
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
config_test.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2017 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import os 00019 import sys 00020 import json 00021 import pytest 00022 from mock import patch 00023 from hypothesis import given 00024 from hypothesis.strategies import sampled_from 00025 from os.path import join, isfile, dirname, abspath 00026 from tools.build_api import get_config 00027 from tools.targets import set_targets_json_location, Target, TARGET_NAMES 00028 from tools.config import ConfigException, Config 00029 00030 def compare_config (cfg, expected): 00031 """Compare the output of config against a dictionary of known good results 00032 00033 :param cfg: the configuration to check 00034 :param expected: what to expect in that config 00035 """ 00036 try: 00037 for k in cfg: 00038 if cfg[k].value != expected[k]: 00039 return "'%s': expected '%s', got '%s'" % (k, expected[k], cfg[k].value) 00040 except KeyError: 00041 return "Unexpected key '%s' in configuration data" % k 00042 for k in expected: 00043 if k not in ["expected_macros", "expected_features"] + cfg.keys(): 00044 return "Expected key '%s' was not found in configuration data" % k 00045 return "" 00046 00047 def data_path (path): 00048 """The expected data file for a particular test 00049 00050 :param path: the path to the test 00051 """ 00052 return join(path, "test_data.json") 00053 00054 def is_test (path): 00055 """Does a directory represent a test? 00056 00057 :param path: the path to the test 00058 """ 00059 return isfile(data_path(path)) 00060 00061 root_dir = abspath(dirname(__file__)) 00062 00063 @pytest.mark.parametrize("name", filter(lambda d: is_test(join(root_dir, d)), 00064 os.listdir(root_dir))) 00065 def test_config (name): 00066 """Run a particular configuration test 00067 00068 :param name: test name (same as directory name) 00069 """ 00070 test_dir = join(root_dir, name) 00071 test_data = json.load(open(data_path(test_dir))) 00072 targets_json = os.path.join(test_dir, "targets.json") 00073 set_targets_json_location(targets_json if isfile(targets_json) else None) 00074 for target, expected in test_data.items(): 00075 try: 00076 cfg, macros, features = get_config(test_dir, target, "GCC_ARM") 00077 res = compare_config(cfg, expected) 00078 assert not(res), res 00079 expected_macros = expected.get("expected_macros", None) 00080 expected_features = expected.get("expected_features", None) 00081 00082 if expected_macros is not None: 00083 macros = Config.config_macros_to_macros(macros) 00084 assert sorted(expected_macros) == sorted(macros) 00085 if expected_features is not None: 00086 assert sorted(expected_features) == sorted(features) 00087 except ConfigException as e: 00088 err_msg = e.message 00089 if "exception_msg" not in expected: 00090 assert not(err_msg), "Unexpected Error: %s" % e 00091 else: 00092 assert expected["exception_msg"] in err_msg 00093 00094 00095 @pytest.mark.parametrize("target", ["K64F"]) 00096 def test_init_app_config (target): 00097 """ 00098 Test that the initialisation correctly uses app_config 00099 00100 :param target: The target to use 00101 """ 00102 set_targets_json_location() 00103 with patch.object(Config, '_process_config_and_overrides'),\ 00104 patch('tools.config.json_file_to_dict') as mock_json_file_to_dict: 00105 app_config = "app_config" 00106 mock_return = {'config': {'test': False}} 00107 mock_json_file_to_dict.return_value = mock_return 00108 00109 config = Config(target, app_config=app_config) 00110 00111 mock_json_file_to_dict.assert_called_with(app_config) 00112 assert config.app_config_data == mock_return 00113 00114 00115 @pytest.mark.parametrize("target", ["K64F"]) 00116 def test_init_no_app_config (target): 00117 """ 00118 Test that the initialisation works without app config 00119 00120 :param target: The target to use 00121 """ 00122 set_targets_json_location() 00123 with patch.object(Config, '_process_config_and_overrides'),\ 00124 patch('tools.config.json_file_to_dict') as mock_json_file_to_dict: 00125 config = Config(target) 00126 00127 mock_json_file_to_dict.assert_not_called() 00128 assert config.app_config_data == {} 00129 00130 00131 @pytest.mark.parametrize("target", ["K64F"]) 00132 def test_init_no_app_config_with_dir (target): 00133 """ 00134 Test that the initialisation works without app config and with a 00135 specified top level directory 00136 00137 :param target: The target to use 00138 """ 00139 set_targets_json_location() 00140 with patch.object(Config, '_process_config_and_overrides'),\ 00141 patch('os.path.isfile') as mock_isfile, \ 00142 patch('tools.config.json_file_to_dict') as mock_json_file_to_dict: 00143 directory = '.' 00144 path = os.path.join('.', 'mbed_app.json') 00145 mock_return = {'config': {'test': False}} 00146 mock_json_file_to_dict.return_value = mock_return 00147 mock_isfile.return_value = True 00148 00149 config = Config(target, [directory]) 00150 00151 mock_isfile.assert_called_with(path) 00152 mock_json_file_to_dict.assert_called_once_with(path) 00153 assert config.app_config_data == mock_return 00154 00155 00156 @pytest.mark.parametrize("target", ["K64F"]) 00157 def test_init_override_app_config (target): 00158 """ 00159 Test that the initialisation uses app_config instead of top_level_dir 00160 when both are specified 00161 00162 :param target: The target to use 00163 """ 00164 set_targets_json_location() 00165 with patch.object(Config, '_process_config_and_overrides'),\ 00166 patch('tools.config.json_file_to_dict') as mock_json_file_to_dict: 00167 app_config = "app_config" 00168 directory = '.' 00169 mock_return = {'config': {'test': False}} 00170 mock_json_file_to_dict.return_value = mock_return 00171 00172 config = Config(target, [directory], app_config=app_config) 00173 00174 mock_json_file_to_dict.assert_called_once_with(app_config) 00175 assert config.app_config_data == mock_return
Generated on Sun Jul 17 2022 08:25:21 by
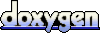