
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
build_api_test.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2016 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import unittest 00019 from collections import namedtuple 00020 from mock import patch, MagicMock 00021 from tools.build_api import prepare_toolchain, build_project, build_library,\ 00022 scan_resources 00023 from tools.toolchains import TOOLCHAINS 00024 00025 """ 00026 Tests for build_api.py 00027 """ 00028 make_mock_target = namedtuple( 00029 "Target", "init_hooks name features core supported_toolchains") 00030 00031 class BuildApiTests (unittest.TestCase): 00032 """ 00033 Test cases for Build Api 00034 """ 00035 00036 def setUp (self): 00037 """ 00038 Called before each test case 00039 00040 :return: 00041 """ 00042 self.target = "K64F" 00043 self.src_paths = ['.'] 00044 self.toolchain_name = "ARM" 00045 self.build_path = "build_path" 00046 00047 def tearDown (self): 00048 """ 00049 Called after each test case 00050 00051 :return: 00052 """ 00053 pass 00054 00055 @patch('tools.toolchains.arm.ARM_STD.parse_dependencies', 00056 return_value=["foo"]) 00057 @patch('tools.toolchains.mbedToolchain.need_update', 00058 side_effect=[i % 2 for i in range(3000)]) 00059 @patch('os.mkdir') 00060 @patch('tools.toolchains.exists', return_value=True) 00061 @patch('tools.toolchains.mbedToolchain.dump_build_profile') 00062 @patch('tools.utils.run_cmd', return_value=("", "", 0)) 00063 def test_always_complete_build(self, *_): 00064 with MagicMock() as notify: 00065 toolchain = prepare_toolchain(self.src_paths , self.build_path , self.target , 00066 self.toolchain_name , notify=notify) 00067 00068 res = scan_resources(self.src_paths , toolchain) 00069 00070 toolchain.RESPONSE_FILES=False 00071 toolchain.config_processed = True 00072 toolchain.config_file = "junk" 00073 toolchain.compile_sources(res) 00074 00075 assert any('percent' in msg[0] and msg[0]['percent'] == 100.0 00076 for _, msg, _ in notify.mock_calls if msg) 00077 00078 00079 @patch('tools.build_api.Config') 00080 def test_prepare_toolchain_app_config (self, mock_config_init): 00081 """ 00082 Test that prepare_toolchain uses app_config correctly 00083 00084 :param mock_config_init: mock of Config __init__ 00085 :return: 00086 """ 00087 app_config = "app_config" 00088 mock_target = make_mock_target(lambda _, __ : None, 00089 "Junk", [], "Cortex-M3", TOOLCHAINS) 00090 mock_config_init.return_value = namedtuple( 00091 "Config", "target has_regions name")(mock_target, False, None) 00092 00093 prepare_toolchain(self.src_paths , None, self.target , self.toolchain_name , 00094 app_config=app_config) 00095 00096 mock_config_init.assert_called_once_with(self.target , self.src_paths , 00097 app_config=app_config) 00098 00099 @patch('tools.build_api.Config') 00100 def test_prepare_toolchain_no_app_config (self, mock_config_init): 00101 """ 00102 Test that prepare_toolchain correctly deals with no app_config 00103 00104 :param mock_config_init: mock of Config __init__ 00105 :return: 00106 """ 00107 mock_target = make_mock_target(lambda _, __ : None, 00108 "Junk", [], "Cortex-M3", TOOLCHAINS) 00109 mock_config_init.return_value = namedtuple( 00110 "Config", "target has_regions name")(mock_target, False, None) 00111 00112 prepare_toolchain(self.src_paths , None, self.target , self.toolchain_name ) 00113 00114 mock_config_init.assert_called_once_with(self.target , self.src_paths , 00115 app_config=None) 00116 00117 @patch('tools.build_api.scan_resources') 00118 @patch('tools.build_api.mkdir') 00119 @patch('os.path.exists') 00120 @patch('tools.build_api.prepare_toolchain') 00121 def test_build_project_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00122 """ 00123 Test that build_project uses app_config correctly 00124 00125 :param mock_prepare_toolchain: mock of function prepare_toolchain 00126 :param mock_exists: mock of function os.path.exists 00127 :param _: mock of function mkdir (not tested) 00128 :param __: mock of function scan_resources (not tested) 00129 :return: 00130 """ 00131 app_config = "app_config" 00132 mock_exists.return_value = False 00133 mock_prepare_toolchain().link_program.return_value = 1, 2 00134 mock_prepare_toolchain().config = namedtuple( 00135 "Config", "has_regions name lib_config_data")(None, None, {}) 00136 00137 build_project(self.src_paths , self.build_path , self.target , 00138 self.toolchain_name , app_config=app_config) 00139 00140 args = mock_prepare_toolchain.call_args 00141 self.assertTrue('app_config' in args[1], 00142 "prepare_toolchain was not called with app_config") 00143 self.assertEqual(args[1]['app_config'], app_config, 00144 "prepare_toolchain was called with an incorrect app_config") 00145 00146 @patch('tools.build_api.scan_resources') 00147 @patch('tools.build_api.mkdir') 00148 @patch('os.path.exists') 00149 @patch('tools.build_api.prepare_toolchain') 00150 def test_build_project_no_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00151 """ 00152 Test that build_project correctly deals with no app_config 00153 00154 :param mock_prepare_toolchain: mock of function prepare_toolchain 00155 :param mock_exists: mock of function os.path.exists 00156 :param _: mock of function mkdir (not tested) 00157 :param __: mock of function scan_resources (not tested) 00158 :return: 00159 """ 00160 mock_exists.return_value = False 00161 # Needed for the unpacking of the returned value 00162 mock_prepare_toolchain().link_program.return_value = 1, 2 00163 mock_prepare_toolchain().config = namedtuple( 00164 "Config", "has_regions name lib_config_data")(None, None, {}) 00165 00166 build_project(self.src_paths , self.build_path , self.target , 00167 self.toolchain_name ) 00168 00169 args = mock_prepare_toolchain.call_args 00170 self.assertTrue('app_config' in args[1], 00171 "prepare_toolchain was not called with app_config") 00172 self.assertEqual(args[1]['app_config'], None, 00173 "prepare_toolchain was called with an incorrect app_config") 00174 00175 @patch('tools.build_api.scan_resources') 00176 @patch('tools.build_api.mkdir') 00177 @patch('os.path.exists') 00178 @patch('tools.build_api.prepare_toolchain') 00179 def test_build_library_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00180 """ 00181 Test that build_library uses app_config correctly 00182 00183 :param mock_prepare_toolchain: mock of function prepare_toolchain 00184 :param mock_exists: mock of function os.path.exists 00185 :param _: mock of function mkdir (not tested) 00186 :param __: mock of function scan_resources (not tested) 00187 :return: 00188 """ 00189 app_config = "app_config" 00190 mock_exists.return_value = False 00191 00192 build_library(self.src_paths , self.build_path , self.target , 00193 self.toolchain_name , app_config=app_config) 00194 00195 args = mock_prepare_toolchain.call_args 00196 self.assertTrue('app_config' in args[1], 00197 "prepare_toolchain was not called with app_config") 00198 self.assertEqual(args[1]['app_config'], app_config, 00199 "prepare_toolchain was called with an incorrect app_config") 00200 00201 @patch('tools.build_api.scan_resources') 00202 @patch('tools.build_api.mkdir') 00203 @patch('os.path.exists') 00204 @patch('tools.build_api.prepare_toolchain') 00205 def test_build_library_no_app_config (self, mock_prepare_toolchain, mock_exists, _, __): 00206 """ 00207 Test that build_library correctly deals with no app_config 00208 00209 :param mock_prepare_toolchain: mock of function prepare_toolchain 00210 :param mock_exists: mock of function os.path.exists 00211 :param _: mock of function mkdir (not tested) 00212 :param __: mock of function scan_resources (not tested) 00213 :return: 00214 """ 00215 mock_exists.return_value = False 00216 00217 build_library(self.src_paths , self.build_path , self.target , 00218 self.toolchain_name ) 00219 00220 args = mock_prepare_toolchain.call_args 00221 self.assertTrue('app_config' in args[1], 00222 "prepare_toolchain was not called with app_config") 00223 self.assertEqual(args[1]['app_config'], None, 00224 "prepare_toolchain was called with an incorrect app_config") 00225 00226 if __name__ == '__main__': 00227 unittest.main()
Generated on Sun Jul 17 2022 08:25:20 by
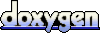