
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
arm_hal_nvm.h
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /* 00018 * Nanostack NVM API. 00019 * 00020 * After NVM initialization (platform_nvm_init(...)) a user can: 00021 * -create key to the NVM by using method platform_nvm_key_create(...) 00022 * -write data to the NVM by using method platform_nvm_write(...) 00023 * -read data from the NVM by using method platform_nvm_read(...) 00024 * -delete the created key by using platform_nvm_key_delete(...) 00025 * -store changed data to the underlying backing store by using method platform_nvm_flush(...). 00026 * 00027 * This NVM API is asynchronous. If API function returns PLATFORM_NVM_OK then provided callback function will be 00028 * called once operation is completed. Callback function carries status parameter that indicates status of the 00029 * operation. If platform API function returns error code then callback will not be called. A new operation can not 00030 * be started until the previous operation has completed. 00031 * 00032 */ 00033 00034 #ifndef _PLATFORM_NVM_H_ 00035 #define _PLATFORM_NVM_H_ 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 /* Enumeration for nvm API function return values */ 00042 typedef enum { 00043 PLATFORM_NVM_OK, 00044 PLATFORM_NVM_KEY_NOT_FOUND, 00045 PLATFORM_NVM_ERROR 00046 } platform_nvm_status; 00047 00048 /** \brief Client registered API callback type. 00049 * 00050 * \param status operation completion status 00051 * \param context client provided context that was used when requesting operation 00052 */ 00053 typedef void (nvm_callback)(platform_nvm_status status, void *context); 00054 00055 /** \brief Initialize NVM. Allocates module static resources and initializes underlying NMV. 00056 * 00057 * \param callback callback function that will be called once initialization is completed. 00058 * \param context A client registered context that will be supplied as an argument to the callback when called. 00059 * 00060 * \return NVM_OK if initialization is in progress and callback will be called. 00061 * \return NVM_ERROR if initialization failed and callback will not be called. 00062 */ 00063 platform_nvm_status platform_nvm_init(nvm_callback *callback, void *context); 00064 00065 /** \brief Free resources reserved by NVM init. 00066 * 00067 * \param callback callback function that will be called once deinitialization is completed. 00068 * \param context A client registered context that will be supplied as an argument to the callback when called. 00069 * 00070 * \return NVM_OK if initialization is in progress and callback will be called. 00071 * \return NVM_ERROR if initialization failed and callback will not be called. 00072 */ 00073 platform_nvm_status platform_nvm_finalize(nvm_callback *callback, void *context); 00074 00075 /** \brief Create key to the NMV. If the key exists then the key will be recreated with new length. 00076 * 00077 * \param callback callback function that will be called once key creation is finished 00078 * \param key_name name of the key to be created 00079 * \param value_len length of data reserved for the key 00080 * \param flags reserved for future use 00081 * \param context A client registered context that will be supplied as an argument to the callback when called 00082 * 00083 * \return NVM_OK if key creation is in progress and callback will be called. 00084 * \return NVM_ERROR if key creation failed and callback will not be called. 00085 * \return Provided callback function will be called once operation is completed. 00086 */ 00087 platform_nvm_status platform_nvm_key_create(nvm_callback *callback, const char *key_name, uint16_t value_len, uint32_t flags, void *context); 00088 00089 /** \brief Delete key from NMV. 00090 * 00091 * \param callback callback function that will be called once key creation is finished 00092 * \param key_name name of the key to be deleted 00093 * \param context A client registered context that will be supplied as an argument to the callback when called 00094 * 00095 * \return NVM_OK if key creation is in progress and callback will be called. 00096 * \return NVM_ERROR if key creation failed and callback will not be called. 00097 * \return Provided callback function will be called once operation is completed. 00098 */ 00099 platform_nvm_status platform_nvm_key_delete(nvm_callback *callback, const char *key_name, void *context); 00100 00101 /** \brief Write data to the NVM. Data will be truncated if the key does not have enough space for the data. 00102 * 00103 * \param callback callback function that will be called once writing is complete 00104 * \param key_name name of the key where data will be written 00105 * \param data buffer to data to be write. Data must be valid until callback is called. 00106 * \param data_len [IN] length of data in bytes. [OUT] number of bytes written. Argument must be valid until a callback is called. 00107 * \param context A client registered context that will be supplied as an argument to the callback when called. 00108 * 00109 * \return NVM_OK if data writing is in progress and callback will be called. 00110 * \return NVM_ERROR if data writing failed and callback will not be called. 00111 * \return Provided callback function will be called once operation is completed. 00112 */ 00113 platform_nvm_status platform_nvm_write(nvm_callback *callback, const char *key_name, const void *data, uint16_t *data_len, void *context); 00114 00115 /** \brief Read key value from the NVM. 00116 * 00117 * \param callback callback function that will be called once reading is complete 00118 * \param key_name name of the key whose data will be read 00119 * \param buf buffer where data will be copied. Argument must be valid until a callback is called. 00120 * \param buf_len [IN] provided buffer length in bytes. [OUT] bytes read. Argument must be valid until callback is called. 00121 * \param context A client registered context that will be supplied as an argument to the callback when called. 00122 * 00123 * \return NVM_OK if data reading is in progress and callback will be called. 00124 * \return NVM_ERROR if data reading failed and callback will not be called. 00125 * \return Provided callback function will be called once operation is completed. 00126 */ 00127 platform_nvm_status platform_nvm_read(nvm_callback *callback, const char *key_name, void *buf, uint16_t *buf_len, void *context); 00128 00129 /** \brief Store changed data to the backing store. This operation will write changed data to SRAM/Flash. 00130 * 00131 * \param callback callback function that will be called once flushing is complete 00132 * \param context A client registered context that will be supplied as an argument to the callback when called. 00133 * 00134 * \return NVM_OK if data flushing is in progress and callback will be called. 00135 * \return NVM_ERROR if data flushing failed and callback will not be called. 00136 * \return Provided callback function will be called once operation is completed. 00137 */ 00138 platform_nvm_status platform_nvm_flush(nvm_callback *callback, void *context); 00139 00140 #ifdef __cplusplus 00141 } 00142 #endif 00143 #endif /* _PLATFORM_NVM_H_ */ 00144
Generated on Sun Jul 17 2022 08:25:19 by
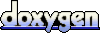