
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
TCPServer.h
00001 00002 /* TCPServer 00003 * Copyright (c) 2015 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef TCPSERVER_H 00019 #define TCPSERVER_H 00020 00021 #include "netsocket/Socket.h" 00022 #include "netsocket/TCPSocket.h" 00023 #include "netsocket/NetworkStack.h" 00024 #include "netsocket/NetworkInterface.h" 00025 #include "rtos/Semaphore.h" 00026 00027 00028 /** TCP socket server 00029 * @addtogroup netsocket 00030 */ 00031 class TCPServer : public Socket { 00032 public: 00033 /** Create an uninitialized socket 00034 * 00035 * Must call open to initialize the socket on a network stack. 00036 */ 00037 TCPServer(); 00038 00039 /** Create a socket on a network interface 00040 * 00041 * Creates and opens a socket on the network stack of the given 00042 * network interface. 00043 * 00044 * @param stack Network stack as target for socket 00045 */ 00046 template <typename S> 00047 TCPServer(S *stack) 00048 : _pending(0), _accept_sem(0) 00049 { 00050 open(stack); 00051 } 00052 00053 /** Destroy a socket 00054 * 00055 * Closes socket if the socket is still open 00056 */ 00057 virtual ~TCPServer(); 00058 00059 /** Listen for connections on a TCP socket 00060 * 00061 * Marks the socket as a passive socket that can be used to accept 00062 * incoming connections. 00063 * 00064 * @param backlog Number of pending connections that can be queued 00065 * simultaneously, defaults to 1 00066 * @return 0 on success, negative error code on failure 00067 */ 00068 nsapi_error_t listen(int backlog = 1); 00069 00070 /** Accepts a connection on a TCP socket 00071 * 00072 * The server socket must be bound and set to listen for connections. 00073 * On a new connection, creates a network socket using the specified 00074 * socket instance. 00075 * 00076 * By default, accept blocks until data is sent. If socket is set to 00077 * non-blocking or times out, NSAPI_ERROR_WOULD_BLOCK is returned 00078 * immediately. 00079 * 00080 * @param connection TCPSocket instance that will handle the incoming connection. 00081 * @param address Destination for the remote address or NULL 00082 * @return 0 on success, negative error code on failure 00083 */ 00084 nsapi_error_t accept(TCPSocket *connection, SocketAddress *address = NULL); 00085 00086 protected: 00087 virtual nsapi_protocol_t get_proto(); 00088 virtual void event(); 00089 00090 volatile unsigned _pending; 00091 rtos::Semaphore _accept_sem; 00092 }; 00093 00094 00095 #endif
Generated on Sun Jul 17 2022 08:25:32 by
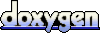