
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
coap_service_api.h
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 #ifndef COAP_SERVICE_API_H_ 00020 #define COAP_SERVICE_API_H_ 00021 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif 00025 00026 #include <string.h> 00027 00028 #include "ns_types.h" 00029 #include "mbed-coap/sn_coap_header.h" 00030 #include "ns_address.h" 00031 00032 /** 00033 * This interface is used in sending and receiving of CoAP messages to multicast address and receive multiple responses. 00034 */ 00035 00036 // Allowed_methods 00037 #define COAP_SERVICE_ACCESS_ALL_ALLOWED 0x0F 00038 #define COAP_SERVICE_ACCESS_GET_ALLOWED 0x01 00039 #define COAP_SERVICE_ACCESS_PUT_ALLOWED 0x02 00040 #define COAP_SERVICE_ACCESS_POST_ALLOWED 0x04 00041 #define COAP_SERVICE_ACCESS_DELETE_ALLOWED 0x08 00042 00043 // Bits for service options 00044 #define COAP_SERVICE_OPTIONS_NONE 0x00 00045 #define COAP_SERVICE_OPTIONS_VIRTUAL_SOCKET 0x01 00046 #define COAP_SERVICE_OPTIONS_SECURE 0x02 00047 #define COAP_SERVICE_OPTIONS_EPHEMERAL_PORT 0x04 00048 /** Coap interface selected as socket interface */ 00049 #define COAP_SERVICE_OPTIONS_SELECT_SOCKET_IF 0x08 00050 /** Register to COAP multicast groups */ 00051 #define COAP_SERVICE_OPTIONS_MULTICAST_JOIN 0x10 00052 /** Link-layer security bypass option is set*/ 00053 #define COAP_SERVICE_OPTIONS_SECURE_BYPASS 0x80 00054 00055 // Bits for request options 00056 #define COAP_REQUEST_OPTIONS_NONE 0x00 00057 #define COAP_REQUEST_OPTIONS_ADDRESS_DEFAULT 0x00//!< default is not setting either short or long. 00058 #define COAP_REQUEST_OPTIONS_ADDRESS_LONG 0x01 00059 #define COAP_REQUEST_OPTIONS_ADDRESS_SHORT 0x02 00060 #define COAP_REQUEST_OPTIONS_MULTICAST 0x04 //!< indicates that CoAP library support multicasting 00061 #define COAP_REQUEST_OPTIONS_SECURE_BYPASS 0x08 00062 00063 extern const uint8_t COAP_MULTICAST_ADDR_LINK_LOCAL[16]; //!< ff02::fd, COAP link local multicast address 00064 extern const uint8_t COAP_MULTICAST_ADDR_ADMIN_LOCAL[16]; //!< ff03::fd, COAP admin-local multicast address 00065 extern const uint8_t COAP_MULTICAST_ADDR_SITE_LOCAL[16]; //!> ff05::fd, COAP site-local multicast address 00066 00067 /** 00068 * \brief Service message response receive callback. 00069 * 00070 * Function that handles CoAP service message receiving and parsing 00071 * 00072 * \param msg_id Id number of the current message. 00073 * \param source_address IPv6 source address. 00074 * \param source_port Source port 00075 * \param response_ptr Pointer to CoAP header structure. 00076 * 00077 * \return 0 for success / -1 for failure 00078 */ 00079 typedef int coap_service_response_recv(int8_t service_id, uint8_t source_address[static 16], uint16_t source_port, sn_coap_hdr_s *response_ptr); 00080 00081 /** 00082 * \brief CoAP service request callback 00083 * 00084 * CoAP service request message receiving and parsing function 00085 * 00086 * \param service_id Id number of the current service. 00087 * \param source_address IPv6 source address. 00088 * \param source_port Source port 00089 * \param request_ptr Pointer to CoAP header structure. 00090 * 00091 * \return Status 00092 */ 00093 typedef int coap_service_request_recv_cb(int8_t service_id, uint8_t source_address[static 16], uint16_t source_port, sn_coap_hdr_s *request_ptr); 00094 00095 /** 00096 * \brief Security service start callback 00097 * 00098 * Starts security service handling and fetches device password. 00099 * 00100 * \param service_id Id number of the current service. 00101 * \param address Address of sender 00102 * \param port Port of the device 00103 * 00104 * \return 0 for success / -1 for failure 00105 */ 00106 typedef int coap_service_security_start_cb(int8_t service_id, uint8_t address[static 16], uint16_t port, uint8_t* pw, uint8_t *pw_len); 00107 00108 /** 00109 * \brief CoAP service security done callback 00110 * 00111 * CoAP service security done callback function. 00112 * 00113 * \param service_id Id number of the current service. 00114 * \param address Address of sender 00115 * \param keyblock Security key (40 bits) 00116 * 00117 * \return 0 for success / -1 for failure 00118 */ 00119 typedef int coap_service_security_done_cb(int8_t service_id, uint8_t address[static 16], uint8_t keyblock[static 40]); 00120 00121 /** 00122 * \brief Initialise server instance. 00123 * 00124 * Initialise Thread services for the registered application. 00125 * 00126 * \param interface_id Informs registered application interface id. This parameter is passed to socket implementation. 00127 * \param listen_port Port that Application wants to use for communicate with coap server. 00128 * \param service_options Options of the current service. 00129 * \param *start_ptr Callback to inform security handling is started and to fetch device password. 00130 * \param *coap_security_done_cb Callback to inform security handling is done. 00131 * 00132 * \return service_id / -1 for failure 00133 */ 00134 extern int8_t coap_service_initialize(int8_t interface_id, uint16_t listen_port, uint8_t service_options, coap_service_security_start_cb *start_ptr, coap_service_security_done_cb *coap_security_done_cb); 00135 00136 /** 00137 * \brief Service delete 00138 * 00139 * Removes all data related to this instance 00140 * 00141 * \param service_id Id number of the current service. 00142 */ 00143 extern void coap_service_delete( int8_t service_id ); 00144 00145 /** 00146 * \brief Close secure connection 00147 * 00148 * Closes secure connection (if present), but leaves socket open. 00149 * 00150 * \param service_id Id number of the current service. 00151 */ 00152 extern void coap_service_close_secure_connection(int8_t service_id, uint8_t destination_addr_ptr[static 16], uint16_t port); 00153 00154 /** 00155 * \brief Sets password for device 00156 * 00157 * \param service_id Service id 00158 * \param address Device address 00159 * \param port Device port 00160 * \param pw_ptr Pointer to password. 00161 * \param pw_len Lenght of password. 00162 * 00163 * \return 0 for success / -1 for failure 00164 */ 00165 //int coap_service_security_key_set(int8_t service_id, uint8_t address[static 16], uint16_t port, uint8_t *pw_ptr, uint8_t pw_len); 00166 00167 /** 00168 * \brief Virtual socket sent callback. 00169 * 00170 * Sent data to virtual socket. 00171 * 00172 * \param service_id Id number of the current service. 00173 * \param destination_addr_ptr Receiver IPv6 address. 00174 * \param port Receiver port number. 00175 * \param *data_ptr Pointer to the data. 00176 * \param data_len Lenght of the data. 00177 * 00178 * \return 0 for success / -1 for failure 00179 */ 00180 typedef int coap_service_virtual_socket_send_cb(int8_t service_id, uint8_t destination_addr_ptr[static 16], uint16_t port, const uint8_t *data_ptr, uint16_t data_len); 00181 00182 /** 00183 * \brief Virtual socket read. 00184 * 00185 * Receive data from virtual socket. 00186 * 00187 * \param service_id Id number of the current service. 00188 * \param source_addr_ptr Receiver IPv6 address. 00189 * \param port Receiver port number. 00190 * \param *data_ptr Pointer to the data 00191 * \param data_len Lenght of the data 00192 * 00193 * \return 0 for success / -1 for failure 00194 */ 00195 extern int16_t coap_service_virtual_socket_recv(int8_t service_id, uint8_t source_addr_ptr[static 16], uint16_t port, uint8_t *data_ptr, uint16_t data_len); 00196 00197 /** 00198 * \brief Set virtual socket 00199 * 00200 * Sets virtual socket for CoAP services. 00201 * 00202 * \param service_id Id number of the current service. 00203 * \param *send_method_ptr Callback to coap virtual socket. 00204 * 00205 * \return 0 for success / -1 for failure 00206 */ 00207 extern int16_t coap_service_virtual_socket_set_cb(int8_t service_id, coap_service_virtual_socket_send_cb *send_method_ptr); 00208 00209 /** 00210 * \brief Register unsecure callback methods to CoAP server 00211 * 00212 * Register application and informs CoAP services unsecure registery callback function. 00213 * 00214 * \param service_id Id number of the current service. 00215 * \param *uri Uri address. 00216 * \param port port that Application wants to use for communicate with coap server. 00217 * \param allowed_method Informs method that is allowed to use (used defines described above). 00218 * \param *request_recv_cb CoAP service request receive callback function pointer. 00219 * 00220 * \return 0 for success / -1 for failure 00221 */ 00222 extern int8_t coap_service_register_uri(int8_t service_id, const char *uri, uint8_t allowed_method, coap_service_request_recv_cb *request_recv_cb); 00223 00224 /** 00225 * \brief Unregister unsecure callback methods to CoAP server 00226 * 00227 * Register application and informs CoAP services unsecure registery callback function. 00228 * 00229 * \param service_id Id number of the current service. 00230 * \param *uri Uri address. 00231 * 00232 * \return 0 for success / -1 for failure 00233 */ 00234 extern int8_t coap_service_unregister_uri(int8_t service_id, const char *uri); 00235 00236 /** 00237 * \brief Sends CoAP service request 00238 * 00239 * Build and sends CoAP service request message. 00240 * 00241 * \param service_id Id number of the current service. 00242 * \param options Options defined above. 00243 * \param destination_addr IPv6 address. 00244 * \param destination_port Destination port 00245 * \param msg_type Message type can be found from sn_coap_header. 00246 * \param msg_code Message code can be found from sn_coap_header. 00247 * \param *uri Uri address. 00248 * \param cont_type Content type can be found from sn_coap_header. 00249 * \param payload_ptr Pointer to message content. 00250 * \param payload_len Lenght of the message. 00251 * \param *request_response_cb Callback to inform result of the request. 00252 * 00253 * \return msg_id Id number of the current message. 00254 */ 00255 extern uint16_t coap_service_request_send(int8_t service_id, uint8_t options, const uint8_t destination_addr[static 16], uint16_t destination_port, sn_coap_msg_type_e msg_type, sn_coap_msg_code_e msg_code, const char *uri, 00256 sn_coap_content_format_e cont_type, const uint8_t *payload_ptr, uint16_t payload_len, coap_service_response_recv *request_response_cb); 00257 00258 /** 00259 * \brief Sends CoAP service response 00260 * 00261 * Build and sends CoAP service response message. 00262 * 00263 * \param service_id Id number of the current service. 00264 * \param msg_id Message ID number. 00265 * \param options Options defined above. 00266 * \param response_ptr Pointer to CoAP header structure. 00267 * 00268 * \return -1 For failure 00269 *- 0 For success 00270 */ 00271 extern int8_t coap_service_response_send(int8_t service_id, uint8_t options, sn_coap_hdr_s *request_ptr, sn_coap_msg_code_e message_code, sn_coap_content_format_e content_type, const uint8_t *payload_ptr,uint16_t payload_len); 00272 00273 /** 00274 * \brief Delete CoAP request transaction 00275 * 00276 * Removes pending CoAP transaction from service. 00277 * 00278 * \param service_id Id number of the current service. 00279 * \param msg_id Message ID number. 00280 * 00281 * \return -1 For failure 00282 *- 0 For success 00283 */ 00284 extern int8_t coap_service_request_delete(int8_t service_id, uint16_t msg_id); 00285 00286 /** 00287 * \brief Set DTLS handshake timeout values 00288 * 00289 * Configures the DTLS handshake timeout values. 00290 * 00291 * \param service_id Id number of the current service. 00292 * \param min Initial timeout value. 00293 * \param max Maximum value of timeout. 00294 * 00295 * \return -1 For failure 00296 *- 0 For success 00297 */ 00298 extern int8_t coap_service_set_handshake_timeout(int8_t service_id, uint32_t min, uint32_t max); 00299 00300 /** 00301 * \brief Set CoAP duplication message buffer size 00302 * 00303 * Configures the CoAP duplication message buffer size. 00304 * 00305 * \param service_id Id number of the current service. 00306 * \param size Buffer size (messages) 00307 * 00308 * \return -1 For failure 00309 *- 0 For success 00310 */ 00311 extern int8_t coap_service_set_duplicate_message_buffer(int8_t service_id, uint8_t size); 00312 #ifdef __cplusplus 00313 } 00314 #endif 00315 00316 #endif /* COAP_SERVICE_API_H_ */
Generated on Sun Jul 17 2022 08:25:21 by
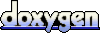