
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
build_everything.py
00001 #! /usr/bin/env python 00002 """ 00003 mbed SDK 00004 Copyright (c) 2011-2013 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 """ 00018 import sys 00019 from time import time 00020 from os.path import join, abspath, dirname, normpath 00021 from optparse import OptionParser 00022 import json 00023 00024 # Be sure that the tools directory is in the search path 00025 ROOT = abspath(join(dirname(__file__), "..")) 00026 sys.path.insert(0, ROOT) 00027 00028 from tools.build_api import build_library 00029 from tools.build_api import write_build_report 00030 from tools.targets import TARGET_MAP, TARGET_NAMES 00031 from tools.toolchains import TOOLCHAINS 00032 from tools.test_exporters import ReportExporter, ResultExporterType 00033 from tools.test_api import find_tests, build_tests, test_spec_from_test_builds 00034 from tools.build_release import OFFICIAL_MBED_LIBRARY_BUILD 00035 00036 if __name__ == '__main__': 00037 try: 00038 parser = OptionParser() 00039 00040 parser.add_option("--source", dest="source_dir", 00041 default=None, help="The source (input) directory (for sources other than tests). Defaults to current directory.", action="append") 00042 00043 parser.add_option("--build", dest="build_dir", 00044 default=None, help="The build (output) directory") 00045 00046 parser.add_option('-c', '--clean', 00047 dest='clean', 00048 metavar=False, 00049 action="store_true", 00050 help='Clean the build directory') 00051 00052 parser.add_option('-a', '--all', dest="all", default=False, action="store_true", 00053 help="Build every target (including unofficial targets) and with each of the supported toolchains") 00054 00055 parser.add_option('-o', '--official', dest="official_only", default=False, action="store_true", 00056 help="Build using only the official toolchain for each target") 00057 00058 parser.add_option("-D", "", 00059 action="append", 00060 dest="macros", 00061 help="Add a macro definition") 00062 00063 parser.add_option("-j", "--jobs", type="int", dest="jobs", 00064 default=0, help="Number of concurrent jobs. Default: 0/auto (based on host machine's number of CPUs)") 00065 00066 parser.add_option("-v", "--verbose", action="store_true", dest="verbose", 00067 default=False, help="Verbose diagnostic output") 00068 00069 parser.add_option("-t", "--toolchains", dest="toolchains", help="Use toolchains names separated by comma") 00070 00071 parser.add_option("-p", "--platforms", dest="platforms", default="", help="Build only for the platform namesseparated by comma") 00072 00073 parser.add_option("", "--config", action="store_true", dest="list_config", 00074 default=False, help="List the platforms and toolchains in the release in JSON") 00075 00076 parser.add_option("", "--test-spec", dest="test_spec", 00077 default=None, help="Destination path for a test spec file that can be used by the Greentea automated test tool") 00078 00079 parser.add_option("", "--build-report-junit", dest="build_report_junit", help="Output the build results to an junit xml file") 00080 00081 parser.add_option("--continue-on-build-fail", action="store_true", dest="continue_on_build_fail", 00082 default=False, help="Continue trying to build all tests if a build failure occurs") 00083 00084 options, args = parser.parse_args() 00085 00086 # Get set of valid targets 00087 all_platforms = set(TARGET_NAMES) 00088 bad_platforms = set() 00089 platforms = set() 00090 if options.platforms != "": 00091 platforms = set(options.platforms.split(",")) 00092 bad_platforms = platforms.difference(all_platforms) 00093 platforms = platforms.intersection(all_platforms) 00094 elif options.all: 00095 platforms = all_platforms 00096 else: 00097 platforms = set(x[0] for x in OFFICIAL_MBED_LIBRARY_BUILD) 00098 bad_platforms = platforms.difference(all_platforms) 00099 platforms = platforms.intersection(all_platforms) 00100 00101 for bad_platform in bad_platforms: 00102 print "Platform '%s' is not a valid platform. Skipping." % bad_platform 00103 00104 if options.platforms: 00105 print "Limiting build to the following platforms: %s" % ",".join(platforms) 00106 00107 # Get set of valid toolchains 00108 all_toolchains = set(TOOLCHAINS) 00109 bad_toolchains = set() 00110 toolchains = set() 00111 00112 if options.toolchains: 00113 toolchains = set(options.toolchains.split(",")) 00114 bad_toolchains = toolchains.difference(all_toolchains) 00115 toolchains = toolchains.intersection(all_toolchains) 00116 else: 00117 toolchains = all_toolchains 00118 00119 for bad_toolchain in bad_toolchains: 00120 print "Toolchain '%s' is not a valid toolchain. Skipping." % bad_toolchain 00121 00122 if options.toolchains: 00123 print "Limiting build to the following toolchains: %s" % ",".join(toolchains) 00124 00125 build_config = {} 00126 00127 for platform in platforms: 00128 target = TARGET_MAP[platform] 00129 00130 if options.official_only: 00131 default_toolchain = getattr(target, 'default_toolchain', 'ARM') 00132 build_config[platform] = list(toolchains.intersection(set([default_toolchain]))) 00133 else: 00134 build_config[platform] = list(toolchains.intersection(set(target.supported_toolchains))) 00135 00136 if options.list_config: 00137 print json.dumps(build_config, indent=4) 00138 sys.exit(0) 00139 00140 # Ensure build directory is set 00141 if not options.build_dir: 00142 print "[ERROR] You must specify a build path" 00143 sys.exit(1) 00144 00145 # Default base source path is the current directory 00146 base_source_paths = options.source_dir 00147 if not base_source_paths: 00148 base_source_paths = ['.'] 00149 00150 00151 start = time() 00152 build_report = {} 00153 build_properties = {} 00154 test_builds = {} 00155 total_build_success = True 00156 00157 for target_name, target_toolchains in build_config.iteritems(): 00158 target = TARGET_MAP[target_name] 00159 00160 for target_toolchain in target_toolchains: 00161 library_build_success = True 00162 00163 try: 00164 build_directory = join(options.build_dir, target_name, target_toolchain) 00165 # Build sources 00166 build_library(base_source_paths, build_directory, target, target_toolchain, 00167 jobs=options.jobs, 00168 clean=options.clean, 00169 report=build_report, 00170 properties=build_properties, 00171 name="mbed-os", 00172 macros=options.macros, 00173 verbose=options.verbose, 00174 archive=False) 00175 except Exception, e: 00176 library_build_success = False 00177 print "Failed to build library" 00178 print e 00179 00180 if options.continue_on_build_fail or library_build_success: 00181 # Build all the tests 00182 all_tests = find_tests(base_source_paths[0], target_name, toolchain_name) 00183 test_build_success, test_build = build_tests(all_tests, [build_directory], build_directory, target, target_toolchain, 00184 clean=options.clean, 00185 report=build_report, 00186 properties=build_properties, 00187 macros=options.macros, 00188 verbose=options.verbose, 00189 jobs=options.jobs, 00190 continue_on_build_fail=options.continue_on_build_fail) 00191 00192 if not test_build_success: 00193 total_build_success = False 00194 print "Failed to build some tests, check build log for details" 00195 00196 test_builds.update(test_build) 00197 else: 00198 total_build_success = False 00199 break 00200 00201 # If a path to a test spec is provided, write it to a file 00202 if options.test_spec: 00203 test_spec_data = test_spec_from_test_builds(test_builds) 00204 00205 # Create the target dir for the test spec if necessary 00206 # mkdir will not create the dir if it already exists 00207 test_spec_dir = dirname(options.test_spec) 00208 if test_spec_dir: 00209 mkdir(test_spec_dir) 00210 00211 try: 00212 with open(options.test_spec, 'w') as f: 00213 f.write(json.dumps(test_spec_data, indent=2)) 00214 except IOError, e: 00215 print "[ERROR] Error writing test spec to file" 00216 print e 00217 00218 # If a path to a JUnit build report spec is provided, write it to a file 00219 if options.build_report_junit: 00220 report_exporter = ReportExporter(ResultExporterType.JUNIT) 00221 report_exporter.report_to_file(build_report, options.build_report_junit, test_suite_properties=build_properties) 00222 00223 print "\n\nCompleted in: (%.2f)s" % (time() - start) 00224 00225 print_report_exporter = ReportExporter(ResultExporterType.PRINT, package="build") 00226 status = print_report_exporter.report(build_report) 00227 00228 if status: 00229 sys.exit(0) 00230 else: 00231 sys.exit(1) 00232 00233 except KeyboardInterrupt, e: 00234 print "\n[CTRL+c] exit" 00235 except Exception,e: 00236 import traceback 00237 traceback.print_exc(file=sys.stdout) 00238 print "[ERROR] %s" % str(e) 00239 sys.exit(1)
Generated on Sun Jul 17 2022 08:25:20 by
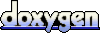