
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
SynchronizedIntegral.h
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef MBEDMICRO_RTOS_MBED_THREADS_SYNCHRONIZED_INTEGRAL 00019 #define MBEDMICRO_RTOS_MBED_THREADS_SYNCHRONIZED_INTEGRAL 00020 00021 #include <rtos.h> 00022 #include "LockGuard.h" 00023 00024 /** 00025 * Thread safe wrapper for integral types. 00026 * @tparam T type of the integral 00027 */ 00028 template<typename T> 00029 class SynchronizedIntegral { 00030 public: 00031 SynchronizedIntegral(T value) : _mutex(), _value(value) { } 00032 00033 // preincrement operator 00034 T operator++() { 00035 LockGuard lock(_mutex); 00036 return ++_value; 00037 } 00038 00039 // predecrement operator 00040 T operator--() { 00041 LockGuard lock(_mutex); 00042 return --_value; 00043 } 00044 00045 // post increment operator 00046 T operator++(int) { 00047 LockGuard lock(_mutex); 00048 return _value++; 00049 } 00050 00051 // post decrement operator 00052 T operator--(int) { 00053 LockGuard lock(_mutex); 00054 return _value--; 00055 } 00056 00057 // conversion operator, used also for <,>,<=,>=,== and != 00058 operator T() const { 00059 LockGuard lock(_mutex); 00060 return _value; 00061 } 00062 00063 // access to the internal mutex 00064 rtos::Mutex& internal_mutex() { 00065 return _mutex; 00066 } 00067 00068 private: 00069 mutable rtos::Mutex _mutex; 00070 T _value; 00071 }; 00072 00073 #endif /* MBEDMICRO_RTOS_MBED_THREADS_SYNCHRONIZED_INTEGRAL */
Generated on Sun Jul 17 2022 08:25:31 by
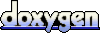