
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
coap_connection_handler_stub.c
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 00005 #include <string.h> 00006 #include "coap_connection_handler.h" 00007 #include "coap_security_handler.h" 00008 #include "ns_list.h" 00009 #include "ns_trace.h" 00010 #include "nsdynmemLIB.h" 00011 #include "socket_api.h" 00012 #include "net_interface.h" 00013 #include "eventOS_callback_timer.h" 00014 #include "coap_connection_handler_stub.h" 00015 00016 thread_conn_handler_stub_def thread_conn_handler_stub; 00017 00018 int coap_connection_handler_virtual_recv(coap_conn_handler_t *handler, uint8_t address[static 16], uint16_t port, uint8_t *data_ptr, uint16_t data_len) 00019 { 00020 return thread_conn_handler_stub.int_value; 00021 } 00022 00023 coap_conn_handler_t *connection_handler_create(int (*recv_cb)(int8_t socket_id, uint8_t src_address[static 16], uint16_t port, const uint8_t dst_address[static 16], unsigned char *, int), 00024 int (*send_cb)(int8_t socket_id, uint8_t const address[static 16], uint16_t port, const void *, int), 00025 int (*pw_cb)(int8_t socket_id, uint8_t address[static 16], uint16_t port, uint8_t *pw_ptr, uint8_t *pw_len), 00026 void(*done_cb)(int8_t socket_id, uint8_t address[static 16], uint16_t port, uint8_t keyblock[static KEY_BLOCK_LEN]) ) 00027 { 00028 thread_conn_handler_stub.send_to_sock_cb = send_cb; 00029 thread_conn_handler_stub.receive_from_sock_cb = recv_cb; 00030 thread_conn_handler_stub.get_passwd_cb = pw_cb; 00031 thread_conn_handler_stub.sec_done_cb = done_cb; 00032 return thread_conn_handler_stub.handler_obj; 00033 } 00034 00035 void connection_handler_destroy( coap_conn_handler_t *handler, bool multicast_group_leave) 00036 { 00037 00038 } 00039 void connection_handler_close_secure_connection( coap_conn_handler_t *handler, uint8_t destination_addr_ptr[static 16], uint16_t port ) 00040 { 00041 00042 } 00043 00044 int coap_connection_handler_open_connection(coap_conn_handler_t *handler, uint16_t listen_port, bool use_ephemeral_port, bool is_secure, bool is_real_socket, bool bypassSec) 00045 { 00046 return thread_conn_handler_stub.int_value; 00047 } 00048 00049 int coap_connection_handler_send_data(coap_conn_handler_t *handler, const ns_address_t *dest_addr, const uint8_t dst_address[static 16], uint8_t *data_ptr, uint16_t data_len, bool bypass_link_sec) 00050 { 00051 return thread_conn_handler_stub.int_value; 00052 } 00053 00054 bool coap_connection_handler_socket_belongs_to(coap_conn_handler_t *handler, int8_t socket_id) 00055 { 00056 return thread_conn_handler_stub.bool_value; 00057 } 00058 00059 int8_t coap_connection_handler_set_timeout(coap_conn_handler_t *handler, uint32_t min, uint32_t max) 00060 { 00061 return 0; 00062 } 00063 00064 void coap_connection_handler_exec(uint32_t time) 00065 { 00066 00067 }
Generated on Sun Jul 17 2022 08:25:21 by
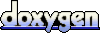