
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
LockGuard.h
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef MBEDMICRO_RTOS_MBED_THREADS_LOCK_GUARD 00019 #define MBEDMICRO_RTOS_MBED_THREADS_LOCK_GUARD 00020 00021 #include <rtos.h> 00022 00023 /** 00024 * RAII mutex locker. 00025 * The mutex pass in the constructor will be locked for the lifetime of 00026 * the LockGuard instance. 00027 */ 00028 class LockGuard { 00029 public: 00030 /** 00031 * Construct a LockGuard instance and ackire ownership of mutex in input. 00032 * @param mutex The mutex to ackire ownership of. 00033 */ 00034 LockGuard(rtos::Mutex& mutex) : _mutex(mutex) { 00035 _mutex.lock(); 00036 } 00037 00038 /** 00039 * Destruct the lock and release the inner mutex. 00040 */ 00041 ~LockGuard() { 00042 _mutex.unlock(); 00043 } 00044 00045 private: 00046 LockGuard(const LockGuard&); 00047 LockGuard& operator=(const LockGuard&); 00048 rtos::Mutex& _mutex; 00049 }; 00050 00051 #endif /* MBEDMICRO_RTOS_MBED_THREADS_LOCK_GUARD */
Generated on Sun Jul 17 2022 08:25:24 by
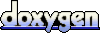