
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
box_config.h
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_BOX_CONFIG_H__ 00018 #define __UVISOR_API_BOX_CONFIG_H__ 00019 00020 #include "api/inc/uvisor_exports.h" 00021 #include "api/inc/debug_exports.h" 00022 #include "api/inc/page_allocator_exports.h" 00023 #include "api/inc/rpc_exports.h" 00024 #include <stddef.h> 00025 #include <stdint.h> 00026 #include <sys/reent.h> 00027 00028 UVISOR_EXTERN const uint32_t __uvisor_mode; 00029 UVISOR_EXTERN void const * const public_box_cfg_ptr; 00030 00031 /* All pointers in the box index need to be 4-byte aligned. 00032 * We therefore also need to round up all sizes to 4-byte multiples to 00033 * provide the space to be able to align the pointers to 4-bytes. */ 00034 #define __UVISOR_BOX_ROUND_4(size) \ 00035 (((size) + 3UL) & ~3UL) 00036 00037 #define UVISOR_DISABLED 0 00038 #define UVISOR_PERMISSIVE 1 00039 #define UVISOR_ENABLED 2 00040 00041 #define UVISOR_SET_MODE(mode) \ 00042 UVISOR_SET_MODE_ACL_COUNT(mode, NULL, 0) 00043 00044 #define UVISOR_SET_MODE_ACL(mode, acl_list) \ 00045 UVISOR_SET_MODE_ACL_COUNT(mode, acl_list, UVISOR_ARRAY_COUNT(acl_list)) 00046 00047 #define UVISOR_SET_MODE_ACL_COUNT(mode, acl_list, acl_list_count) \ 00048 uint8_t __attribute__((section(".keep.uvisor.bss.boxes"), aligned(32))) __reserved_stack[UVISOR_STACK_BAND_SIZE]; \ 00049 \ 00050 UVISOR_EXTERN const uint32_t __uvisor_mode = (mode); \ 00051 \ 00052 static const __attribute__((section(".keep.uvisor.cfgtbl"), aligned(4))) UvisorBoxConfig public_box_cfg = { \ 00053 UVISOR_BOX_MAGIC, \ 00054 UVISOR_BOX_VERSION, \ 00055 { \ 00056 sizeof(RtxBoxIndex), \ 00057 0, \ 00058 0, \ 00059 sizeof(uvisor_rpc_t), \ 00060 sizeof(uvisor_ipc_t), \ 00061 0, \ 00062 }, \ 00063 0, \ 00064 NULL, \ 00065 NULL, \ 00066 acl_list, \ 00067 acl_list_count \ 00068 }; \ 00069 \ 00070 UVISOR_EXTERN const __attribute__((section(".keep.uvisor.cfgtbl_ptr_first"), aligned(4))) void * const public_box_cfg_ptr = &public_box_cfg; 00071 00072 /* Creates a global page heap with at least `minimum_number_of_pages` each of size `page_size` in bytes. 00073 * The total page heap size is at least `minimum_number_of_pages * page_size`. */ 00074 #define UVISOR_SET_PAGE_HEAP(page_size, minimum_number_of_pages) \ 00075 const uint32_t __uvisor_page_size = (page_size); \ 00076 uint8_t __attribute__((section(".keep.uvisor.page_heap"))) \ 00077 public_page_heap_reserved[ (page_size) * (minimum_number_of_pages) ] 00078 00079 00080 /* this macro selects an overloaded macro (variable number of arguments) */ 00081 #define __UVISOR_BOX_MACRO(_1, _2, _3, _4, NAME, ...) NAME 00082 00083 #define __UVISOR_BOX_CONFIG(box_name, acl_list, acl_list_count, stack_size, context_size) \ 00084 \ 00085 uint8_t __attribute__((section(".keep.uvisor.bss.boxes"), aligned(32))) \ 00086 box_name ## _reserved[ \ 00087 UVISOR_STACK_SIZE_ROUND( \ 00088 ( \ 00089 (UVISOR_MIN_STACK(stack_size) + \ 00090 __UVISOR_BOX_ROUND_4(context_size) + \ 00091 __UVISOR_BOX_ROUND_4(__uvisor_box_heapsize) + \ 00092 __UVISOR_BOX_ROUND_4(sizeof(RtxBoxIndex)) + \ 00093 __UVISOR_BOX_ROUND_4(sizeof(uvisor_rpc_outgoing_message_queue_t)) + \ 00094 __UVISOR_BOX_ROUND_4(sizeof(uvisor_rpc_incoming_message_queue_t)) + \ 00095 __UVISOR_BOX_ROUND_4(sizeof(uvisor_rpc_fn_group_queue_t)) + \ 00096 __UVISOR_BOX_ROUND_4(sizeof(struct _reent)) \ 00097 ) \ 00098 * 8) \ 00099 / 6)]; \ 00100 \ 00101 static const __attribute__((section(".keep.uvisor.cfgtbl"), aligned(4))) UvisorBoxConfig box_name ## _cfg = { \ 00102 UVISOR_BOX_MAGIC, \ 00103 UVISOR_BOX_VERSION, \ 00104 { \ 00105 sizeof(RtxBoxIndex), \ 00106 context_size, \ 00107 sizeof(struct _reent), \ 00108 sizeof(uvisor_rpc_t), \ 00109 sizeof(uvisor_ipc_t), \ 00110 __uvisor_box_heapsize, \ 00111 }, \ 00112 UVISOR_MIN_STACK(stack_size), \ 00113 __uvisor_box_lib_config, \ 00114 __uvisor_box_namespace, \ 00115 acl_list, \ 00116 acl_list_count \ 00117 }; \ 00118 \ 00119 UVISOR_EXTERN const __attribute__((section(".keep.uvisor.cfgtbl_ptr"), aligned(4))) void * const box_name ## _cfg_ptr = &box_name ## _cfg; 00120 00121 #define UVISOR_BOX_EXTERN(box_name) \ 00122 UVISOR_EXTERN const __attribute__((section(".keep.uvisor.cfgtbl_ptr"), aligned(4))) void * const box_name ## _cfg_ptr; 00123 00124 #define __UVISOR_BOX_CONFIG_NOCONTEXT(box_name, acl_list, stack_size) \ 00125 __UVISOR_BOX_CONFIG(box_name, acl_list, UVISOR_ARRAY_COUNT(acl_list), stack_size, 0) \ 00126 00127 #define __UVISOR_BOX_CONFIG_CONTEXT(box_name, acl_list, stack_size, context_type) \ 00128 __UVISOR_BOX_CONFIG(box_name, acl_list, UVISOR_ARRAY_COUNT(acl_list), stack_size, sizeof(context_type)) \ 00129 UVISOR_EXTERN context_type *const *const __uvisor_ps; 00130 00131 #define __UVISOR_BOX_CONFIG_NOACL(box_name, stack_size, context_type) \ 00132 __UVISOR_BOX_CONFIG(box_name, NULL, 0, stack_size, sizeof(context_type)) \ 00133 UVISOR_EXTERN context_type *const *const __uvisor_ps; 00134 00135 #define __UVISOR_BOX_CONFIG_NOACL_NOCONTEXT(box_name, stack_size) \ 00136 __UVISOR_BOX_CONFIG(box_name, NULL, 0, stack_size, 0) 00137 00138 #define UVISOR_BOX_CONFIG_ACL(...) \ 00139 __UVISOR_BOX_MACRO(__VA_ARGS__, __UVISOR_BOX_CONFIG_CONTEXT, \ 00140 __UVISOR_BOX_CONFIG_NOCONTEXT, \ 00141 __UVISOR_BOX_CONFIG_NOACL_NOCONTEXT)(__VA_ARGS__) 00142 00143 #define UVISOR_BOX_CONFIG_CTX(...) \ 00144 __UVISOR_BOX_MACRO(__VA_ARGS__, __UVISOR_BOX_CONFIG_CONTEXT, \ 00145 __UVISOR_BOX_CONFIG_NOACL, \ 00146 __UVISOR_BOX_CONFIG_NOACL_NOCONTEXT)(__VA_ARGS__) 00147 00148 #define UVISOR_BOX_CONFIG(...) \ 00149 UVISOR_BOX_CONFIG_ACL(__VA_ARGS__) 00150 00151 /* Use this macro before box defintion (for example, UVISOR_BOX_CONFIG) to 00152 * define the name of your box. If you don't want a name, use this macro with 00153 * box_namespace as NULL. */ 00154 #define UVISOR_BOX_NAMESPACE(box_namespace) \ 00155 static const char *const __uvisor_box_namespace = box_namespace 00156 00157 /* Use this macro before UVISOR_BOX_CONFIG to define the function the main 00158 * thread of your box will use for its body. If you don't want a main thread, 00159 * too bad: you have to have one. */ 00160 #define UVISOR_BOX_MAIN(function, priority, stack_size) \ 00161 static const uvisor_box_main_t __uvisor_box_main = { \ 00162 function, \ 00163 priority, \ 00164 stack_size, \ 00165 }; \ 00166 static const void * const __uvisor_box_lib_config = &__uvisor_box_main; 00167 00168 #define UVISOR_BOX_HEAPSIZE(heap_size) \ 00169 static const uint32_t __uvisor_box_heapsize = heap_size; 00170 00171 #define __uvisor_ctx (((UvisorBoxIndex *) __uvisor_ps)->bss.address_of.context) 00172 00173 00174 /* Use this macro after calling the box configuration macro, in order to register your box as a debug box. 00175 * It will create a valid debug driver struct with the halt_error_func parameter as its halt_error() function */ 00176 #define UVISOR_DEBUG_DRIVER(box_name, halt_error_func) \ 00177 UVISOR_EXTERN TUvisorDebugDriver const __uvisor_debug_driver; \ 00178 TUvisorDebugDriver const __uvisor_debug_driver = { \ 00179 UVISOR_DEBUG_BOX_MAGIC, \ 00180 UVISOR_DEBUG_BOX_VERSION, \ 00181 &box_name ## _cfg, \ 00182 halt_error_func \ 00183 }; 00184 00185 /* Use this macro after calling the box configuration macro, in order to 00186 * register the public box as a debug box. */ 00187 #define UVISOR_PUBLIC_BOX_DEBUG_DRIVER(halt_error_func) \ 00188 UVISOR_DEBUG_DRIVER(public_box, halt_error_func) 00189 00190 00191 #endif /* __UVISOR_API_BOX_CONFIG_H__ */
Generated on Sun Jul 17 2022 08:25:20 by
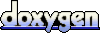