
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
api_test.py
00001 """Tests for the toolchain sub-system""" 00002 import sys 00003 import os 00004 from string import printable 00005 from copy import deepcopy 00006 from mock import MagicMock, patch 00007 from hypothesis import given, settings 00008 from hypothesis.strategies import text, lists, fixed_dictionaries, booleans 00009 00010 ROOT = os.path.abspath(os.path.join(os.path.dirname(__file__), "..", "..", 00011 "..")) 00012 sys.path.insert(0, ROOT) 00013 00014 from tools.toolchains import TOOLCHAIN_CLASSES, LEGACY_TOOLCHAIN_NAMES,\ 00015 Resources, TOOLCHAIN_PATHS, mbedToolchain 00016 from tools.targets import TARGET_MAP 00017 00018 def test_instantiation (): 00019 """Test that all exported toolchain may be instantiated""" 00020 for name, tc_class in TOOLCHAIN_CLASSES.items(): 00021 cls = tc_class(TARGET_MAP["K64F"]) 00022 assert name == cls.name or\ 00023 name == LEGACY_TOOLCHAIN_NAMES[cls.name] 00024 00025 ALPHABET = [char for char in printable if char not in [u'.', u'/']] 00026 00027 @given(fixed_dictionaries({ 00028 'common': lists(text()), 00029 'c': lists(text()), 00030 'cxx': lists(text()), 00031 'asm': lists(text()), 00032 'ld': lists(text())}), 00033 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00034 def test_toolchain_profile_c (profile, source_file): 00035 """Test that the appropriate profile parameters are passed to the 00036 C compiler""" 00037 filename = deepcopy(source_file) 00038 filename[-1] += ".c" 00039 to_compile = os.path.join(*filename) 00040 with patch('os.mkdir') as _mkdir: 00041 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00042 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00043 toolchain.inc_md5 = "" 00044 toolchain.build_dir = "" 00045 toolchain.config = MagicMock(app_config_location=None) 00046 for parameter in profile['c'] + profile['common']: 00047 assert any(parameter in cmd for cmd in toolchain.cc), \ 00048 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00049 parameter) 00050 compile_command = toolchain.compile_command(to_compile, 00051 to_compile + ".o", []) 00052 for parameter in profile['c'] + profile['common']: 00053 assert any(parameter in cmd for cmd in compile_command), \ 00054 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00055 parameter) 00056 00057 @given(fixed_dictionaries({ 00058 'common': lists(text()), 00059 'c': lists(text()), 00060 'cxx': lists(text()), 00061 'asm': lists(text()), 00062 'ld': lists(text())}), 00063 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00064 def test_toolchain_profile_cpp (profile, source_file): 00065 """Test that the appropriate profile parameters are passed to the 00066 C++ compiler""" 00067 filename = deepcopy(source_file) 00068 filename[-1] += ".cpp" 00069 to_compile = os.path.join(*filename) 00070 with patch('os.mkdir') as _mkdir: 00071 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00072 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00073 toolchain.inc_md5 = "" 00074 toolchain.build_dir = "" 00075 toolchain.config = MagicMock(app_config_location=None) 00076 for parameter in profile['cxx'] + profile['common']: 00077 assert any(parameter in cmd for cmd in toolchain.cppc), \ 00078 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00079 parameter) 00080 compile_command = toolchain.compile_command(to_compile, 00081 to_compile + ".o", []) 00082 for parameter in profile['cxx'] + profile['common']: 00083 assert any(parameter in cmd for cmd in compile_command), \ 00084 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00085 parameter) 00086 00087 @given(fixed_dictionaries({ 00088 'common': lists(text()), 00089 'c': lists(text()), 00090 'cxx': lists(text()), 00091 'asm': lists(text()), 00092 'ld': lists(text())}), 00093 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00094 def test_toolchain_profile_asm (profile, source_file): 00095 """Test that the appropriate profile parameters are passed to the 00096 Assembler""" 00097 filename = deepcopy(source_file) 00098 filename[-1] += ".s" 00099 to_compile = os.path.join(*filename) 00100 with patch('os.mkdir') as _mkdir: 00101 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00102 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00103 toolchain.inc_md5 = "" 00104 toolchain.build_dir = "" 00105 for parameter in profile['asm']: 00106 assert any(parameter in cmd for cmd in toolchain.asm), \ 00107 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00108 parameter) 00109 compile_command = toolchain.compile_command(to_compile, 00110 to_compile + ".o", []) 00111 if not compile_command: 00112 assert compile_command, to_compile 00113 for parameter in profile['asm']: 00114 assert any(parameter in cmd for cmd in compile_command), \ 00115 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00116 parameter) 00117 00118 for name, Class in TOOLCHAIN_CLASSES.items(): 00119 CLS = Class(TARGET_MAP["K64F"]) 00120 assert name == CLS.name or name == LEGACY_TOOLCHAIN_NAMES[CLS.name] 00121 00122 @given(fixed_dictionaries({ 00123 'common': lists(text()), 00124 'c': lists(text()), 00125 'cxx': lists(text()), 00126 'asm': lists(text()), 00127 'ld': lists(text(min_size=1))}), 00128 lists(text(min_size=1, alphabet=ALPHABET), min_size=1)) 00129 def test_toolchain_profile_ld (profile, source_file): 00130 """Test that the appropriate profile parameters are passed to the 00131 Linker""" 00132 filename = deepcopy(source_file) 00133 filename[-1] += ".o" 00134 to_compile = os.path.join(*filename) 00135 with patch('os.mkdir') as _mkdir,\ 00136 patch('tools.toolchains.mbedToolchain.default_cmd') as _dflt_cmd: 00137 for _, tc_class in TOOLCHAIN_CLASSES.items(): 00138 toolchain = tc_class(TARGET_MAP["K64F"], build_profile=profile) 00139 toolchain.RESPONSE_FILES = False 00140 toolchain.inc_md5 = "" 00141 toolchain.build_dir = "" 00142 for parameter in profile['ld']: 00143 assert any(parameter in cmd for cmd in toolchain.ld), \ 00144 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00145 parameter) 00146 toolchain.link(to_compile + ".elf", [to_compile], [], [], None) 00147 compile_cmd = _dflt_cmd.call_args_list 00148 if not compile_cmd: 00149 assert compile_cmd, to_compile 00150 for parameter in profile['ld']: 00151 assert any(parameter in cmd[0][0] for cmd in compile_cmd), \ 00152 "Toolchain %s did not propagate arg %s" % (toolchain.name, 00153 parameter) 00154 00155 for name, Class in TOOLCHAIN_CLASSES.items(): 00156 CLS = Class(TARGET_MAP["K64F"]) 00157 assert name == CLS.name or name == LEGACY_TOOLCHAIN_NAMES[CLS.name] 00158 00159 00160 @given(lists(text(alphabet=ALPHABET, min_size=1), min_size=1)) 00161 def test_detect_duplicates(filenames): 00162 c_sources = [os.path.join(name, "dupe.c") for name in filenames] 00163 s_sources = [os.path.join(name, "dupe.s") for name in filenames] 00164 cpp_sources = [os.path.join(name, "dupe.cpp") for name in filenames] 00165 with MagicMock() as notify: 00166 toolchain = TOOLCHAIN_CLASSES["ARM"](TARGET_MAP["K64F"], notify=notify) 00167 res = Resources() 00168 res.c_sources = c_sources 00169 res.s_sources = s_sources 00170 res.cpp_sources = cpp_sources 00171 assert res.detect_duplicates(toolchain) == 1,\ 00172 "Not Enough duplicates found" 00173 00174 _, (notification, _), _ = notify.mock_calls[1] 00175 assert "dupe.o" in notification["message"] 00176 assert "dupe.s" in notification["message"] 00177 assert "dupe.c" in notification["message"] 00178 assert "dupe.cpp" in notification["message"] 00179 00180 @given(text(alphabet=ALPHABET + ["/"], min_size=1)) 00181 @given(booleans()) 00182 @given(booleans()) 00183 @settings(max_examples=20) 00184 def test_path_specified_gcc(gcc_loc, exists_at_loc, exists_in_path): 00185 with patch('tools.toolchains.gcc.exists') as _exists: 00186 with patch('tools.toolchains.gcc.find_executable') as _find: 00187 _exists.return_value = exists_at_loc 00188 _find.return_value = exists_in_path 00189 TOOLCHAIN_PATHS['GCC_ARM'] = gcc_loc 00190 toolchain_class = TOOLCHAIN_CLASSES["GCC_ARM"] 00191 found_p = toolchain_class.check_executable() 00192 assert found_p == (exists_at_loc or exists_in_path) 00193 if exists_at_loc: 00194 assert TOOLCHAIN_PATHS['GCC_ARM'] == gcc_loc 00195 elif exists_in_path: 00196 assert TOOLCHAIN_PATHS['GCC_ARM'] == ''
Generated on Sun Jul 17 2022 08:25:18 by
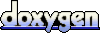