
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
cc.h
00001 /* 00002 * Copyright (c) 2001-2003 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __CC_H__ 00033 #define __CC_H__ 00034 00035 #include <stdint.h> 00036 #include <stddef.h> /* for size_t */ 00037 00038 #if LWIP_USE_EXTERNAL_MBEDTLS 00039 #include "mbedtls/md5.h" 00040 #endif 00041 00042 00043 /* ARM/LPC17xx is little endian only */ 00044 #if !defined(BYTE_ORDER) || (BYTE_ORDER != LITTLE_ENDIAN && BYTE_ORDER != BIG_ENDIAN) 00045 #ifdef BYTE_ORDER 00046 #undef BYTE_ORDER 00047 #endif 00048 #define BYTE_ORDER LITTLE_ENDIAN 00049 #endif 00050 00051 /* Use LWIP error codes */ 00052 #define LWIP_PROVIDE_ERRNO 00053 00054 #if defined(__arm__) && defined(__ARMCC_VERSION) && (__ARMCC_VERSION < 6010050) 00055 /* Keil uVision4 tools */ 00056 #define PACK_STRUCT_BEGIN __packed 00057 #define PACK_STRUCT_STRUCT 00058 #define PACK_STRUCT_END 00059 #define PACK_STRUCT_FIELD(fld) fld 00060 #define ALIGNED(n) __align(n) 00061 #elif defined (__IAR_SYSTEMS_ICC__) 00062 /* IAR Embedded Workbench tools */ 00063 #define PACK_STRUCT_BEGIN __packed 00064 #define PACK_STRUCT_STRUCT 00065 #define PACK_STRUCT_END 00066 #define PACK_STRUCT_FIELD(fld) fld 00067 #define IAR_STR(a) #a 00068 #define ALIGNED(n) _Pragma(IAR_STR(data_alignment= ## n ##)) 00069 #else 00070 /* GCC tools (CodeSourcery) */ 00071 #define PACK_STRUCT_BEGIN 00072 #define PACK_STRUCT_STRUCT __attribute__ ((__packed__)) 00073 #define PACK_STRUCT_END 00074 #define PACK_STRUCT_FIELD(fld) fld 00075 #define ALIGNED(n) __attribute__((aligned (n))) 00076 #endif 00077 00078 /* Provide Thumb-2 routines for GCC to improve performance */ 00079 #if defined(TOOLCHAIN_GCC) && defined(__thumb2__) 00080 #define MEMCPY(dst,src,len) thumb2_memcpy(dst,src,len) 00081 #define LWIP_CHKSUM thumb2_checksum 00082 /* Set algorithm to 0 so that unused lwip_standard_chksum function 00083 doesn't generate compiler warning */ 00084 #define LWIP_CHKSUM_ALGORITHM 0 00085 00086 void* thumb2_memcpy(void* pDest, const void* pSource, size_t length); 00087 uint16_t thumb2_checksum(const void* pData, int length); 00088 #else 00089 /* Used with IP headers only */ 00090 #define LWIP_CHKSUM_ALGORITHM 1 00091 #endif 00092 00093 00094 #ifdef LWIP_DEBUG 00095 00096 #if MBED_CONF_LWIP_USE_MBED_TRACE 00097 void lwip_mbed_tracef_debug(const char *fmt, ...); 00098 void lwip_mbed_tracef_error(const char *fmt, ...); 00099 void lwip_mbed_tracef_warn(const char *fmt, ...); 00100 void lwip_mbed_assert_fail(const char *msg, const char *func, const char *file, unsigned int line); 00101 00102 #define LWIP_PLATFORM_DIAG(vars) lwip_mbed_tracef_debug vars 00103 #define LWIP_PLATFORM_DIAG_SEVERE(vars) lwip_mbed_tracef_error vars 00104 #define LWIP_PLATFORM_DIAG_SERIOUS(vars) lwip_mbed_tracef_error vars 00105 #define LWIP_PLATFORM_DIAG_WARNING(vars) lwip_mbed_tracef_warn vars 00106 00107 #define LWIP_PLATFORM_ASSERT(message) lwip_mbed_assert_fail(message, __func__, __FILE__, __LINE__) 00108 00109 #else // MBED_CONF_LWIP_USE_MBED_TRACE 00110 #include <stdio.h> 00111 00112 void assert_printf(char *msg, int line, char *file); 00113 00114 /* Plaform specific diagnostic output */ 00115 #define LWIP_PLATFORM_DIAG(vars) printf vars 00116 #define LWIP_PLATFORM_ASSERT(flag) { assert_printf((flag), __LINE__, __FILE__); } 00117 #endif // MBED_CONF_LWIP_USE_MBED_TRACE 00118 #endif 00119 00120 #if TRACE_TO_ASCII_HEX_DUMP 00121 #define TRACE_TO_ASCII_HEX_DUMPF(prefix, len, data) trace_to_ascii_hex_dump(prefix, len, data) 00122 void trace_to_ascii_hex_dump(char* prefix, int len, char *data); 00123 #else 00124 #define TRACE_TO_ASCII_HEX_DUMPF(prefix, len, data) ((void)0) 00125 #endif 00126 00127 #include "cmsis.h" 00128 #define LWIP_PLATFORM_HTONS(x) __REV16(x) 00129 #define LWIP_PLATFORM_HTONL(x) __REV(x) 00130 00131 /* Define the memory area for the lwip's memory pools */ 00132 #ifndef MEMP_SECTION 00133 #if defined(TARGET_LPC4088) || defined(TARGET_LPC4088_DM) 00134 # if defined (__ICCARM__) 00135 # define MEMP_SECTION 00136 # elif defined(TOOLCHAIN_GCC_CR) 00137 # define MEMP_SECTION __attribute__((section(".data.$RamPeriph32"))) 00138 # else 00139 # define MEMP_SECTION __attribute__((section("AHBSRAM0"),aligned)) 00140 # endif 00141 #elif defined(TARGET_LPC1768) 00142 # if defined (__ICCARM__) 00143 # define MEMP_SECTION 00144 # elif defined(TOOLCHAIN_GCC_CR) 00145 # define MEMP_SECTION __attribute__((section(".data.$RamPeriph32"))) 00146 # else 00147 # define MEMP_SECTION __attribute__((section("AHBSRAM1"),aligned)) 00148 # endif 00149 #endif 00150 #endif 00151 00152 #ifdef MEMP_SECTION 00153 #define SET_MEMP_SECTION(name) extern uint8_t MEMP_SECTION name[] 00154 00155 #if defined (__ICCARM__) 00156 #pragma default_variable_attributes = @ ".ethusbram" 00157 #endif 00158 SET_MEMP_SECTION(memp_memory_REASSDATA_base); 00159 SET_MEMP_SECTION(memp_memory_TCP_PCB_LISTEN_base); 00160 SET_MEMP_SECTION(memp_memory_PBUF_POOL_base); 00161 SET_MEMP_SECTION(memp_memory_NETCONN_base); 00162 SET_MEMP_SECTION(memp_memory_IGMP_GROUP_base); 00163 SET_MEMP_SECTION(memp_memory_UDP_PCB_base); 00164 SET_MEMP_SECTION(memp_memory_TCP_PCB_base); 00165 SET_MEMP_SECTION(memp_memory_FRAG_PBUF_base); 00166 SET_MEMP_SECTION(memp_memory_PBUF_base); 00167 SET_MEMP_SECTION(memp_memory_MLD6_GROUP_base); 00168 SET_MEMP_SECTION(memp_memory_IP6_REASSDATA_base); 00169 SET_MEMP_SECTION(memp_memory_NETBUF_base); 00170 SET_MEMP_SECTION(memp_memory_TCPIP_MSG_INPKT_base); 00171 SET_MEMP_SECTION(memp_memory_SYS_TIMEOUT_base); 00172 SET_MEMP_SECTION(memp_memory_TCP_SEG_base); 00173 SET_MEMP_SECTION(memp_memory_TCPIP_MSG_API_base); 00174 SET_MEMP_SECTION(memp_memory_PPP_PCB_base); 00175 SET_MEMP_SECTION(memp_memory_PPPOS_PCB_base); 00176 SET_MEMP_SECTION(memp_memory_PPP_PCB_base); 00177 #if defined (__ICCARM__) 00178 #pragma default_variable_attributes = 00179 #endif 00180 #endif 00181 00182 #endif /* __CC_H__ */
Generated on Sun Jul 17 2022 08:25:20 by
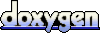