
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GenericAccessService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_GENERIC_ACCESS_SERVICE_H_ 00018 #define BLE_PAL_GENERIC_ACCESS_SERVICE_H_ 00019 00020 #include "GapTypes.h" 00021 #include "ble/ArrayView.h " 00022 #include "ble/blecommon.h" 00023 #include "ble/GapAdvertisingData.h" 00024 #include "ble/Gap.h" 00025 00026 namespace ble { 00027 namespace pal { 00028 00029 /** 00030 * Manage state of the GAP service exposed by the GATT server. 00031 * 00032 * @see Bluetooth 4.2 Vol 3 PartC: 12 - GAP service and characteristics for GATT 00033 * server. 00034 */ 00035 struct GenericAccessService { 00036 00037 /** 00038 * Empty, default, constructor 00039 */ 00040 GenericAccessService() { } 00041 00042 /** 00043 * Virtual destructor 00044 */ 00045 virtual ~GenericAccessService() { } 00046 00047 /** 00048 * Acquire the length of the device name. 00049 * The length can range from 0 (no device name) to 248 octets 00050 * 00051 * @param length The length of the device name currently stored in the GAP 00052 * service. 00053 * 00054 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00055 * otherwise. 00056 * 00057 * @see Bluetooth 4.2 Vol 3 PartC: 12.1 - Device Name Characteristic 00058 */ 00059 virtual ble_error_t get_device_name_length(uint8_t& length) = 0; 00060 00061 /** 00062 * Get the current device name. 00063 * The result is stored in the array pass in input if the operation 00064 * succeed. Prior to this call the length of the device name can be acquired 00065 * with a call to get_device_name_length. 00066 * 00067 * @param The array which will host the device name 00068 * 00069 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00070 * otherwise. 00071 * 00072 * @see Bluetooth 4.2 Vol 3 PartC: 12.1 - Device Name Characteristic 00073 */ 00074 virtual ble_error_t get_device_name(ArrayView<uint8_t>& array) = 0; 00075 00076 /** 00077 * Set the value of the device name characteristic exposed by the GAP GATT 00078 * service. 00079 * 00080 * @param device_name The name of the device. If NULL the device name 00081 * value has a length of 0. 00082 * 00083 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00084 * otherwise. 00085 * 00086 * @see Bluetooth 4.2 Vol 3 PartC: 12.1 - Device Name Characteristic 00087 */ 00088 virtual ble_error_t set_device_name(const uint8_t* device_name) = 0; 00089 00090 /** 00091 * Acquire the appearance stored in the appearance characteristic of the GAP 00092 * GATT service. 00093 * 00094 * @param appearance: If the call succeed will contain the value of the 00095 * appearance characteristic. 00096 * 00097 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00098 * otherwise. 00099 * 00100 * @see Bluetooth 4.2 Vol 3 PartC: 12.2 - Appearance Characteristic 00101 */ 00102 virtual ble_error_t get_appearance( 00103 GapAdvertisingData::Appearance& appearance 00104 ) = 0; 00105 00106 /** 00107 * Set the value of the appearance characteristic of the GAP GATT service. 00108 * 00109 * @param appearance: The appearance to set. 00110 * 00111 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00112 * otherwise. 00113 * 00114 * @see Bluetooth 4.2 Vol 3 PartC: 12.2 - Appearance Characteristic 00115 */ 00116 virtual ble_error_t set_appearance( 00117 GapAdvertisingData::Appearance appearance 00118 ) = 0; 00119 00120 /** 00121 * Acquire the peripheral prefered connection parameters stored in the GAP 00122 * GATT service. 00123 * 00124 * @param parameters: If the call succeed will contain the value of 00125 * the peripheral prefered connection parameters characteristic. 00126 * 00127 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00128 * otherwise. 00129 * 00130 * @see Bluetooth 4.2 Vol 3 PartC: 12.3 - Peripheral Preferred Connection 00131 * Parameters Characteristic 00132 */ 00133 virtual ble_error_t get_peripheral_prefered_connection_parameters( 00134 ::Gap::ConnectionParams_t& parameters 00135 ) = 0; 00136 00137 /** 00138 * set the value of the peripheral prefered connection parameters stored in 00139 * the GAP GATT service. 00140 * 00141 * @param parameters: If the peripheral prefered connection parameters 00142 * to set. 00143 * 00144 * @return BLE_ERROR_NONE in case of success or the appropriate error code 00145 * otherwise. 00146 * 00147 * @see Bluetooth 4.2 Vol 3 PartC: 12.3 - Peripheral Preferred Connection 00148 * Parameters Characteristic 00149 */ 00150 virtual ble_error_t set_peripheral_prefered_connection_parameters( 00151 const ::Gap::ConnectionParams_t& parameters 00152 ) = 0; 00153 00154 private: 00155 GenericAccessService(const GenericAccessService&); 00156 GenericAccessService& operator=(const GenericAccessService&); 00157 }; 00158 00159 } // namespace pal 00160 } // namespace ble 00161 00162 #endif /* BLE_PAL_GENERIC_ACCESS_SERVICE_H_ */
Generated on Sun Jul 17 2022 08:25:23 by
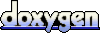