
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
cfstore_test.h
Go to the documentation of this file.
00001 /** @file cfstore_test.h 00002 * 00003 * mbed Microcontroller Library 00004 * Copyright (c) 2006-2016 ARM Limited 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); 00007 * you may not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, 00014 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 * 00018 * Header file for test support data structures and function API. 00019 */ 00020 #ifndef __CFSTORE_TEST_H 00021 #define __CFSTORE_TEST_H 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 #include "configuration_store.h" 00028 00029 /* Defines */ 00030 #define CFSTORE_INIT_1_TABLE_HEAD { "a", ""} 00031 #define CFSTORE_INIT_1_TABLE_MID_NODE { "0123456789abcdef0123456", "abcdefghijklmnopqrstuvwxyz"} 00032 #define CFSTORE_INIT_1_TABLE_TAIL { "yotta.hello-world.animal{wobbly-dog}{foot}backRight", "present"} 00033 #define CFSTORE_TEST_RW_TABLE_SENTINEL 0xffffffff 00034 #define CFSTORE_TEST_BYTE_DATA_TABLE_SIZE 256 00035 #define CFSTORE_UTEST_MSG_BUF_SIZE 256 00036 #define CFSTORE_UTEST_DEFAULT_TIMEOUT_MS 10000 00037 #define CFSTORE_MBED_HOSTTEST_TIMEOUT 60 00038 00039 /* support macro for make string for utest _MESSAGE macros, which dont support formatted output */ 00040 #define CFSTORE_TEST_UTEST_MESSAGE(_buf, _max_len, _fmt, ...) \ 00041 do \ 00042 { \ 00043 snprintf((_buf), (_max_len), (_fmt), __VA_ARGS__); \ 00044 }while(0); 00045 00046 00047 /* 00048 * Structures 00049 */ 00050 00051 /* kv data for test */ 00052 typedef struct cfstore_kv_data_t { 00053 const char* key_name; 00054 const char* value; 00055 } cfstore_kv_data_t; 00056 00057 typedef struct cfstore_test_rw_data_entry_t 00058 { 00059 uint32_t offset; 00060 char rw_char; 00061 } cfstore_test_rw_data_entry_t; 00062 00063 00064 extern cfstore_kv_data_t cfstore_test_init_1_data[]; 00065 extern cfstore_test_rw_data_entry_t cfstore_test_rw_data_table[]; 00066 extern const char* cfstore_test_opcode_str[]; 00067 extern const uint8_t cfstore_test_byte_data_table[CFSTORE_TEST_BYTE_DATA_TABLE_SIZE]; 00068 00069 int32_t cfstore_test_check_node_correct(const cfstore_kv_data_t* node); 00070 int32_t cfstore_test_create(const char* key_name, const char* data, size_t* len, ARM_CFSTORE_KEYDESC* kdesc); 00071 int32_t cfstore_test_create_table(const cfstore_kv_data_t* table); 00072 int32_t cfstore_test_delete(const char* key_name); 00073 int32_t cfstore_test_delete_all(void); 00074 int32_t cfstore_test_dump(void); 00075 int32_t cfstore_test_init_1(void); 00076 int32_t cfstore_test_kv_is_found(const char* key_name, bool* bfound); 00077 int32_t cfstore_test_kv_name_gen(char* name, const size_t len); 00078 int32_t cfstore_test_read(const char* key_name, char* data, size_t* len); 00079 int32_t cfstore_test_startup(void); 00080 int32_t cfstore_test_write(const char* key_name, const char* data, size_t* len); 00081 00082 #ifdef __cplusplus 00083 } 00084 #endif 00085 00086 #endif /* __CFSTORE_TEST_H */
Generated on Sun Jul 17 2022 08:25:21 by
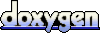