
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ThreadInterface.cpp
00001 #include "ThreadInterface.h" 00002 #include "include/thread_tasklet.h" 00003 #include "callback_handler.h" 00004 #include "mesh_system.h" 00005 #include "randLIB.h" 00006 00007 #include "ns_trace.h" 00008 #define TRACE_GROUP "nsth" 00009 00010 nsapi_error_t ThreadInterface::initialize(NanostackRfPhy *phy) 00011 { 00012 return MeshInterfaceNanostack::initialize(phy); 00013 } 00014 00015 int ThreadInterface::connect() 00016 { 00017 nanostack_lock(); 00018 00019 if (register_phy() < 0) { 00020 nanostack_unlock(); 00021 return NSAPI_ERROR_DEVICE_ERROR ; 00022 } 00023 00024 // After the RF is up, we can seed the random from it. 00025 randLIB_seed_random(); 00026 00027 mesh_error_t status = init(); 00028 if (status != MESH_ERROR_NONE) { 00029 nanostack_unlock(); 00030 return map_mesh_error(status); 00031 } 00032 00033 status = mesh_connect(); 00034 if (status != MESH_ERROR_NONE) { 00035 nanostack_unlock(); 00036 return map_mesh_error(status); 00037 } 00038 00039 // Release mutex before blocking 00040 nanostack_unlock(); 00041 00042 // In Thread wait connection for ever: 00043 // -routers will create new network and get local connectivity 00044 // -end devices will get connectivity once attached to existing network 00045 // -devices without network settings gets connectivity once commissioned and attached to network 00046 int32_t count = connect_semaphore.wait(osWaitForever); 00047 00048 if (count <= 0) { 00049 return NSAPI_ERROR_DHCP_FAILURE ; // sort of... 00050 } 00051 return 0; 00052 } 00053 00054 int ThreadInterface::disconnect() 00055 { 00056 nanostack_lock(); 00057 00058 mesh_error_t status = mesh_disconnect(); 00059 00060 nanostack_unlock(); 00061 00062 return map_mesh_error(status); 00063 } 00064 00065 mesh_error_t ThreadInterface::init() 00066 { 00067 if (eui64 == NULL) { 00068 return MESH_ERROR_PARAM; 00069 } 00070 thread_tasklet_init(); 00071 __mesh_handler_set_callback(this); 00072 thread_tasklet_device_config_set(eui64, NULL); 00073 _network_interface_id = thread_tasklet_network_init(_device_id); 00074 00075 if (_network_interface_id == -2) { 00076 return MESH_ERROR_PARAM; 00077 } else if (_network_interface_id == -3) { 00078 return MESH_ERROR_MEMORY; 00079 } else if (_network_interface_id < 0) { 00080 return MESH_ERROR_UNKNOWN; 00081 } 00082 return MESH_ERROR_NONE; 00083 } 00084 00085 bool ThreadInterface::getOwnIpAddress(char *address, int8_t len) 00086 { 00087 tr_debug("getOwnIpAddress()"); 00088 if (thread_tasklet_get_ip_address(address, len) == 0) { 00089 return true; 00090 } 00091 return false; 00092 } 00093 00094 mesh_error_t ThreadInterface::mesh_connect() 00095 { 00096 int8_t status; 00097 tr_debug("connect()"); 00098 00099 status = thread_tasklet_connect(&__mesh_handler_c_callback, _network_interface_id); 00100 00101 if (status >= 0) { 00102 return MESH_ERROR_NONE; 00103 } else if (status == -1) { 00104 return MESH_ERROR_PARAM; 00105 } else if (status == -2) { 00106 return MESH_ERROR_MEMORY; 00107 } else if (status == -3) { 00108 return MESH_ERROR_STATE; 00109 } else { 00110 return MESH_ERROR_UNKNOWN; 00111 } 00112 } 00113 00114 00115 mesh_error_t ThreadInterface::mesh_disconnect() 00116 { 00117 int8_t status; 00118 00119 status = thread_tasklet_disconnect(true); 00120 00121 if (status >= 0) { 00122 return MESH_ERROR_NONE; 00123 } 00124 00125 return MESH_ERROR_UNKNOWN; 00126 }
Generated on Sun Jul 17 2022 08:25:32 by
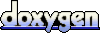