
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
LoWPANNDInterface.cpp
00001 #include "LoWPANNDInterface.h" 00002 #include "include/nd_tasklet.h" 00003 #include "callback_handler.h" 00004 #include "mesh_system.h" 00005 #include "randLIB.h" 00006 00007 #include "ns_trace.h" 00008 #define TRACE_GROUP "nslp" 00009 00010 nsapi_error_t LoWPANNDInterface::initialize(NanostackRfPhy *phy) 00011 { 00012 return MeshInterfaceNanostack::initialize(phy); 00013 } 00014 00015 int LoWPANNDInterface::connect() 00016 { 00017 nanostack_lock(); 00018 00019 if (register_phy() < 0) { 00020 nanostack_unlock(); 00021 return NSAPI_ERROR_DEVICE_ERROR ; 00022 } 00023 00024 // After the RF is up, we can seed the random from it. 00025 randLIB_seed_random(); 00026 00027 mesh_error_t status = init(); 00028 if (status != MESH_ERROR_NONE) { 00029 nanostack_unlock(); 00030 return map_mesh_error(status); 00031 } 00032 00033 status = mesh_connect(); 00034 if (status != MESH_ERROR_NONE) { 00035 nanostack_unlock(); 00036 return map_mesh_error(status); 00037 } 00038 00039 // Release mutex before blocking 00040 nanostack_unlock(); 00041 00042 // wait connection for ever 00043 int32_t count = connect_semaphore.wait(osWaitForever); 00044 00045 if (count <= 0) { 00046 return NSAPI_ERROR_DHCP_FAILURE ; // sort of... 00047 } 00048 return 0; 00049 00050 } 00051 00052 int LoWPANNDInterface::disconnect() 00053 { 00054 nanostack_lock(); 00055 00056 mesh_error_t status = mesh_disconnect(); 00057 00058 nanostack_unlock(); 00059 00060 return map_mesh_error(status); 00061 } 00062 00063 mesh_error_t LoWPANNDInterface::init() 00064 { 00065 nd_tasklet_init(); 00066 __mesh_handler_set_callback(this); 00067 _network_interface_id = nd_tasklet_network_init(_device_id); 00068 00069 if (_network_interface_id == -2) { 00070 return MESH_ERROR_PARAM; 00071 } else if (_network_interface_id == -3) { 00072 return MESH_ERROR_MEMORY; 00073 } else if (_network_interface_id < 0) { 00074 return MESH_ERROR_UNKNOWN; 00075 } 00076 return MESH_ERROR_NONE; 00077 } 00078 00079 mesh_error_t LoWPANNDInterface::mesh_connect() 00080 { 00081 int8_t status = -9; // init to unknown error 00082 tr_debug("connect()"); 00083 00084 status = nd_tasklet_connect(&__mesh_handler_c_callback, _network_interface_id); 00085 00086 if (status >= 0) { 00087 return MESH_ERROR_NONE; 00088 } else if (status == -1) { 00089 return MESH_ERROR_PARAM; 00090 } else if (status == -2) { 00091 return MESH_ERROR_MEMORY; 00092 } else if (status == -3) { 00093 return MESH_ERROR_STATE; 00094 } else { 00095 return MESH_ERROR_UNKNOWN; 00096 } 00097 } 00098 00099 mesh_error_t LoWPANNDInterface::mesh_disconnect() 00100 { 00101 int8_t status = -1; 00102 00103 status = nd_tasklet_disconnect(true); 00104 00105 if (status >= 0) { 00106 return MESH_ERROR_NONE; 00107 } 00108 00109 return MESH_ERROR_UNKNOWN; 00110 } 00111 00112 bool LoWPANNDInterface::getOwnIpAddress(char *address, int8_t len) 00113 { 00114 tr_debug("getOwnIpAddress()"); 00115 if (nd_tasklet_get_ip_address(address, len) == 0) { 00116 return true; 00117 } 00118 return false; 00119 } 00120 00121 bool LoWPANNDInterface::getRouterIpAddress(char *address, int8_t len) 00122 { 00123 tr_debug("getRouterIpAddress()"); 00124 if (nd_tasklet_get_router_ip_address(address, len) == 0) { 00125 return true; 00126 } 00127 return false; 00128 }
Generated on Sun Jul 17 2022 08:25:24 by
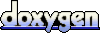