
Rtos API example
Mail< T, queue_sz > Class Template Reference
[Mail class]
The Mail class allow to control, send, receive, or wait for mail. More...
#include <Mail.h>
Inherits NonCopyable< Mail< T, queue_sz > >.
Public Member Functions | |
Mail () | |
Create and Initialise Mail queue. | |
bool | empty () const |
Check if the mail queue is empty. | |
bool | full () const |
Check if the mail queue is full. | |
T * | alloc (uint32_t millisec=0) |
Allocate a memory block of type T. | |
T * | calloc (uint32_t millisec=0) |
Allocate a memory block of type T and set memory block to zero. | |
osStatus | put (T *mptr) |
Put a mail in the queue. | |
osEvent | get (uint32_t millisec=osWaitForever) |
Get a mail from a queue. | |
osStatus | free (T *mptr) |
Free a memory block from a mail. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
template<typename T, uint32_t queue_sz>
class rtos::Mail< T, queue_sz >
The Mail class allow to control, send, receive, or wait for mail.
A mail is a memory block that is send to a thread or interrupt service routine.
- Template Parameters:
-
T data type of a single message element. queue_sz maximum number of messages in queue.
- Note:
- Memory considerations: The mail data store and control structures will be created on current thread's stack, both for the mbed OS and underlying RTOS objects (static or dynamic RTOS memory pools are not being used).
Definition at line 56 of file Mail.h.
Constructor & Destructor Documentation
Member Function Documentation
T* alloc | ( | uint32_t | millisec = 0 ) |
T* calloc | ( | uint32_t | millisec = 0 ) |
bool empty | ( | ) | const |
osStatus free | ( | T * | mptr ) |
bool full | ( | ) | const |
osEvent get | ( | uint32_t | millisec = osWaitForever ) |
osStatus put | ( | T * | mptr ) |
Put a mail in the queue.
- Parameters:
-
mptr memory block previously allocated with Mail::alloc or Mail::calloc.
- Returns:
- status code that indicates the execution status of the function.
Generated on Sun Jul 17 2022 08:25:44 by
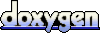