
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GattService.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_GATT_SERVICE_H__ 00018 #define MBED_GATT_SERVICE_H__ 00019 00020 #include "UUID.h " 00021 #include "GattCharacteristic.h" 00022 00023 /** 00024 * @addtogroup ble 00025 * @{ 00026 * @addtogroup gatt 00027 * @{ 00028 * @addtogroup server 00029 * @{ 00030 */ 00031 00032 /** 00033 * Representation of a GattServer service. 00034 * 00035 * A service is a cohesive collection of characteristics. It is identified by a 00036 * UUID and starts at a specific handle of its GattServer. 00037 */ 00038 class GattService { 00039 public: 00040 enum { 00041 /** 00042 * UUID of the Alert Notification service. 00043 */ 00044 UUID_ALERT_NOTIFICATION_SERVICE = 0x1811, 00045 00046 /** 00047 * UUID of the Battery service. 00048 */ 00049 UUID_BATTERY_SERVICE = 0x180F, 00050 00051 /** 00052 * UUID of the Blood Pressure service. 00053 */ 00054 UUID_BLOOD_PRESSURE_SERVICE = 0x1810, 00055 00056 /** 00057 * UUID of the Current Time service. 00058 */ 00059 UUID_CURRENT_TIME_SERVICE = 0x1805, 00060 00061 /** 00062 * UUID of the Cycling Speed and Cadence (CSC) service. 00063 */ 00064 UUID_CYCLING_SPEED_AND_CADENCE = 0x1816, 00065 00066 /** 00067 * UUID of the Device Information Service (DIS). 00068 */ 00069 UUID_DEVICE_INFORMATION_SERVICE = 0x180A, 00070 00071 /** 00072 * UUID of the environmental service. 00073 */ 00074 UUID_ENVIRONMENTAL_SERVICE = 0x181A, 00075 00076 /** 00077 * UUID of the Glucose service. 00078 */ 00079 UUID_GLUCOSE_SERVICE = 0x1808, 00080 00081 /** 00082 * UUID of the health thermometer. 00083 */ 00084 UUID_HEALTH_THERMOMETER_SERVICE = 0x1809, 00085 00086 /** 00087 * UUID of the Heart Rate service. 00088 */ 00089 UUID_HEART_RATE_SERVICE = 0x180D, 00090 00091 /** 00092 * UUID of the Human Interface Device (HID) service. 00093 */ 00094 UUID_HUMAN_INTERFACE_DEVICE_SERVICE = 0x1812, 00095 00096 /** 00097 * UUID of the Immediate Alert service. 00098 */ 00099 UUID_IMMEDIATE_ALERT_SERVICE = 0x1802, 00100 00101 /** 00102 * UUID of the Link Loss service. 00103 */ 00104 UUID_LINK_LOSS_SERVICE = 0x1803, 00105 00106 /** 00107 * UUID of the Next DST change service. 00108 */ 00109 UUID_NEXT_DST_CHANGE_SERVICE = 0x1807, 00110 00111 /** 00112 * UUID of the Phone Alert Status service. 00113 */ 00114 UUID_PHONE_ALERT_STATUS_SERVICE = 0x180E, 00115 00116 /** 00117 * UUID of the Reference Time Update service. 00118 */ 00119 UUID_REFERENCE_TIME_UPDATE_SERVICE = 0x1806, 00120 00121 /** 00122 * UUID of the Running Speed and Cadence (RSC) service. 00123 */ 00124 UUID_RUNNING_SPEED_AND_CADENCE = 0x1814, 00125 00126 /** 00127 * UUID of the Scan Parameter service. 00128 */ 00129 UUID_SCAN_PARAMETERS_SERVICE = 0x1813, 00130 00131 /** 00132 * UUID of the TX power service. 00133 */ 00134 UUID_TX_POWER_SERVICE = 0x1804 00135 }; 00136 00137 public: 00138 /** 00139 * Construct a GattService. 00140 * 00141 * @param[in] uuid The UUID assigned to this service. 00142 * @param[in] characteristics A pointer to the array of characteristics that 00143 * belongs to the service. 00144 * @param[in] numCharacteristics The number of characteristics. 00145 * 00146 * @important The characteristics of the service must remain valid while the 00147 * GattServer uses the service. 00148 */ 00149 GattService( 00150 const UUID &uuid, 00151 GattCharacteristic *characteristics[], 00152 unsigned numCharacteristics 00153 ) : 00154 _primaryServiceID(uuid), 00155 _characteristicCount(numCharacteristics), 00156 _characteristics(characteristics), 00157 _handle(0) { 00158 } 00159 00160 /** 00161 * Get this service's UUID. 00162 * 00163 * @return A reference to the service's UUID. 00164 */ 00165 const UUID &getUUID(void) const 00166 { 00167 return _primaryServiceID; 00168 } 00169 00170 /** 00171 * Get the handle of the service declaration attribute in the ATT table. 00172 * 00173 * @return The service's handle. 00174 */ 00175 uint16_t getHandle(void) const 00176 { 00177 return _handle; 00178 } 00179 00180 /** 00181 * Get the total number of characteristics within this service. 00182 * 00183 * @return The total number of characteristics within this service. 00184 */ 00185 uint8_t getCharacteristicCount(void) const 00186 { 00187 return _characteristicCount; 00188 } 00189 00190 /** 00191 * Set the handle of the service declaration attribute in the ATT table. 00192 * 00193 * @important Application code must not use this API. 00194 * 00195 * @param[in] handle The service's handle. 00196 */ 00197 void setHandle(uint16_t handle) 00198 { 00199 _handle = handle; 00200 } 00201 00202 /** 00203 * Get this service's characteristic at a specific index. 00204 * 00205 * @param[in] index The index of the characteristic. 00206 * 00207 * @return A pointer to the characteristic at index @p index. 00208 */ 00209 GattCharacteristic *getCharacteristic(uint8_t index) 00210 { 00211 if (index >= _characteristicCount) { 00212 return NULL; 00213 } 00214 00215 return _characteristics[index]; 00216 } 00217 00218 private: 00219 /** 00220 * This service's UUID. 00221 */ 00222 UUID _primaryServiceID; 00223 00224 /** 00225 * Total number of characteristics within this service. 00226 */ 00227 uint8_t _characteristicCount; 00228 00229 /** 00230 * An array with pointers to the characteristics added to this service. 00231 */ 00232 GattCharacteristic **_characteristics; 00233 00234 /** 00235 * Handle of the service declaration attribute in the ATT table. 00236 * 00237 * @note The underlying BLE stack generally assigns this handle when the 00238 * service is added to the ATT table. 00239 */ 00240 uint16_t _handle; 00241 }; 00242 00243 /** 00244 * @} 00245 * @} 00246 * @} 00247 */ 00248 00249 #endif /* ifndef MBED_GATT_SERVICE_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
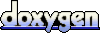