
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
arch.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Support for different processor and compiler architectures 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Adam Dunkels <adam@sics.se> 00035 * 00036 */ 00037 #ifndef LWIP_HDR_ARCH_H 00038 #define LWIP_HDR_ARCH_H 00039 00040 #ifndef LITTLE_ENDIAN 00041 #define LITTLE_ENDIAN 1234 00042 #endif 00043 00044 #ifndef BIG_ENDIAN 00045 #define BIG_ENDIAN 4321 00046 #endif 00047 00048 #include "arch/cc.h" 00049 00050 /** 00051 * @defgroup compiler_abstraction Compiler/platform abstraction 00052 * @ingroup sys_layer 00053 * All defines related to this section must not be placed in lwipopts.h, 00054 * but in arch/cc.h! 00055 * These options cannot be \#defined in lwipopts.h since they are not options 00056 * of lwIP itself, but options of the lwIP port to your system. 00057 * @{ 00058 */ 00059 00060 /** Define the byte order of the system. 00061 * Needed for conversion of network data to host byte order. 00062 * Allowed values: LITTLE_ENDIAN and BIG_ENDIAN 00063 */ 00064 #ifndef BYTE_ORDER 00065 #define BYTE_ORDER LITTLE_ENDIAN 00066 #endif 00067 00068 /** Define random number generator function of your system */ 00069 #ifdef __DOXYGEN__ 00070 #define LWIP_RAND() ((u32_t)rand()) 00071 #endif 00072 00073 /** Platform specific diagnostic output.\n 00074 * Note the default implementation pulls in printf, which may 00075 * in turn pull in a lot of standard libary code. In resource-constrained 00076 * systems, this should be defined to something less resource-consuming. 00077 */ 00078 #ifndef LWIP_PLATFORM_DIAG 00079 #define LWIP_PLATFORM_DIAG(x) do {printf x;} while(0) 00080 #include <stdio.h> 00081 #include <stdlib.h> 00082 #endif 00083 00084 /** Platform specific assertion handling.\n 00085 * Note the default implementation pulls in printf, fflush and abort, which may 00086 * in turn pull in a lot of standard libary code. In resource-constrained 00087 * systems, this should be defined to something less resource-consuming. 00088 */ 00089 #ifndef LWIP_PLATFORM_ASSERT 00090 #define LWIP_PLATFORM_ASSERT(x) do {printf("Assertion \"%s\" failed at line %d in %s\n", \ 00091 x, __LINE__, __FILE__); fflush(NULL); abort();} while(0) 00092 #include <stdio.h> 00093 #include <stdlib.h> 00094 #endif 00095 00096 /** Define this to 1 in arch/cc.h of your port if you do not want to 00097 * include stddef.h header to get size_t. You need to typedef size_t 00098 * by yourself in this case. 00099 */ 00100 #ifndef LWIP_NO_STDDEF_H 00101 #define LWIP_NO_STDDEF_H 0 00102 #endif 00103 00104 #if !LWIP_NO_STDDEF_H 00105 #include <stddef.h> /* for size_t */ 00106 #endif 00107 00108 /** Define this to 1 in arch/cc.h of your port if your compiler does not provide 00109 * the stdint.h header. You need to typedef the generic types listed in 00110 * lwip/arch.h yourself in this case (u8_t, u16_t...). 00111 */ 00112 #ifndef LWIP_NO_STDINT_H 00113 #define LWIP_NO_STDINT_H 0 00114 #endif 00115 00116 /* Define generic types used in lwIP */ 00117 #if !LWIP_NO_STDINT_H 00118 #include <stdint.h> 00119 typedef uint8_t u8_t; 00120 typedef int8_t s8_t; 00121 typedef uint16_t u16_t; 00122 typedef int16_t s16_t; 00123 typedef uint32_t u32_t; 00124 typedef int32_t s32_t; 00125 typedef uintptr_t mem_ptr_t; 00126 #endif 00127 00128 /** Define this to 1 in arch/cc.h of your port if your compiler does not provide 00129 * the inttypes.h header. You need to define the format strings listed in 00130 * lwip/arch.h yourself in this case (X8_F, U16_F...). 00131 */ 00132 #ifndef LWIP_NO_INTTYPES_H 00133 #define LWIP_NO_INTTYPES_H 0 00134 #endif 00135 00136 /* Define (sn)printf formatters for these lwIP types */ 00137 #if !LWIP_NO_INTTYPES_H 00138 #include <inttypes.h> 00139 #ifndef X8_F 00140 #define X8_F "02" PRIx8 00141 #endif 00142 #ifndef U16_F 00143 #define U16_F PRIu16 00144 #endif 00145 #ifndef S16_F 00146 #define S16_F PRId16 00147 #endif 00148 #ifndef X16_F 00149 #define X16_F PRIx16 00150 #endif 00151 #ifndef U32_F 00152 #define U32_F PRIu32 00153 #endif 00154 #ifndef S32_F 00155 #define S32_F PRId32 00156 #endif 00157 #ifndef X32_F 00158 #define X32_F PRIx32 00159 #endif 00160 #ifndef SZT_F 00161 #define SZT_F PRIuPTR 00162 #endif 00163 #endif 00164 00165 /** Define this to 1 in arch/cc.h of your port if your compiler does not provide 00166 * the limits.h header. You need to define the type limits yourself in this case 00167 * (e.g. INT_MAX). 00168 */ 00169 #ifndef LWIP_NO_LIMITS_H 00170 #define LWIP_NO_LIMITS_H 0 00171 #endif 00172 00173 /* Include limits.h? */ 00174 #if !LWIP_NO_LIMITS_H 00175 #include <limits.h> 00176 #endif 00177 00178 /** C++ const_cast<target_type>(val) equivalent to remove constness from a value (GCC -Wcast-qual) */ 00179 #ifndef LWIP_CONST_CAST 00180 #define LWIP_CONST_CAST(target_type, val) ((target_type)((ptrdiff_t)val)) 00181 #endif 00182 00183 /** Get rid of alignment cast warnings (GCC -Wcast-align) */ 00184 #ifndef LWIP_ALIGNMENT_CAST 00185 #define LWIP_ALIGNMENT_CAST(target_type, val) LWIP_CONST_CAST(target_type, val) 00186 #endif 00187 00188 /** Get rid of warnings related to pointer-to-numeric and vice-versa casts, 00189 * e.g. "conversion from 'u8_t' to 'void *' of greater size" 00190 */ 00191 #ifndef LWIP_PTR_NUMERIC_CAST 00192 #define LWIP_PTR_NUMERIC_CAST(target_type, val) LWIP_CONST_CAST(target_type, val) 00193 #endif 00194 00195 /** Allocates a memory buffer of specified size that is of sufficient size to align 00196 * its start address using LWIP_MEM_ALIGN. 00197 * You can declare your own version here e.g. to enforce alignment without adding 00198 * trailing padding bytes (see LWIP_MEM_ALIGN_BUFFER) or your own section placement 00199 * requirements.\n 00200 * e.g. if you use gcc and need 32 bit alignment:\n 00201 * \#define LWIP_DECLARE_MEMORY_ALIGNED(variable_name, size) u8_t variable_name[size] \_\_attribute\_\_((aligned(4)))\n 00202 * or more portable:\n 00203 * \#define LWIP_DECLARE_MEMORY_ALIGNED(variable_name, size) u32_t variable_name[(size + sizeof(u32_t) - 1) / sizeof(u32_t)] 00204 */ 00205 #ifndef LWIP_DECLARE_MEMORY_ALIGNED 00206 #define LWIP_DECLARE_MEMORY_ALIGNED(variable_name, size) u8_t variable_name[LWIP_MEM_ALIGN_BUFFER(size)] 00207 #endif 00208 00209 /** Calculate memory size for an aligned buffer - returns the next highest 00210 * multiple of MEM_ALIGNMENT (e.g. LWIP_MEM_ALIGN_SIZE(3) and 00211 * LWIP_MEM_ALIGN_SIZE(4) will both yield 4 for MEM_ALIGNMENT == 4). 00212 */ 00213 #ifndef LWIP_MEM_ALIGN_SIZE 00214 #define LWIP_MEM_ALIGN_SIZE(size) (((size) + MEM_ALIGNMENT - 1U) & ~(MEM_ALIGNMENT-1U)) 00215 #endif 00216 00217 /** Calculate safe memory size for an aligned buffer when using an unaligned 00218 * type as storage. This includes a safety-margin on (MEM_ALIGNMENT - 1) at the 00219 * start (e.g. if buffer is u8_t[] and actual data will be u32_t*) 00220 */ 00221 #ifndef LWIP_MEM_ALIGN_BUFFER 00222 #define LWIP_MEM_ALIGN_BUFFER(size) (((size) + MEM_ALIGNMENT - 1U)) 00223 #endif 00224 00225 /** Align a memory pointer to the alignment defined by MEM_ALIGNMENT 00226 * so that ADDR % MEM_ALIGNMENT == 0 00227 */ 00228 #ifndef LWIP_MEM_ALIGN 00229 #define LWIP_MEM_ALIGN(addr) ((void *)(((mem_ptr_t)(addr) + MEM_ALIGNMENT - 1) & ~(mem_ptr_t)(MEM_ALIGNMENT-1))) 00230 #endif 00231 00232 #ifdef __cplusplus 00233 extern "C" { 00234 #endif 00235 00236 /** Packed structs support. 00237 * Placed BEFORE declaration of a packed struct.\n 00238 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00239 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00240 */ 00241 #ifndef PACK_STRUCT_BEGIN 00242 #define PACK_STRUCT_BEGIN 00243 #endif /* PACK_STRUCT_BEGIN */ 00244 00245 /** Packed structs support. 00246 * Placed AFTER declaration of a packed struct.\n 00247 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00248 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00249 */ 00250 #ifndef PACK_STRUCT_END 00251 #define PACK_STRUCT_END 00252 #endif /* PACK_STRUCT_END */ 00253 00254 /** Packed structs support. 00255 * Placed between end of declaration of a packed struct and trailing semicolon.\n 00256 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00257 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00258 */ 00259 #ifndef PACK_STRUCT_STRUCT 00260 #if defined(__GNUC__) || defined(__clang__) 00261 #define PACK_STRUCT_STRUCT __attribute__((packed)) 00262 #else 00263 #define PACK_STRUCT_STRUCT 00264 #endif 00265 #endif /* PACK_STRUCT_STRUCT */ 00266 00267 /** Packed structs support. 00268 * Wraps u32_t and u16_t members.\n 00269 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00270 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00271 */ 00272 #ifndef PACK_STRUCT_FIELD 00273 #define PACK_STRUCT_FIELD(x) x 00274 #endif /* PACK_STRUCT_FIELD */ 00275 00276 /** Packed structs support. 00277 * Wraps u8_t members, where some compilers warn that packing is not necessary.\n 00278 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00279 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00280 */ 00281 #ifndef PACK_STRUCT_FLD_8 00282 #define PACK_STRUCT_FLD_8(x) PACK_STRUCT_FIELD(x) 00283 #endif /* PACK_STRUCT_FLD_8 */ 00284 00285 /** Packed structs support. 00286 * Wraps members that are packed structs themselves, where some compilers warn that packing is not necessary.\n 00287 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00288 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00289 */ 00290 #ifndef PACK_STRUCT_FLD_S 00291 #define PACK_STRUCT_FLD_S(x) PACK_STRUCT_FIELD(x) 00292 #endif /* PACK_STRUCT_FLD_S */ 00293 00294 /** Packed structs support using \#include files before and after struct to be packed.\n 00295 * The file included BEFORE the struct is "arch/bpstruct.h".\n 00296 * The file included AFTER the struct is "arch/epstruct.h".\n 00297 * This can be used to implement struct packing on MS Visual C compilers, see 00298 * the Win32 port in the lwIP contrib repository for reference. 00299 * For examples of packed struct declarations, see include/lwip/prot/ subfolder.\n 00300 * A port to GCC/clang is included in lwIP, if you use these compilers there is nothing to do here. 00301 */ 00302 #ifdef __DOXYGEN__ 00303 #define PACK_STRUCT_USE_INCLUDES 00304 #endif 00305 00306 /** Eliminates compiler warning about unused arguments (GCC -Wextra -Wunused). */ 00307 #ifndef LWIP_UNUSED_ARG 00308 #define LWIP_UNUSED_ARG(x) (void)x 00309 #endif /* LWIP_UNUSED_ARG */ 00310 00311 /** 00312 * @} 00313 */ 00314 00315 #ifdef __cplusplus 00316 } 00317 #endif 00318 00319 #endif /* LWIP_HDR_ARCH_H */
Generated on Sun Jul 17 2022 08:25:18 by
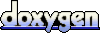