
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
MeshInterfaceNanostack.h
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MESHINTERFACENANOSTACK_H 00018 #define MESHINTERFACENANOSTACK_H 00019 #include "mbed.h" 00020 00021 #include "MeshInterface.h" 00022 #include "NanostackRfPhy.h" 00023 #include "mesh_interface_types.h" 00024 00025 class MeshInterfaceNanostack : public MeshInterface { 00026 public: 00027 00028 /** Attach phy and initialize the mesh 00029 * 00030 * Initializes a mesh interface on the given phy. Not needed if 00031 * the phy is passed to the mesh's constructor. 00032 * 00033 * @return 0 on success, negative on failure 00034 */ 00035 nsapi_error_t initialize(NanostackPhy *phy); 00036 00037 /** Start the interface 00038 * 00039 * @return 0 on success, negative on failure 00040 */ 00041 virtual nsapi_error_t connect() = 0; 00042 00043 /** Stop the interface 00044 * 00045 * @return 0 on success, negative on failure 00046 */ 00047 virtual nsapi_error_t disconnect() = 0; 00048 00049 /** Get the internally stored IP address 00050 /return IP address of the interface or null if not yet connected 00051 */ 00052 virtual const char *get_ip_address(); 00053 00054 /** Get the internally stored MAC address 00055 /return MAC address of the interface 00056 */ 00057 virtual const char *get_mac_address(); 00058 00059 /** 00060 * \brief Callback from C-layer 00061 * \param state state of the network 00062 * */ 00063 void mesh_network_handler(mesh_connection_status_t status); 00064 00065 protected: 00066 MeshInterfaceNanostack(); 00067 MeshInterfaceNanostack(NanostackPhy *phy); 00068 nsapi_error_t register_phy(); 00069 virtual NetworkStack * get_stack(void); 00070 00071 /** 00072 * \brief Read own global IP address 00073 * 00074 * \param address is where the IP address will be copied 00075 * \param len is the length of the address buffer, must be at least 40 bytes 00076 * \return true if address is read successfully, false otherwise 00077 */ 00078 virtual bool getOwnIpAddress(char *address, int8_t len) = 0; 00079 00080 NanostackPhy *phy; 00081 /** Network interface ID */ 00082 int8_t _network_interface_id; 00083 /** Registered device ID */ 00084 int8_t _device_id; 00085 uint8_t eui64[8]; 00086 char ip_addr_str[40]; 00087 char mac_addr_str[24]; 00088 Semaphore connect_semaphore; 00089 }; 00090 00091 #endif /* MESHINTERFACENANOSTACK_H */
Generated on Sun Jul 17 2022 08:25:28 by
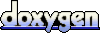