
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
SimpleAttServerMessage.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_SIMPLEATTSERVERMESSAGE_H_ 00018 #define BLE_PAL_SIMPLEATTSERVERMESSAGE_H_ 00019 00020 #include "AttServerMessage.h" 00021 00022 namespace ble { 00023 namespace pal { 00024 00025 /** 00026 * Simple implementation of ble::pal::AttFindInformationResponse. 00027 * It should fit for any vendor stack exposing a proper ATT interface. 00028 */ 00029 struct SimpleAttFindInformationResponse : public AttFindInformationResponse { 00030 /** 00031 * Format of the UUID in the response. 00032 */ 00033 enum Format { 00034 FORMAT_16_BIT_UUID = 0x01,//!< FORMAT_16_BIT_UUID 00035 FORMAT_128_BIT_UUID = 0x02//!< FORMAT_128_BIT_UUID 00036 }; 00037 00038 /** 00039 * Construct an AttFindInformationResponse from format and an array of bytes 00040 * containing the information data. 00041 * @param format The format of the information data. 00042 * @param information_data The information data whose format is determined 00043 * by the Format field 00044 */ 00045 SimpleAttFindInformationResponse( 00046 Format format, ArrayView<const uint8_t> information_data 00047 ) : AttFindInformationResponse(), 00048 _format(format), _information_data(information_data), 00049 _item_size(information_data_item_size()) { 00050 } 00051 00052 /** 00053 * @see ble::pal::AttFindInformationResponse::size 00054 */ 00055 virtual size_t size () const { 00056 return _information_data.size() / _item_size; 00057 } 00058 00059 /** 00060 * @see ble::pal::AttFindInformationResponse::operator[] 00061 */ 00062 virtual information_data_t operator[] (size_t index) const { 00063 const uint8_t* item = &_information_data[index * _item_size]; 00064 00065 information_data_t result; 00066 memcpy(&result.handle, item, sizeof(result.handle)); 00067 00068 if (_format == FORMAT_16_BIT_UUID) { 00069 uint16_t short_uuid = 0; 00070 memcpy(&short_uuid, item + 2, sizeof(short_uuid)); 00071 result.uuid = UUID(short_uuid); 00072 } else { 00073 result.uuid = UUID(item + 2, UUID::LSB); 00074 } 00075 00076 return result; 00077 } 00078 00079 private: 00080 size_t information_data_item_size() const { 00081 return sizeof(attribute_handle_t) + ((_format == FORMAT_16_BIT_UUID) ? 2 : 16); 00082 } 00083 00084 const Format _format; 00085 const ArrayView<const uint8_t> _information_data; 00086 const size_t _item_size; 00087 }; 00088 00089 00090 /** 00091 * Simple implementation of ble::pal::AttFindByTypeValueResponse. 00092 * It should fit for any vendor stack exposing a proper ATT interface. 00093 */ 00094 struct SimpleAttFindByTypeValueResponse : public AttFindByTypeValueResponse { 00095 /** 00096 * Construct a AttFindByTypeValueResponse from a raw array containing the 00097 * Handle Informations. 00098 * @param handles raw array containing one or more Handle Informations. 00099 */ 00100 SimpleAttFindByTypeValueResponse(ArrayView<const uint8_t> handles) : 00101 AttFindByTypeValueResponse(), _handles(handles) { 00102 } 00103 00104 /** 00105 * @see ble::pal::AttFindByTypeValueResponse::size 00106 */ 00107 virtual std::size_t size () const { 00108 return _handles.size() / item_size; 00109 } 00110 00111 /** 00112 * @see ble::pal::AttFindByTypeValueResponse::operator[] 00113 */ 00114 virtual attribute_handle_range_t operator[] (size_t index) const { 00115 attribute_handle_range_t result; 00116 const uint8_t* item = &_handles[index * item_size]; 00117 memcpy(&result.begin, item, sizeof(result.begin)); 00118 memcpy(&result.end, item + sizeof(result.begin), sizeof(result.end)); 00119 return result; 00120 } 00121 00122 private: 00123 static const size_t item_size = 4; 00124 const ArrayView<const uint8_t> _handles; 00125 }; 00126 00127 00128 /** 00129 * Simple implementation of ble::pal::AttReadByTypeResponse. 00130 * It should fit for any vendor stack exposing a proper ATT interface. 00131 */ 00132 struct SimpleAttReadByTypeResponse : public AttReadByTypeResponse { 00133 /** 00134 * Construct an AttReadByTypeResponse from the size of each attribute 00135 * handle-value pair and a list of attribute data. 00136 * @param element_size The size of each attribute-handle pair. 00137 * @param attribute_data Raw bytes array containing the list of attribute 00138 * data. 00139 */ 00140 SimpleAttReadByTypeResponse( 00141 uint8_t element_size, ArrayView<const uint8_t> attribute_data 00142 ) : AttReadByTypeResponse(), 00143 _attribute_data(attribute_data), _element_size(element_size) { 00144 } 00145 00146 /** 00147 * @see ble::pal::AttReadByTypeResponse::size 00148 */ 00149 virtual size_t size () const { 00150 return _attribute_data.size() / _element_size; 00151 } 00152 00153 /** 00154 * @see ble::pal::AttReadByTypeResponse::operator[] 00155 */ 00156 virtual attribute_data_t operator[] (size_t index) const { 00157 const uint8_t* item = &_attribute_data[index * _element_size]; 00158 uint16_t handle; 00159 memcpy(&handle, item, sizeof(handle)); 00160 00161 attribute_data_t result = { 00162 handle, 00163 ArrayView<const uint8_t> ( 00164 item + sizeof(handle), 00165 _element_size - sizeof(handle) 00166 ) 00167 }; 00168 00169 return result; 00170 } 00171 00172 private: 00173 ArrayView<const uint8_t> _attribute_data; 00174 uint8_t _element_size; 00175 }; 00176 00177 00178 /** 00179 * Simple implementation of ble::pal::AttReadByGroupTypeResponse. 00180 * It should fit for any vendor stack exposing a proper ATT interface. 00181 */ 00182 struct SimpleAttReadByGroupTypeResponse : public AttReadByGroupTypeResponse { 00183 /** 00184 * Construct an instance of AttReadByGroupTypeResponse from the size of each 00185 * attribute data and a byte array containing the list of attribute data. 00186 * @param element_size The size of each Attribute Data 00187 * @param attribute_data Byte array containing the list of Attribute Data. 00188 */ 00189 SimpleAttReadByGroupTypeResponse( 00190 uint8_t element_size, ArrayView<const uint8_t> attribute_data 00191 ) : AttReadByGroupTypeResponse(), 00192 _attribute_data(attribute_data), _element_size(element_size) { 00193 } 00194 00195 /** 00196 * @see ble::pal::AttReadByGroupTypeResponse::size 00197 */ 00198 virtual size_t size () const { 00199 return _attribute_data.size() / _element_size; 00200 } 00201 00202 /** 00203 * @see ble::pal::AttReadByGroupTypeResponse::operator[] 00204 */ 00205 virtual attribute_data_t operator[] (size_t index) const { 00206 const uint8_t* item = &_attribute_data[index * _element_size]; 00207 uint16_t begin; 00208 uint16_t end; 00209 00210 memcpy(&begin, item, sizeof(begin)); 00211 memcpy(&end, item + sizeof(begin), sizeof(end)); 00212 00213 attribute_data_t result = { 00214 { begin, end }, 00215 ArrayView<const uint8_t> ( 00216 item + sizeof(begin) + sizeof(end), 00217 _element_size - (sizeof(begin) + sizeof(end)) 00218 ) 00219 }; 00220 00221 return result; 00222 } 00223 00224 private: 00225 ArrayView<const uint8_t> _attribute_data; 00226 uint8_t _element_size; 00227 }; 00228 00229 } // namespace pal 00230 } // namespace ble 00231 00232 #endif /* BLE_PAL_SIMPLEATTSERVERMESSAGE_H_ */
Generated on Sun Jul 17 2022 08:25:30 by
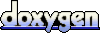