN: key descriptor, specifying how the details of the key 00380 * create operations. Note the following cases: 00381 * - 1) if key_name is not present in the CFSTORE and kdesc is NULL 00382 * (i.e. a new KV pair is being created), 00383 * then CFSTORE will chose the most appropriate defaults 00384 * e.g. CFSTORE will store the KV in nv store if the value size 00385 * is large, with no security guarantees just safety. 00386 * - 2) if key_name is present in the CFSTORE and kdesc is NULL 00387 * (i.e. there is a pre-existing KV pair), 00388 * then CFSTORE will grow/shrink the value data buffer to 00389 * value_len octets. This idiom can be used to increase/decrease 00390 * the size of the KV value storage. 00391 * - 3) If the kdesc->flags.storage_detect == 1 then the function 00392 * does not create the key but reports whether the key 00393 * with the specified size and storage media attributes 00394 * could/could not be created by configuration storage. 00395 * 00396 * @param hkey 00397 * IN: pointer to client owned buffer of CFSTORE_HANDLE_BUFSIZE 00398 * bytes 00399 * OUT: on success, hkey is a valid handle to a KV. 00400 * 00401 * @return 00402 * 00403 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00404 * ARM_CFSTORE_DRIVER::(*Create)() asynchronous completion command code 00405 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00406 * @param status 00407 * ARM_DRIVER_OK => success, KV deleted, else failure. 00408 * @param cmd_code == CFSTORE_OPCODE_CREATE 00409 * @param client context 00410 * as registered with ARM_CFSTORE_DRIVER::(*Initialize)() 00411 * @param hkey now contains returned handle to newly created key. 00412 */ 00413 int32_t (*Create)(const char* key_name, ARM_CFSTORE_SIZE value_len, const ARM_CFSTORE_KEYDESC* kdesc, ARM_CFSTORE_HANDLE hkey); 00414 00415 00416 /** @brief Delete key-value from configuration store 00417 * 00418 * This method is used in the following ways: 00419 * - (*Open)() to get a handle to the key, (*Delete)() to delete the key, 00420 * (*Close)() to close the handle to the key. 00421 * - (*Find)() to get a handle to the key, (*Delete)() to delete the key, 00422 * (*Close)() to close the handle to the key. 00423 * 00424 * @param hkey 00425 * IN: the handle of the key to delete. 00426 * 00427 * @return 00428 * 00429 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00430 * ARM_CFSTORE_DRIVER::(*Delete)() asynchronous completion command code 00431 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00432 * @param status 00433 * ARM_DRIVER_OK => success, KV deleted, else failure. 00434 * @param cmd_code == CFSTORE_OPCODE_DELETE 00435 * @param client context 00436 * as registered with ARM_CFSTORE_DRIVER::(*Initialize)() 00437 * @param hkey, unused. 00438 * 00439 */ 00440 int32_t (*Delete)(ARM_CFSTORE_HANDLE hkey); 00441 00442 00443 /** @brief find stored keys that match a query string 00444 * 00445 * find a list of pre-existing keys according to a query pattern. 00446 * The search pattern can have the following formats 00447 * - 'com.arm.mbed.wifi.accesspoint.essid'. Find whether an object 00448 * exists that has a key matching 00449 * 'com.arm.mbed.wifi.accesspoint.essid' 00450 * - 'com.arm.mbed.wifi.accesspoint*.essid'. Find all keys that 00451 * start with the substring 'com.arm.mbed.wifi.accesspoint' and 00452 * end with the substring '.essid' 00453 * - 'com.arm.mbed.your-registry-module-name.*'. Find all key_name 00454 * strings that begin with the substring 00455 * 'com.arm.mbed.your-registry-module-name.' 00456 * - 'com.arm.mbed.hello-world.animal{dog}{foot}{*}'. Find all key_name 00457 * strings beginning com.arm.mbed.hello-world.animal{dog}{foot} 00458 * - 'com.arm.mbed.hello-world.animal{dog}{foot}*' 00459 * - 'com.arm.mbed.hello-world.animal{dog*3}' 00460 * 00461 * It is possible to iterate over the list of matching keys by 00462 * using the idiom below (in the synchronous case): 00463 * ``` 00464 * int32_t ret = ARM_DRIVER_ERROR; 00465 * const char* key_name_query = "*"; 00466 * char key_name[CFSTORE_KEY_NAME_MAX_LENGTH+1]; 00467 * uint8_t len = CFSTORE_KEY_NAME_MAX_LENGTH+1; 00468 * ARM_CFSTORE_HANDLE_INIT(next); 00469 * ARM_CFSTORE_HANDLE_INIT(prev); 00470 * 00471 * while(drv->Find(key_name_query, prev, next) == ARM_DRIVER_OK) 00472 * { 00473 * len = CFSTORE_KEY_NAME_MAX_LENGTH+1; 00474 * drv->GetKeyName(next, key_name, &len); 00475 * printf("Found matching key-value with key_name=%s\n", key_name); 00476 * CFSTORE_HANDLE_SWAP(prev, next); 00477 * } 00478 * ``` 00479 * The iteration over the find results can be terminated before 00480 * the end of the list is reached by closing the last open 00481 * file handle and not making any further calls to 00482 * find() e.g. 00483 * 00484 * ``` 00485 * int32_t ret = ARM_DRIVER_ERROR; 00486 * const char* key_name_query = "*"; 00487 * char key_name[CFSTORE_KEY_NAME_MAX_LENGTH+1]; 00488 * uint8_t len = CFSTORE_KEY_NAME_MAX_LENGTH+1; 00489 * ARM_CFSTORE_HANDLE_INIT(next); 00490 * ARM_CFSTORE_HANDLE_INIT(prev); 00491 * 00492 * while(drv->Find(key_name_query, prev, next) == ARM_DRIVER_OK) 00493 * { 00494 * len = CFSTORE_KEY_NAME_MAX_LENGTH+1; 00495 * drv->GetKeyName(next, key_name, &len); 00496 * if(strncmp(key_name, "my.key_name", CFSTORE_KEY_NAME_MAX_LENGTH)) 00497 * { 00498 * printf("Found matching key-value with key_name=%s\n", key_name; 00499 * // terminating walk of find results 00500 * drv->Close(next); 00501 * break; 00502 * CFSTORE_HANDLE_SWAP(prev, next); 00503 * } 00504 * ``` 00505 * 00506 * @param key_name_query 00507 * IN: a search string to find. This can include the wildcard '*' 00508 * character 00509 * @param previous 00510 * IN: On the first call to (*Find) then previous is a pointer 00511 * (the handle) to a buffer initialised to 0. 00512 * On the subsequent calls to (*Find), a previously returned 00513 * key handle can be supplied as the previous argument. The next 00514 * available search result will be returned. If no further search 00515 * results are available then (*Find) will return 00516 * ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND. Once the 00517 * file handle has been supplied to the function as the prev 00518 * argument, CFSTORE close the open file handle. Otherwise, the 00519 * client must call (*Close)() on the open file handle. 00520 * @param next 00521 * IN: pointer to client owned buffer of CFSTORE_HANDLE_BUFSIZE 00522 * bytes to hold the 00523 * OUT: Success: In the case that a match has been found then hkey 00524 * is a valid handle to an open KV. The key must be explicitly closed 00525 * by the client. Note this is a read-only key. 00526 * Async operation: The storage at hkey must be available until after 00527 * the completion notification has been received by the client. 00528 * @return 00529 * On success (finding a KV matching the query) ARM_DRIVER_OK is 00530 * returned. If a KV is not found matching the description then 00531 * ARM_CFSTORE_DRIVER_ERROR_KEY_NOT_FOUND is returned. 00532 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00533 * ARM_CFSTORE_DRIVER::(*Find)() asynchronous completion command code 00534 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00535 * @param status 00536 * ARM_DRIVER_OK => success, hkey contains open key 00537 * else failure. 00538 * @param cmd_code == CFSTORE_OPCODE_FIND 00539 * @param client context 00540 * as registered with ARM_CFSTORE_DRIVER::(*Initialize)() 00541 * @param hkey 00542 * ARM_DRIVER_OK => contains returned handle to newly found key. 00543 * else, indeterminate data. 00544 */ 00545 int32_t (*Find)(const char* key_name_query, const ARM_CFSTORE_HANDLE previous, ARM_CFSTORE_HANDLE next); 00546 00547 00548 /** @brief 00549 * 00550 * Flush (write) the configuration changes to the nv backing store. 00551 * 00552 * All open key handles must be closed before flushing the CFSTORE to nv 00553 * backing store. 00554 * 00555 * @return 00556 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00557 * ARM_CFSTORE_DRIVER::(*Flush)() asynchronous completion command code 00558 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00559 * @param status 00560 * ARM_DRIVER_OK => success, hkey contains open key 00561 * else failure. 00562 * @param cmd_code == CFSTORE_OPCODE_FLUSH 00563 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00564 * @param hkey, unused 00565 */ 00566 int32_t (*Flush)(void); 00567 00568 00569 /** @brief Get configuration store capabilities. 00570 * 00571 * This synchronous function returns a ARM_CFSTORE_CAPABILITIES 00572 * information structure describing configuration store implementation 00573 * attributes. 00574 * 00575 * @return \ref ARM_CFSTORE_CAPABILITIES 00576 */ 00577 ARM_CFSTORE_CAPABILITIES (*GetCapabilities)(void); 00578 00579 00580 /** @brief Get the name of an open key handle. 00581 * 00582 * @param hkey 00583 * open handle of key to get the key_name 00584 * @param key_name. The key name string is guaranteed to be terminated 00585 * with '\0'. 00586 * @param key_len, the length of the buffer available at data to receive 00587 * the key_name string 00588 * @return 00589 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00590 * ARM_CFSTORE_DRIVER::(*GetKeyName)() asynchronous completion command code 00591 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00592 * @param status 00593 * ARM_DRIVER_OK => success, key_name contains key_name string, len 00594 * contains length of string. 00595 * else failure. 00596 * @param cmd_code == CFSTORE_OPCODE_GET_KEY_NAME 00597 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00598 * @param hkey supplied to the GetKeyName() call at hkey. 00599 * @param key_name 00600 * on success, the buffer holds the name of the key 00601 * @param key_len 00602 * on success, the supplied integer is set to strlen(key_name)+1, where 00603 * the additional character corresponds to the terminating null. 00604 * 00605 */ 00606 int32_t (*GetKeyName)(ARM_CFSTORE_HANDLE hkey, char* key_name, uint8_t *key_len); 00607 00608 00609 /** @brief Get the status of the configuration store. 00610 * 00611 * @return 00612 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation 00613 * ARM_DRIVER_OK if the command has been accepted and 00614 * enqueued by the underlying controller, else an appropriate 00615 * error code. Getting a return value of ARM_DRIVER_OK 00616 * implies that basic argument validation was successful, and the 00617 * caller can expect a command completion callback to be invoked 00618 * at a later time. 00619 * 00620 * ARM_CFSTORE_DRIVER::(*GetStatus)() asynchronous completion command code 00621 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00622 * @param status 00623 * status of next command pending completion 00624 * @param cmd_code 00625 * next command pending completion. 00626 * @param client context registered ARM_CFSTORE_DRIVER::(*Initialize)() 00627 * @param hkey 00628 * unused. 00629 */ 00630 ARM_CFSTORE_STATUS (*GetStatus)(void); 00631 00632 00633 /** @brief Get the value length from an open key handle 00634 * 00635 * @param hkey 00636 * open handle of key for which to get value length 00637 * @param value_len, the location in which to store the value length. 00638 * @return 00639 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00640 * ARM_CFSTORE_DRIVER::(*GetValueLen)() asynchronous completion command code 00641 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00642 * @param status 00643 * ARM_DRIVER_OK => success, value_len contains length value. 00644 * else failure. 00645 * @param cmd_code == CFSTORE_OPCODE_GET_VALUE_LEN 00646 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00647 * @param hkey supplied to the GetValueLen() call at hkey. 00648 * 00649 */ 00650 int32_t (*GetValueLen)(ARM_CFSTORE_HANDLE hkey, ARM_CFSTORE_SIZE *value_len); 00651 00652 00653 /** @brief Initialize the Configuration Store 00654 * 00655 * This function: 00656 * - initialised the configuration store service 00657 * - allows the client to subscribe to event notifications, in particular 00658 * the asynchronous completion events for the driver dispatch interface 00659 * (see ARM_CFSTORE_OPCODE). 00660 * 00661 * The configuration store should be initialised as follows: 00662 * drv->initialise(client_callback, client_callback_context); 00663 * drv->PowerControl(ARM_POWER_FULL) 00664 * where: 00665 * - client_callback is a client implemented callback function. 00666 * - client_callback_context is a client registered context that 00667 * will be supplied as an argument to the client_callback 00668 * when called. 00669 * - PowerControl indicates that any underlying system services 00670 * that are in the low power state should be powered up. 00671 * 00672 * The configuration store should be de-initialised as follows: 00673 * drv->PowerControl(ARM_POWER_OFF) 00674 * drv->Deinitialise(); 00675 * 00676 * @param client_callback 00677 * this is a client implemented callback function for 00678 * receiving asynchronous event notifications (see 00679 * ARM_CFSTORE_CALLBACK). NULL indicates client does 00680 * not subscribed to event notifications. 00681 * 00682 * @param client_callback_context 00683 * this is the client registered context that 00684 * will be supplied as an argument to the client_callback 00685 * when called. This argument can be NULL. 00686 * 00687 * @return 00688 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00689 * ARM_CFSTORE_DRIVER::(*Initialize)() asynchronous completion command code 00690 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00691 * @param status 00692 * ARM_DRIVER_OK => success, configuration store initialised, 00693 * else failure 00694 * @param CFSTORE_OPCODE_INITIALIZE 00695 * @param client context registered ARM_CFSTORE_DRIVER::(*Initialize)() 00696 * @param hkey, unused. 00697 * 00698 */ 00699 int32_t (*Initialize)(ARM_CFSTORE_CALLBACK callback, void* client_context); 00700 00701 00702 /** @brief Function to set the target configuration store power state. 00703 * 00704 * @param state 00705 * \ref ARM_POWER_STATE. The target power-state for the 00706 * configuration store. The parameter state can have the 00707 * following values: 00708 * - ARM_POWER_FULL => set the mode to full power state 00709 * - ARM_POWER_LOW => set the mode to low power state 00710 * - ARM_POWER_OFF => set the mode to off power state 00711 * 00712 * @return 00713 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00714 * CFSTORE_OPCODE_POWER_CONTROL 00715 * ARM_CFSTORE_DRIVER::(*PowerControl)() asynchronous completion command code 00716 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00717 * @param status 00718 * ARM_DRIVER_OK => success, power control set, else failure. 00719 * @param cmd_code == CFSTORE_OPCODE_POWER_CONTROL 00720 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00721 * @param hkey, unused. 00722 */ 00723 int32_t (*PowerControl)(ARM_POWER_STATE state); 00724 00725 00726 /** @brief Read the value data associated with a specified key. 00727 * 00728 * @param hkey 00729 * IN: the handle returned from a previous call to (*Open)() to 00730 * get a handle to the key 00731 * @param data 00732 * IN: a pointer to a data buffer supplied by the caller for CFSTORE to 00733 * fill with value data 00734 * OUT: on ARM_DRIVER_OK the data is (or will be when asynchronously 00735 * completed) available in the buffer. The data will be read from 00736 * the current form the current location (see (*Rseek)(). 00737 * @param len 00738 * IN: the client specifies the length of the buffer available at data 00739 * OUT: the CFSTORE specifies how many octets have been stored in the 00740 * supplied buffer. Note fewer octets may be stored than the input len 00741 * depending on the CFSTORE internal representation of the value. 00742 * 00743 * @return 00744 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00745 * return_value == ARM_DRIVER_OK (==0) => asynchronous 00746 * transaction pending. 00747 * return_value > 0 => synchronous completion of read with the 00748 * number of bytes read == return_value 00749 * return_value < 0, error condition. 00750 * 00751 * ARM_CFSTORE_DRIVER::(*Read)() asynchronous completion command code 00752 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00753 * @param status 00754 * >= 0 => success with number of bytes read as indicated by 00755 * the value of status 00756 * < 0 => error, data in the data buffer is undefined, len is 00757 * undefined. 00758 * @param cmd_code == CFSTORE_OPCODE_READ 00759 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00760 * @param hkey, unused. 00761 */ 00762 int32_t (*Read)(ARM_CFSTORE_HANDLE hkey, void* data, ARM_CFSTORE_SIZE* len); 00763 00764 00765 /** @brief Open a key-value object for future operations. 00766 * 00767 * @param key_name 00768 * IN: zero terminated string specifying the key name. 00769 * @param flags 00770 * can open a RW key in read only mode. 00771 * @param hkey 00772 * IN: pointer to client owned buffer of CFSTORE_HANDLE_BUFSIZE 00773 * bytes 00774 * OUT: on success, hkey is a valid handle to an open KV. 00775 * 00776 * @return 00777 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00778 * ARM_CFSTORE_DRIVER::(*Open)() asynchronous completion command code 00779 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00780 * @param status 00781 * ARM_DRIVER_OK => success, KV opened, else failure. 00782 * @param cmd_code == CFSTORE_OPCODE_OPEN 00783 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00784 * @param hkey now contains returned handle to newly opened key. 00785 */ 00786 int32_t (*Open)(const char* key_name, ARM_CFSTORE_FMODE flags, ARM_CFSTORE_HANDLE hkey); 00787 00788 00789 /** @brief Move the position of the read pointer within a value 00790 * 00791 * @param hkey 00792 * IN: the key referencing the value data for which the read 00793 * location should be updated. Note that this function can 00794 * only be called on pre-existing keys opened with read-only 00795 * flag. Performing a seek operation on a writable key will fail. 00796 * @param offset 00797 * IN: the new offset position from the start of the value data 00798 * 00799 * @return 00800 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00801 * ARM_CFSTORE_DRIVER::(*Rseek)() asynchronous completion command code 00802 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00803 * @param status 00804 * >=0 => success, read location set, else failure. 00805 * upon success, the function returns the current read 00806 * location measured from the beginning of the data value. 00807 * <0 => error 00808 * @param cmd_code == CFSTORE_OPCODE_RSEEK 00809 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00810 * @param hkey, unused. 00811 */ 00812 int32_t (*Rseek)(ARM_CFSTORE_HANDLE hkey, ARM_CFSTORE_OFFSET offset); 00813 00814 00815 /** @brief Function to de-initialise the Configuration Store 00816 * 00817 * @return 00818 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00819 * CFSTORE_OPCODE_UNINITIALIZE 00820 * ARM_CFSTORE_DRIVER::(*Uninitialize)() asynchronous completion command code 00821 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00822 * @param status 00823 * ARM_DRIVER_OK => success, read location set, else failure. 00824 * @param cmd_code == CFSTORE_OPCODE_UNINITIALIZE 00825 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00826 * @param hkey, unused. 00827 */ 00828 int32_t (*Uninitialize)(void); 00829 00830 00831 /** @brief Write the value data associated with a specified key 00832 * 00833 * @note Note that Write() only supports sequential-access. 00834 * 00835 * @param hkey 00836 * IN: the key for which value data will be written 00837 * @param data 00838 * IN: a pointer to a data buffer supplied by the caller for CFSTORE to 00839 * write as the binary blob value data. 00840 * Async operation: the buffer must be available until after 00841 * completion notification is received by the client. 00842 * @param len 00843 * IN: the client specifies the length of the data buffer available. 00844 * len must not exceed the value_len field specified when the 00845 * KV pair was created. 00846 * OUT: the CFSTORE specifies how many octets have been stored. 00847 * Note that fewer octets may be stored than the input len 00848 * depending on the CFSTORE internal representation of the value. 00849 * Async operation: the len storage location must be available 00850 * until after completion notification is received by the client. 00851 * 00852 * @return 00853 * See REFERENCE_1 and the ARM_CFSTORE_CALLBACK documentation. 00854 * return_value == ARM_DRIVER_OK (==0) => asynchronous 00855 * transaction pending. 00856 * return_value > 0 => synchronous completion of write with the 00857 * number of bytes written == return_value 00858 * return_value < 0, error condition. 00859 * 00860 * ARM_CFSTORE_DRIVER::(*Write)() asynchronous completion 00861 * (*ARM_CFSTORE_CALLBACK) function argument values on return: 00862 * @param status 00863 * >= 0 => success with the number bytes written equal to the value 00864 * of the return status 00865 * ARM_CFSTORE_CALLBACK status argument < 0 => error 00866 * @param cmd_code == CFSTORE_OPCODE_WRITE 00867 * @param client context, registered ARM_CFSTORE_DRIVER::(*Initialize)() 00868 * @param hkey, unused. 00869 */ 00870 int32_t (*Write)(ARM_CFSTORE_HANDLE hkey, const char* data, ARM_CFSTORE_SIZE* len); 00871 00872 } const ARM_CFSTORE_DRIVER; 00873 00874 00875 00876 MBED_DEPRECATED_SINCE("mbed-os-5.5", "CFSTORE replace by FATFileSystem.") 00877 extern ARM_CFSTORE_DRIVER cfstore_driver; 00878 00879 #ifdef __cplusplus 00880 } 00881 #endif 00882 00883 #endif /* __CONFIGURATION_STORE_H */
Generated on Sun Jul 17 2022 08:25:21 by
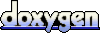