
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
build_release.py
00001 #! /usr/bin/env python 00002 """ 00003 mbed SDK 00004 Copyright (c) 2011-2013 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 """ 00018 import sys 00019 from time import time 00020 from os.path import join, abspath, dirname, normpath 00021 from optparse import OptionParser 00022 import json 00023 from shutil import copy 00024 00025 # Be sure that the tools directory is in the search path 00026 ROOT = abspath(join(dirname(__file__), "..")) 00027 sys.path.insert(0, ROOT) 00028 00029 from tools.build_api import build_mbed_libs 00030 from tools.build_api import write_build_report 00031 from tools.build_api import get_mbed_official_release 00032 from tools.options import extract_profile 00033 from tools.targets import TARGET_MAP, TARGET_NAMES 00034 from tools.test_exporters import ReportExporter, ResultExporterType 00035 from tools.test_api import SingleTestRunner 00036 from tools.test_api import singletest_in_cli_mode 00037 from tools.paths import TEST_DIR, MBED_LIBRARIES 00038 from tools.tests import TEST_MAP 00039 00040 OFFICIAL_MBED_LIBRARY_BUILD = get_mbed_official_release('2') 00041 00042 if __name__ == '__main__': 00043 parser = OptionParser() 00044 parser.add_option('-o', '--official', dest="official_only", default=False, action="store_true", 00045 help="Build using only the official toolchain for each target") 00046 parser.add_option("-j", "--jobs", type="int", dest="jobs", 00047 default=1, help="Number of concurrent jobs (default 1). Use 0 for auto based on host machine's number of CPUs") 00048 parser.add_option("-v", "--verbose", action="store_true", dest="verbose", 00049 default=False, help="Verbose diagnostic output") 00050 parser.add_option("-t", "--toolchains", dest="toolchains", help="Use toolchains names separated by comma") 00051 00052 parser.add_option("--profile", dest="profile", action="append", default=[]) 00053 00054 parser.add_option("-p", "--platforms", dest="platforms", default="", help="Build only for the platform namesseparated by comma") 00055 00056 parser.add_option("-L", "--list-config", action="store_true", dest="list_config", 00057 default=False, help="List the platforms and toolchains in the release in JSON") 00058 00059 parser.add_option("", "--report-build", dest="report_build_file_name", help="Output the build results to an junit xml file") 00060 00061 parser.add_option("", "--build-tests", dest="build_tests", help="Build all tests in the given directories (relative to /libraries/tests)") 00062 00063 00064 options, args = parser.parse_args() 00065 00066 00067 00068 if options.list_config: 00069 print json.dumps(OFFICIAL_MBED_LIBRARY_BUILD, indent=4) 00070 sys.exit() 00071 00072 start = time() 00073 build_report = {} 00074 build_properties = {} 00075 00076 platforms = None 00077 if options.platforms != "": 00078 platforms = set(options.platforms.split(",")) 00079 00080 if options.build_tests: 00081 # Get all paths 00082 directories = options.build_tests.split(',') 00083 for i in range(len(directories)): 00084 directories[i] = normpath(join(TEST_DIR, directories[i])) 00085 00086 test_names = [] 00087 00088 for test_id in TEST_MAP.keys(): 00089 # Prevents tests with multiple source dirs from being checked 00090 if isinstance( TEST_MAP[test_id].source_dir, basestring): 00091 test_path = normpath(TEST_MAP[test_id].source_dir) 00092 for directory in directories: 00093 if directory in test_path: 00094 test_names.append(test_id) 00095 00096 mut_counter = 1 00097 mut = {} 00098 test_spec = { 00099 "targets": {} 00100 } 00101 00102 if options.toolchains: 00103 print "Only building using the following toolchains: %s" % (options.toolchains) 00104 00105 for target_name, toolchain_list in OFFICIAL_MBED_LIBRARY_BUILD: 00106 toolchains = None 00107 if platforms is not None and not target_name in platforms: 00108 print("Excluding %s from release" % target_name) 00109 continue 00110 00111 if target_name not in TARGET_NAMES: 00112 print "Target '%s' is not a valid target. Excluding from release" 00113 continue 00114 00115 if options.official_only: 00116 toolchains = (getattr(TARGET_MAP[target_name], 'default_toolchain', 'ARM'),) 00117 else: 00118 toolchains = toolchain_list 00119 00120 if options.toolchains: 00121 toolchainSet = set(toolchains) 00122 toolchains = toolchainSet.intersection(set((options.toolchains).split(','))) 00123 00124 mut[str(mut_counter)] = { 00125 "mcu": target_name 00126 } 00127 00128 mut_counter += 1 00129 00130 test_spec["targets"][target_name] = toolchains 00131 00132 single_test = SingleTestRunner(_muts=mut, 00133 _parser=parser, 00134 _opts=options, 00135 _opts_report_build_file_name=options.report_build_file_name, 00136 _test_spec=test_spec, 00137 _opts_test_by_names=",".join(test_names), 00138 _opts_verbose=options.verbose, 00139 _opts_only_build_tests=True, 00140 _opts_suppress_summary=True, 00141 _opts_jobs=options.jobs, 00142 _opts_include_non_automated=True, 00143 _opts_build_report=build_report, 00144 _opts_build_properties=build_properties) 00145 # Runs test suite in CLI mode 00146 test_summary, shuffle_seed, test_summary_ext, test_suite_properties_ext, new_build_report, new_build_properties = single_test.execute() 00147 else: 00148 for target_name, toolchain_list in OFFICIAL_MBED_LIBRARY_BUILD: 00149 if platforms is not None and not target_name in platforms: 00150 print("Excluding %s from release" % target_name) 00151 continue 00152 00153 if target_name not in TARGET_NAMES: 00154 print "Target '%s' is not a valid target. Excluding from release" 00155 continue 00156 00157 if options.official_only: 00158 toolchains = (getattr(TARGET_MAP[target_name], 'default_toolchain', 'ARM'),) 00159 else: 00160 toolchains = toolchain_list 00161 00162 if options.toolchains: 00163 print "Only building using the following toolchains: %s" % (options.toolchains) 00164 toolchainSet = set(toolchains) 00165 toolchains = toolchainSet.intersection(set((options.toolchains).split(','))) 00166 00167 for toolchain in toolchains: 00168 id = "%s::%s" % (target_name, toolchain) 00169 00170 profile = extract_profile(parser, options, toolchain) 00171 00172 try: 00173 built_mbed_lib = build_mbed_libs(TARGET_MAP[target_name], 00174 toolchain, 00175 verbose=options.verbose, 00176 jobs=options.jobs, 00177 report=build_report, 00178 properties=build_properties, 00179 build_profile=profile) 00180 00181 except Exception, e: 00182 print str(e) 00183 00184 # copy targets.json file as part of the release 00185 copy(join(dirname(abspath(__file__)), '..', 'targets', 'targets.json'), MBED_LIBRARIES) 00186 00187 # Write summary of the builds 00188 if options.report_build_file_name: 00189 file_report_exporter = ReportExporter(ResultExporterType.JUNIT, package="build") 00190 file_report_exporter.report_to_file(build_report, options.report_build_file_name, test_suite_properties=build_properties) 00191 00192 print "\n\nCompleted in: (%.2f)s" % (time() - start) 00193 00194 print_report_exporter = ReportExporter(ResultExporterType.PRINT, package="build") 00195 status = print_report_exporter.report(build_report) 00196 00197 if not status: 00198 sys.exit(1)
Generated on Sun Jul 17 2022 08:25:20 by
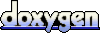