
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
NanostackEthernetInterface.cpp
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "NanostackEthernetInterface.h" 00017 #include "mesh_system.h" 00018 #include "callback_handler.h" 00019 #include "enet_tasklet.h" 00020 00021 nsapi_error_t NanostackEthernetInterface::initialize(NanostackEthernetPhy *phy) 00022 { 00023 nsapi_error_t err = MeshInterfaceNanostack::initialize(phy); 00024 if (err != NSAPI_ERROR_OK ) 00025 return err; 00026 00027 nanostack_lock(); 00028 00029 if (register_phy() < 0) { 00030 nanostack_unlock(); 00031 return NSAPI_ERROR_DEVICE_ERROR ; 00032 } 00033 00034 enet_tasklet_init(); 00035 __mesh_handler_set_callback(this); 00036 _network_interface_id = enet_tasklet_network_init(_device_id); 00037 00038 nanostack_unlock(); 00039 00040 if (_network_interface_id < 0) 00041 return MESH_ERROR_UNKNOWN; 00042 else 00043 return MESH_ERROR_NONE; 00044 } 00045 00046 int NanostackEthernetInterface::connect() 00047 { 00048 nanostack_lock(); 00049 int8_t status = enet_tasklet_connect(&__mesh_handler_c_callback, _network_interface_id); 00050 nanostack_unlock(); 00051 00052 if (status == -1) { 00053 return MESH_ERROR_PARAM; 00054 } else if (status == -2) { 00055 return MESH_ERROR_MEMORY; 00056 } else if (status == -3) { 00057 return MESH_ERROR_STATE; 00058 } else if (status != 0) { 00059 return MESH_ERROR_UNKNOWN; 00060 } 00061 00062 int32_t count = connect_semaphore.wait(30000); 00063 00064 if (count <= 0) { 00065 return NSAPI_ERROR_DHCP_FAILURE ; // sort of... 00066 } 00067 return 0; 00068 } 00069 00070 int NanostackEthernetInterface::disconnect() 00071 { 00072 enet_tasklet_disconnect(); 00073 return 0; 00074 } 00075 00076 bool NanostackEthernetInterface::getOwnIpAddress(char *address, int8_t len) 00077 { 00078 return enet_tasklet_get_ip_address(address, len)?false:true; 00079 } 00080 00081 bool NanostackEthernetInterface::getRouterIpAddress(char *address, int8_t len) 00082 { 00083 return false; 00084 }
Generated on Sun Jul 17 2022 08:25:28 by
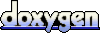