
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
build.py
00001 #! /usr/bin/env python2 00002 """ 00003 mbed SDK 00004 Copyright (c) 2011-2013 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 00018 LIBRARIES BUILD 00019 """ 00020 import sys 00021 from time import time 00022 from os.path import join, abspath, dirname 00023 00024 00025 # Be sure that the tools directory is in the search path 00026 ROOT = abspath(join(dirname(__file__), "..")) 00027 sys.path.insert(0, ROOT) 00028 00029 00030 from tools.toolchains import TOOLCHAINS, TOOLCHAIN_CLASSES, TOOLCHAIN_PATHS 00031 from tools.toolchains import mbedToolchain 00032 from tools.targets import TARGET_NAMES, TARGET_MAP 00033 from tools.options import get_default_options_parser 00034 from tools.options import extract_profile 00035 from tools.options import extract_mcus 00036 from tools.build_api import build_library, build_mbed_libs, build_lib 00037 from tools.build_api import mcu_toolchain_matrix 00038 from tools.build_api import print_build_results 00039 from tools.settings import CPPCHECK_CMD, CPPCHECK_MSG_FORMAT 00040 from utils import argparse_filestring_type, args_error 00041 from tools.settings import CPPCHECK_CMD, CPPCHECK_MSG_FORMAT, CLI_COLOR_MAP 00042 from utils import argparse_filestring_type, argparse_dir_not_parent 00043 00044 if __name__ == '__main__': 00045 start = time() 00046 00047 # Parse Options 00048 parser = get_default_options_parser() 00049 00050 parser.add_argument("--source", dest="source_dir", type=argparse_filestring_type, 00051 default=None, help="The source (input) directory", action="append") 00052 00053 parser.add_argument("--build", dest="build_dir", type=argparse_dir_not_parent(ROOT), 00054 default=None, help="The build (output) directory") 00055 00056 parser.add_argument("--no-archive", dest="no_archive", action="store_true", 00057 default=False, help="Do not produce archive (.ar) file, but rather .o") 00058 00059 # Extra libraries 00060 parser.add_argument("-r", "--rtos", 00061 action="store_true", 00062 dest="rtos", 00063 default=False, 00064 help="Compile the rtos") 00065 00066 parser.add_argument("--rpc", 00067 action="store_true", 00068 dest="rpc", 00069 default=False, 00070 help="Compile the rpc library") 00071 00072 parser.add_argument("-u", "--usb", 00073 action="store_true", 00074 dest="usb", 00075 default=False, 00076 help="Compile the USB Device library") 00077 00078 parser.add_argument("-d", "--dsp", 00079 action="store_true", 00080 dest="dsp", 00081 default=False, 00082 help="Compile the DSP library") 00083 00084 parser.add_argument( "--cpputest", 00085 action="store_true", 00086 dest="cpputest_lib", 00087 default=False, 00088 help="Compiles 'cpputest' unit test library (library should be on the same directory level as mbed repository)") 00089 00090 parser.add_argument("-D", 00091 action="append", 00092 dest="macros", 00093 help="Add a macro definition") 00094 00095 parser.add_argument("-S", "--supported-toolchains", 00096 action="store_true", 00097 dest="supported_toolchains", 00098 default=False, 00099 help="Displays supported matrix of MCUs and toolchains") 00100 00101 parser.add_argument('-f', '--filter', 00102 dest='general_filter_regex', 00103 default=None, 00104 help='For some commands you can use filter to filter out results') 00105 00106 parser.add_argument("-j", "--jobs", type=int, dest="jobs", 00107 default=0, help="Number of concurrent jobs. Default: 0/auto (based on host machine's number of CPUs)") 00108 parser.add_argument("-N", "--artifact-name", dest="artifact_name", 00109 default=None, help="The built project's name") 00110 00111 parser.add_argument("-v", "--verbose", 00112 action="store_true", 00113 dest="verbose", 00114 default=False, 00115 help="Verbose diagnostic output") 00116 00117 parser.add_argument("--silent", 00118 action="store_true", 00119 dest="silent", 00120 default=False, 00121 help="Silent diagnostic output (no copy, compile notification)") 00122 00123 parser.add_argument("-x", "--extra-verbose-notifications", 00124 action="store_true", 00125 dest="extra_verbose_notify", 00126 default=False, 00127 help="Makes compiler more verbose, CI friendly.") 00128 00129 options = parser.parse_args() 00130 00131 # Only prints matrix of supported toolchains 00132 if options.supported_toolchains: 00133 print mcu_toolchain_matrix(platform_filter=options.general_filter_regex) 00134 exit(0) 00135 00136 00137 # Get target list 00138 targets = extract_mcus(parser, options) if options.mcu else TARGET_NAMES 00139 00140 # Get toolchains list 00141 toolchains = options.tool if options.tool else TOOLCHAINS 00142 00143 if options.source_dir and not options.build_dir: 00144 args_error(parser, "argument --build is required by argument --source") 00145 00146 if options.color: 00147 # This import happens late to prevent initializing colorization when we don't need it 00148 import colorize 00149 if options.verbose: 00150 notify = mbedToolchain.print_notify_verbose 00151 else: 00152 notify = mbedToolchain.print_notify 00153 notify = colorize.print_in_color_notifier(CLI_COLOR_MAP, notify) 00154 else: 00155 notify = None 00156 00157 # Get libraries list 00158 libraries = [] 00159 00160 # Additional Libraries 00161 if options.rpc: 00162 libraries.extend(["rpc"]) 00163 if options.usb: 00164 libraries.append("usb") 00165 if options.dsp: 00166 libraries.extend(["dsp"]) 00167 if options.cpputest_lib: 00168 libraries.extend(["cpputest"]) 00169 00170 # Build results 00171 failures = [] 00172 successes = [] 00173 skipped = [] 00174 00175 for toolchain in toolchains: 00176 if not TOOLCHAIN_CLASSES[toolchain].check_executable(): 00177 search_path = TOOLCHAIN_PATHS[toolchain] or "No path set" 00178 args_error(parser, "Could not find executable for %s.\n" 00179 "Currently set search path: %s" 00180 % (toolchain, search_path)) 00181 00182 for toolchain in toolchains: 00183 for target in targets: 00184 tt_id = "%s::%s" % (toolchain, target) 00185 if toolchain not in TARGET_MAP[target].supported_toolchains: 00186 # Log this later 00187 print "%s skipped: toolchain not supported" % tt_id 00188 skipped.append(tt_id) 00189 else: 00190 try: 00191 mcu = TARGET_MAP[target] 00192 profile = extract_profile(parser, options, toolchain) 00193 if options.source_dir: 00194 lib_build_res = build_library(options.source_dir, options.build_dir, mcu, toolchain, 00195 extra_verbose=options.extra_verbose_notify, 00196 verbose=options.verbose, 00197 silent=options.silent, 00198 jobs=options.jobs, 00199 clean=options.clean, 00200 archive=(not options.no_archive), 00201 macros=options.macros, 00202 name=options.artifact_name, 00203 build_profile=profile) 00204 else: 00205 lib_build_res = build_mbed_libs(mcu, toolchain, 00206 extra_verbose=options.extra_verbose_notify, 00207 verbose=options.verbose, 00208 silent=options.silent, 00209 jobs=options.jobs, 00210 clean=options.clean, 00211 macros=options.macros, 00212 build_profile=profile) 00213 00214 for lib_id in libraries: 00215 build_lib(lib_id, mcu, toolchain, 00216 extra_verbose=options.extra_verbose_notify, 00217 verbose=options.verbose, 00218 silent=options.silent, 00219 clean=options.clean, 00220 macros=options.macros, 00221 jobs=options.jobs, 00222 build_profile=profile) 00223 if lib_build_res: 00224 successes.append(tt_id) 00225 else: 00226 skipped.append(tt_id) 00227 except Exception, e: 00228 if options.verbose: 00229 import traceback 00230 traceback.print_exc(file=sys.stdout) 00231 sys.exit(1) 00232 failures.append(tt_id) 00233 print e 00234 00235 00236 # Write summary of the builds 00237 print 00238 print "Completed in: (%.2f)s" % (time() - start) 00239 print 00240 00241 for report, report_name in [(successes, "Build successes:"), 00242 (skipped, "Build skipped:"), 00243 (failures, "Build failures:"), 00244 ]: 00245 if report: 00246 print print_build_results(report, report_name), 00247 00248 if failures: 00249 sys.exit(1)
Generated on Sun Jul 17 2022 08:25:20 by
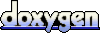