
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
SPIHalfDuplex.h
00001 /* mbed Microcontroller Library - SPIHalfDuplex 00002 * Copyright (c) 2010-2011 ARM Limited. All rights reserved. 00003 */ 00004 #ifndef MBED_SPIHALFDUPLEX_H 00005 #define MBED_SPIHALFDUPLEX_H 00006 00007 #include "platform.h" 00008 00009 #if DEVICE_SPI 00010 00011 #include "SPI.h" 00012 00013 namespace mbed { 00014 00015 /** A SPI half-duplex master, used for communicating with SPI slave devices 00016 * over a shared data line. 00017 * 00018 * The default format is set to 8-bits for both master and slave, and a 00019 * clock frequency of 1MHz 00020 * 00021 * Most SPI devies will also require Chip Select and Reset signals. These 00022 * can be controlled using <DigitalOut> pins. 00023 * 00024 * Although this is for a shared data line, both MISO and MOSI are defined, 00025 * and should be tied together externally to the mbed. This class handles 00026 * the tri-stating of the MOSI pin. 00027 * 00028 * Example: 00029 * @code 00030 * // Send a byte to a SPI half-duplex slave, and record the response 00031 * 00032 * #include "mbed.h" 00033 * 00034 * SPIHalfDuplex device(p5, p6, p7) // mosi, miso, sclk 00035 * 00036 * int main() { 00037 * int respone = device.write(0xAA); 00038 * } 00039 * @endcode 00040 */ 00041 00042 class SPIHalfDuplex : public SPI { 00043 00044 public: 00045 00046 /** Create a SPI half-duplex master connected to the specified pins 00047 * 00048 * Pin Options: 00049 * (5, 6, 7) or (11, 12, 13) 00050 * 00051 * mosi or miso can be specfied as NC if not used 00052 * 00053 * @param mosi SPI Master Out, Slave In pin 00054 * @param miso SPI Master In, Slave Out pin 00055 * @param sclk SPI Clock pin 00056 * @param name (optional) A string to identify the object 00057 */ 00058 SPIHalfDuplex(PinName mosi, PinName miso, PinName sclk); 00059 00060 /** Write to the SPI Slave and return the response 00061 * 00062 * @param value Data to be sent to the SPI slave 00063 * 00064 * @returns 00065 * Response from the SPI slave 00066 */ 00067 virtual int write(int value); 00068 00069 /** Set the number of databits expected from the slave, from 4-16 00070 * 00071 * @param sbits Number of expected bits in the slave response 00072 */ 00073 void slave_format(int sbits); 00074 00075 protected: 00076 PinName _mosi; 00077 PinName _miso; 00078 int _sbits; 00079 }; // End of class 00080 00081 } // End of namespace mbed 00082 00083 #endif 00084 00085 #endif
Generated on Sun Jul 17 2022 08:25:30 by
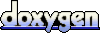